Slash Java Latency: Mastering Chronicle Queues
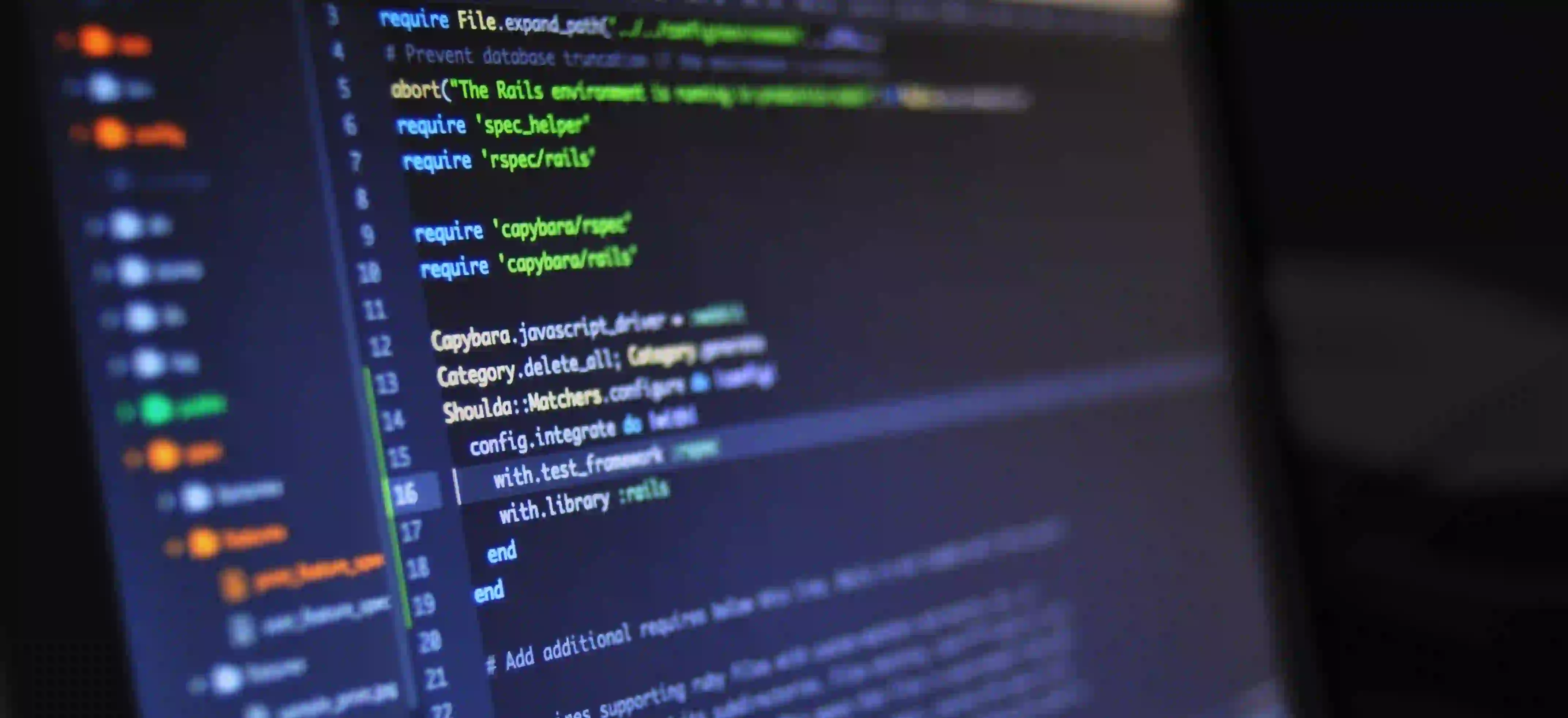
Slash Java Latency: Mastering Chronicle Queues
In the world of high-performance Java applications, minimizing latency is paramount. Whether you're building a financial trading system, a real-time analytics platform, or any other low-latency application, the ability to process messages quickly and efficiently is critical.
One tool that can help you master Java latency is Chronicle Queues. Chronicle Queues is a high-performance, low-latency messaging library that provides an efficient and reliable way to send and receive messages between threads, processes, or even different machines.
What is Chronicle Queues?
Chronicle Queues is part of the Chronicle software stack, a suite of libraries and tools designed to help developers build high-performance systems. It provides an API that allows you to create a queue of messages, which can be read and written by multiple threads or processes.
Unlike traditional message queues, Chronicle Queues keeps the messages in memory for very low latency. It also leverages off-heap memory and memory-mapped files to store the messages, reducing the amount of garbage collection overhead.
Why Choose Chronicle Queues?
There are several reasons why you might choose Chronicle Queues for your low-latency Java application:
1. Ultra-Low Latency
Chronicle Queues is designed to minimize latency as much as possible. By keeping the messages in memory, leveraging off-heap memory and memory-mapped files, and using a lock-free algorithm for multi-threaded access, it achieves ultra-low latency compared to traditional message queues.
2. High Throughput
Not only does Chronicle Queues provide low latency, but it also offers high throughput. It is capable of handling millions of messages per second, making it suitable for even the most demanding applications.
3. Support for Multi-Process Communication
Chronicle Queues offers support for communicating between different processes or even different machines. This makes it a great choice for distributed systems that need to exchange messages efficiently.
4. Flexibility and Scalability
Chronicle Queues provides a flexible API that allows you to customize the behavior of the queue to fit your specific needs. It also scales well, allowing you to handle large amounts of data without sacrificing performance.
5. Backward Compatibility
Another advantage of Chronicle Queues is its backward compatibility guarantee. Once you've built your application using Chronicle Queues, future versions of the library will ensure that it can still read the data files created by older versions.
Getting Started with Chronicle Queues
Now that we've discussed the benefits of Chronicle Queues, let's dive into how you can get started using it.
Installing Chronicle Queues
To get started with Chronicle Queues, you'll need to add the appropriate dependency to your project. You can find the latest version of Chronicle Queues on their GitHub page.
Once you have the dependency added, you're ready to start using Chronicle Queues in your Java application.
Creating a Queue
To create a Chronicle Queue, you'll need to instantiate a ChronicleQueue
object. You'll also need to specify the directory where the queue's data files will be stored.
Here's an example of how you can create a Chronicle Queue:
String queueDir = "/path/to/queue/directory";
ChronicleQueue queue = ChronicleQueue.singleBuilder(queueDir).build();
Writing Messages to the Queue
Once you have a Chronicle Queue, you can start writing messages to it. To write a message, you'll need to create a DocumentContext
object, which represents a single message in the queue.
Here's an example of how you can write a message to a Chronicle Queue:
try (DocumentContext dc = queue.acquireAppender().writingDocument()) {
// Write your message here
dc.wire().write("Hello, world!");
}
Reading Messages from the Queue
To read messages from a Chronicle Queue, you'll need to create an ExcerptTailer
object. The ExcerptTailer
provides a way to iterate over the messages in the queue.
Here's an example of how you can read messages from a Chronicle Queue:
ExcerptTailer tailer = queue.createTailer();
while (tailer.readDocument()) {
// Read and process each message
String message = tailer.wire().read().text();
System.out.println(message);
}
Closing the Queue
When you're done using a Chronicle Queue, it's important to close it properly to release any system resources it may be using.
Here's how you can close a Chronicle Queue:
queue.close();
Best Practices for Using Chronicle Queues
To get the most out of Chronicle Queues, here are some best practices to keep in mind:
1. Use Proper Threading Models
Chronicle Queues is designed to handle multi-threaded access efficiently. However, it's important to use the appropriate threading models to avoid contention and maximize performance. Consider using lock-free algorithms and thread pools to handle concurrent access to the queue.
2. Optimize for Memory Usage
Chronicle Queues leverages off-heap memory and memory-mapped files to store messages. To minimize memory usage, consider using a binary format for your messages and using fixed-length fields whenever possible.
3. Monitor Performance
Monitoring the performance of your Chronicle Queues is crucial to ensuring that your application is performing optimally. Measure the throughput and latency of your queues and use tools like Java Flight Recorder or VisualVM to profile your application and identify any performance bottlenecks.
4. Consider Compression
If your messages are large or your application is bandwidth-limited, consider compressing the messages before writing them to the Chronicle Queue. Chronicle Queues provides built-in support for compression, which can significantly reduce the amount of data that needs to be written and read.
5. Experiment and Fine-Tune
Every application is different, and what works well for one may not work as well for another. Experiment with different configurations and optimizations to find the best settings for your specific use case. Fine-tune your Chronicle Queues implementation based on your performance requirements and latency constraints.
Wrapping Up
In this blog post, we've explored Chronicle Queues and how it can help you slash Java latency in your applications. We've discussed its benefits, getting started with Chronicle Queues, best practices for usage, and the importance of monitoring and fine-tuning.
By mastering Chronicle Queues and following the best practices outlined in this post, you'll be well on your way to building high-performance, low-latency Java applications that can handle even the most demanding workloads. So go ahead, give Chronicle Queues a try and experience the power of ultra-low latency messaging for yourself!