Boost Search Speed: Mastering Lucene Index Creation
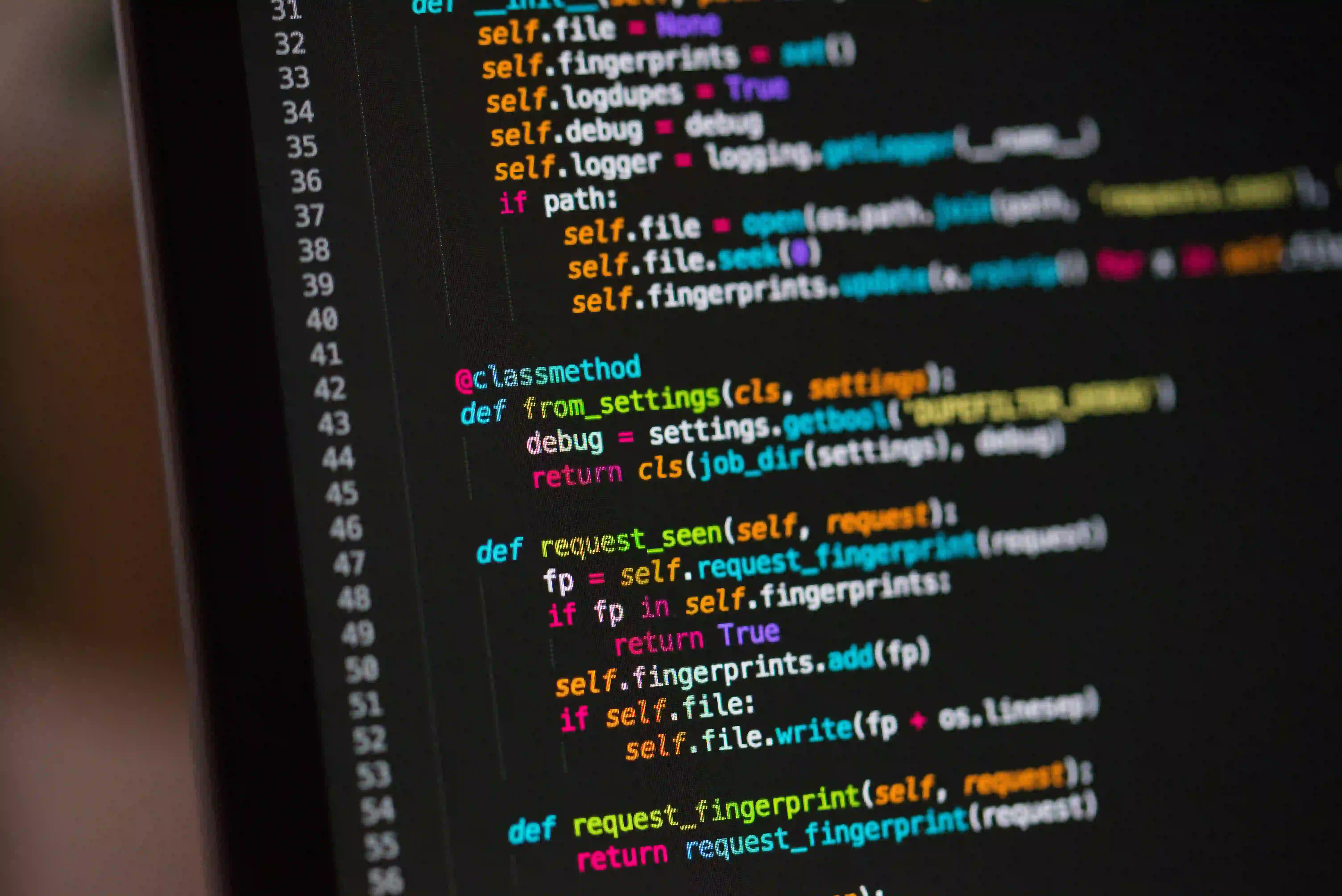
Boost Search Speed: Mastering Lucene Index Creation
In today's digital age, speed is the name of the game. We want information at our fingertips instantly, and that includes searching through vast amounts of data. If you're working with Java and need to implement powerful search functionality, Lucene is your best friend.
Lucene is an open-source, high-performance search engine library written in Java. It provides a simple and efficient way to create indexes for your search data. In this blog post, we will delve into Lucene index creation and explore the best practices to boost search speed.
Understanding Lucene Indexing
Before we dive into creating a Lucene index, let's understand what indexing actually means in the context of Lucene.
An index is a data structure that allows for quick and efficient searching and retrieval of documents. In Lucene, a document is the basic unit of information, and it consists of fields. Fields are key-value pairs that represent specific attributes of the document, such as title, author, or content.
When you create a Lucene index, you essentially create a catalog of your documents, organizing them in a way that allows for fast searching. Lucene uses inverted indexes, which store a mapping from each unique term to the documents that contain that term. This inverted index structure enables lightning-fast searches by quickly filtering documents that match a given query.
Best Practices for Index Creation
Now that we have a good understanding of Lucene indexing, let's dive into some best practices to follow when creating indexes.
1. Choose the Right Analyzer
Lucene provides a wide range of analyzers that help process your text data. An analyzer is responsible for tokenizing the text, converting it to lowercase, removing stopwords (common words like "a" or "the"), and applying stemming (reducing words to their root form).
Choosing the right analyzer that suits your data is crucial for optimal indexing. For example, if you're indexing medical records, you might want to use an analyzer that handles medical terminology well. On the other hand, if you're indexing news articles, a different analyzer may be more appropriate.
You can create custom analyzers by combining different tokenizers, filters, and char filters provided by Lucene. Experimenting with different analyzers can help you achieve the best search results for your specific use case.
2. Optimize Field Storage
When defining your document fields, you have control over how the data is stored. Lucene provides multiple options, each with its own trade-offs.
For textual data, you can choose between storing the full content, storing only parts of it (as indexed), or not storing it at all. If you don't need to access the full content after indexing, storing only the indexed form can save a significant amount of disk space.
Another important consideration is the index options. Lucene offers three levels of indexing granularity: IndexOptions.NONE
, IndexOptions.DOCS
, and IndexOptions.DOCS_AND_FREQS_AND_POSITIONS
. The more detailed the indexing, the slower the indexing process becomes, but the more flexibility you have in searching. Choose the appropriate level based on your search requirements.
3. Batch Indexing
If you have a large dataset, indexing it all at once can be resource-intensive and time-consuming. To optimize the indexing process, consider using batch indexing.
Batch indexing involves splitting your data into smaller chunks and indexing them independently. This approach allows for parallel processing, utilizing multiple threads or even distributing the indexing process across multiple machines. By splitting the workload, you can significantly reduce the indexing time and improve overall performance.
4. Avoid Frequent Commits
Lucene offers the option to commit changes to the index after each document is indexed. While this provides real-time visibility of indexed documents, it can be a major performance bottleneck. Frequent commits result in additional disk I/O operations, which can slow down the indexing process.
Instead, consider batching your commits by indexing multiple documents before committing the changes. This reduces the number of disk I/O operations and improves indexing speed.
5. Monitor and Optimize Memory Usage
Lucene heavily relies on memory for its operations. To achieve optimal performance, it's crucial to monitor and optimize memory usage during the indexing process.
One important aspect to consider is the JVM heap size. Increasing the heap size can allow for larger indexing buffers, resulting in improved performance. However, increasing the heap size too much can lead to longer garbage collection times, causing performance degradation. It's important to find the right balance for your specific use case.
Another memory-related consideration is the use of field caches. Field caches can boost search performance by preloading frequently accessed field values into memory. However, excessive use of field caches can result in excessive memory consumption. Be mindful of the field caches you choose to utilize and their impact on memory usage.
Putting it into Practice
Now that we have discussed some best practices, let's put them into practice by creating a simple Lucene index.
First, you need to add the Lucene dependency to your project. You can do this by adding the following Maven dependency:
<dependency>
<groupId>org.apache.lucene</groupId>
<artifactId>lucene-core</artifactId>
<version>8.9.0</version>
</dependency>
Next, let's create the index:
import org.apache.lucene.analysis.Analyzer;
import org.apache.lucene.analysis.standard.StandardAnalyzer;
import org.apache.lucene.document.Document;
import org.apache.lucene.document.Field;
import org.apache.lucene.document.TextField;
import org.apache.lucene.index.IndexWriter;
import org.apache.lucene.index.IndexWriterConfig;
import org.apache.lucene.store.Directory;
import org.apache.lucene.store.FSDirectory;
import java.io.IOException;
import java.nio.file.Path;
import java.nio.file.Paths;
public class LuceneIndexCreator {
public static void main(String[] args) {
String indexPath = "/path/to/index/directory";
Analyzer analyzer = new StandardAnalyzer();
try {
Directory directory = FSDirectory.open(Paths.get(indexPath));
IndexWriterConfig config = new IndexWriterConfig(analyzer);
IndexWriter indexWriter = new IndexWriter(directory, config);
// Iterate over your documents and add them to the index
for (YourDocument document : yourDocuments) {
Document luceneDocument = new Document();
luceneDocument.add(new TextField("title", document.getTitle(), Field.Store.YES));
luceneDocument.add(new TextField("content", document.getContent(), Field.Store.YES));
// Add more fields as needed
indexWriter.addDocument(luceneDocument);
}
indexWriter.commit();
indexWriter.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
In the code snippet above, we create an IndexWriter
and specify the analyzer to be used for text processing. We then iterate over our documents and create a Lucene Document
for each document, adding fields such as "title", "content", and any additional fields specific to your use case. Finally, we commit the changes and close the IndexWriter
.
Remember to handle exceptions appropriately and consider factors such as error handling and resilience when incorporating this code into your application.
Closing the Chapter
Creating an efficient Lucene index is essential for fast and accurate search functionality. By following best practices such as choosing the right analyzer, optimizing field storage, implementing batch indexing, avoiding frequent commits, and monitoring memory usage, you can significantly improve search speed.
Remember that each use case may have unique requirements, so it's important to experiment and fine-tune your Lucene indexing process accordingly. Stay up to date with the latest Lucene releases and explore additional features and optimizations to continually refine your search solution.
Now, armed with the knowledge gained from this blog post, go forth and master Lucene index creation to optimize search speed like never before!
To learn more about Lucene and its capabilities, refer to the official Lucene documentation. For a deeper understanding of Lucene internals and advanced optimization techniques, check out the book Lucene in Action.