ThreadLocal Dangers in Web Apps: Avoiding Pitfalls!
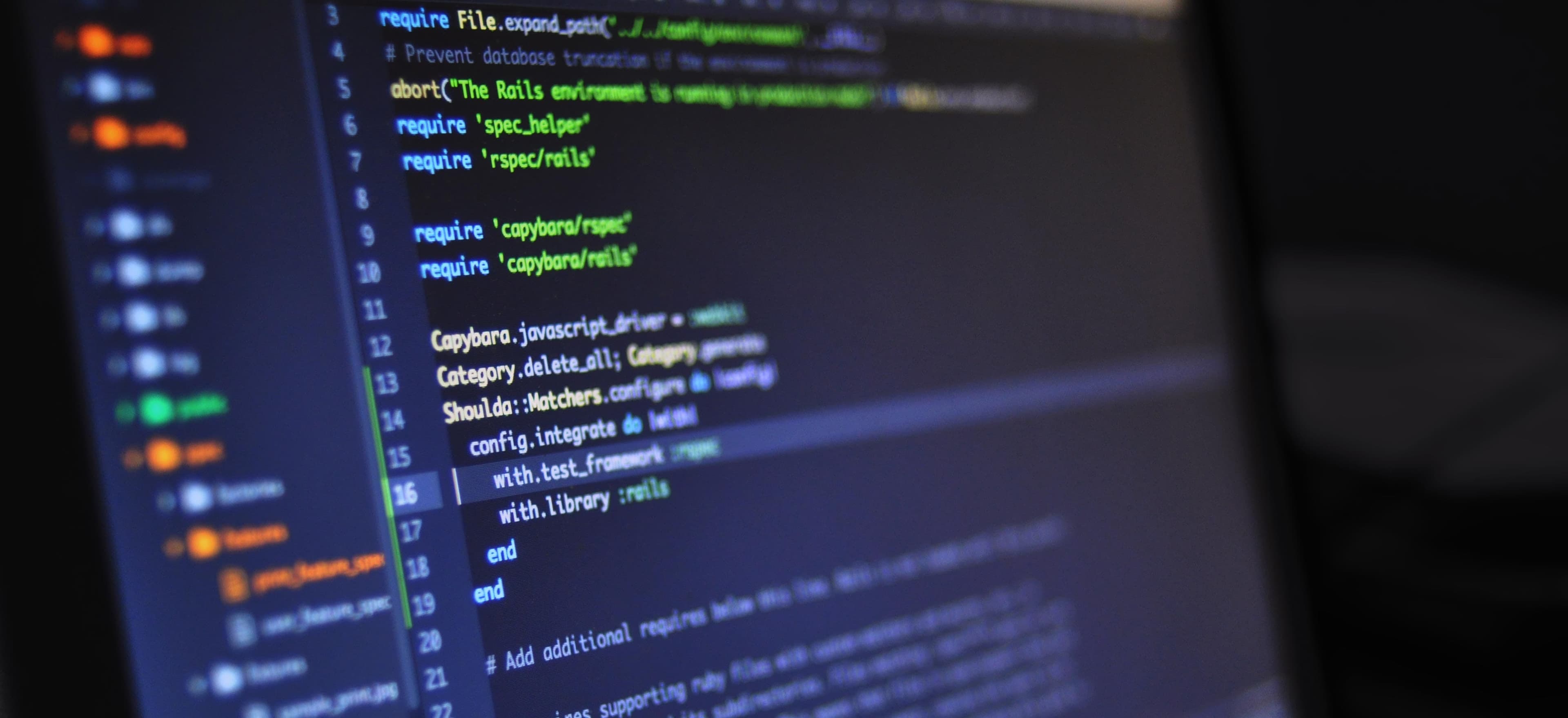
- Published on
ThreadLocal Dangers in Web Apps: Avoiding Pitfalls!
Java developers often face the challenge of managing state in a multithreaded environment. One tempting solution is the ThreadLocal
construct - an excellent tool for maintaining thread-confined variables. However, when used in web applications, specifically in settings like servlets or Spring controllers, ThreadLocal
can lead to severe issues if not handled with care. In this post, we will delve into the common pitfalls and how to mitigate them, ensuring your web app's robustness and scalability.
What is ThreadLocal?
ThreadLocal
in Java provides a way to maintain variables at a thread level, meaning that each thread accessing a ThreadLocal
variant gets its isolated copy. It's particularly useful when you need to pass information around without explicitly passing it - consider it as a form of global storage within the confines of a single thread.
Imagine ThreadLocal
as a special kind of map, where the key is the thread itself. Here's how to declare and use it:
ThreadLocal<Integer> threadLocalValue = new ThreadLocal<>();
// Set the value in a thread
threadLocalValue.set(123);
// Retrieve the value in the same thread
Integer value = threadLocalValue.get();
The Dangers of ThreadLocal in Web Apps
Web applications typically run in containers that leverage thread pools. When a request is received, a thread is assigned to it, but once the request is processed, the thread is returned to the pool for reuse. This is where the danger lies with ThreadLocal
. If not managed properly, you can end up in a situation where data "leaks" across requests.
Here’s why:
-
Thread Reuse: Since application servers reuse threads, any
ThreadLocal
variable left uncleared can be accessed by subsequent requests if it's not properly cleaned up, leading to unpredictable behavior or security issues. -
Memory Leaks: Uncollected thread-local variables can easily lead to memory leaks, especially in long-lived threads.
-
Complex Debugging: Tracking down issues caused by stray
ThreadLocal
data can be challenging, as the problematic state is not easily observable.
Best Practices for Using ThreadLocal in Web Apps
Let's look at some best practices to avoid the pitfalls associated with ThreadLocal
usage in web apps.
1. Always Clean Up After Yourself
After the use of ThreadLocal
is complete, you should always remove its contents. The best way to ensure this is by using a finally
block or try-with-resources pattern, which requires a custom AutoCloseable
wrapper.
ThreadLocal<MyObject> myThreadLocal = new ThreadLocal<>();
try {
myThreadLocal.set(new MyObject());
// ... do work with thread-local value
} finally {
myThreadLocal.remove(); // Important!
}
The remove
method ensures that the value does not linger around once the method execution is finished, thereby preventing accidental access by another thread.
2. Use with Caution in Web Environments
If you’re in a servlet environment, a safe way to use ThreadLocal
is to integrate it within an HTTP filter or Spring interceptor and clean it up at the end of the request.
public class ThreadLocalCleanupFilter implements Filter {
private static final ThreadLocal<MyObject> myThreadLocal = new ThreadLocal<>();
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain) throws IOException, ServletException {
try {
myThreadLocal.set(new MyObject());
chain.doFilter(request, response);
} finally {
myThreadLocal.remove();
}
}
// other necessary filter methods would be implemented here...
}
In this code snippet, we ensure that before a request finishes processing, ThreadLocal
data is eradicated; hence, the next request served by the same thread starts with a clean slate.
3. Scope ThreadLocal
Use to a Single Method When Possible
One way to reduce the risk of ThreadLocal
data persisting beyond its intended lifespan is by limiting its scope as much as possible.
public void someMethod() {
ThreadLocal<MyObject> myThreadLocal = new ThreadLocal<>();
try {
myThreadLocal.set(new MyObject());
// ... do work exclusively within this method scope
} finally {
myThreadLocal.remove();
}
}
By confining the ThreadLocal
within a single method, you reduce the chances of forgetting to clean up.
4. Consider Alternatives to ThreadLocal
Before opting for ThreadLocal
, explore other options like passing data through method parameters or employing more explicit state management strategies. Framework features, such as Spring's RequestContextHolder
or Java EE's @RequestScoped
, may offer safer and more transparent ways to handle request-scoped data.
Handling ThreadLocal in a Spring WebApp
For those using the Spring framework, let's walk through how you can safely implement ThreadLocal
storage with minimal risk. Spring offers several scopes (like request
, session
, and application
) that can manage bean lifecycle in respect to web requests. In most cases, these should be preferred over ThreadLocal
. Nonetheless, if you must use ThreadLocal
, here's how to do it safely:
@Component
public class SpringManagedThreadLocalBean implements DisposableBean {
private static final ThreadLocal<MyObject> myThreadLocal = new ThreadLocal<>();
public MyObject getMyObject() {
return myThreadLocal.get();
}
public void setMyObject(MyObject obj) {
myThreadLocal.set(obj);
}
@Override
public void destroy() {
myThreadLocal.remove(); // Cleanup upon bean destruction
}
}
By implementing DisposableBean
, you leverage Spring's lifecycle management to ensure ThreadLocal
is cleaned up.
Wrapping Up
While ThreadLocal
is a powerful feature for managing thread-specific data, it comes with its set of challenges—especially within web applications. Always be mindful of the lifecycle of your threads and ensure that all ThreadLocal
variables are removed when no longer needed. By following the best practices mentioned above, you can avoid common pitfalls and ensure your web application operates reliably and securely.
For further reading on ThreadLocal
and its intricacies, Oracle's official Java documentation can be an excellent starting point (ThreadLocal JavaDocs). Moreover, to understand more about managing beans in a web application context with Spring, refer to the Spring Framework Documentation on bean scopes.
Remember, with great power comes great responsibility. Use ThreadLocal
judiciously!
Happy coding!