Crack the Code: Master Debugging with These Top Tips!
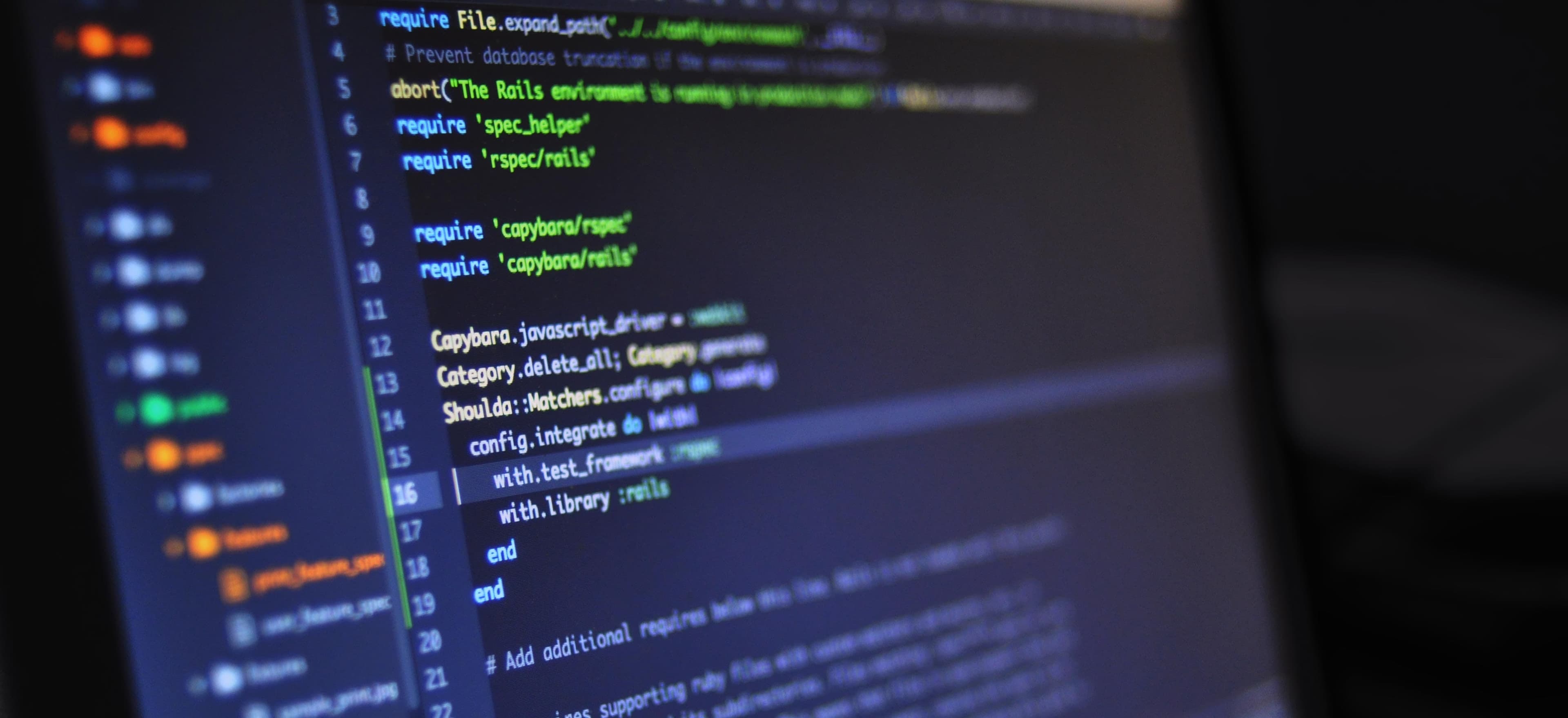
- Published on
Crack the Code: Master Debugging with These Top Tips!
Establishing the Context
Debugging is an essential skill for every Java developer. Whether you are a beginner or an experienced programmer, you will inevitably encounter bugs in your code that need to be fixed. However, debugging can be a frustrating and time-consuming process if you don't have the right approach. In this blog post, we will discuss some of the top tips and techniques that can help you become a master at debugging Java code. So let's get started!
1. Understand the Problem
Before you dive into debugging, it is crucial to fully understand the problem you are trying to solve. Take the time to read and analyze the error messages, stack traces, and any other available information. Often, these error messages provide valuable hints about what went wrong and where to look for the issue.
2. Reproduce the Bug
One of the first steps in debugging is to reproduce the bug. By replicating the issue, you can gain a better understanding of the problem and find its root cause. Try to isolate the bug by creating a minimal, self-contained example code, commonly known as a Minimal, Complete, and Verifiable Example (MCVE). This simplified code will make it easier to analyze and fix the problem.
Here's an example to illustrate this:
public class Calculator {
public int divide(int dividend, int divisor) {
return dividend / divisor;
}
}
public class Main {
public static void main(String[] args) {
Calculator calculator = new Calculator();
System.out.println(calculator.divide(10, 0));
}
}
In the above example, we have a Calculator
class with a divide
method that performs integer division. In the Main
class, we create an instance of Calculator
and try to divide 10 by 0. This will result in an ArithmeticException
with the message "java.lang.ArithmeticException: / by zero".
By running this code snippet, we can reproduce the bug and observe the error message. This simple example helps us understand the problem and focus on fixing it.
3. Use Debugging Tools
Java provides various debugging tools, such as Integrated Development Environments (IDEs) like IntelliJ IDEA, Eclipse, and NetBeans. These IDEs come with built-in debuggers that allow you to step through your code, set breakpoints, inspect variables, and view the call stack.
For example, in IntelliJ IDEA, you can set a breakpoint by clicking on the left margin of a line of code. When you run the program in debug mode, it will pause at that line, allowing you to examine the variable values and step through the code line by line.
Debugging tools can be immensely helpful in pinpointing the exact location and cause of a bug. Make sure to familiarize yourself with the debugger of your chosen IDE.
4. Print Debugging Statements
Sometimes, the power of a simple print statement cannot be underestimated. Adding print statements to your code can help you understand the program's flow and identify where the bug might be occurring.
Consider the following example:
public class Circle {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
public double calculateArea() {
double area = Math.PI * radius * radius;
System.out.println("Radius: " + radius);
System.out.println("Area: " + area);
return area;
}
}
public class Main {
public static void main(String[] args) {
Circle circle = new Circle(-5.0);
circle.calculateArea();
}
}
In the above code, we have a Circle
class with a calculateArea
method that calculates the area of a circle based on its radius. In the Main
class, we create an instance of Circle
with a negative radius (-5.0). This will result in incorrect calculations and potentially lead to unexpected behavior.
By adding print statements in the calculateArea
method, we can observe the values of radius
and area
during runtime. This helps us identify the issue and realize that the negative radius is causing the problem.
While printing debugging statements can be useful, make sure to remove or comment them out once you have fixed the bug. Leaving unnecessary print statements in your code can clutter it and make future debugging more difficult.
5. Divide and Conquer
If you are dealing with a large and complex codebase, it can be overwhelming to debug the entire system at once. In such cases, it is best to divide and conquer.
Start by narrowing down the scope of the bug. Identify the components or modules that are directly involved in the issue and focus your debugging efforts on those specific areas. By isolating the problematic sections, you can make the debugging process more manageable and efficient.
It can also be helpful to comment out or temporarily disable irrelevant code sections to narrow down the cause of the bug. Gradually reintroduce the disabled code until the problem reappears, and you can identify the exact source of the bug.
6. Make Use of Assertions
Assertions are a powerful tool for debugging and testing in Java. They allow you to specify certain conditions that must hold true at specific points in your code. If an assertion fails during runtime, an AssertionError
is thrown, indicating that something is wrong.
Here's an example:
public class Calculator {
public int divide(int dividend, int divisor) {
assert divisor != 0 : "Divisor cannot be zero!";
return dividend / divisor;
}
}
public class Main {
public static void main(String[] args) {
Calculator calculator = new Calculator();
System.out.println(calculator.divide(10, 0));
}
}
In the divide
method of the Calculator
class, we use an assertion to check if the divisor is zero. If it is, the assertion will fail, and an AssertionError
will be thrown with the specified error message.
Using assertions can help catch and report errors early during development, making it easier to identify and fix the underlying problems.
7. Consult the Documentation and Community
When stuck with a difficult bug, don't hesitate to consult the official Java documentation, forums, and online communities. Often, someone else might have encountered a similar issue and found a solution. These resources can provide valuable insights, tips, and alternative approaches to consider.
Sites like Stack Overflow are particularly useful for searching and asking specific programming questions. Just make sure to search for existing answers before posting a new question, as chances are your issue has already been addressed.
8. Use Version Control to Track Changes
Using a version control system, such as Git, can be immensely helpful in tracking code changes and identifying the introduction of bugs. By committing your code frequently and adding descriptive commit messages, you can easily identify when a bug was introduced and revert back to a working state if needed.
Additionally, you can create a new branch to isolate the debugging process from the main codebase. This allows you to experiment, make changes without affecting the working code, and discard the branch once the bug is fixed.
Wrapping Up
Debugging is an essential skill for every Java developer. By following these top tips and techniques, you can become a master at finding and fixing bugs in your code. Remember to understand the problem, reproduce the bug, use the right tools, print debugging statements, divide and conquer, make use of assertions, consult the documentation and community, and use version control to track changes.
Happy debugging! 🐞