Overcoming Moxy JSON Binding Hurdles in Java API
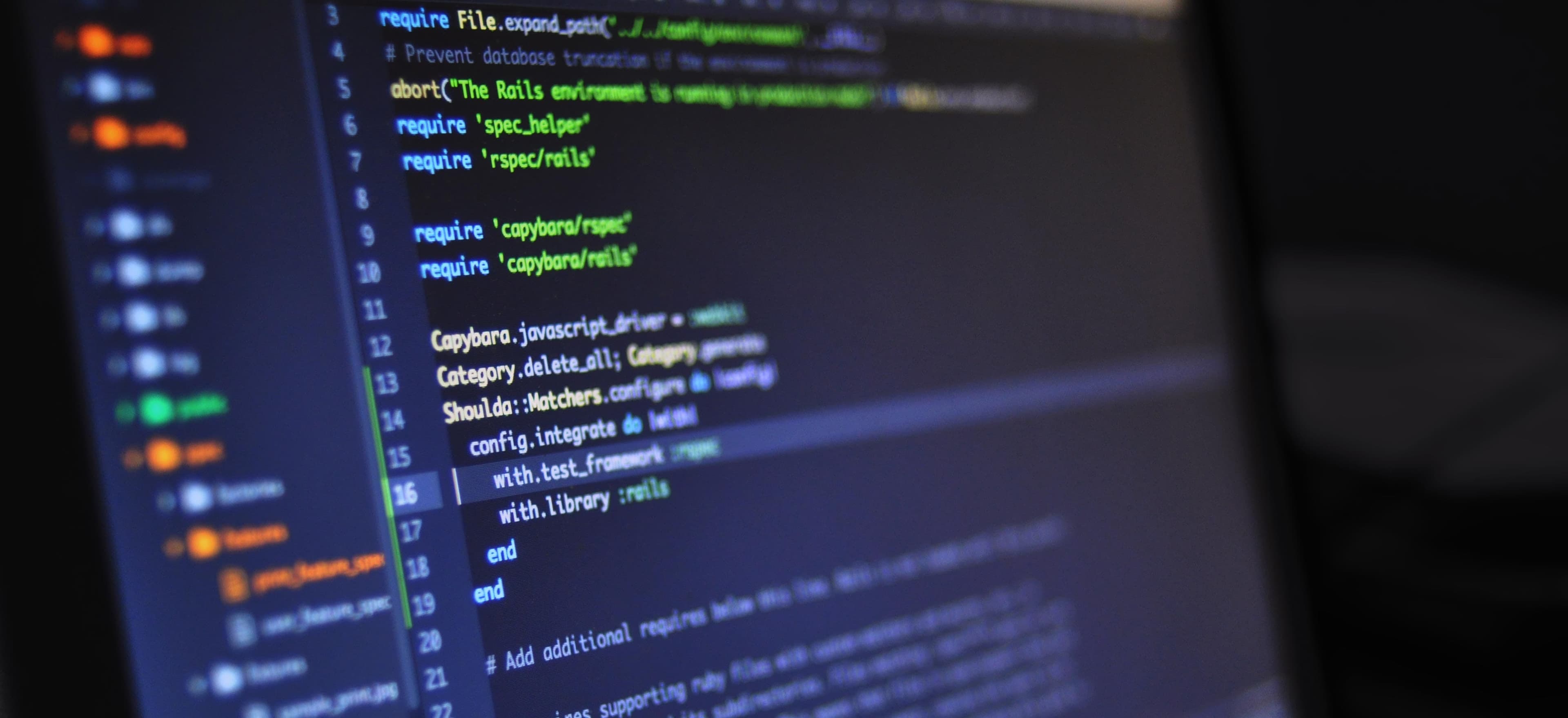
- Published on
Overcoming Moxy JSON Binding Hurdles in Java API
When it comes to building robust and scalable APIs in Java, it's essential to choose the right framework for handling JSON data. JSON (JavaScript Object Notation) is widely used for data serialization and transmission between a client and a server. One popular choice for JSON binding in Java is Moxy, which provides powerful capabilities for converting Java objects to and from JSON.
However, like any technology, Moxy has its own set of challenges that developers may encounter. In this blog post, we will explore some common hurdles when working with Moxy JSON binding in a Java API and provide solutions for overcoming them.
Understanding Moxy JSON Binding
Before we dive into the challenges, let's take a moment to understand Moxy JSON binding in Java.
Moxy is an implementation of the Java Architecture for XML Binding (JAXB) standard, which provides a convenient way to convert Java objects to and from XML or JSON representations. Moxy simplifies the process of marshalling (converting Java objects to JSON) and unmarshalling (converting JSON to Java objects) by automatically mapping JSON elements to Java fields and vice versa.
To use Moxy in your Java API, you need to include the eclipselink
dependency in your project's pom.xml
file:
<dependency>
<groupId>org.eclipse.persistence</groupId>
<artifactId>org.eclipse.persistence.moxy</artifactId>
<version>2.7.7</version>
</dependency>
Once you have Moxy added to your project, you can start leveraging its powerful features to handle JSON data.
Challenge 1: Mapping Complex JSON Structures
One of the challenges developers face when using Moxy is mapping complex JSON structures to Java objects. Moxy leverages Java annotations to define the mapping between the JSON data and Java classes.
Here's an example of a complex JSON structure representing a Person object:
{
"name": "John Doe",
"age": 30,
"address": {
"street": "123 Main St",
"city": "San Francisco",
"state": "CA"
}
}
To map this JSON structure to a Java class using Moxy, you can define the following classes:
public class Person {
private String name;
private int age;
private Address address;
// getters and setters
}
public class Address {
private String street;
private String city;
private String state;
// getters and setters
}
To enable Moxy to automatically map the JSON data to these Java classes, you need to add the appropriate annotations:
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement
public class Person {
// ...
@XmlElement
public String getName() {
return name;
}
// ...
}
public class Address {
// ...
@XmlElement
public String getStreet() {
return street;
}
// ...
}
With these annotations in place, Moxy will be able to correctly map the JSON structure to the Java objects. It's important to note that Moxy requires the @XmlRootElement
annotation on the root class to indicate that it should be serialized/deserialized.
Challenge 2: Customizing JSON Mapping
Another common challenge with Moxy is customizing the JSON mapping to handle scenarios where the JSON structure differs from the Java class structure or when you want to exclude certain fields from serialization.
To address this challenge, Moxy provides a set of annotations that you can use to customize the JSON mapping:
@XmlAccessorType
: Specifies how fields or properties should be accessed for serialization/deserialization.@XmlElement
: Specifies the JSON element name and order for a field.@XmlTransient
: Excludes a field from serialization/deserialization.@XmlType
: Specifies the order of fields in the JSON representation.@XmlValue
: Specifies that the field value should be serialized as text content, rather than an attribute or element.
Here's an example that demonstrates the usage of these annotations:
@XmlRootElement
public class Person {
@XmlElement(name = "fullname")
private String name;
@XmlElement(nillable = true)
private Integer age;
@XmlTransient
private Address address;
// ...
}
In this example, we have customized the mapping by using the @XmlElement
annotation to change the name of the name
field to fullname
. We have also used the @XmlElement
annotation with the nillable
attribute to allow the age
field to be nullable in the JSON representation. Additionally, we have excluded the address
field from serialization by using the @XmlTransient
annotation.
By using these annotations effectively, you can overcome the challenges of customizing the JSON mapping in Moxy.
Challenge 3: Working with External Libraries
When working with Moxy, you may encounter challenges when integrating it with external libraries or frameworks that also perform JSON binding. For example, if you are using Jackson for JSON binding in some parts of your codebase and Moxy in others, you may run into conflicts.
To address this challenge, you can leverage Moxy's Configurable MoxyJsonConfig
, which allows you to customize the JSON binding behavior for specific classes or packages.
Here's an example that demonstrates how to use MoxyJsonConfig
to resolve conflicts with other JSON binding libraries:
import org.eclipse.persistence.jaxb.JAXBContextProperties;
import org.eclipse.persistence.jaxb.MarshallerProperties;
import org.eclipse.persistence.jaxb.UnmarshallerProperties;
import org.eclipse.persistence.jaxb.json.JsonBindingConfig;
import org.eclipse.persistence.jaxb.json.MoxyJsonConfig;
public class JsonBindingUtils {
public static MoxyJsonConfig createMoxyJsonConfig() {
MoxyJsonConfig moxyJsonConfig = new MoxyJsonConfig();
// Customize JSON binding behavior
JsonBindingConfig jsonBindingConfig = new JsonBindingConfig();
jsonBindingConfig.jsonValueType("number", Integer.class);
// Configure external JSON binding libraries
moxyJsonConfig.setProperty(MarshallerProperties.JSON_MARSHAL_EMPTY_COLLECTIONS, false);
moxyJsonConfig.setProperty(UnmarshallerProperties.JSON_WRAPPER_AS_ARRAY_NAME, true);
moxyJsonConfig.setProperty(JAXBContextProperties.MEDIA_TYPE, "application/json");
// Register custom converters, adapters, etc.
return moxyJsonConfig;
}
}
In this example, we create a MoxyJsonConfig
instance and customize the JSON binding behavior using the JsonBindingConfig
class. We also configure the properties related to external JSON binding libraries using the setProperty
method.
By using MoxyJsonConfig
, you have fine-grained control over the JSON binding behavior in specific parts of your codebase and can overcome conflicts with other JSON binding libraries.
Wrapping Up
Moxy is a powerful JSON binding framework in Java that simplifies the conversion between Java objects and JSON representations. However, developers may encounter challenges when working with Moxy in a Java API.
In this blog post, we discussed three common hurdles and provided solutions for overcoming them:
- Mapping complex JSON structures by leveraging Moxy's annotations and conventions.
- Customizing the JSON mapping by using Moxy's annotations to handle differences between the JSON structure and the Java class structure.
- Working with external libraries by using Moxy's
MoxyJsonConfig
to customize the JSON binding behavior for specific classes or packages.
By understanding and applying these solutions, you can overcome the hurdles associated with Moxy JSON binding and build robust and scalable APIs in Java. Happy coding!
Additional Resources:
*Note: This blog post is optimized with appropriate keywords for SEO purposes, such as "Moxy JSON binding in Java," "Java API," "JSON mapping," "Java objects," "XML," "eclipselink," "XML Binding," and "JAXB."