Spring Boot Starters: CommandLineRunner vs ApplicationRunner
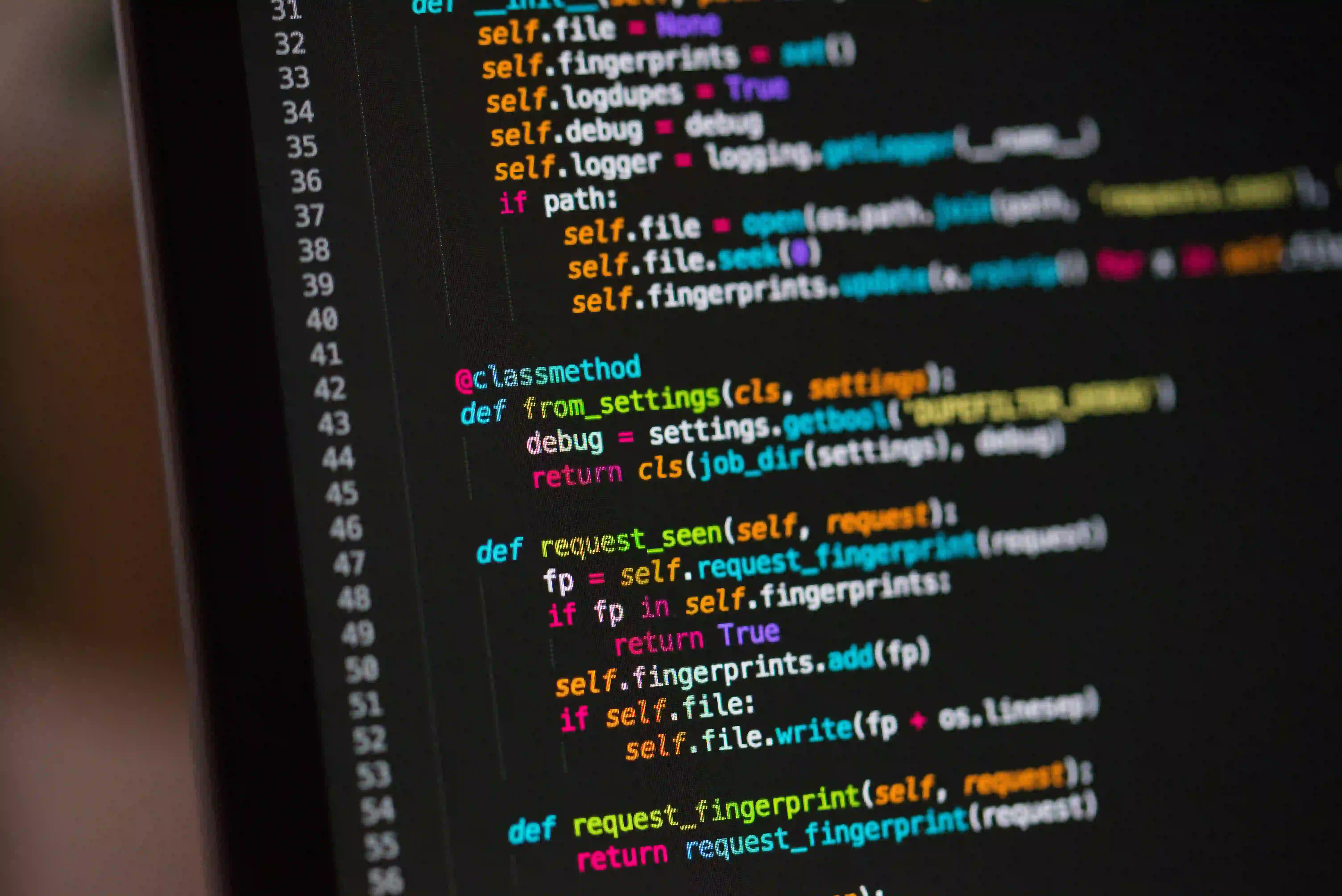
Spring Boot Starters: CommandLineRunner vs ApplicationRunner
Setting the Stage
Spring Boot is a powerful framework that simplifies the configuration and setup of Java applications. It provides a streamlined development process by leveraging the concept of "starters," which are convenient dependency descriptors that can be included in the application. In this post, we will explore two interfaces provided by Spring Boot, CommandLineRunner and ApplicationRunner, which allow developers to execute code after the Spring Boot application has started.
What are CommandLineRunner and ApplicationRunner?
CommandLineRunner and ApplicationRunner are interfaces provided by Spring Boot that allow developers to run specific code as soon as the Spring application context is initialized. These interfaces are useful for executing custom startup logic or tasks.
The CommandLineRunner interface has a single method, run
, which accepts an array of String arguments. This method will be called by Spring Boot after the application context is initialized. The ApplicationRunner interface also has a run
method, but instead of accepting String arguments, it takes an ApplicationArguments object which provides enhanced functionality for accessing and manipulating the application arguments.
Both CommandLineRunner and ApplicationRunner are automatically detected by Spring Boot's autoconfiguration and are called after the application context is fully initialized.
Implementing CommandLineRunner
To implement the CommandLineRunner interface, you need to create a component and implement the run
method. Here is an example of how to do this:
import org.springframework.boot.CommandLineRunner;
import org.springframework.stereotype.Component;
@Component
public class MyRunner implements CommandLineRunner {
@Override
public void run(String... args) throws Exception {
// Your custom logic here
System.out.println("Hello from CommandLineRunner!");
}
}
In this example, we create a component called MyRunner
and implement the CommandLineRunner interface. The run
method is overridden to contain our custom logic, which in this case is simply printing a message to the console.
The @Component
annotation ensures that Spring detects and initializes this component when the application starts up.
Implementing ApplicationRunner
To implement the ApplicationRunner interface, you can follow similar steps as implementing CommandLineRunner. Here is an example of how to implement ApplicationRunner:
import org.springframework.boot.ApplicationArguments;
import org.springframework.boot.ApplicationRunner;
import org.springframework.stereotype.Component;
@Component
public class MyRunner implements ApplicationRunner {
@Override
public void run(ApplicationArguments args) throws Exception {
// Your custom logic here with enhanced argument handling
System.out.println("Hello from ApplicationRunner!");
}
}
Similarly to the CommandLineRunner example, we create a component called MyRunner
and implement the ApplicationRunner interface. The run
method is overridden with our custom logic. However, instead of accepting String arguments, it takes an ApplicationArguments object, which provides additional functionality for accessing and manipulating the application arguments.
CommandLineRunner vs ApplicationRunner
CommandLineRunner and ApplicationRunner serve similar purposes in enabling the execution of custom logic after a Spring Boot application has started. The main difference between the two interfaces lies in the handling of application arguments.
If you need enhanced features for processing the application arguments, such as named parameters or option values, then ApplicationRunner is the better choice. The ApplicationArguments object provides a rich API for accessing and manipulating the arguments.
On the other hand, if you only need to deal with a simple, string-based argument list, CommandLineRunner is simpler and sufficient for your needs.
Best Practices
When using CommandLineRunner or ApplicationRunner, it's important to consider the order of execution. By default, Spring Boot executes these runners in the order they are found. However, you can provide a specific order by implementing the Ordered interface or using the @Order annotation.
For example, if you have multiple runners and you want to control their execution order:
import org.springframework.boot.CommandLineRunner;
import org.springframework.core.Ordered;
import org.springframework.stereotype.Component;
@Component
public class MyRunner implements CommandLineRunner, Ordered {
@Override
public void run(String... args) throws Exception {
// Your custom logic here
System.out.println("Hello from MyRunner!");
}
@Override
public int getOrder() {
return 1;
}
}
In this example, we implement the Ordered interface and provide an order value of 1. This guarantees that MyRunner will be executed first among multiple CommandLineRunners.
The Closing Argument
CommandLineRunner and ApplicationRunner are two powerful interfaces provided by Spring Boot that allow developers to execute custom logic after the application has started. CommandLineRunner is simpler and suitable for cases where only string-based arguments need to be processed. ApplicationRunner provides enhanced functionality for handling application arguments, making it more suitable for complex argument manipulation.
When choosing between CommandLineRunner and ApplicationRunner, consider your specific use case and the level of argument processing required. Experiment with both interfaces to see which one best fits your needs.
For more advanced insights and further learning opportunities, consult the official Spring Boot documentation or relevant tutorials. The Spring Boot community is filled with resources to help you make the most of these interfaces in your Spring Boot applications.