Building a JavaFX Nest-like Thermostat: Part 1
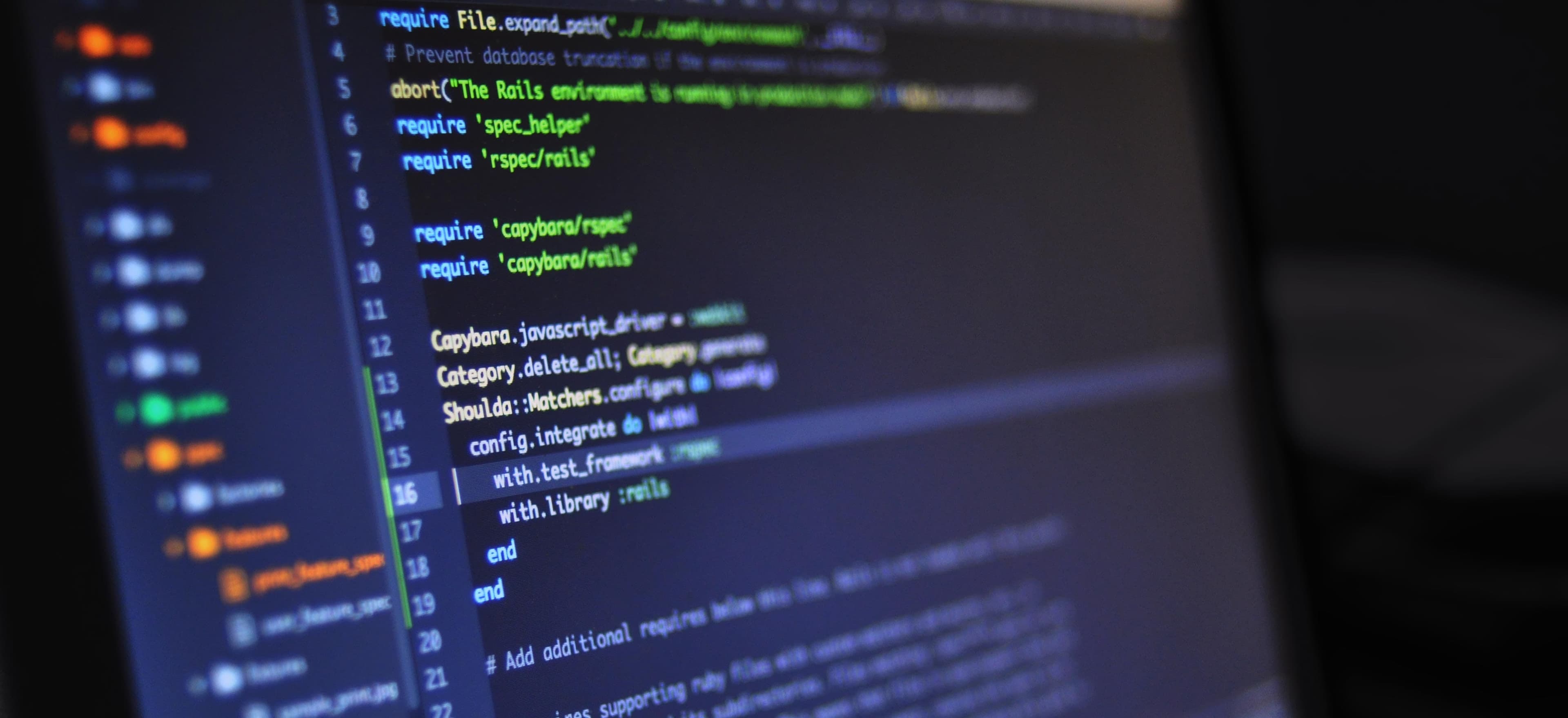
- Published on
Building a JavaFX Nest-like Thermostat: Part 1
Diving Into the Subject
In today's world of smart home devices, creating intuitive and visually appealing user interfaces (UIs) is essential. One such popular device is the Nest Thermostat, known for its sleek design and user-friendly interface. In this blog post series, we will explore how to build a JavaFX Nest-like thermostat, step by step. JavaFX is a powerful tool for creating desktop applications with modern UIs, making it a perfect choice for this project.
Why JavaFX?
Before diving into the project, let's take a moment to discuss why JavaFX is the preferred tool for modern Java UI applications. In the past, Java developers relied on UI frameworks like Swing and AWT. While they served their purpose, they lacked the rich feature set and modern capabilities that JavaFX now offers.
JavaFX stands out with its scene graph architecture, which allows for efficient rendering and smooth animations. Additionally, JavaFX provides CSS-like styling, making it easy to create visually appealing UIs. Furthermore, JavaFX supports FXML, a markup language for defining UI layouts, allowing for better separation of UI and code logic. With these features, JavaFX has rightfully taken its place as the go-to framework for UI development in Java.
Prerequisites
Before we start building our JavaFX Nest-like thermostat, let's make sure we have all the necessary prerequisites. You will need the following:
- Java Development Kit (JDK) version 8 or above
- An Integrated Development Environment (IDE) like Eclipse or IntelliJ IDEA
- JavaFX SDK, which can be downloaded from the OpenJFX website
- Scene Builder, a visual layout tool for designing UI components
Make sure you have these prerequisites installed and set up on your machine before proceeding.
Setting Up Your JavaFX Project
Now that we have all the prerequisites in place, let's set up our JavaFX project. Here's a step-by-step guide:
- Open your IDE and create a new JavaFX project.
- Configure the JavaFX SDK in your project settings. Specify the path to the JavaFX SDK you downloaded earlier.
- Structure your project into packages or modules for better organization. For this blog post series, we will structure our project into packages like
com.myproject.view
,com.myproject.model
, andcom.myproject.controller
.
To get started, here's a basic code snippet demonstrating how to create a simple JavaFX application:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class Main extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
// Create a root pane
StackPane rootPane = new StackPane();
// Create a scene using the root pane
Scene scene = new Scene(rootPane, 800, 600);
// Set the scene on the primary stage
primaryStage.setScene(scene);
// Show the primary stage
primaryStage.show();
}
}
In the code snippet above, we define a basic JavaFX application by extending the Application
class. We override the start
method to create a root pane, create a scene using the root pane, set the scene on the primary stage, and finally show the primary stage. This sets up a blank JavaFX application window.
Designing the Thermostat UI
Now that we have our JavaFX project set up, let's dive into designing the Nest-like thermostat UI. The UI will consist of various components, such as temperature displays, adjustment controls, and buttons. In this section, we will discuss the layout and design of the thermostat UI.
The Layout
The layout of the thermostat UI is crucial to achieve the desired Nest-like appearance. We will be using several JavaFX components to create the UI, including Circle
, Label
, and Button
. By utilizing these components, we can create a visually appealing and intuitive thermostat interface.
Here's an example code snippet for creating the central temperature display using a Circle
and Label
:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.layout.Pane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.stage.Stage;
public class Main extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
// Create a root pane
Pane rootPane = new Pane();
// Create a circle for the temperature display
Circle temperatureCircle = new Circle(200, 200, 150);
temperatureCircle.setFill(Color.WHITE);
temperatureCircle.setStroke(Color.BLACK);
// Create a label for the temperature value
Label temperatureLabel = new Label("75°F");
temperatureLabel.setLayoutX(200 - temperatureLabel.getWidth() / 2);
temperatureLabel.setLayoutY(200 - temperatureLabel.getHeight() / 2);
// Add the circle and label to the root pane
rootPane.getChildren().addAll(temperatureCircle, temperatureLabel);
// Create a scene using the root pane
Scene scene = new Scene(rootPane, 800, 600);
// Set the scene on the primary stage
primaryStage.setScene(scene);
// Show the primary stage
primaryStage.show();
}
}
In the code snippet above, we create a Circle
object for the temperature display and customize its appearance using setFill
and setStroke
methods. We create a Label
object for displaying the temperature value. We position the label at the center of the circle using the setLayoutX
and setLayoutY
methods. Finally, we add both the circle and the label to the root pane.
By using components like Circle
, Label
, and Pane
, we can start building our Nest-like thermostat UI.
Adding Interactivity
A good thermostat UI should be interactive and responsive to user input. In this section, we will discuss how to make the thermostat UI respond to user interactions such as touch or mouse events on the temperature adjustment controls.
In JavaFX, event handling is straightforward. We can attach event handlers to UI components and specify the actions to be performed when a particular event occurs. Let's take a look at an example code snippet for adding event handlers to the temperature controls:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.input.MouseEvent;
import javafx.scene.layout.Pane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.stage.Stage;
public class Main extends Application {
private Label temperatureLabel;
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
// Create a root pane
Pane rootPane = new Pane();
// Create a circle for the temperature control
Circle temperatureCircle = new Circle(200, 200, 150);
temperatureCircle.setFill(Color.WHITE);
temperatureCircle.setStroke(Color.BLACK);
// Create a label for the temperature value
temperatureLabel = new Label("75°F");
temperatureLabel.setLayoutX(200 - temperatureLabel.getWidth() / 2);
temperatureLabel.setLayoutY(200 - temperatureLabel.getHeight() / 2);
// Add event handlers for temperature control
temperatureCircle.setOnMouseDragged(this::handleTemperatureDrag);
// Add the circle and label to the root pane
rootPane.getChildren().addAll(temperatureCircle, temperatureLabel);
// Create a scene using the root pane
Scene scene = new Scene(rootPane, 800, 600);
// Set the scene on the primary stage
primaryStage.setScene(scene);
// Show the primary stage
primaryStage.show();
}
private void handleTemperatureDrag(MouseEvent event) {
// Get the mouse coordinates relative to the center of the temperature circle
double x = event.getX() - temperatureCircle.getCenterX();
double y = event.getY() - temperatureCircle.getCenterY();
// Calculate the angle from the mouse coordinates
double angle = Math.atan2(y, x);
// Convert the angle to a temperature value
double temperature = scaleAngleToTemperature(angle);
// Update the temperature label
temperatureLabel.setText(String.format("%.1f°F", temperature));
}
private double scaleAngleToTemperature(double angle) {
// Scale the angle to a temperature value
return angle * 100 + 75;
}
}
In the code snippet above, we use the setOnMouseDragged
method to attach an event handler to the temperature control circle. The event handler method handleTemperatureDrag
is called whenever the user moves the mouse while dragging over the circle.
Inside the event handler, we calculate the angle from the mouse coordinates relative to the center of the circle. We then convert the angle to a temperature value using the scaleAngleToTemperature
method. Finally, we update the temperature label with the new temperature value.
By implementing event handlers, we can make the thermostat UI respond to user interactions like dragging the temperature control.
Styling the UI
To make our JavaFX Nest-like thermostat resemble the sleek design of the original Nest Thermostat, applying CSS styling is essential. With JavaFX, achieving a modern and clean look is easy using CSS-like styling.
Let's take a look at an exemplary CSS code snippet that can be used to style the central temperature display:
.label {
-fx-font-size: 48px;
-fx-text-fill: white;
}
.circle {
-fx-fill: #4285F4;
-fx-stroke: #4285F4;
-fx-stroke-width: 5px;
}
In the code snippet above, we define CSS styles for the Label
and Circle
components. We set the font size and text color for the label using the -fx-font-size
and -fx-text-fill
properties. For the circle, we specify the fill color, stroke color, and stroke width using the -fx-fill
, -fx-stroke
, and -fx-stroke-width
properties, respectively.
By applying CSS styles like these, we can enhance the visual appeal and modernize the design of our thermostat UI.
Running the Thermostat Application
To see our JavaFX Nest-like thermostat in action, we need to compile and run the application. Let's go through the steps:
- Make sure you have the JavaFX SDK properly configured in your IDE.
- Compile the JavaFX application using the command-line or IDE tools.
- Run the compiled class file, specifying the main class.
java --module-path /path/to/javafx-sdk/lib --add-modules javafx.controls,javafx.fxml Main
By executing the above command, you will be able to see your JavaFX Nest-like thermostat application running.
If you encounter any issues during the compilation or execution, make sure to double-check your JDK and JavaFX SDK versions and paths. Sometimes, forgetting to include the JavaFX modules can lead to class not found errors. Troubleshoot these issues by referring to the JavaFX documentation or relevant online resources.
Final Considerations
In this first part of the Building a JavaFX Nest-like Thermostat series, we explored why JavaFX is an excellent choice for creating modern UI applications. We discussed the history of Java UI frameworks and highlighted the rich feature set, scene graph architecture, CSS-like styling, and FXML capabilities of JavaFX.
We also went through the prerequisites and set up our JavaFX project. We designed the thermostat UI by using various JavaFX components and discussed how to make the UI interactive by adding event handlers. Additionally, we explored CSS styling to enhance the visual appeal of our thermostat UI.
In the next part of this series, we will dive deeper into building the Nest-like thermostat and explore more complex features such as animation, saving the state, and connecting to a mock backend. Stay tuned!
Further Reading
To learn more about JavaFX and UI development, check out the following resources:
- JavaFX Documentation
- JavaFX Tutorial by Oracle
- JavaFX CSS Reference Guide
- JavaFX Material Design Library
- JavaFX Beginner's Guide by Oracle
Feel free to experiment with the code and UI design on your own. Don't forget to share your thoughts and questions in the comments section below. Stay tuned for Part 2 of the Building a JavaFX Nest-like Thermostat series, where we take our UI to the next level!