Java Woes: Crafting Realistic Connection Issues in Tests
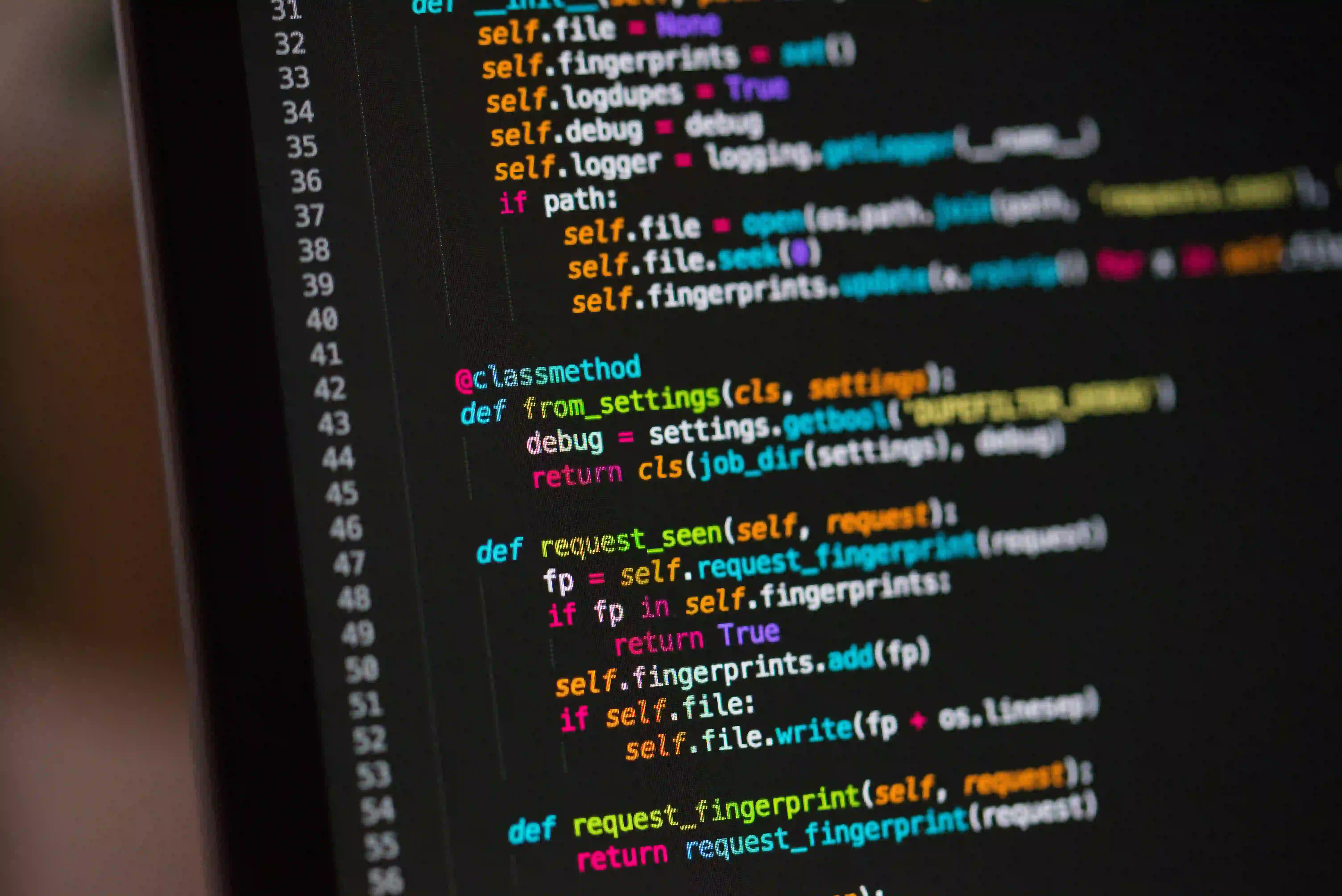
Java Woes: Crafting Realistic Connection Issues in Tests
As a Java developer, ensuring that your application behaves correctly in the face of external service failures is a key responsibility. The resilience of network connections is a common concern, one that demands rigorous testing. This post will teach you how to simulate realistic connection issues within your tests, allowing you to craft Java applications that can withstand the unpredictable nature of network environments.
Importance of Testing for Connection Issues
Connection failures are inevitable. Databases go down, APIs timeout, and packets get lost in the ether. Testing how your applications handle these failures ensures stability and builds trust with your users. It isn't enough to just write tests; your tests need to mimic the chaos of the real world.
Starting Simple: Exception Mocking
One of the simplest ways to begin is by mocking the objects that manage your network connections. If you're using an HTTP client or a database driver, you can often use a mocking framework like Mockito to ensure that exceptions are thrown under certain conditions.
import static org.mockito.Mockito.*;
// Assuming we have an HTTP client that we want to mock...
HttpClient httpClient = mock(HttpClient.class);
when(httpClient.execute(any())).thenThrow(new IOException("Simulated network failure"));
This code creates a mock HttpClient
instance, which throws an IOException
whenever it attempts to make an HTTP request. It's basic, but it allows you to test how your application reacts to network failures.
Stepping Up: Fault Injection
Mocking exceptions gets you partway there, but what about connection timeouts, slow responses, or corrupt data packets? This is where fault injection comes in. Fault injection is a testing method that involves intentionally introducing errors into a system to ensure it can cope with failure.
In Java, this can often be achieved through libraries like Toxiproxy or Chaos Monkey. These tools allow you to programmatically introduce various kinds of network faults and observe how your application handles them.
Implementing an Injected Failure
Take the next step with an example using Toxiproxy. This powerful library can simulate network conditions such as latency, disconnected connections, and more.
// Start by creating a Toxiproxy instance and a proxy
ToxiproxyClient client = new ToxiproxyClient("localhost", 8474);
Proxy proxy = client.createProxy("example", "localhost:8080", "example.com:80");
// Then apply a fault, like latency...
proxy.toxics().latency("latency", ToxicDirection.DOWNSTREAM, 1000);
// ...or simulate a connection cut-off
proxy.toxics().timeout("timeout", ToxicDirection.DOWNSTREAM, 500);
Now, when you run your tests against localhost:8080
, they’ll simulate the specified network conditions. This means your tests are now facing the delays and disconnections users might encounter in the real world.
Applying Realism to Your Tests
Real-world network issues are often erratic and unpredictable. To truly take your fault injection tests to the next level, consider adding variability and randomness to your tests.
Here's an example of how you can introduce random latency using Toxiproxy:
import java.util.Random;
// Create a random latency between 100ms and 1000ms
Random rand = new Random();
int minLatency = 100;
int maxLatency = 1000;
int randomLatency = rand.nextInt((maxLatency - minLatency) + 1) + minLatency;
proxy.toxics().latency("randomLatency", ToxicDirection.DOWNSTREAM, randomLatency);
With the code above, each test run will have different latency, providing a more robust evaluation of how well your application can handle variable network conditions.
Validating Your Application's Response
Once you have established your fault injection setup, the next step is to verify that your application reacts as expected. This often means writing assertions that confirm whether retries occur, fallbacks engage, or meaningful errors are communicated to the user.
For instance, if you're writing a web service that must fall back to a cache when a database call fails, your tests need to confirm that behavior:
@Test
public void shouldFallbackToCacheWhenDatabaseIsDown() {
// Inject fault
proxy.toxics().timeout("databaseTimeout", ToxicDirection.DOWNSTREAM, 500);
// Make a request to your service
Result result = myService.performActionThatRequiresDb();
// Assert the result comes from the fallback cache
assertTrue("Result should be from the cache", result.isFromCache());
}
This method tests the specific behavior expected when the database cannot be reached within a reasonable time frame.
Embracing Chaos in Your CI Pipeline
To maximize the efficacy of these network resilience tests, consider incorporating them into your continuous integration (CI) pipeline. Running fault injection tests alongside your regular unit and integration tests helps catch regressions and increases confidence in the robustness of your code.
Wrapping Up
Remember, testing for connection issues in Java isn't just about crafting a fail-safe system – it's about ensuring your application can gracefully handle the unexpected. By employing mocking and fault injection, you can simulate a wide range of connection problems. And by making these tests as realistic as possible, you ensure that when the inevitable occurs, your application will face it without missing a beat.
Strengthening your application against connection issues not only improves reliability but also enhances your user experience. It marks you as a developer who values resilience and reliability in software engineering. So, employ these techniques, and let your applications be the ones that stand tall when the network environment turns hostile.
For further reading:
- Learn more about mocking with Mockito here.
- Dive into fault injection testing with Toxiproxy by exploring its documentation.
Happy Testing!