Monorepo Mayhem: Streamline Your Gradle Builds!
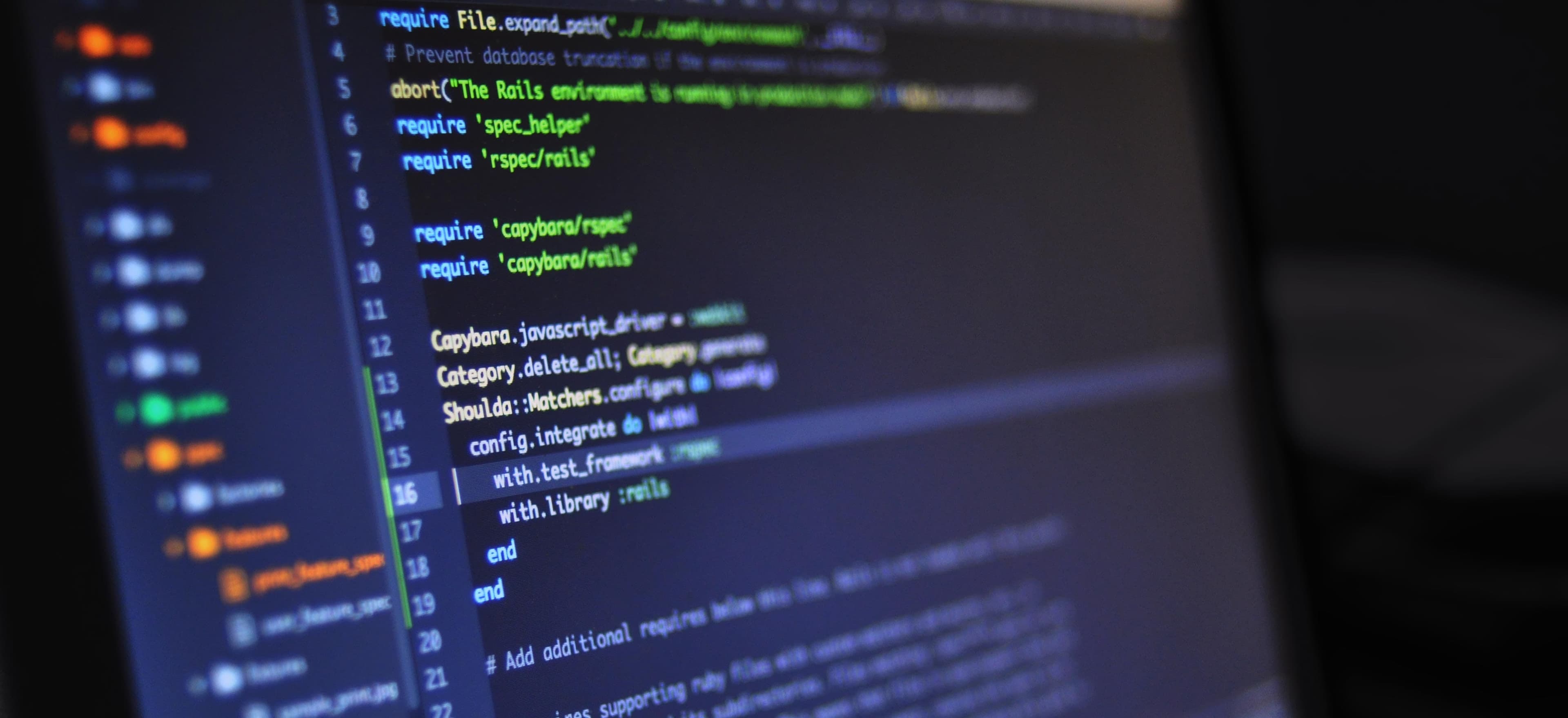
- Published on
Monorepo Mayhem: Streamline Your Gradle Builds!
Have you ever worked on a software project with multiple modules or services and found yourself struggling to manage the build process? If so, you're not alone. Many development teams face the challenge of coordinating and optimizing a complex build system, especially when working with a monorepo.
In this blog post, we will explore how to streamline your Gradle builds in a monorepo environment. We'll discuss the benefits of using a monorepo and delve into some best practices for configuring Gradle to handle multiple modules efficiently. So grab your coffee and let's dive in!
Understanding the Monorepo
Before we begin, let's define what a monorepo is. A monorepo, short for "monolithic repository," is a software development approach where all code for a project is stored in a single repository. Instead of having separate repositories for each module or service, all related code resides in one centralized location.
One of the main advantages of a monorepo is the ease of collaboration. With all code in one place, developers can easily share and modify code across modules, leading to better code reuse and faster iteration. However, managing a monorepo's complexity can be challenging, especially when it comes to build orchestration.
The Gradle Build System
Gradle is a powerful build automation tool that provides excellent support for building and managing projects with multiple modules. It uses a Groovy-based DSL (Domain-Specific Language) or Kotlin to define build scripts, making it flexible and extensible.
To get started, make sure you have Gradle installed on your machine. You can download the latest version from the official Gradle website here.
Managing Dependencies with Gradle
One of the key challenges when working with a monorepo is managing dependencies between modules. In a traditional build system, each module may have its own dependencies, and ensuring that these dependencies are resolved correctly can be a headache.
In Gradle, you can define dependencies for each module in a centralized manner using a project-level build.gradle
file. This allows you to specify common dependencies that should be used across all modules, as well as module-specific dependencies.
Let's take a look at an example build.gradle
file for a multi-module project:
// Top-level build.gradle
subprojects {
apply plugin: 'java'
repositories {
mavenCentral()
}
dependencies {
// Common dependencies
implementation 'org.springframework.boot:spring-boot-starter'
testImplementation 'junit:junit:4.12'
}
}
By applying the java
plugin and defining dependencies at the project level, Gradle will automatically apply these dependencies to all subprojects. This eliminates the need to repeat dependency declarations across module build scripts.
You can also specify module-specific dependencies by adding them to individual module build scripts. This allows you to customize the dependencies for each module while still benefiting from the centralized management.
// Module build.gradle
dependencies {
implementation project(':shared-utils')
implementation 'org.apache.commons:commons-lang3:3.12.0'
}
In this example, the module depends on another module called shared-utils
and an external library commons-lang3
. By declaring these dependencies in the module build script, Gradle will resolve them correctly during the build process.
Build Incrementality
Another challenge with a monorepo is the overall build performance. As the number of modules increases, so does the time required to build the entire project. Waiting for a full build after every change can be time-consuming and hinder developer productivity.
Gradle addresses this issue by leveraging build incrementality. Gradle tracks the changes made to the source code and only rebuilds the affected parts of the project. This significantly speeds up the build process, especially when working with a monorepo.
To benefit from build incrementality, make sure to follow good software engineering practices. Keep your modules decoupled and avoid unnecessary dependencies between them. A well-structured monorepo will help Gradle determine the exact parts of the project that need to be rebuilt, resulting in faster incremental builds.
Parallel Execution
In addition to build incrementality, Gradle also supports parallel execution of tasks. By default, Gradle executes tasks sequentially, one after the other. However, for monorepos with multiple modules, this approach may not be efficient.
Enabling parallel execution can speed up the build process by running independent tasks concurrently. Gradle automatically determines the task dependencies and ensures that tasks that depend on each other are executed in the correct order.
To enable parallel execution, add the following configuration to your settings.gradle
file:
// settings.gradle
org.gradle.parallel=true
With parallel execution enabled, Gradle will automatically utilize the available resources to execute tasks concurrently, resulting in faster builds for monorepos with multiple modules.
Build Caching
Caching build artifacts is another technique to speed up Gradle builds in a monorepo. When a build is executed, Gradle stores the compiled classes, dependencies, and other outputs in a cache directory. On subsequent builds, Gradle can reuse these cached artifacts, avoiding the need to recompile everything from scratch.
To take advantage of build caching, ensure that your Gradle configuration specifies the correct caching options. Gradle supports different caching mechanisms, such as file-based or remote build caches. By default, Gradle uses the local file system as the build cache location.
Here's an example of configuring Gradle to use the build cache:
// settings.gradle
buildCache {
local {
directory = "$rootDir/.gradle/build-cache"
}
}
In this example, we configure Gradle to use a local build cache located at ./gradle/build-cache
. Adjust the directory
value to a suitable location on your machine.
By enabling build caching, Gradle can skip the compilation of unchanged modules and reuse the cached artifacts, resulting in significant performance improvements, especially for monorepos with many modules.
Bringing It All Together
Monorepo development can streamline collaboration and promote code reuse, but it also introduces complexity when it comes to build management. By following the tips and techniques discussed in this blog post, you can effectively streamline your Gradle builds in a monorepo environment.
Remember to define dependencies at the project level, take advantage of Gradle's build incrementality, enable parallel execution for faster builds, and leverage build caching to avoid unnecessary recompilation.
Gradle provides a robust and flexible platform for managing monorepo builds, and with the right configuration, you can optimize your development process and improve overall productivity.
For more information on Gradle and its features, visit the official Gradle documentation here.
Happy coding!