Mastering JUnit & Mockito: Solving Test Dependency Woes
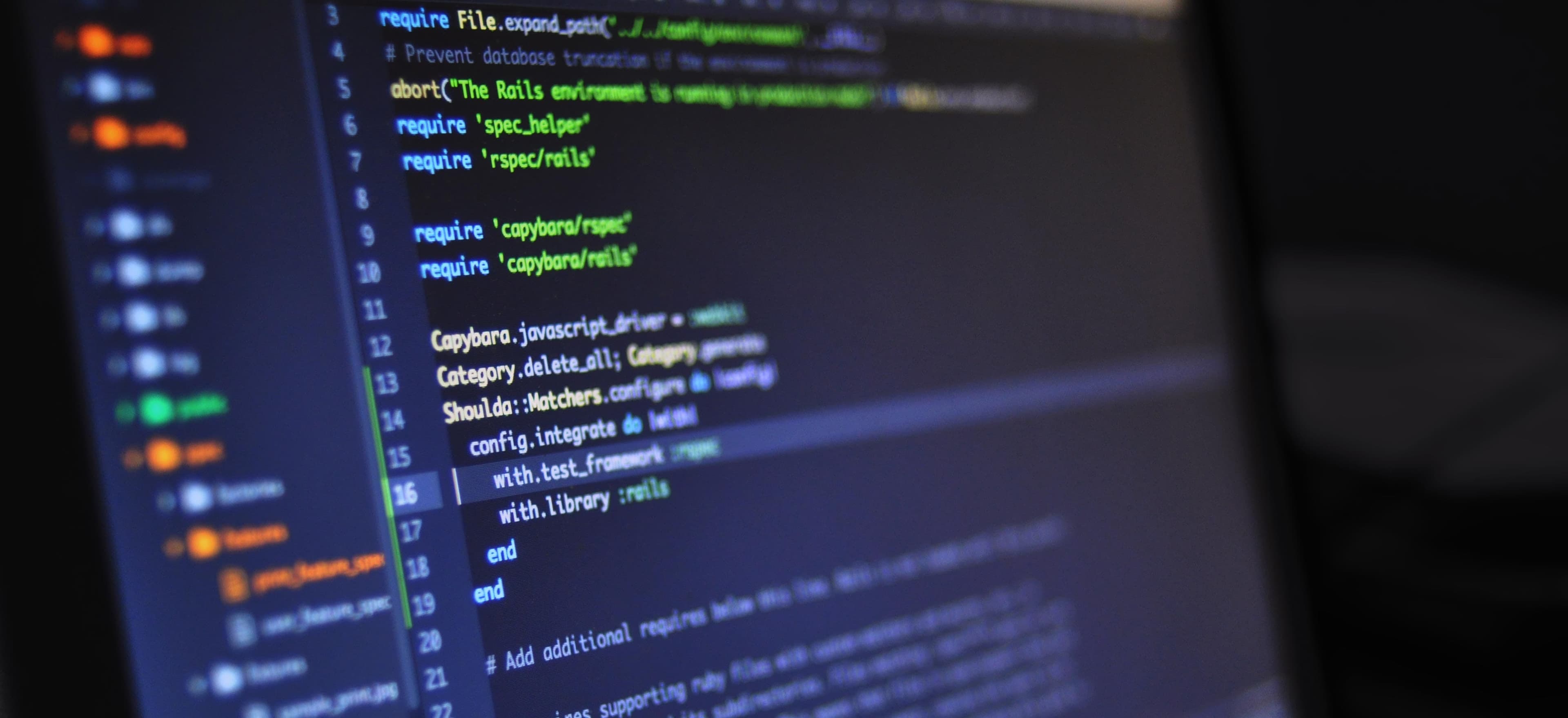
- Published on
Mastering JUnit & Mockito: Solving Test Dependency Woes
Unit testing is an essential part of any software development process. It allows developers to ensure that the code they write works as expected, reducing the probability of introducing bugs and improving maintainability. However, unit testing can become rather challenging when it comes to dealing with test dependencies. This is where JUnit and Mockito come in, providing powerful tools to overcome these obstacles. In this blog post, we will explore how to master JUnit and Mockito to solve test dependency woes.
Test Dependency Woes
Before diving into JUnit and Mockito, let's understand what test dependencies are and why they can become problematic.
Test dependencies occur when a unit test relies on external resources such as databases, web services, or file systems. These resources may not always be available during the test execution, making it challenging to guarantee consistent and reliable test results. Additionally, test dependencies can slow down the overall test execution time, making the testing process less efficient.
JUnit: The Test Framework
JUnit is a popular Java test framework that provides a rich set of annotations and assertions to write unit tests. It enables developers to define test cases, execute them, and assert the expected behavior of the code being tested.
Writing JUnit Tests
Let's start by writing a simple JUnit test for a hypothetical Calculator
class that performs basic mathematical operations.
import org.junit.jupiter.api.Assertions;
import org.junit.jupiter.api.Test;
class CalculatorTest {
@Test
void testAddition() {
Calculator calculator = new Calculator();
int result = calculator.add(2, 3);
Assertions.assertEquals(5, result);
}
}
In this example, we import the necessary JUnit classes and annotate the test method with @Test
. The testAddition
method creates an instance of the Calculator
class, calls the add
method with two numbers (2 and 3), and asserts that the result is equal to 5 using Assertions.assertEquals
. If the assertion fails, JUnit will mark the test as failed.
Running JUnit Tests
To run the JUnit tests, we can use popular build tools like Maven or Gradle. For example, in Maven, we can execute the tests by running the command mvn test
. This will compile the code, run the tests, and display the test results.
$ mvn test
...
-------------------------------------------------------
T E S T S
-------------------------------------------------------
Running CalculatorTest
Tests run: 1, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.015 s - in CalculatorTest
Results:
Tests run: 1, Failures: 0, Errors: 0, Skipped: 0
...
JUnit makes it easy to execute tests and provides detailed information about the test results, helping identify any issues with the code being tested.
Handling Test Dependencies with Mockito
Now, let's move on to using Mockito, a popular mocking framework that can help us solve test dependency woes.
Mockito allows us to create mock objects that simulate the behavior of real dependencies. By using mocks, we can isolate the code under test and avoid the need for real external resources.
To illustrate the usage of Mockito, let's consider a scenario where our Calculator
class uses an external MathService
to perform complex mathematical operations.
class Calculator {
private MathService mathService;
public Calculator(MathService mathService) {
this.mathService = mathService;
}
public int performComplexCalculation(int a, int b) {
// Complex calculation implementation using mathService
}
}
In the above example, the Calculator
class has a constructor that accepts an instance of MathService
. The performComplexCalculation
method performs some complex calculation using the mathService
.
To test the Calculator
class, we need a mock MathService
instead of a real implementation. This is where Mockito comes into play.
Using Mockito to Create Mock Objects
Mockito provides a simple and intuitive API to create mock objects. Let's see how we can use Mockito to create a mock MathService
and test the Calculator
class.
import org.mockito.Mockito;
class CalculatorTest {
@Test
void testPerformComplexCalculation() {
MathService mathServiceMock = Mockito.mock(MathService.class);
Calculator calculator = new Calculator(mathServiceMock);
Mockito.when(mathServiceMock.add(2, 3)).thenReturn(5);
int result = calculator.performComplexCalculation(2, 3);
Assertions.assertEquals(5, result);
Mockito.verify(mathServiceMock).add(2, 3);
}
}
In this example, we use the Mockito.mock
method to create a mock MathService
object. We then pass this mock instance to the Calculator
class constructor.
Next, we use Mockito.when
to configure the behavior of the mock for the add
method. We specify that when the add
method is called with arguments 2 and 3, it should return 5. This way, we simulate the behavior of the real MathService
without actually calling it.
Finally, we invoke the performComplexCalculation
method and assert that the result is equal to 5. We also use Mockito.verify
to verify that the add
method of the mock MathService
was called with the expected arguments.
Benefits of Using Mockito
Using Mockito for creating mock objects brings several benefits to the table:
-
Isolation: Mock objects isolate the code under test by replacing real dependencies with simulated ones. This helps to focus on the specific behavior being tested without worrying about external components.
-
Control: With Mockito, we have full control over the behavior of the mock objects. We can specify the return values, throw exceptions, or verify method invocations, allowing us to test various scenarios easily.
-
Efficiency: Mock objects are lightweight and fast, reducing the execution time of the unit tests. This improves the overall efficiency of the testing process.
Closing the Chapter
Unit testing is crucial for building robust and reliable software. However, test dependencies can pose challenges when verifying the behavior of code that relies on external resources. JUnit and Mockito provide powerful tools to overcome these test dependency woes.
In this blog post, we explored how to write JUnit tests for basic functionality and learned how to use Mockito to create mock objects and simulate the behavior of external dependencies. We also discussed the benefits of using Mockito, such as isolation, control, and efficiency.
By mastering JUnit and Mockito, you can write effective unit tests that are capable of handling test dependency issues, leading to more reliable and maintainable code. So go ahead, start exploring JUnit and Mockito, and take your unit testing skills to the next level!
If you'd like to dive deeper into JUnit and Mockito, here are some additional resources:
- JUnit User Guide
- Mockito Documentation
- Testing in Java: JUnit vs. TestNG
Happy testing!