Mastering Juzu Portlets: Avoid This Common eXo Pitfall!
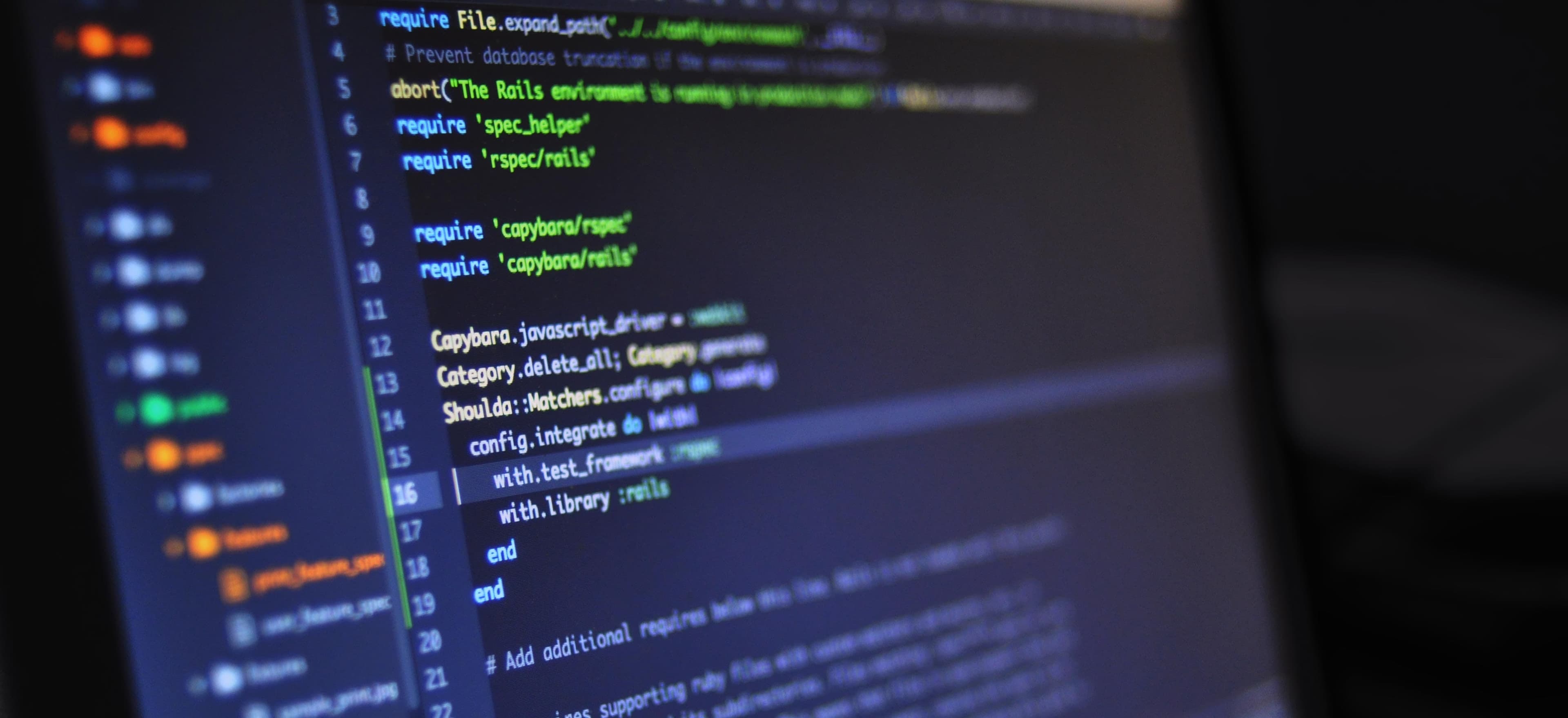
- Published on
Mastering Juzu Portlets: Avoid This Common eXo Pitfall!
In the realm of enterprise portal platforms, eXo Platform stands out with its robust framework and the ability to extend its functionality through portlets. One of the most modern and efficient ways to develop portlets for eXo Platform is by using Juzu, a web framework that simplifies writing MVC applications. However, for many Java developers, especially those new to eXo Platform, a common pitfall awaits when venturing into Juzu portlet development. This blog post aims to navigate you through best practices and troubleshooting common issues to master Juzu portlets and optimize your eXo experience.
Understanding Juzu Framework
Before diving into the nitty-gritty details, let's establish a clear understanding of the Juzu framework. Juzu (Juzu Web Framework) provides a straightforward approach to writing MVC-based portlet applications that run seamlessly on eXo Platform. With Juzu, developers can focus on creating functionality rather than fussing over boilerplate code, due to its ability to define routes, inject dependencies, and render views with minimal effort.
Setting Up the Development Environment
Getting started with Juzu requires setting up your development environment properly. You need Maven for dependency management and building the project, and an IDE like Eclipse or IntelliJ IDEA for writing and maintaining your code. For step-by-step instructions to set up your environment, visit the eXo Platform Documentation.
Once your environment is ready, you can create a Juzu portlet project using Maven archetypes provided by eXo. Here's an example command to generate a Juzu project:
mvn archetype:generate \
-DarchetypeGroupId=org.exoplatform.platform \
-DarchetypeArtifactId=maven-juzu-archetype \
-DarchetypeVersion=LATEST
The Common Pitfall in Juzu Portlet Development
When crafting Juzu portlets for eXo Platform, developers often stumble upon an issue: application scope loss. This occurs when you expect to maintain state across user interactions but find that your portlet behaves as if it’s resetting each time.
The culprit? Incorrect use of Scopes.
To understand Scopes in Juzu, we need to know that Juzu has @SessionScoped
and @RequestScoped
annotations to control the lifecycle of beans. Here's where the "why" comes into play:
- @SessionScoped: Beans are alive and well for the user's entire session. This is where you keep user-specific state information across multiple interactions.
- @RequestScoped: These beans are short-lived and persist only for the duration of a single HTTP request. They do not retain state after the request is completed.
Ensure you use the correct scope by assessing whether your data should survive beyond the current request.
import javax.inject.Named;
import org.juzu.Scope;
import org.juzu.inject.ProviderFactory;
@Named
@SessionScoped
public class UserData {
private String username;
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
}
In the example above, UserData
is a @SessionScoped
bean, meaning that once setUsername
is called, the username will be accessible throughout the user's interaction with the eXo application until the session is destroyed.
Avoiding the Scope Confusion
The key to mastering Juzu portlets is understanding when to use each scope. Here's a tip to avoid this common pitfall: always start with @RequestScoped
, and only upgrade to @SessionScoped
if the state must persist across multiple user interactions or HTTP requests.
What happens if you get it wrong? Well, using @SessionScoped
indiscriminately can lead to memory leakage and session clutter with needless data. That equates to reduced application performance and a poor user experience. Conversely, overusing @RequestScoped
can prevent your application from remembering the user's actions from one request to another.
To illustrate, here's a snippet using a @RequestScoped
bean:
import javax.inject.Named;
@Named
@RequestScoped
public class RequestData {
private String requestId;
public String getRequestId() {
return requestId;
}
public void setRequestId(String requestId) {
this.requestId = requestId;
}
}
This bean would be ideal for keeping track of a request-specific piece of data, such as an authentication token or a transaction identifier, which doesn't need to be remembered once the request is finished.
Dependency Injection
Another tremendous advantage of Juzu is its support for dependency injection out-of-the-box. Dependency injection in Juzu allows for easy management of portlet dependencies, promoting loose coupling and testability. It integrates with popular DI frameworks like Spring and Guice, making it easy to inject services into your portlets.
Here’s a quick example of how you can inject an @ApplicationScoped
service into your Juzu controller:
import org.juzu.Path;
import org.juzu.View;
import javax.inject.Inject;
public class MyPortletController {
@Inject
MyService myService;
@View
public void display() {
// Use the injected MyService instance
myService.performService();
}
}
@ApplicationScoped
public class MyService {
public void performService() {
// Perform some application-wide service logic
}
}
Beyond Basics
It's tempting to stop once your Juzu portlets are up and running, but there's much more to explore. For instance:
- AJAX and Juzu: Enhancing user experience with asynchronous requests
- Unit Testing Juzu Applications: Ensuring reliability with thorough tests
- Performance Optimization: Caching strategies, minimizing server round-trips
Final Thoughts
Mastering Juzu portlets requires understanding the eXo Platform and Juzu frameworks at a deep level. Avoiding the common pitfall of incorrect scope usage can save you from many headaches down the line. Remember to keep your application's performance in mind and only hold onto data as long as necessary.
Embrace the power of Juzu to write clean, maintainable, and efficient portlets for eXo Platform. The difference between a good application and a great one lies in the details. Avoid the common pitfalls, and you’re well on your way to creating outstanding eXo portlets that stand the test of time.
Happy coding, Java enthusiasts!
Interested in learning more about advanced eXo platform development? Explore more here.