Boosting Java Performance: Mastering ReadWriteLock
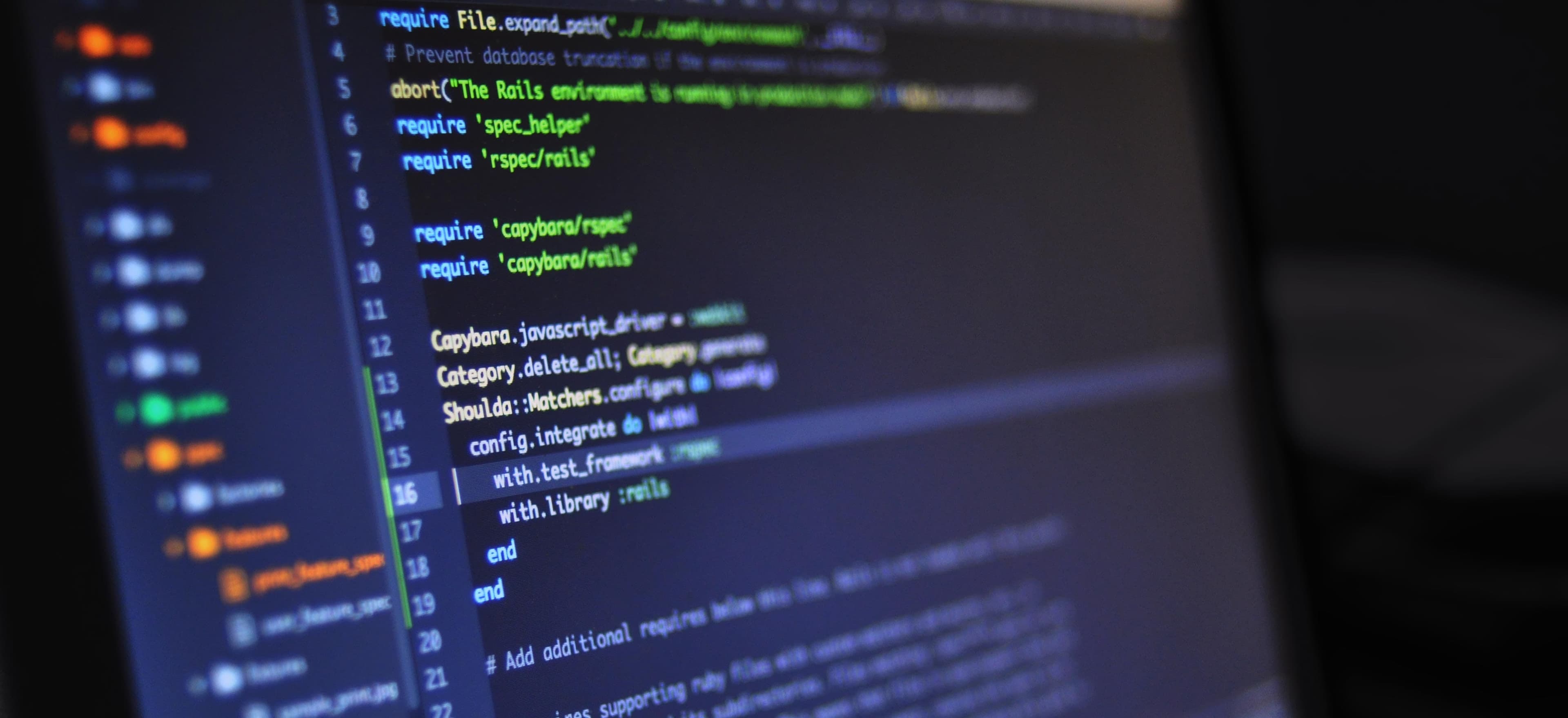
- Published on
Boosting Java Performance: Mastering ReadWriteLock
A Brief Overview
Concurrent programming is an essential part of modern software development, allowing multiple tasks to be executed simultaneously. In Java, concurrency is achieved using threads, which can execute different parts of a program concurrently. However, managing access to shared resources among these threads can be challenging and can potentially lead to issues like race conditions or deadlocks. That's where the ReadWriteLock comes into play.
Understanding ReadWriteLock
What is ReadWriteLock?
The ReadWriteLock interface in Java provides a way to implement multiple readers single writer locks. It allows multiple threads to read a shared resource simultaneously, but only one thread can write to the resource at a time.
In the context of concurrency, a lock is a synchronization construct that ensures only one thread at a time can access a shared resource. The ReadWriteLock interface provides two types of locks: read locks and write locks.
Why Use ReadWriteLock?
ReadWriteLock is advantageous over other locking mechanisms like the synchronized keyword or ReentrantLock in scenarios where there are many more reads compared to writes on a shared resource. In such cases, ReadWriteLock offers better parallelism and performance. It allows multiple threads to read the resource simultaneously, thus maximizing concurrency and improving throughput.
Using a synchronized block or ReentrantLock for every read operation can lead to unnecessary contention and decreased performance, as these mechanisms enforce exclusive access to the resource for each read operation. ReadWriteLock, on the other hand, provides a more granular approach to synchronization, allowing concurrent reading and exclusive writing.
In situations where the number of write operations is significantly higher than read operations, ReadWriteLock may not provide much performance improvement compared to other locking mechanisms. It is crucial to understand the characteristics of the application and the access patterns to make an informed decision on whether to use ReadWriteLock or not.
Working With ReadWriteLock in Java
To work with ReadWriteLock in Java, we use the ReentrantReadWriteLock
class, which implements the ReadWriteLock interface. Let's take a closer look at how to use it.
The ReentrantReadWriteLock Class
The ReentrantReadWriteLock
class provides a straightforward way to work with ReadWriteLocks in Java. It implements the ReadWriteLock interface and provides the necessary methods to acquire read and write locks.
To create an instance of ReentrantReadWriteLock
, we can simply call its constructor:
ReadWriteLock lock = new ReentrantReadWriteLock();
Acquiring Read and Write Locks
Once we have an instance of ReentrantReadWriteLock
, we can use it to acquire read and write locks. The read lock allows multiple threads to read the shared resource simultaneously, while the write lock ensures exclusive access for a single thread performing a write operation.
To obtain the read lock or the write lock, we can use the readLock()
and writeLock()
methods of the ReentrantReadWriteLock
instance, respectively:
Lock readLock = lock.readLock();
Lock writeLock = lock.writeLock();
Implementing ReadWriteLock in Code
ReadLock Usage
Let's consider a scenario where we have a shared resource that can be read by multiple threads concurrently. In such cases, we can use the read lock to allow multiple readers access to the resource simultaneously while ensuring exclusive access for writers.
Here's an example code snippet that demonstrates how to use the read lock in Java:
// Assume we have a shared resource called sharedResource
// Acquire the read lock
readLock.lock();
try {
// Perform read operations on the shared resource
// ...
} finally {
// Release the read lock
readLock.unlock();
}
In the above code snippet, we first acquire the read lock using the lock()
method of the read lock object. Within the try
block, we can perform any read operations on the shared resource. Finally, in the finally
block, we release the read lock using the unlock()
method to allow other threads to acquire the lock.
WriteLock Usage
In situations where we need to update a shared resource that should only be modified by one thread at a time, we can use the write lock provided by ReadWriteLock.
Consider the following code snippet that demonstrates the usage of the write lock:
// Assume we have a shared resource called sharedResource
// Acquire the write lock
writeLock.lock();
try {
// Perform write operations on the shared resource
// ...
} finally {
// Release the write lock
writeLock.unlock();
}
In the above code snippet, we acquire the write lock using the lock()
method of the write lock object. Within the try
block, we can perform any necessary write operations on the shared resource. Finally, in the finally
block, we release the write lock using the unlock()
method.
It's important to note that when a thread holds a write lock, no other thread, including those holding read locks, can access the shared resource until the write lock is released. This ensures that only one thread is modifying the shared resource at any given time.
Common Pitfalls and Best Practices
While working with ReadWriteLocks, there are certain pitfalls and best practices that developers should be aware of to prevent issues and optimize performance.
Tips for Avoiding Deadlocks
Deadlocks can occur when multiple threads are waiting for locks that are held by other threads, resulting in a situation where none of the threads can proceed. Here are some tips for avoiding deadlocks when using ReadWriteLock:
- Avoid acquiring a read lock while already holding a write lock, as this can lead to potential deadlocks.
- Be cautious when using condition variables with ReadWriteLock, as they can introduce synchronization complications.
To prevent deadlocks, it's essential to analyze your application's locking requirements and ensure proper lock acquisition and release procedures. Here's an example code snippet that illustrates a pattern to avoid common deadlocking scenarios:
// Assuming we have two locks, lockA and lockB
// Acquire the locks in a specific order
if (lockA.tryLock()) {
try {
if (lockB.tryLock()) {
try {
// Perform operations with the acquired locks
// ...
} finally {
lockB.unlock();
}
} else {
// Handle the case when lockB is already locked
}
} finally {
lockA.unlock();
}
} else {
// Handle the case when lockA is already locked
}
In the above code snippet, we attempt to acquire the locks lockA
and lockB
. By always acquiring the locks in a specific order and releasing them in the opposite order, we can effectively avoid deadlocks.
Performance Considerations
When considering the performance impact of using ReadWriteLock, there are several factors to take into account. While ReadWriteLock offers significant performance improvements in read-heavy scenarios, it might not provide substantial benefits in situations where the number of write operations outweighs the read operations.
The overhead of acquiring and releasing locks should also be considered. Acquiring a read lock is generally faster than acquiring a write lock, as it doesn't require exclusive access to the shared resource. However, write locks can potentially introduce contention and increase the waiting time for threads trying to acquire the lock.
It's crucial to analyze the access patterns of your application and determine whether the benefits of ReadWriteLock outweigh the performance overhead. In some cases, using other synchronization mechanisms like the synchronized keyword or ReentrantLock may be more appropriate.
The Last Word
ReadWriteLock is a powerful tool for managing shared resources in Java applications, particularly when there are many more reads than writes. It allows multiple threads to read the resource concurrently while ensuring exclusive access for write operations.
In this article, we've covered the basics of ReadWriteLock, its advantages over other locking mechanisms, and how to use it in Java applications. We've explored the ReentrantReadWriteLock class and demonstrated how to acquire read and write locks. We've also discussed common pitfalls and best practices when working with ReadWriteLocks.
By mastering ReadWriteLock, you can unlock the potential for improved concurrency and performance in your Java applications. Experiment with it in your own projects and share your experiences with fellow developers. Together, we can continue to optimize Java performance and create more efficient and scalable applications.
Further Reading and Resources
- Official Java documentation on ReadWriteLock
- Baeldung's Guide to Java Concurrency for further in-depth tutorials and examples
- Java Concurrency in Practice by Brian Goetz et al. – a comprehensive book on concurrency in Java
- Oracle's Java Tutorials on concurrency in Java