Mastering JSF & Spring: Seamless Pagination Tips
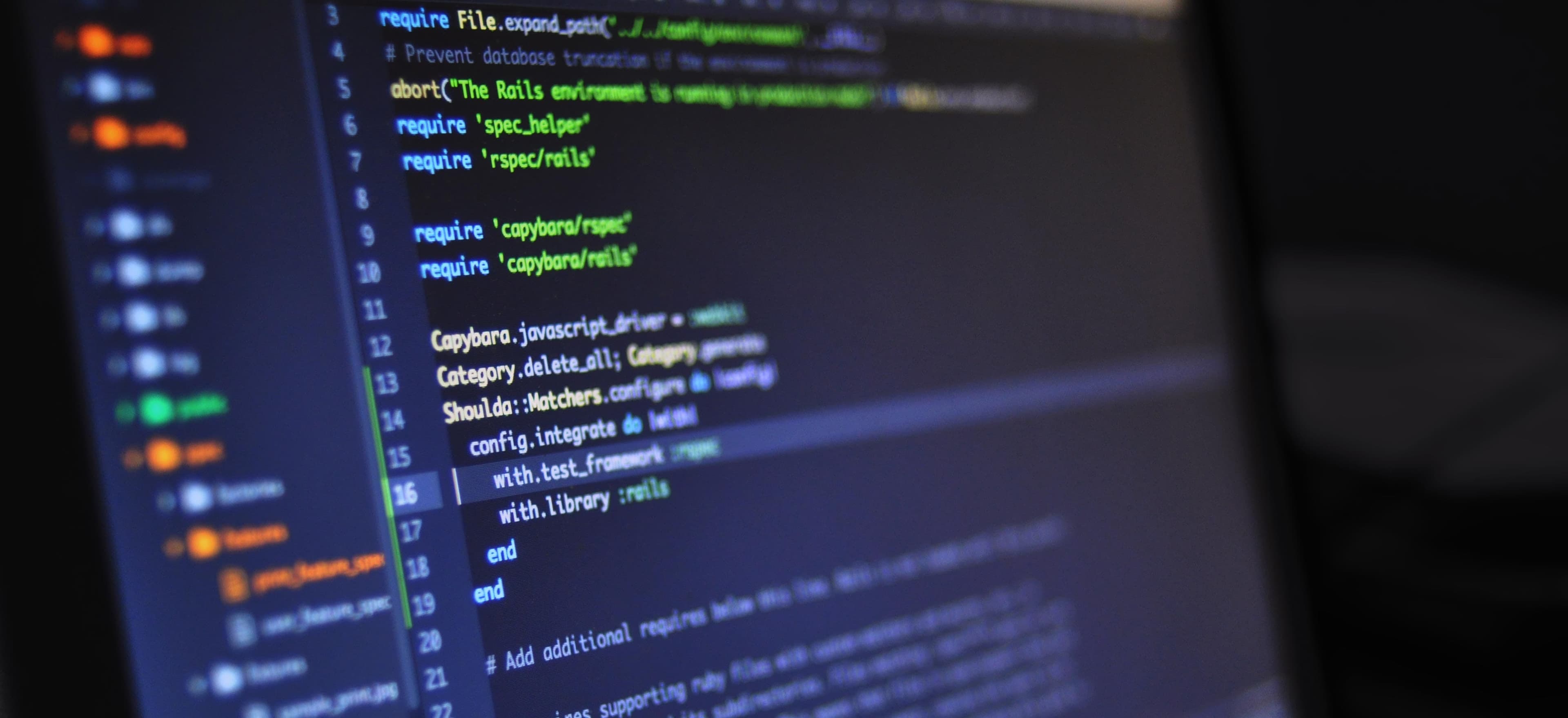
- Published on
Mastering JSF & Spring: Seamless Pagination Tips
Pagination is an essential feature in any web application that deals with large amounts of data. It allows users to navigate through numerous pages of data in a manageable and efficient manner. In this blog post, we will explore how to implement seamless pagination using JSF (JavaServer Faces) and Spring, two powerful frameworks for building Java web applications. We will discuss the importance of pagination, the challenges it poses, and provide some tips for achieving a seamless pagination experience.
Why is Pagination Important?
Imagine you have a web application that displays a table with thousands of records. Without pagination, loading and rendering all the data on a single page would be slow, resource-intensive, and overwhelming for users. Pagination helps solve these issues by breaking the data into smaller, more manageable chunks, allowing users to browse through the data with ease.
The Challenges of Pagination
Implementing pagination can be challenging, especially when dealing with a complex web application that involves multiple layers and frameworks. Here are some common challenges you may encounter:
-
Data Fetching: Retrieving data from a database or external API is the first step in pagination. It is crucial to efficiently retrieve only the data needed for the current page to avoid unnecessary network traffic and resource consumption.
-
UI Updates: Updating the user interface to display the correct page of data without refreshing the entire page requires careful handling of AJAX requests and partial rendering. Seamlessly updating the table without disrupting the user's context is a key aspect of a good pagination experience.
-
Sorting and Filtering: Users often want to sort or filter the data based on specific criteria. Integrating sorting and filtering features alongside pagination adds an extra layer of complexity. It is essential to ensure that sorting and filtering are performed only on the currently displayed data and not on the entire dataset.
Implementing Seamless Pagination in JSF & Spring
To achieve seamless pagination using JSF and Spring, we can follow these tips:
Tip 1: Fetch Data Lazily
Fetching the data lazily means retrieving only the necessary data for the current page. This can significantly improve performance by reducing the amount of data transferred over the network and reducing the load on the server.
One way to accomplish lazy data fetching is by utilizing the javax.faces.event.AjaxBehaviorEvent
in JSF. This event is triggered when the user interacts with the pagination controls, such as clicking on the next or previous page. By handling this event in a managed bean, we can fetch the appropriate data for the current page and update the UI accordingly.
Here's an example of a JSF managed bean method that handles the pagination event:
@ManagedBean
@RequestScoped
public class PaginationBean {
private List<MyData> data;
private int currentPage;
private int pageSize;
@PostConstruct
public void init() {
// Initialize default values
currentPage = 1;
pageSize = 10;
// Fetch data for the initial page
fetchData();
}
public void fetchData() {
// Retrieve data for the current page using the currentPage and pageSize variables
// Update the data property with the fetched data
// Perform any necessary sorting or filtering operations
// Update the UI with the new data
}
// Getters and setters
}
In this example, the init()
method is annotated with @PostConstruct
, ensuring that it is executed after the bean is constructed and all dependencies are injected. This method initializes default values for the pagination properties, such as the current page number and page size. It also fetches the initial data for the first page.
The fetchData()
method is responsible for fetching the data for the current page, performing any necessary sorting or filtering operations, and updating the UI with the new data. By binding the pagination controls to this method, changes in the current page or page size will trigger an AJAX request, resulting in the appropriate data being fetched and displayed.
Tip 2: Use AJAX for Partial Rendering
Updating the user interface without refreshing the entire page is crucial for achieving seamless pagination. JSF provides built-in support for AJAX-based partial rendering, allowing us to update specific components or areas of the page dynamically.
To use AJAX for partial rendering, we can use the <f:ajax>
tag in our JSF view. This tag can be placed within any JSF component that triggers an action, such as a button or a link. By configuring the <f:ajax>
tag with the appropriate attributes, we can define which components should be updated when the action is performed.
Here's an example of using <f:ajax>
for partial rendering in a JSF view:
<h:commandButton value="Next Page">
<f:ajax listener="#{paginationBean.fetchData}" render="dataTable" />
</h:commandButton>
In this example, when the user clicks the "Next Page" button, the associated AJAX listener method in the managed bean (paginationBean.fetchData()
) will be invoked. After fetching the data for the next page, the render
attribute specifies that the component with the id "dataTable" should be updated.
Using the render
attribute, we can specify multiple components to be updated simultaneously, separated by spaces. This allows us to update different parts of the page, such as the table itself, pagination controls, or even a summary section displaying the total number of records.
Tip 3: Perform Sorting and Filtering on Displayed Data Only
Enabling sorting and filtering alongside pagination can greatly enhance the usability of your application. However, it is important to ensure that these operations are performed only on the currently displayed data, rather than on the entire dataset.
To achieve this, we need to keep track of the applied sorting and filtering criteria and apply them along with the pagination properties. This can be done by modifying the fetchData()
method in our managed bean to include the necessary logic for sorting and filtering.
Here's an example of how we can perform sorting and filtering on displayed data:
public void fetchData() {
// Retrieve data for the current page using the currentPage and pageSize variables
// Perform sorting based on a sorting property
// Perform filtering based on a filtering criteria
// Update the data property with the fetched and filtered data
// Perform any necessary sorting or filtering operations
// Update the UI with the new data
}
In this example, we assume that the MyData
class has a property that we can use for sorting, such as name
. By adding sorting logic before updating the UI, we ensure that the data is displayed in the desired order.
Similarly, filtering logic can be implemented to filter the data based on specific criteria. This can be achieved by using a search form or input field, where the user can enter filter parameters. The entered criteria can then be applied to the fetched data before updating the UI.
By combining sorting, filtering, and pagination, users can effortlessly navigate through large datasets, while still having control over how the data is displayed.
The Closing Argument
Pagination is a critical feature for any web application dealing with large amounts of data. By implementing seamless pagination using JSF and Spring, we can create a smooth and efficient user experience. Remember to fetch data lazily, use AJAX for partial rendering, and perform sorting and filtering on displayed data only. These tips will help you overcome the challenges of pagination and deliver a high-quality web application.
If you're interested in learning more about JSF and Spring, check out the following resources:
Happy coding!