Master Redis CLI: Solving Slow Command Debugging!
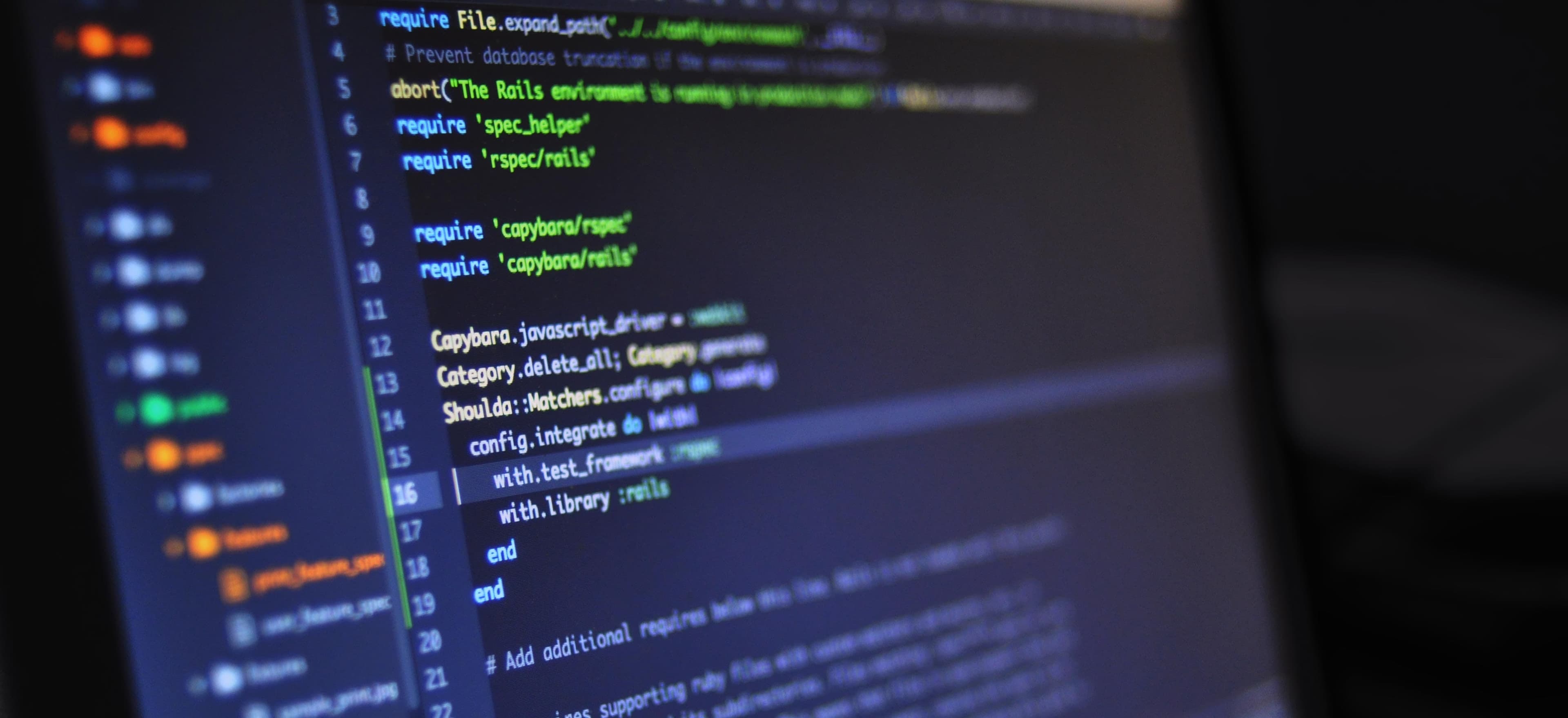
- Published on
Master Redis CLI: Solving Slow Command Debugging!
Introduction
Redis has become a popular choice for many modern-day applications due to its high performance and ability to handle large amounts of data. However, as with any database system, slow commands can have a significant impact on the overall performance and efficiency of an application. Debugging slow commands in Redis is crucial for maintaining a highly functioning application. In this article, we will explore the various tools and techniques provided by Redis CLI to diagnose and resolve slow command issues. By the end of this article, you will have gained a comprehensive understanding of how to master Redis CLI and effectively debug slow commands in Redis.
Understanding Redis CLI
Redis CLI is a command line interface that allows you to interact with Redis instances. It provides a convenient way to manage and debug your Redis deployments. To access the CLI, you first need to ensure that Redis is installed on your machine. You can download and install Redis from the official Redis website (https://redis.io/download). Once Redis is installed, you can access the CLI by running the redis-cli
command.
Here are some basic commands that will help you navigate and access data in the Redis CLI:
GET key
: Retrieve the value associated with a specific key.SET key value
: Set the value of a key.KEYS pattern
: Retrieve all keys matching a specific pattern.FLUSHALL
: Remove all data from the Redis database.
Understanding these basic commands will lay a solid foundation for further exploration of debugging slow commands in Redis CLI.
What Causes Slow Commands in Redis?
There are several common reasons why Redis commands can be slow:
- Heavy commands in large datasets: Some Redis commands, such as
SORT
orSCAN
, can be slow when executed on large datasets. These commands require significant computational resources and can take longer to complete. - Lack of indexing: If proper indexing is not implemented, Redis may need to scan through large amounts of data to retrieve specific values, resulting in slow commands.
- Poor memory management: If Redis runs out of memory, it may need to swap data in and out of the disk, which can significantly slow down command execution.
- Network latency: Slow network connections between the Redis client and server can also contribute to slow command performance.
Identifying slow commands is crucial for maintaining the efficiency and performance of your Redis application. It allows you to pinpoint performance bottlenecks and take appropriate action to optimize your Redis deployment.
Setting Up for Debugging
Before diving into debugging slow commands, it is important to ensure that you have a safe environment for testing and debugging. Creating an isolated Redis environment specifically for debugging purposes is highly recommended. This ensures that any changes made during the debugging process do not affect your production data.
To set up a debugging environment:
- Install Redis on a separate machine or virtual environment.
- Use a different Redis database for debugging, separate from your production database.
- Populate the debugging database with a representative dataset, similar to your production data.
By setting up a separate debugging environment, you can freely experiment and analyze slow commands without risking any negative impact on your production system.
Using SLOWLOG
for Diagnosing Issues
SLOWLOG
is a Redis command that allows you to monitor and diagnose slow commands. It provides a log of the most recent slow operations executed by Redis. You can use SLOWLOG
to identify commands that are taking longer than a specified threshold and investigate the causes behind their slow execution.
Here are the main commands associated with SLOWLOG
:
SLOWLOG GET n
: Retrieve the most recentn
slow operations from the slow log. This command provides detailed information about each slow command, including the command itself, the time it took to execute, and the arguments passed.SLOWLOG LEN
: Get the length of the slow log, i.e., the number of slow operations recorded.SLOWLOG RESET
: Reset the slow log, clearing all slow operations recorded.
To retrieve the most recent slow operations from the Redis slow log, use the following command:
redis-cli SLOWLOG GET
Example: This command retrieves the most recent slow operations from Redis.
Analyzing the slow log will help you identify patterns and potential issues with commands that are consistently slow. By referring to the details provided by SLOWLOG
, you can gain valuable insights into the execution time, arguments, and overall behavior of slow commands.
Understanding INFO COMMANDSTATS
Another useful tool provided by Redis CLI is the INFO COMMANDSTATS
command. This command provides information about the execution time of each Redis command. This can be particularly helpful in identifying slow commands and understanding their impact on overall Redis performance.
To use INFO COMMANDSTATS
, simply run the following command in the Redis CLI:
redis-cli INFO COMMANDSTATS
The output of this command will display statistics for each Redis command, including the total number of calls made, the total execution time, the average execution time, and the maximum execution time.
By analyzing the INFO COMMANDSTATS
output, you can quickly identify which commands are consuming the most time and resources. This information is invaluable for optimizing your Redis deployment and addressing slow commands effectively.
Advanced Tools and Configurations
In addition to the built-in Redis CLI tools, there are several advanced tools and configurations that can further aid in diagnosing and optimizing slow Redis commands.
Some of these tools include:
- redis-stat: A command-line tool that provides real-time monitoring and analysis of Redis servers. It displays various metrics, such as memory usage, command execution time, and network activity. You can install redis-stat by following the instructions provided in the redis-stat GitHub repository.
- Redmon: A web-based monitoring tool for Redis. It provides real-time performance metrics, slow command logs, and memory usage graphs. You can find a detailed guide on setting up Redmon in the official Redmon documentation.
- Redis MONITOR: The
MONITOR
command allows you to see all commands processed by the Redis server in real-time. Although it should be used sparingly in production environments due to its impact on performance, it can be valuable for debugging slow command issues.
Additionally, you can modify Redis configuration settings to adjust thresholds for slow command logging. By changing the values of configuration options such as slowlog-log-slower-than
and slowlog-max-len
, you can customize the logging of slow commands to meet your specific needs.
Writing Code That Avoids Slow Commands
While debugging slow commands is important, it is equally crucial to write code that avoids slow commands from the start. Here are some best practices for writing Redis interaction code that minimizes the likelihood of slow commands:
- Batch operations: Instead of sending individual commands for each operation, use batch operations like
MGET
orEXEC
to minimize round-trip time between the client and server. - Pipelining: Redis supports pipelining, where multiple commands can be sent in a single request. Pipelining reduces network latency by allowing commands to be sent in batches.
- Data sharding: If your application deals with large data sets, consider sharding or partitioning the data across multiple Redis instances. This allows commands to be distributed across multiple servers, reducing the load on individual instances.
- Proper indexing: Ensure that you have indexes defined for frequently accessed fields to reduce the need for full dataset scans.
Let's take a look at an example of how to optimize Redis interactions by using a pattern called object-relational mapping (ORM) in Java:
// Java example for optimizing Redis interactions to avoid slow commands
// Using an ORM library like Jedis, connect to the Redis server
Jedis jedis = new Jedis("localhost", 6379);
// Use the Jedis commands to interact with Redis efficiently
jedis.set("user:1:name", "John Doe");
jedis.set("user:1:email", "john@example.com");
// Retrieve user data efficiently without individual commands
Map<String, String> userData = jedis.hgetAll("user:1");
// Close the connection after use
jedis.close();
In this example, we use the Jedis library to establish a connection with the Redis server. Instead of individually setting and getting each user field, we use the hset
and hgetAll
commands to efficiently store and retrieve user data in Redis. This results in fewer round-trips between the client and server, reducing the likelihood of slow commands.
Case Study: Troubleshooting a Real-Life Scenario
To put the learning into practice, let's consider a real-life scenario where slow Redis commands were affecting an application.
Scenario: A social media platform is experiencing performance issues due to slow Redis commands. Users are complaining about slow response times and frequent timeouts.
Steps to identify and resolve the issue:
- Analyze slow log: Use the
SLOWLOG GET
command to retrieve the most recent slow operations from the Redis slow log. Analyze the output to identify the commands with the highest execution time. - Review command arguments: Examine the arguments passed to the slow commands identified in step 1. Look for any patterns or inconsistencies that may be contributing to their slow execution.
- Optimize data retrieval: Refactor the code to use batch operations or pipelining, reducing the number of round-trips between the client and server.
- Monitor and measure: Install a monitoring tool like redis-stat or Redmon to track the performance of Redis in real-time. Use the gathered metrics to identify any further optimization opportunities.
- Test and iterate: Continuously test and validate your changes to ensure they have the desired impact on performance. Measure response times and user feedback to assess the effectiveness of your optimizations.
By following these steps, you can gradually improve the performance of your Redis deployment by addressing slow command issues.
In Conclusion, Here is What Matters
Debugging slow commands in Redis is crucial for maintaining high-performance applications. In this article, we explored the various tools and techniques provided by Redis CLI to diagnose and resolve slow command issues. We examined the use of SLOWLOG
to monitor and investigate slow commands, as well as the INFO COMMANDSTATS
command for profiling command execution time. We also covered advanced tools like redis-stat
and Redmon, as well as configuration settings to adjust thresholds for slow command logging. Additionally, we discussed best practices for writing Redis interaction code that avoids slow commands.
By mastering Redis CLI and understanding how to debug slow commands, you can optimize the performance and efficiency of your Redis deployments. Remember to analyze the slow log, profile command execution time, and make use of advanced tools and configurations. With these techniques, you can ensure that your Redis-powered applications deliver fast and responsive experiences to your users.
We hope this article has provided you with valuable insights into debugging slow commands in Redis. We would love to hear about your experiences and suggestions in the comments!
SEO Optimization Tips
To optimize this article for search engines, we can target the following keywords: Redis CLI, debug slow Redis commands, Redis SLOWLOG
, optimizing Redis performance. Here are some tips for integrating these keywords effectively:
- Introduction: Include the keywords in the introduction to provide a clear context and relevance to the topic.
- Subheadings: Use the primary keywords in the subheadings to improve search engine visibility.
- Content: Seamlessly integrate the keywords into the text where appropriate and ensure a natural flow of the content.
- External sources: Link to authoritative sources such as the official Redis documentation or reputable tech blogs that discuss Redis performance.
- Meta tags and URL slug: Use relevant meta tags and create a descriptive URL slug that reflects the topic and primary keywords.
By following these SEO optimization tips, this article will have a better chance of ranking higher in search engine results and attracting relevant readers interested in Redis CLI and optimizing Redis performance.