Master Kafka Streams: Top Techniques for Dev Success!
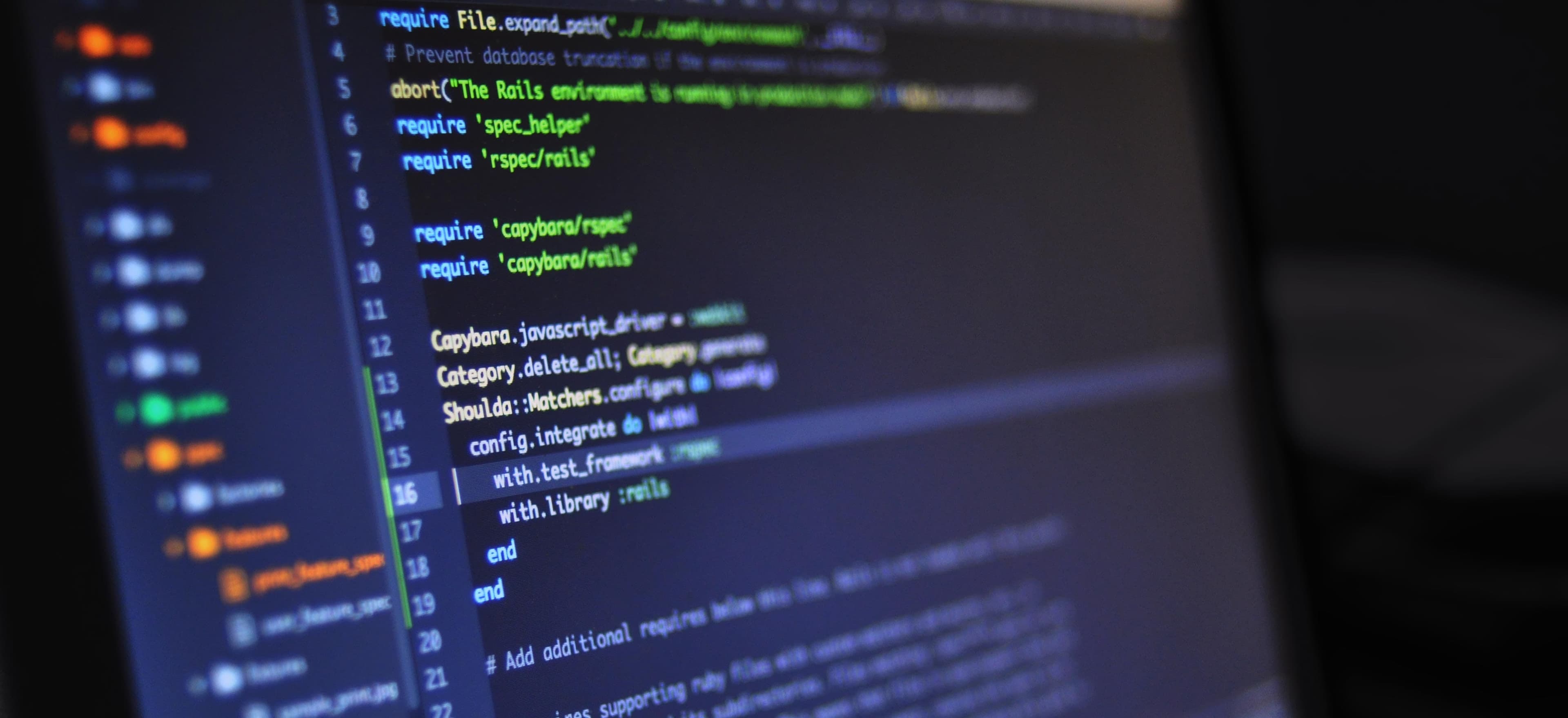
- Published on
Master Kafka Streams: Top Techniques for Dev Success!
Establishing the Context
In today's era of big data and real-time analytics, being able to process and analyze streaming data is crucial. Apache Kafka has emerged as a powerful distributed streaming platform that provides scalable and fault-tolerant stream processing capabilities. Kafka Streams, a component of Apache Kafka, allows developers to build real-time streaming applications with ease. In this expert guide, we will delve into the world of Kafka Streams and explore the top techniques every developer needs to know for building robust streaming applications.
Prerequisites
Before diving into Kafka Streams, it is important to have some basic knowledge and tools in place. Here are the prerequisites for getting started with Kafka Streams:
-
Familiarity with Apache Kafka: It is essential to have a basic understanding of Apache Kafka and its core concepts such as topics, producers, consumers, and brokers.
-
Basic understanding of stream processing concepts: Having a grasp of stream processing concepts like windowing, aggregations, and stateful operations will greatly enhance your understanding of Kafka Streams.
-
Java development environment set up: Kafka Streams is built on Java, so having a Java development environment set up is necessary. Install the latest version of Java Development Kit (JDK).
-
Apache Kafka cluster: You will need an Apache Kafka cluster to work with Kafka Streams. Setting up a simple local cluster using Kafka's quickstart guide is sufficient for learning purposes.
Now that we have the prerequisites sorted, let's move on to setting up the Kafka Streams environment.
Step 1: Setting up the Kafka Streams Environment
To begin working with Kafka Streams in a Java project, it is important to set up the necessary dependencies. Here's a step-by-step guide on how to do that:
-
Create a new Maven or Gradle project: Start by creating a new Maven or Gradle project, depending on your preferred build tool.
-
Add the Kafka Streams dependency: Open your project's build file (pom.xml for Maven or build.gradle for Gradle) and add the Kafka Streams dependency.
<!-- For Maven -->
<dependency>
<groupId>org.apache.kafka</groupId>
<artifactId>kafka-streams</artifactId>
<version>2.10</version>
</dependency>
// For Gradle
implementation 'org.apache.kafka:kafka-streams:2.10'
- Sync the project: After adding the dependency, sync your project with the build tool to download the necessary jars.
This will set up the basic Kafka Streams environment in your Java project. The Kafka Streams dependency provides all the necessary classes and utilities required for building streaming applications.
Step 2: Configuring Kafka Streams
Now that we have the Kafka Streams environment set up, it's time to configure it. Kafka Streams provides a set of configuration parameters that can be customized according to your application's needs.
Here are some important configuration parameters:
-
Application ID: Every Kafka Streams application needs a unique application ID. This ID is used for identifying the application and maintaining its state.
-
Bootstrap servers: Specify the bootstrap servers of your Kafka cluster. These are the initial set of brokers used by Kafka Streams for bootstrapping and discovering the rest of the cluster.
-
Serdes: Serdes play a crucial role in Kafka Streams applications as they handle key-value serialization and deserialization. You need to specify the appropriate serdes for your key and value types. Kafka Streams provides built-in serdes for common types like strings, integers, and so on.
Here's an example of how to set up a StreamsConfig
instance with the required configuration:
Properties config = new Properties();
config.put(StreamsConfig.APPLICATION_ID_CONFIG, "my-streams-application");
config.put(StreamsConfig.BOOTSTRAP_SERVERS_CONFIG, "localhost:9092");
config.put(StreamsConfig.DEFAULT_KEY_SERDE_CLASS_CONFIG, Serdes.String().getClass());
config.put(StreamsConfig.DEFAULT_VALUE_SERDE_CLASS_CONFIG, Serdes.String().getClass());
StreamsConfig streamsConfig = new StreamsConfig(config);
In this example, we have set the application ID as "my-streams-application" and the bootstrap servers as "localhost:9092". We have also specified the default serdes as Serdes.String()
.
It's important to carefully configure Kafka Streams according to your application's needs as it directly affects the performance and fault tolerance of your streaming application.
Step 3: Building Your First Stream Processor Topology
Now that we have the environment set up and configured, let's dive into building our first stream processor topology.
In Kafka Streams, stream processing logic is defined by creating a directed acyclic graph called a topology. This topology represents the data flow and transformations that the incoming data will undergo.
The two fundamental abstractions in Kafka Streams are KStream
and KTable
.
-
KStream: Represents an abstraction of a record stream that consists of key-value pairs. It can be thought of as an unbounded sequence of events that are immutable and ordered by time.
-
KTable: Represents an abstraction of a changelog stream that consists of a table with updates. It can be thought of as a database table where each key corresponds to the latest associated value.
Let's look at an example of a simple processor topology that takes a stream of text messages and counts the occurrences of each word:
KStream<String, String> input = builder.stream("my-input-topic");
KTable<String, Long> wordCounts = input
.flatMapValues(value -> Arrays.asList(value.toLowerCase().split("\\s+")))
.groupBy((key, word) -> word)
.count(Materialized.as("word-counts-store"));
wordCounts.toStream().to("my-output-topic", Produced.with(Serdes.String(), Serdes.Long()));
In this example, we start by creating a KStream
by consuming records from the "my-input-topic". We then perform a series of transformations on the stream, such as splitting the incoming text into individual words, grouping the words by key, and counting the occurrences of each word. Finally, we write the results to an output topic called "my-output-topic".
Each step in the topology has a specific purpose and is designed to achieve a specific outcome. Commenting on each step in your code will help explain what it does and why it's designed this way.
Step 4: Stateful Operations and Windows
As we move forward with building more complex stream processing applications, we often encounter scenarios where stateful operations and windows are required.
-
Stateful operations: Stateful operations are those that require maintaining some kind of state as the stream of data is being processed. Examples include aggregations, joins, and filtering based on historical data.
-
Windows: Windows are a way to segment the stream of data based on time or other criteria. Kafka Streams supports different window types such as tumbling, hopping, sliding, and session windows.
Let's look at an example of a stateful operation using windowing:
KStream<String, Integer> input = builder.stream("my-input-topic");
KTable<Windowed<String>, Long> windowedCounts = input
.groupByKey()
.windowedBy(TimeWindows.of(Duration.ofMinutes(5)))
.count();
windowedCounts.toStream()
.foreach((key, count) -> System.out.println("Window: " + key.window() + ", Key: " + key.key() + ", Count: " + count));
In this example, we create a windowed stream by grouping the input stream by key and specifying a window of 5 minutes. We then perform a count operation on the windowed stream, resulting in a KTable
of windowed counts. Finally, we print each window, key, and count using the foreach
operation.
Understanding different window types and when to use them is crucial for effective real-time data processing and resource utilization.
Step 5: Testing Kafka Streams Applications
Testing plays a vital role in ensuring the correctness and reliability of stream processing applications. Kafka Streams provides a powerful tool called the Topology Test Driver, which allows you to write tests for your Kafka Streams topology.
Here's an example of how to test a simple Kafka Streams application using the Topology Test Driver:
Topology topology = builder.build();
TopologyTestDriver testDriver = new TopologyTestDriver(topology, streamsConfig);
TestInputTopic<String, String> inputTopic = testDriver.createInputTopic("my-input-topic",
new StringSerializer(), new StringSerializer());
TestOutputTopic<String, Long> outputTopic = testDriver.createOutputTopic("my-output-topic",
new StringDeserializer(), new LongDeserializer());
inputTopic.pipeInput("key", "value");
KeyValue<String, Long> result = outputTopic.readKeyValue();
assertEquals("key", result.key);
assertEquals(1L, result.value);
In this example, we create a Topology
from the builder and initialize a TopologyTestDriver
with the topology and streams configuration. We then create input and output topics using the appropriate serializers and deserializers. Finally, we write a test message to the input topic and assert the expected result received from the output topic.
Testing Kafka Streams applications helps to catch bugs early on, verify the correctness of the processing logic, and ensure the application behaves as expected in different scenarios.
Best Practices for Kafka Streams Development
While working with Kafka Streams, it's important to follow some best practices to ensure the efficient and reliable functioning of your streaming applications. Here are some best practices to keep in mind:
-
Handling late events: Design your Kafka Streams application to handle late-arriving events by using techniques such as watermarking or event time processing.
-
State store management: Proper management of state stores is crucial for good performance and scalability. Make sure to estimate the size of your state stores and configure appropriate cache sizes and retention policies.
-
Monitoring and metrics: Implement monitoring and metrics in your Kafka Streams application to gain insights into the health, performance, and behavior of your application. Tools like Prometheus and Grafana can be used for visualizing and analyzing the metrics.
-
Scaling and fault tolerance strategies: Consider the scalability and fault tolerance aspects while designing your application. Use Kafka Streams' built-in fault tolerance features such as standby tasks and use the appropriate partitioning strategies for your input and output topics.
Following these best practices will help you build efficient, reliable, and scalable Kafka Streams applications.
Advanced Techniques: Custom Serdes and Processors
While Kafka Streams provides a wide range of built-in serializers and deserializers (Serdes) and operators to manipulate streams, there are cases where you may need to implement custom logic.
Custom Serdes: You may encounter scenarios where the built-in serdes are not sufficient for your data types. In such cases, you can implement custom serdes by extending the org.apache.kafka.common.serialization.Serdes
class and providing your own serialization and deserialization logic.
public class MyCustomSerde<T> extends Serdes.WrapperSerde<T> {
public MyCustomSerde() {
super(new MyCustomSerializer(), new MyCustomDeserializer());
}
}
Custom Processors: Kafka Streams provides a high-level DSL for stream processing, but for more complex use cases, you may need to use the lower-level Processor API. The Processor API allows you to define custom processors that can perform any arbitrary operations on the input streams.
public class MyCustomProcessor implements Processor<String, String> {
private ProcessorContext context;
@Override
public void init(ProcessorContext context) {
this.context = context;
}
@Override
public void process(String key, String value) {
// Custom processing logic
// ...
// Forward the processed record to the downstream processors or sink
context.forward(key, value);
context.commit();
}
@Override
public void close() {
// Cleanup logic
}
}
Implementing custom logic using custom serdes and processors allows you to handle complex scenarios and tailor your Kafka Streams application to your specific requirements.
Real-World Applications of Kafka Streams
Kafka Streams is widely used in various real-world scenarios where real-time stream processing is required. Let's take a look at some examples:
-
Fraud detection: Kafka Streams allows the real-time processing of large volumes of financial transactions, enabling fraud detection systems to detect and prevent fraudulent activities in real time.
-
Recommendation systems: Kafka Streams provides the necessary tools for building recommendation systems that can process user behavior data, analyze patterns, and make personalized recommendations in real time.
-
Real-time analytics: Kafka Streams is extensively used for real-time analytics, allowing organizations to perform computations over continuous streams of data and generate fast insights that drive decision-making.
-
Internet of Things (IoT): Kafka Streams plays a crucial role in IoT applications by enabling the processing of high-volume, high-velocity data generated by IoT devices in real time.
By leveraging Kafka Streams, organizations can build scalable and performant systems to enable real-time decision-making and extract valuable insights from streaming data.
Wrapping Up
In this expert guide, we have explored the world of Kafka Streams and the top techniques every developer needs to know for building robust streaming applications. We started by setting up the Kafka Streams environment, configuring it, and building our first stream processor topology. We then dived into stateful operations, windows, testing, and best practices for Kafka Streams development. Additionally, we covered advanced techniques using custom serdes and processors and discussed real-world applications of Kafka Streams.
Kafka Streams provides developers with a powerful and user-friendly framework for processing and analyzing streaming data in real time. By mastering Kafka