Boost Code Quality: Adopt Test-Driven Development!
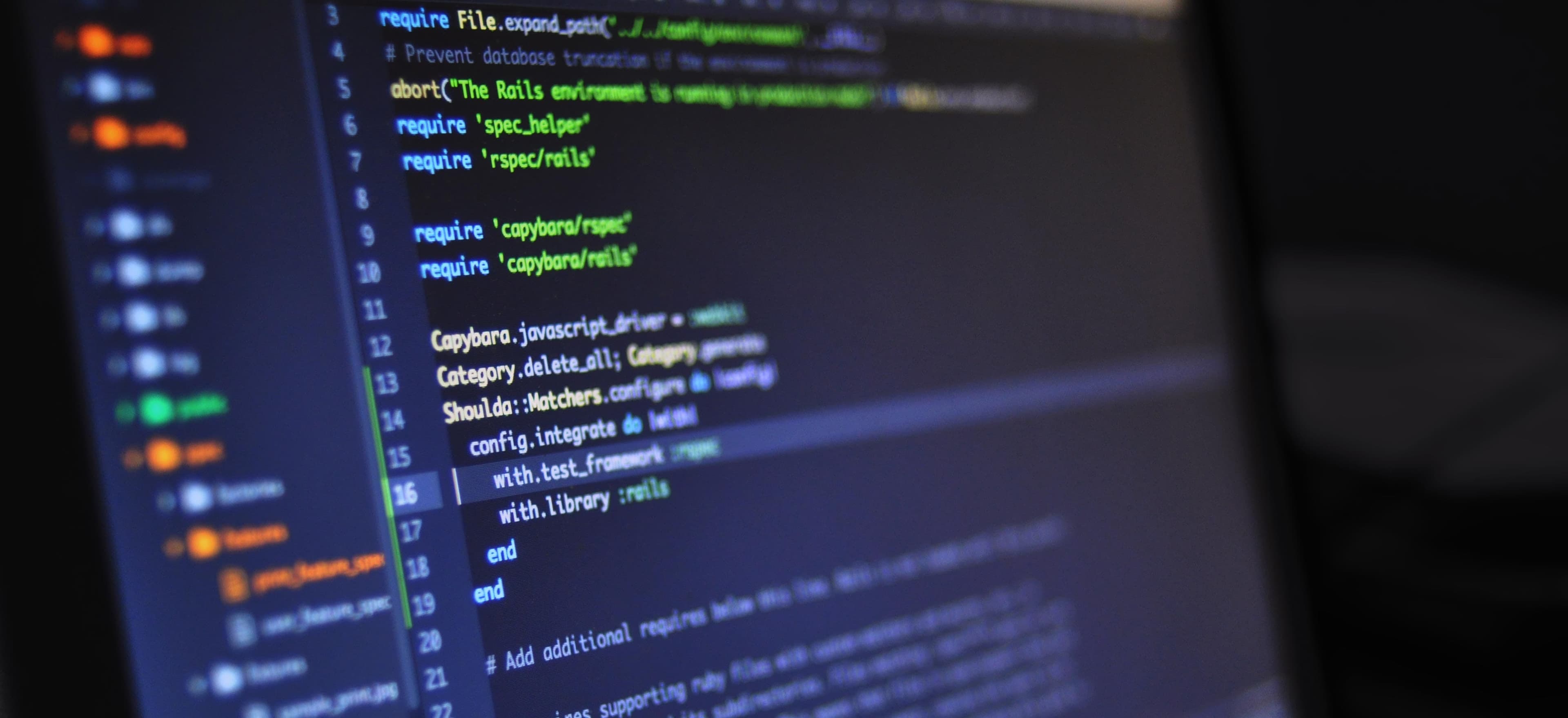
- Published on
Boost Code Quality: Adopt Test-Driven Development!
As developers, we all strive to write clean, efficient, and bug-free code. However, achieving high code quality can be challenging, especially in complex projects. That's where test-driven development (TDD) comes into the picture. TDD is a software development approach that focuses on writing automated tests before writing the actual code. By following the TDD approach, you can ensure that your code is thoroughly tested, maintainable, and of high quality. In this blog post, we'll explore the benefits of TDD and provide a step-by-step guide to help you get started.
What is Test-Driven Development (TDD)?
Test-driven development (TDD) is an iterative software development approach that puts testing at the forefront. The TDD process follows a simple and repeatable cycle: write a failing test, write just enough code to make the test pass, and then refactor the code for better design and maintainability.
The core idea behind TDD is to drive the development process through tests. By writing tests first, you gain a clear understanding of the desired behavior and specifications of your code. This ensures that you don't end up with untested code and helps avoid bugs and regressions down the line.
The Benefits of Test-Driven Development
Improved Code Quality
One of the primary benefits of adopting TDD is improved code quality. By writing tests upfront, you are forced to think about the desired behavior and edge cases before writing the code. This leads to better code design and architecture, reducing the chances of introducing bugs and making your code more maintainable.
Faster Feedback Loops
TDD promotes a tight feedback loop, allowing you to detect and fix issues early in the development process. When a test fails, you immediately know that something is wrong with your code. This quick feedback loop helps you identify and fix issues before they become larger and more complex to debug.
Simplified Refactoring
Refactoring is an essential part of software development. However, refactoring can be a daunting task if you don't have adequate tests in place. With TDD, you can confidently refactor your code, knowing that if you break something, the failing tests will let you know. This encourages you to make continuous improvements to your code without the fear of introducing bugs.
Increased Code Coverage
TDD encourages writing tests for every feature and use case, leading to high code coverage. Code coverage measures the effectiveness of your tests by determining how much of your code is actually executed. By striving for high code coverage, you can be confident that most of your code is being tested and that you have a safety net in place against regressions.
Better Collaboration and Documentation
Tests serve not only as a safety net but also as documentation for your code. When working in a team, tests provide clarity and increase the understanding of the codebase. Additionally, writing testable code forces you to think about the design and interfaces, making it easier for other developers to collaborate with you.
How to Adopt Test-Driven Development
Now that you understand the benefits of TDD, let's walk through a step-by-step guide to help you adopt this approach in your Java projects.
Step 1: Understand the Requirements
To start with TDD, you need a clear understanding of the requirements and expected behavior of the feature you'll be implementing. This involves having discussions with stakeholders, understanding user stories, and defining acceptance criteria. Once you are confident about the requirements, you can move on to writing tests.
Step 2: Write a Failing Test
In TDD, you always begin by writing a test that checks the desired behavior. Start by creating a test class for the feature or functionality you're implementing. Use a testing framework like JUnit or TestNG to write your tests. Write a failing test that verifies the expected outcome but without any implementation code.
Here's an example of a failing test using JUnit:
import org.junit.jupiter.api.Assertions;
import org.junit.jupiter.api.Test;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
int result = calculator.add(2, 2);
Assertions.assertEquals(4, result);
}
}
In this example, we have a failing test for the add
method of a Calculator
class. The test asserts that adding 2 and 2 should result in 4, but we haven't implemented the add
method yet, so the test will fail.
Step 3: Write the Minimum Implementation Code
Now that you have a failing test, it's time to write the minimum amount of code to make the test pass. In TDD, the goal is not to write perfect production code upfront but to get your tests to pass as quickly as possible.
Here's an example of a minimal implementation for the add
method:
public class Calculator {
public int add(int a, int b) {
return a + b;
}
}
With this minimal implementation, the failing test from step 2 will now pass.
Step 4: Refactor for Better Design
Once your test passes, it's time to refactor your code for better design, readability, and maintainability. Refactoring involves improving the structure and organization of the code without changing its behavior.
In our example, the code is simple, so no refactoring is required. However, in real-world scenarios, refactoring becomes critical as the codebase grows.
Step 5: Repeat the Cycle
After refactoring, you can move on to the next feature or functionality by returning to step 2. Write another failing test, write the minimum implementation code, and then refactor. Repeat this cycle for each feature or use case you need to implement.
Bringing It All Together
Test-driven development (TDD) is a powerful software development approach that can significantly boost code quality. By writing tests first, you ensure that your code is well-tested, maintainable, and less prone to bugs and regressions. TDD promotes a tight feedback loop, simplifies refactoring, increases code coverage, and improves collaboration. By following the step-by-step guide outlined in this blog post, you can start adopting TDD in your Java projects and enjoy its benefits.
So, why wait? Start implementing TDD today and take a step towards writing cleaner, more reliable code!