Sync Smarter: Mastering ReentrantLock with Lambdas!
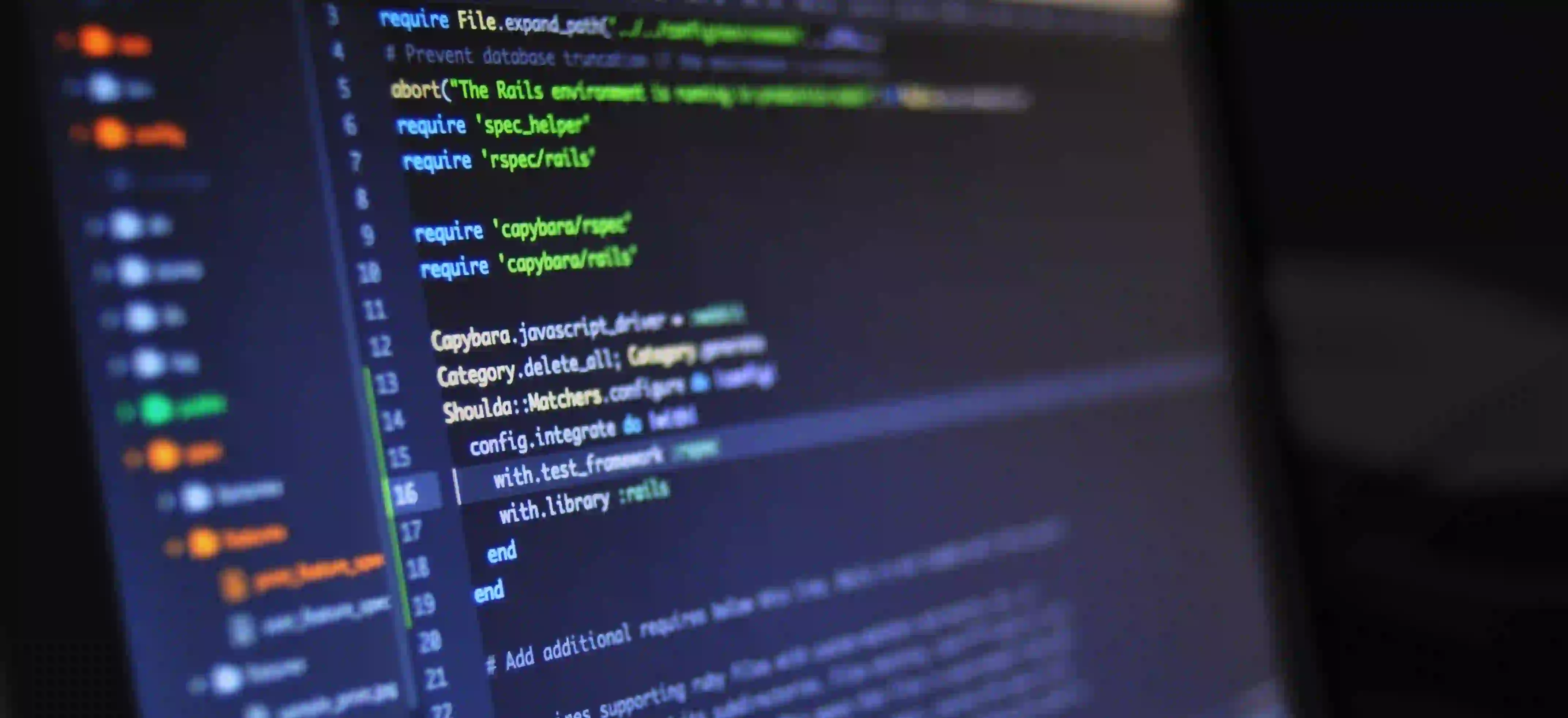
Sync Smarter: Mastering ReentrantLock with Lambdas!
When it comes to synchronization in Java, one powerful tool in our arsenal is the ReentrantLock
class. Unlike synchronized
blocks, which are built into the language, ReentrantLock
provides more flexibility and control over locking and unlocking resources. In this article, we will delve into the world of ReentrantLock
, exploring its features and how we can use it effectively with lambdas.
Understanding ReentrantLock
Before we dive deep into the details, let's have a brief overview of what ReentrantLock
is. In simple terms, it's a lock that allows reentrant behavior, which means that the same thread can acquire the lock multiple times without deadlocking itself. This characteristic makes it useful in complex scenarios where nested locking is required.
Basic Usage
To begin using ReentrantLock
, we first need to instantiate an object of this class. This can be done as follows:
ReentrantLock lock = new ReentrantLock();
Once we have the lock, we can use it to protect a critical section of code using the lock()
and unlock()
methods. Here's a simple example that illustrates this:
lock.lock();
try {
// Critical section of code
} finally {
lock.unlock();
}
The lock()
method acquires the lock, and the unlock()
method releases it. It's important to wrap the critical section of code in a try-finally
block to ensure that the lock is always released, even if an exception occurs.
Advanced Features of ReentrantLock
Fairness
By default, ReentrantLock
uses a non-fair policy, which means that the lock does not guarantee the order in which threads will acquire it. However, we can enable fairness by passing true
to the constructor:
ReentrantLock fairLock = new ReentrantLock(true);
Enabling fairness means that threads requesting the lock will be granted access in the order in which they arrived. While fairness can lead to a potential decrease in performance, it is useful in scenarios where we want to prevent thread starvation.
Condition Variables
Another powerful feature of ReentrantLock
is the ability to create condition variables. Condition variables allow threads to wait until a certain condition is met. This can be useful when we want threads to coordinate with each other.
Let's look at an example where we use condition variables. Suppose we have a shared buffer that can hold a maximum of 10 items. We want the producer threads to wait when the buffer is full and the consumer threads to wait when the buffer is empty.
ReentrantLock lock = new ReentrantLock();
Condition bufferFull = lock.newCondition();
Condition bufferEmpty = lock.newCondition();
void produce() throws InterruptedException {
lock.lock();
try {
while (isBufferFull()) {
bufferFull.await();
}
// Produce an item and add it to the buffer
bufferEmpty.signalAll();
} finally {
lock.unlock();
}
}
void consume() throws InterruptedException {
lock.lock();
try {
while (isBufferEmpty()) {
bufferEmpty.await();
}
// Consume an item from the buffer
bufferFull.signalAll();
} finally {
lock.unlock();
}
}
In the above code, the await()
method is called on the appropriate Condition
object to wait until the desired condition is met. When the condition is satisfied, the corresponding signalAll()
method is called to notify waiting threads that they can proceed.
TryLock
In addition to the usual lock()
method, ReentrantLock
provides a tryLock()
method that can be useful in certain scenarios. This method tries to acquire the lock but returns immediately with a boolean
value indicating whether the lock was obtained or not. Here's an example:
ReentrantLock lock = new ReentrantLock();
if (lock.tryLock()) {
try {
// Critical section of code
} finally {
lock.unlock();
}
} else {
// Lock acquisition failed, handle accordingly
}
The tryLock()
method is particularly useful when we want to avoid waiting for a lock and need to perform an alternative action if the lock is not available.
Simplifying ReentrantLock with Lambdas
Now that we have covered the basics of ReentrantLock
, let's explore how we can further simplify its usage using lambdas. By using functional interfaces from the java.util.concurrent.locks
package, we can encapsulate the lock and unlock operations into simple lambda expressions.
For example, instead of explicitly calling lock.lock()
and lock.unlock()
, we can define a lambda expression and pass it as an argument to a utility method. Here's a simple utility method that encapsulates the lock and unlock operations:
public static void withLock(ReentrantLock lock, Runnable action) {
lock.lock();
try {
action.run();
} finally {
lock.unlock();
}
}
With this utility method, we can now write more concise code that encapsulates the critical section within a lambda expression:
ReentrantLock lock = new ReentrantLock();
withLock(lock, () -> {
// Critical section of code
});
Using lambdas in this way can make our code cleaner and more readable, especially when dealing with multiple locks and complex synchronization scenarios.
Handling Exceptions
When using lambdas with ReentrantLock
, we need to be mindful of exception handling. If the code inside the lambda throws an exception, the lock may not be released, leading to potential deadlocks. To handle this, we can modify our utility method to ensure that the lock is always released, even if an exception occurs:
public static void withLock(ReentrantLock lock, RunnableWithException action) {
lock.lock();
try {
action.run();
} catch (Exception e) {
// Handle the exception appropriately
} finally {
lock.unlock();
}
}
The new withLock
method takes advantage of a custom functional interface RunnableWithException
that allows the lambda expression to throw exceptions:
@FunctionalInterface
public interface RunnableWithException {
void run() throws Exception;
}
Now, the lambda expression can throw exceptions, and the lock will always be released, preventing any potential deadlocks.
Closing Remarks
In this article, we have explored the powerful features of ReentrantLock
and learned how to use it effectively with lambdas. With its reentrant behavior, fairness option, condition variables, and tryLock feature, ReentrantLock
provides a robust mechanism for synchronizing threads in Java.
By encapsulating the lock and unlock operations within lambda expressions, we can simplify our code and improve readability. However, we must remember to handle exceptions properly to ensure that the lock is always released, preventing any potential deadlocks.
Now that you have mastered ReentrantLock
with lambdas, go forth and sync smarter! Happy coding!
References: