Seamless API Gateway Shift: Mastering Automation!
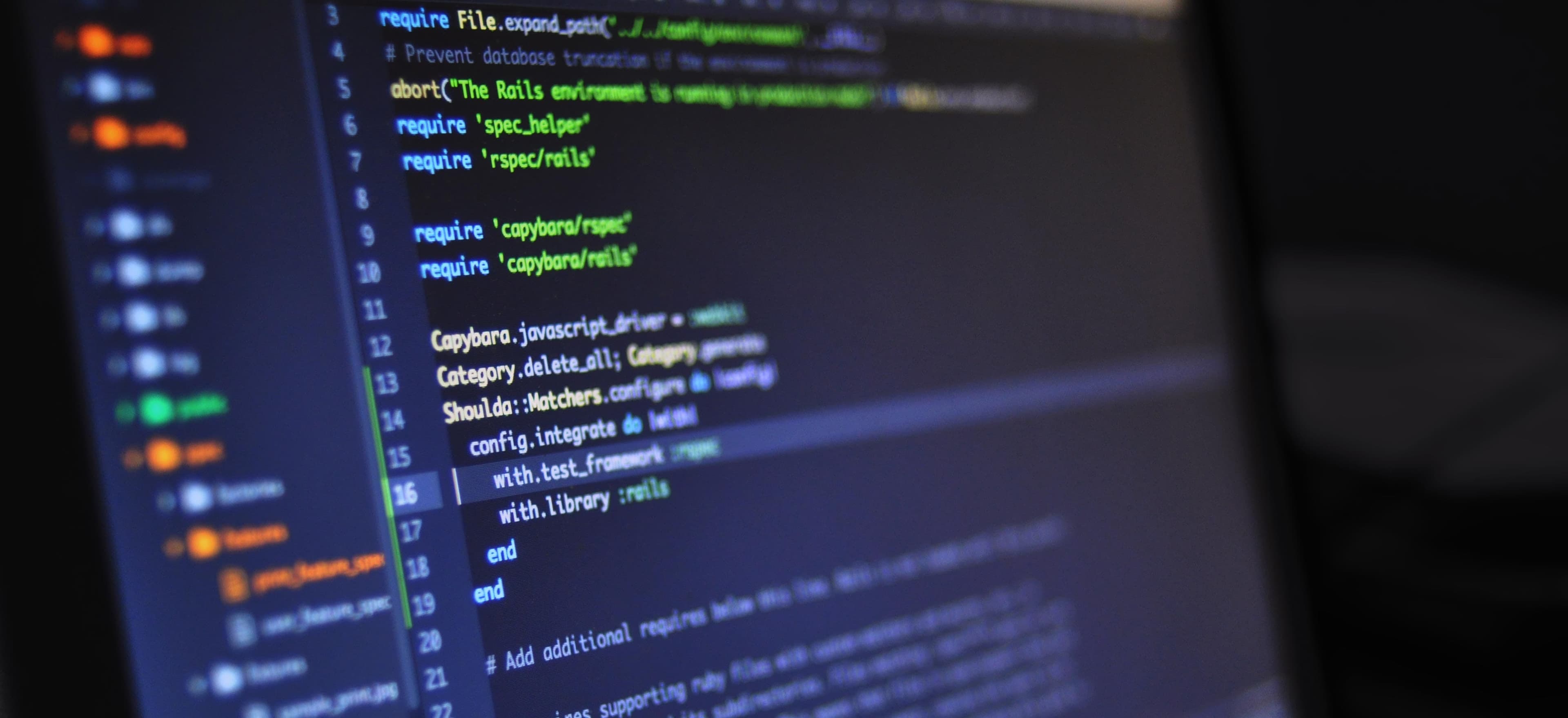
- Published on
Seamless API Gateway Shift: Mastering Automation!
In today's digital landscape, the importance of a robust and efficient API gateway cannot be overstated. As businesses evolve, so do their API strategies, and with the rise of microservices, the need for a dynamic gateway becomes increasingly imperative. In this deep dive, we explore the art of automating the shift to a new API gateway with a Java-based perspective, focusing on minimizing downtime and maintaining continuity.
Understanding the Role of an API Gateway
Before delving into automation, let’s establish what an API gateway is and does. An API gateway is a crucial component in modern web architectures, acting as a reverse proxy to route requests from clients to various microservices. It ensures security, provides load balancing, and aggregates multiple service calls into a single response, enhancing efficiency.
But why might an organization need to shift its API gateway? Reasons vary from seeking better performance, cost-effective solutions, support for newer protocols, and enhanced security features, to needing more robust analytics.
Preparing for an Automated Shift
Taking the leap to a new API gateway should be a meticulous process, with automation as your ally. Here’s how you can prepare for this transition:
- Assessment & Planning: Identify the requirements of your new gateway and how they align with your current and future needs.
- Backup Your Existing Configuration: Protect against data loss; ensure you have a rollback strategy.
- Choose the Right Tools: The Java ecosystem has tools like Jenkins for continuous integration, or Terraform and Ansible for infrastructure as code, which can help with automation.
// Jenkinsfile (pseudo-code)
pipeline {
agent any
stages {
stage('Backup') {
steps {
sh 'Backup current API gateway settings.'
}
}
stage('Deploy New Gateway') {
steps {
sh 'Deploy new API gateway using Terraform.'
}
}
}
}
The snippet above is a basic example of how you could automate the stages of deployment using Jenkins. Note that this is highly simplified for illustration purposes.
Executing the Automated Gateway Shift
Now that you have a plan and tools, it's time to execute your strategy. Your automation scripts should handle the following:
- Service Downtime: No matter how seamless the process, be prepared for possible downtime. Even a few seconds could matter.
- Data Sync: Ensure that all the configuration data is accurately moved to the new gateway without any loss.
- Testing & Validation: Implement automated testing to ensure that the new gateway is functioning as expected.
// Example of an automated test to validate API gateway routing.
// (Requires a testing framework like JUnit)
public class ApiGatewayTest {
@Test
public void testRoute() {
// Send a request to the new API gateway
// Assert the response to check if the routing is correct
}
}
In the above code, a simulated request can help validate whether the new gateway is correctly routing traffic. This is a crucial step to ensure the stability of your system.
Post-Shift Monitoring and Optimizations
With automation handling the heavy lifting, you still need to keep a vigilant eye post-migration. Logging, monitoring, and real-time alerts are essential pillars for understanding the new gateway's performance and health. Java-based tools like Log4j for logging and Metrics for monitoring can be vital (learn more about Log4j practices here).
Why Java for API Gateway Automation?
Java's robust libraries and the vast community support make it an optimal choice for backend systems. It provides high performance and scalability, which are fundamental for managing API gateways.
Code Snippet - Automation Task
Let's dive deeper with a more specific code snippet that illustrates Java's implementation into the automated shift. Assume we’re using a hypothetical tool named GatewayManager
that handles API gateway operations:
import com.example.gateway.GatewayManager;
public class GatewayMigration {
private GatewayManager gatewayManager;
public GatewayMigration(GatewayManager gatewayManager) {
this.gatewayManager = gatewayManager;
}
public void executeMigration() {
try {
// Backup the current configurations before migration begins.
gatewayManager.backupConfig();
// Deploy the new gateway configurations.
gatewayManager.deployNewConfig();
// Verify new gateway operations.
if (!gatewayManager.testNewGateway()) {
// Rollback in case the test fails.
gatewayManager.rollbackToPreviousConfig();
}
} catch (GatewayMigrationException e) {
// Custom exception for handling migration errors.
System.err.println("Migration failed: " + e.getMessage());
}
}
// Main method to execute the migration.
public static void main(String[] args) {
GatewayManager manager = new GatewayManager();
GatewayMigration migration = new GatewayMigration(manager);
migration.executeMigration();
}
}
In this snippet, we create a class GatewayMigration
that encapsulates the logic for migrating an API gateway. It uses a GatewayManager
object which abstracts the details of backup, deployment, and rollback operations.
Why this approach? It offers the following benefits:
- Modularity: Encapsulates the migration process, making the code easy to maintain and modify.
- Error Handling: Provides a clear path for handling failures, such as rolling back to a stable state if necessary.
- Testing: Facilitates unit testing of the migration logic separately from the rest of the application.
Leveraging Third-Party Services
While it's possible to handle this shift in-house with Java tools, third-party API management services like Amazon API Gateway or Apigee can significantly simplify the process. They provide out-of-the-box solutions for managing, deploying, and securing APIs with minimal setup (Discover more about Amazon API Gateway here).
Key Takeaways
Moving to a new API gateway doesn’t have to be a herculean task. With careful planning and the right automation strategy, Java developers can streamline this process, ensuring minimal disruption and a quick return to full operational capacity.
We've discussed why an API gateway is an essential part of a modern infrastructure, outlined steps to prepare for automation, and examined the importance of post-migration monitoring. The practical Java snippets reveal the 'why' behind the code, emphasizing modularity, error handling, and automated testing, which makes Java a powerhouse for such transitions.
Embracing automation with Java when shifting your API gateway ensures a seamless transition, reinforcing your systems against evolving business challenges and technical demands.