Troubleshoot EJB Lookup Failures: Quick Fixes Unveiled
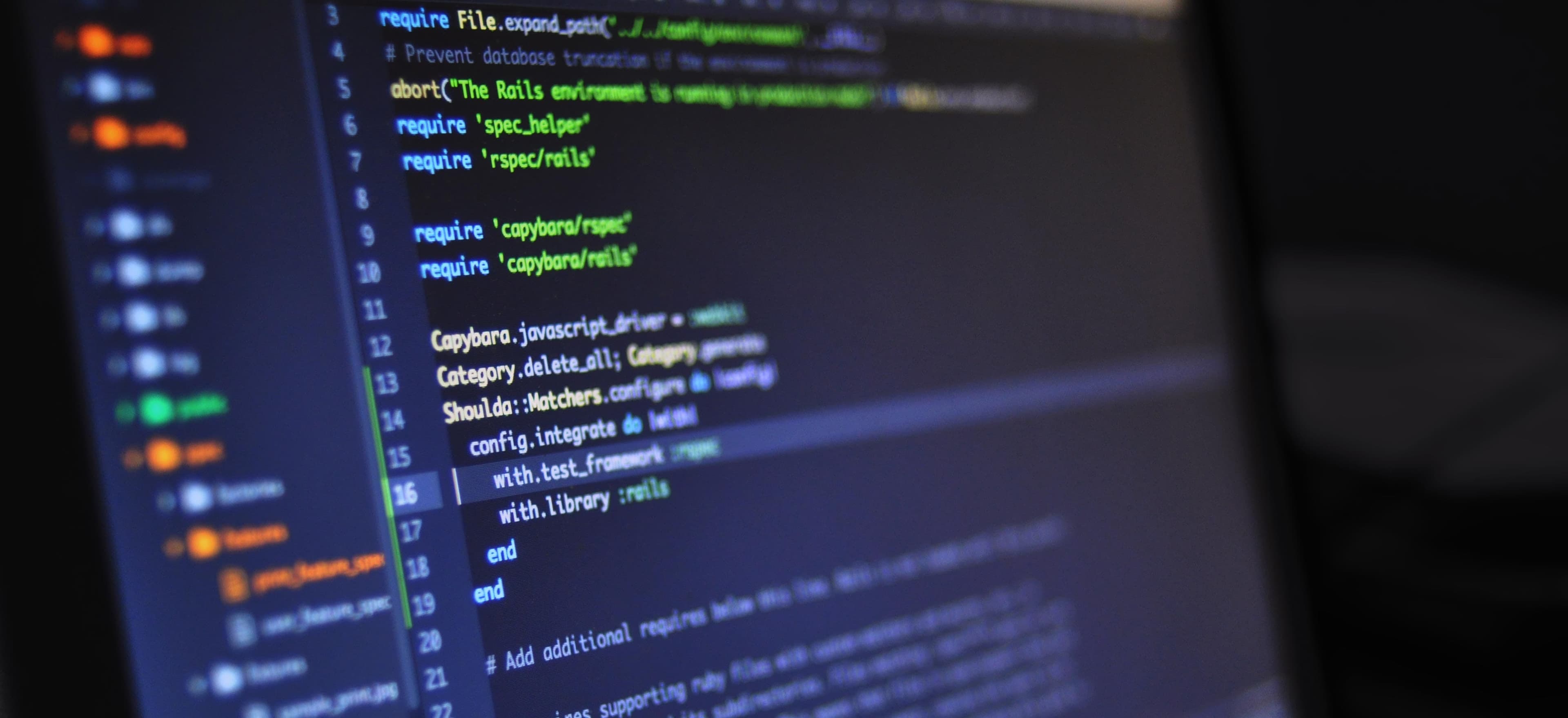
- Published on
Troubleshoot EJB Lookup Failures: Quick Fixes Unveiled
When developing enterprise applications with Java, one technology that often comes into play is Enterprise JavaBeans (EJB). EJB forms the backbone of many Java EE applications, providing a robust framework for building scalable, distributed applications. However, sometimes developers can encounter snags, specifically EJB lookup failures. Understanding and resolving these issues swiftly is crucial for maintaining productivity and ensuring the robustness of your applications.
Understanding EJB Lookup Failures
Before diving into the solutions, let's understand why EJB lookups might fail. An EJB lookup failure can occur due to a variety of reasons such as incorrect JNDI (Java Naming and Directory Interface) names, misconfigurations in the deployment descriptor, network issues, or even classloader problems. These failures halt the interaction between application components, leading to a cascade of related issues that can bring your system to a standstill.
InitialContext ctx = new InitialContext();
MyEJB myEjb = (MyEJB) ctx.lookup("java:comp/env/ejb/MyEJB");
In the snippet above, we are attempting to look up an EJB. If the JNDI name is incorrect or if there's a misconfiguration, the lookup will fail, raising an exception.
Step-by-Step Solutions
Let's explore some of the critical steps you can take to resolve EJB lookup failures and restore your application's functionality.
1. Verify JNDI Names
The most common culprit behind EJB lookup failures is an incorrect JNDI name. Each EJB is registered under a specific name in the JNDI tree, and any discrepancy will result in a NamingException
. Double-check the name specified in the @EJB
annotation or the ejb-jar.xml
descriptor file, making sure it matches the name used in the lookup.
@EJB(name="java:comp/env/ejb/MyEJB", beanInterface=MyEJB.class)
public class MyServiceImpl implements MyService {
// Business logic goes here
}
In this example, we've specified the JNDI name using the @EJB
annotation. Ensure this name is used consistently throughout your application.
2. Review Deployment Descriptors
Sometimes, the issue might lie within the deployment descriptor files such as ejb-jar.xml
or web.xml
for web applications. Confirm that your EJB references are correctly defined in these files.
<ejb-ref>
<ejb-ref-name>ejb/MyEJB</ejb-ref-name>
<ejb-ref-type>Session</ejb-ref-type>
<home>com.myapp.MyEJBHome</home>
<remote>com.myapp.MyEJB</remote>
</ejb-ref>
This XML snippet shows an EJB reference within a deployment descriptor. Check that the EJB reference name and interface settings align with what's declared in your application's code.
3. Ensure Application Server Compatibility
Different application servers might have unique requirements or naming conventions for EJBs. For instance, if you're moving from WildFly to WebSphere, the lookup strings or configuration might vary. Consulting the documentation for your specific application server is advisable to recognize any server-specific requirements.
4. Inspect Network Issues
If your EJBs are deployed on a remote server, network issues could disrupt JNDI lookups. Verifying the network connection between the client and the server, checking firewalls, and ensuring the availability of the naming service are all essential steps.
5. Resolve Classloader Conflicts
Classloader issues can also result in EJB lookup failures, particularly in complex environments where multiple versions of a class might exist. It's important to understand the classloading hierarchy of your application server and ensure that the correct versions of your classes are being loaded.
6. Debugging and Logging
Server logs can provide valuable information when troubleshooting EJB lookup issues. Most application servers have comprehensive logging mechanisms that can be configured for greater verbosity. Use these logs to trace back the exact moment the lookup fails. Turn on verbose logging for the naming subsystem, which can shed light on the problem:
Logger namingLogger = Logger.getLogger("javax.naming");
namingLogger.setLevel(Level.FINEST);
This Java snippet configures the logging level for the javax.naming
package, which handles JNDI lookups.
Tools and Resources
Besides manual checks and configurations, there are tools and resources that can augment your troubleshooting efforts:
- JNDI Browser: Many application servers offer a JNDI browser in their administrative consoles that can assist you in viewing the JNDI tree, making it easier to verify the names and structure.
- EJB Validation Utilities: Some IDEs or build tools offer utilities to validate EJB deployment descriptors and annotations against enterprise application requirements.
In Conclusion, Here is What Matters
EJB lookup failures can be a frustrating obstacle, but by methodically verifying JNDI names, reviewing deployment descriptors, checking server compatibility, inspecting network connections, and resolving classloader conflicts, you can overcome these challenges. Remember to leverage logging and use the available tools to aid in the diagnostic process.
While EJBs might feel like a component of the past, their usage is still prevalent in many enterprise systems. For modern approaches to enterprise Java, you might consider reading about Jakarta EE, the evolution of Java EE.
Finally, maintaining a vigorous and systematic approach to troubleshooting is key to success. Tackle the issue layer by layer, use the resources at hand, and keep abreast of new developments in the world of enterprise Java development. With these tactics, you'll conquer EJB lookup failures and ensure your application runs smoothly.