Breaking Free: 5 Anti-Patterns Derailing Microservices
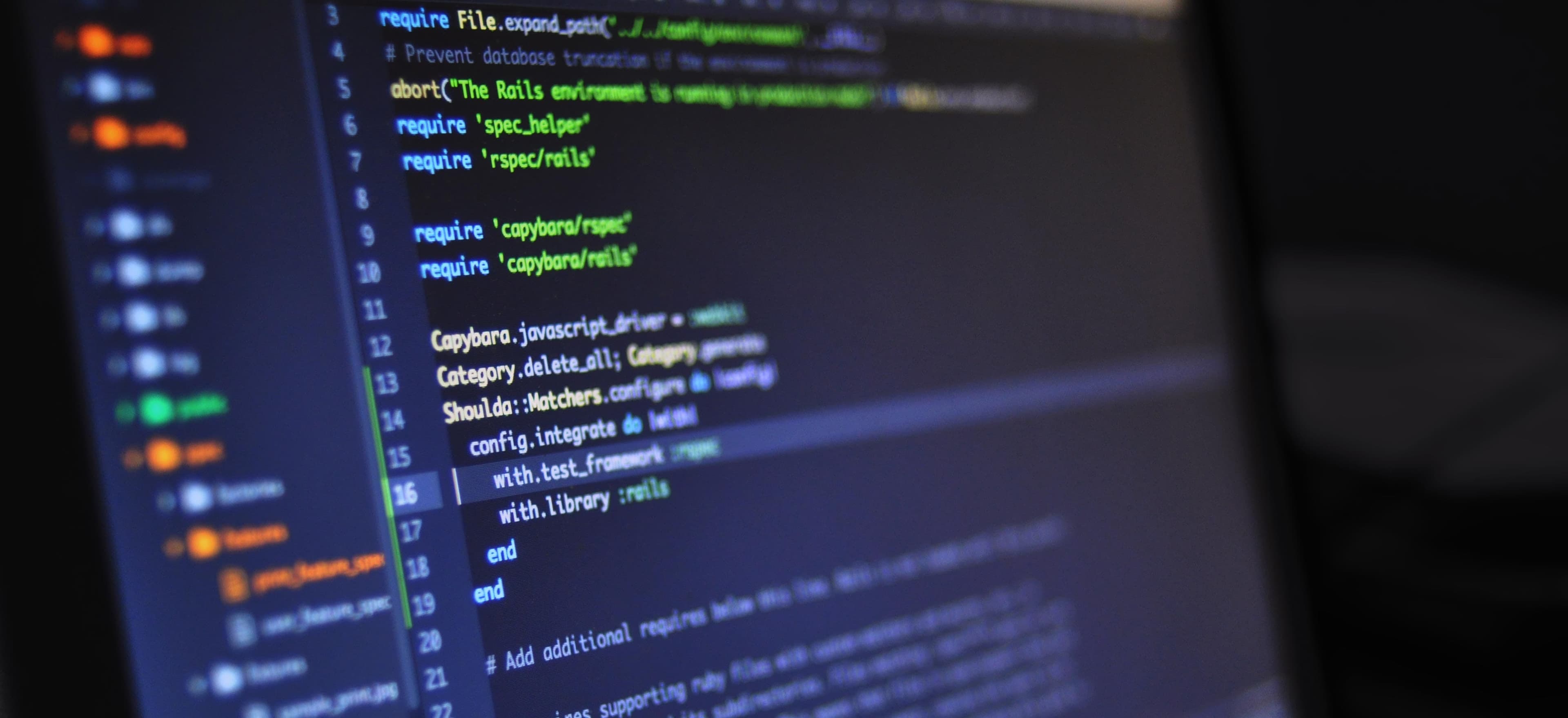
- Published on
Breaking Free: 5 Anti-Patterns Derailing Microservices in Java Applications
Microservices architecture has become the herald of modern application design, touting scalability, ease of deployment, and the flexibility to implement and deploy each service independently. However, with the wrong approach, developers can easily find themselves mired in a quagmire of anti-patterns, leading to a microservices architecture that is bulky, difficult to maintain, and challenging to scale.
In this article, we will dissect five common anti-patterns that Java developers may face when dealing with microservices, offering insights into better practices and coding examples to steer your projects toward success. This is essential for those looking to fully exploit the potential of microservices while avoiding the pitfalls that can transform a fleet-footed arrangement into a lumbering beast of burden.
Anti-Pattern 1: The Distributed Monolith
Problem: When services are too tightly coupled, the integrity of the microservices architecture crumbles into what is known as the 'Distributed Monolith'. This happens when changes in one service necessitate alterations in others, leading to a cascading series of updates that mirror the rigidity of a traditional monolith.
// Example of a tightly coupled client-service interaction in Java
public class OrderServiceClient {
private final PaymentService paymentService; // Tight coupling!
public OrderServiceClient(PaymentService paymentService) {
this.paymentService = paymentService;
}
public void processOrder(Order order) {
// ... Order processing logic
paymentService.processPayment(order.getTotal());
}
}
Solution: Promote loose coupling by relying on asynchronous communication mechanisms like message queues or events. This way, services operate independently, reacting to system-wide changes without direct dependencies.
public class OrderService {
// Use messaging for loose coupling
private MessageQueue orderQueue;
public OrderService(MessageQueue orderQueue) {
this.orderQueue = orderQueue;
}
public void processOrder(Order order) {
// ... Order processing logic
orderQueue.publish(new OrderProcessedEvent(order.getId(), order.getTotal()));
}
}
For more on microservices communication patterns, check out Martin Fowler's guide.
Anti-Pattern 2: Incorrect Service Granularity
Problem: Determining the appropriate size for a microservice is akin to an art form. Services that are too large can be unwieldy, while those that are too small can lead to unnecessary complexity and overhead. An 'incorrectly-sized' microservice strains the system and clouds the business logic.
Solution: Apply domain-driven design (DDD) principles to accurately identify service boundaries that align with business capabilities. Services should encapsulate a bounded context, being cohesive but autonomous.
No direct code snippet is provided for this segment as it's more of a design principle rather than a coding practice.
For an in-depth understanding of DDD, refer to Eric Evans' Domain-Driven Design reference.
Anti-Pattern 3: Shared Data Model
Problem: A shared data model across multiple services can create hidden dependencies that violate the principle of microservices independence. When services depend on a centralized data model, a schema change in one service can propagate issues across the application.
Solution: Each microservice should be the sole owner of its data model. Leveraging the Repository pattern helps abstract the data layer, ensuring that any persistence mechanism changes remain local to the service.
// Example of a Repository class in a microservice
public interface OrderRepository {
void saveOrder(Order order);
Order findById(Long orderId);
// Other CRUD methods
}
With each service managing its own repository, data model ownership is clear and integration points are minimized.
Anti-Pattern 4: The Omnipresent Shared Library
Problem: Libraries are essential. Yet, when microservices excessively depend on widespread shared libraries, the updates to shared code can turn into a nightmare, often requiring multiple services to be deployed simultaneously.
Solution: Minimize dependencies on shared libraries by abstracting only the truly common and stable functionality. Evolve shared libraries cautiously and version them to ensure backward compatibility.
// Wrong: A shared utility class that is overused across services
public class Utility {
public static void complexBusinessCalculation() {
// Logic that might only be relevant for specific services
}
// More utilities
}
// Right: Keeping shared libraries focused and stable
public class LoggingUtility {
public static void logMessage(String message) {
// Generic logging logic used across all microservices
}
// More essential utilities
}
Determine and carefully consider what should be shared to keep services as independent as possible.
Anti-Pattern 5: Ignoring Failures
Problem: Microservices operate over a network, which inherently introduces the possibility of communication failures. Ignoring or improperly handling these failures can lead to cascading problems in the system.
Solution: Implementing resilience patterns like timeouts, retries with exponential backoff, circuit breakers, and bulkheads is essential. Netflix’s Hystrix library, now in maintenance mode, was once the go-to solution for this. Resilience4J, a lightweight, Java 8-native alternative, has since taken up the mantle.
// Implementing a circuit breaker with Resilience4J
CircuitBreakerConfig circuitBreakerConfig = CircuitBreakerConfig.custom()
.failureRateThreshold(50)
.waitDurationInOpenState(Duration.ofMillis(1000))
.ringBufferSizeInHalfOpenState(2)
.ringBufferSizeInClosedState(2)
.build();
CircuitBreakerRegistry circuitBreakerRegistry = CircuitBreakerRegistry.of(circuitBreakerConfig);
CircuitBreaker circuitBreaker = circuitBreakerRegistry.circuitBreaker("myService");
Supplier<String> decoratedSupplier = CircuitBreaker
.decorateSupplier(circuitBreaker, () -> "This is a fallback response");
String response = Try.ofSupplier(decoratedSupplier)
.recover(throwable -> "Hello from Recovery").get();
By planning for failures and incorporating patterns to manage these scenarios, you ensure your services are robust and reliable.
In the realm of Java microservices, recognizing and rectifying anti-patterns is as important as following best practices. Avoid falling into the trap of the distributed monolith through careful decoupling, size services right with DDD, dodge the allure of shared data models, apply prudence in shared library usage, and fortify your services against failures with resilience patterns.
Bid farewell to the detriments of these anti-patterns by understanding the core principles underpinning microservices—autonomy, responsibility, and resilience. As Java developers, our craft elevates when service harmony isn't simply aspirational but practical, tangible, and free of the chains of unintentional yet burdensome dependencies.