Taming JSON with JUnit: Master Hamcrest Matchers!
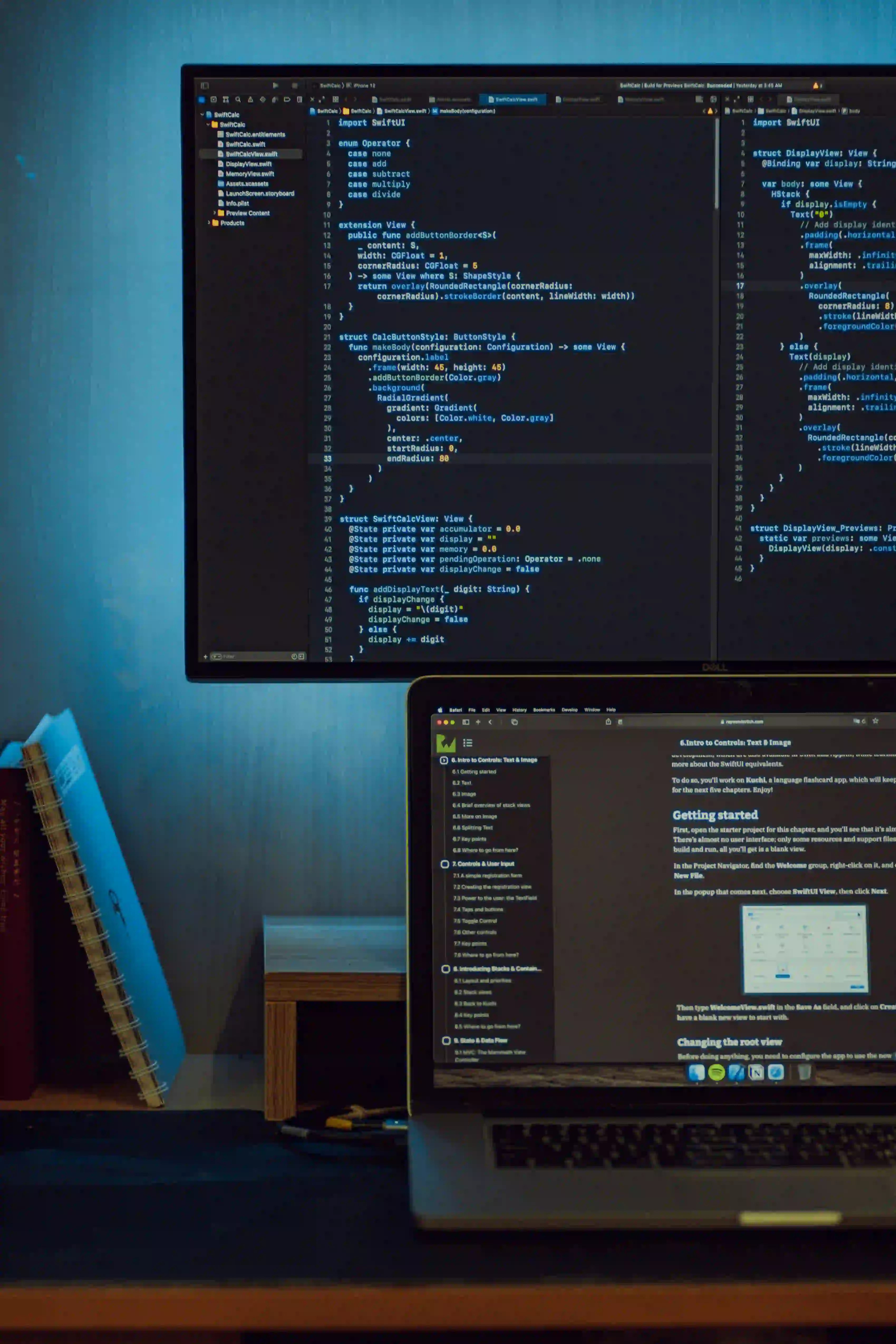
Taming JSON with JUnit: Master Hamcrest Matchers!
The Opening Bytes
JSON (JavaScript Object Notation) is a lightweight data-interchange format widely used in Java applications. It is particularly popular for APIs and data interchange due to its simplicity and flexibility. When it comes to testing Java applications, JUnit is the go-to framework for ensuring code reliability. In the context of testing JSON, the use of Hamcrest matchers can greatly enhance the readability and power of asserting JSON content. In this article, we will explore how to tame JSON with JUnit by mastering the use of Hamcrest matchers.
Understanding JSON in Java
JSON is a format that allows data to be represented in a textual format that is easily readable by both humans and machines. In Java, JSON can be represented as a string or as a hierarchical structure of maps (objects) and lists (arrays). JSON is commonly used for data interchange between different systems, such as web services, and for storing and retrieving data in databases.
In the context of Java development, working with JSON is essential when integrating with external APIs or designing APIs for your own applications. For example, when consuming a web API, you might receive JSON data in the response format. Similarly, when designing a RESTful API, you might need to accept and return JSON data.
Java provides several libraries for handling JSON, such as Jackson, Gson, and JSON-B. These libraries provide convenient APIs for parsing JSON strings into Java objects and vice versa, making it easy to work with JSON in Java applications.
The Role of JUnit in Testing
JUnit is a popular testing framework for Java applications. It provides a simple and elegant way to write unit tests, making it an essential tool for ensuring the reliability and correctness of your code.
The main purpose of JUnit is to provide a structured and standardized way to write and execute tests. With JUnit, you can define test methods that assert the expected behavior of your code. These test methods are organized into test classes, and JUnit takes care of running the tests and reporting the results.
JUnit provides a rich set of assertion methods for verifying the expected behavior of your code. These assertion methods can be used to check whether a value is equal to the expected value, whether a condition is true, or whether an exception is thrown. With JUnit, you can easily write tests that cover different scenarios and edge cases, ensuring that your code is robust and reliable.
Enter Hamcrest: The Matchers Library
Hamcrest is a library that provides a set of matchers for making assertions in tests. Matchers are objects that can be used to specify the expected behavior of your code. With Hamcrest, you can write assertions that are more readable and expressive, making it easier to understand the intent of the tests.
The beauty of Hamcrest is that it integrates seamlessly with JUnit. You can use Hamcrest matchers alongside the regular JUnit assertion methods, enhancing your tests with the added power and flexibility of Hamcrest.
Some of the benefits of using Hamcrest for testing with JSON include:
- Readability: Hamcrest matchers provide a more readable syntax for making assertions compared to traditional JUnit assertions. This makes the tests easier to understand and maintain, especially when dealing with complex JSON objects.
- Flexibility: Hamcrest matchers offer a wide range of matching capabilities, allowing you to specify the expected behavior in a more precise way. This flexibility comes in handy when asserting complex JSON structures with nested objects or arrays.
- Integration with JUnit: Hamcrest integrates seamlessly with JUnit, allowing you to combine the power of both frameworks in your tests. You can use JUnit's test annotations and lifecycle methods alongside Hamcrest matchers, resulting in more robust and comprehensive tests.
To learn more about Hamcrest and explore the available matchers, you can visit the official Hamcrest website at http://hamcrest.org/JavaHamcrest/.
Setting Up Your Environment
Before we can start using JUnit and Hamcrest matchers, we need to set up our environment. This involves adding the necessary dependencies to our project.
If you are using Maven, you can add JUnit and Hamcrest dependencies to your pom.xml
file:
<!-- Maven dependency for JUnit -->
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>5.7.2</version>
<scope>test</scope>
</dependency>
<!-- Maven dependency for Hamcrest -->
<dependency>
<groupId>org.hamcrest</groupId>
<artifactId>hamcrest</artifactId>
<version>2.2</version>
<scope>test</scope>
</dependency>
In the above example, we are adding the JUnit Jupiter API and the Hamcrest dependencies to our project. JUnit Jupiter is the latest version of JUnit and is recommended for new projects. Hamcrest is the main library for matchers and provides a wide range of matchers for different types of assertions.
If you are using Gradle, you can add the following dependencies to your build.gradle
file:
// Gradle dependencies for JUnit and Hamcrest
testImplementation 'org.junit.jupiter:junit-jupiter-api:5.7.2'
testImplementation 'org.hamcrest:hamcrest:2.2'
Similar to the Maven dependencies, these Gradle dependencies will allow us to use JUnit and Hamcrest matchers in our tests.
Writing a Basic Test with JUnit and Hamcrest Matchers
Now that we have our environment set up, let's write a basic test using JUnit and Hamcrest matchers. In this example, we have a JSON object representing a person, and we want to ensure that the object is correctly deserialized from a JSON string.
First, let's define our test class and import the necessary packages:
import org.junit.jupiter.api.Test;
import static org.hamcrest.MatcherAssert.assertThat;
import static org.hamcrest.Matchers.*;
In the above code snippet, we import the Test
annotation from the org.junit.jupiter.api
package, as well as the assertThat
method and the Matchers
class from the org.hamcrest.Matchers
package. These imports allow us to use JUnit annotations and Hamcrest matchers in our tests.
Next, let's define our test method and write the test logic:
@Test
void testDeserialization() {
String json = "{\"name\":\"John\",\"age\":30}";
Person person = Person.fromJson(json);
assertThat(person, allOf(
hasProperty("name", equalTo("John")),
hasProperty("age", equalTo(30))
));
}
In the above code snippet, we define a test method called testDeserialization
. Inside the test method, we start by creating a JSON string representing a person with the name "John" and the age of 30. Next, we deserialize the JSON string into a Person
object using a hypothetical fromJson
method.
Finally, we use the assertThat
method along with some Hamcrest matchers to perform the assertions. In this case, we use the allOf
matcher to combine multiple matchers. The hasProperty
matcher is used to assert that the Person
object has the specified properties with the expected values.
Compared to traditional JUnit assertions, the use of Hamcrest matchers makes the code more readable and expressive. It is clear from the assertion that we expect the Person
object to have the name "John" and the age of 30.
Advanced JSON Assertions with Hamcrest
In addition to basic assertions, Hamcrest provides a wide range of matchers that are well-suited for advanced JSON assertions. Let's explore some of these matchers and their usage.
equalTo: Asserting Equality of JSON
The equalTo
matcher is used to assert that the actual value is equal to the expected value. This matcher can be particularly useful when comparing JSON objects that have a known structure.
@Test
void testEquality() {
String expectedJson = "{\"name\":\"John\",\"age\":30}";
String actualJson = "{\"name\":\"John\",\"age\":30}";
assertThat(actualJson, equalTo(expectedJson));
}
In the above example, we compare two JSON strings that represent a person with the name "John" and the age of 30. The equalTo
matcher ensures that the actual JSON string is equal to the expected JSON string.
hasItem: Asserting Presence of an Element in JSON Array
The hasItem
matcher is used to assert that a JSON array contains a specific element. This matcher can be useful when testing APIs that return an array of JSON objects and you want to verify that a certain object is present.
@Test
void testArrayContainsPerson() {
String jsonArray = "[{\"name\":\"John\",\"age\":30},{\"name\":\"Jane\",\"age\":25}]";
assertThat(jsonArray, hasJsonPath("$[?(@.name == 'John')]"));
}
In the above example, we have a JSON array that contains two person objects. Here, we use the hasJsonPath
matcher to assert that the array contains an object with the name "John". The hasJsonPath
matcher allows us to specify a JSONPath expression to match against the JSON structure.
hasKey: Asserting Presence of a Key in JSON Object
The hasKey
matcher is used to assert that a JSON object contains a specific key. This matcher is useful when testing APIs that return a JSON object and you want to verify the presence of certain properties.
@Test
void testObjectHasKey() {
String jsonObject = "{\"name\":\"John\",\"age\":30}";
assertThat(jsonObject, hasKey("name"));
}
In the above example, we have a JSON object representing a person. We use the hasKey
matcher to assert that the object contains a key named "name".
Dealing with Complex JSON Structures
JSON structures can often be complex, with nested objects and arrays. When testing these complex structures, Hamcrest matchers can simplify the process and make the assertions more readable.
Asserting Nested Objects
To assert the properties of nested objects in JSON, we can use the hasProperty
matcher along with the hasKey
matcher.
@Test
void testNestedObject() {
String json = "{\"person\":{\"name\":\"John\",\"age\":30}}";
assertThat(json, hasJsonPath("$.person"));
assertThat(json, hasJsonPath("$.person.name", equalTo("John")));
assertThat(json, hasJsonPath("$.person.age", equalTo(30)));
}
In the above example, we have a JSON object with a nested object representing a person. We use the hasJsonPath
matcher to assert that the JSON object has a property named "person". Then, we use the hasJsonPath
matcher again to assert the properties of the nested object.
Asserting Arrays of Objects
When asserting arrays of objects, we can use the hasItem
matcher along with the hasProperty
matcher.
@Test
void testArrayofObjects() {
String jsonArray = "[{\"name\":\"John\",\"age\":30},{\"name\":\"Jane\",\"age\":25}]";
assertThat(jsonArray, hasJsonPath("$[0]"));
assertThat(jsonArray, hasItem(allOf(
hasProperty("name", equalTo("John")),
hasProperty("age", equalTo(30))
)));
}
In the above example, we have a JSON array containing two person objects. We use the hasJsonPath
matcher to assert the presence of the first element in the array. Then, we use the hasItem
matcher along with the allOf
matcher to assert the properties of the first person object.
Best Practices for Testing JSON with Hamcrest
When using Hamcrest matchers to test JSON in Java, it's important to keep a few best practices in mind:
-
Isolate Tests: As with any unit testing, it is important to isolate each test case and make sure they are independent of each other. This ensures that failures or changes in one test case do not impact other test cases.
-
Make Readable Assertions: Hamcrest matchers provide a more fluent and readable syntax for assertions. Take advantage of this by writing assertions that clearly express the expected behavior of the code. This makes it easier for other developers to understand the intent of the tests.
-
Limit Scope of Matchers: While Hamcrest provides a wide range of matchers for different types of assertions, it is important to limit the scope of matchers used in each assertion. This helps to keep the tests focused and avoids unnecessary complexity.
Bringing It All Together
In this article, we have explored the power of using JUnit combined with Hamcrest matchers for testing JSON in Java. We started by understanding the significance of JSON in Java applications and the role of JUnit in testing. We then introduced Hamcrest matchers and demonstrated their capabilities in asserting JSON content.
Using Hamcrest matchers, you can write more expressive and readable tests, making it easier to understand the intent of the tests. With the ability to handle complex JSON structures and a wide range of matchers available, Hamcrest provides a valuable tool for testing JSON in Java.
I encourage you to experiment with Hamcrest matchers in your own tests and explore the versatility they offer. By leveraging JUnit and Hamcrest together, you can master the art of testing JSON in Java and ensure the reliability and correctness of your code.
Further Reading and Resources
To learn more about JUnit, Hamcrest, and JSON processing libraries, here are some additional resources:
- JUnit 5 User Guide: The official user guide for JUnit 5, providing detailed documentation and examples.
- Hamcrest Tutorial: A comprehensive tutorial on using Hamcrest matchers for different types of assertions.
- Jackson JSON Processor: The official GitHub repository for the Jackson JSON processing library, providing a powerful and flexible way to handle JSON in Java.