Mastering Spring Boot: Testing Conditionals Effectively
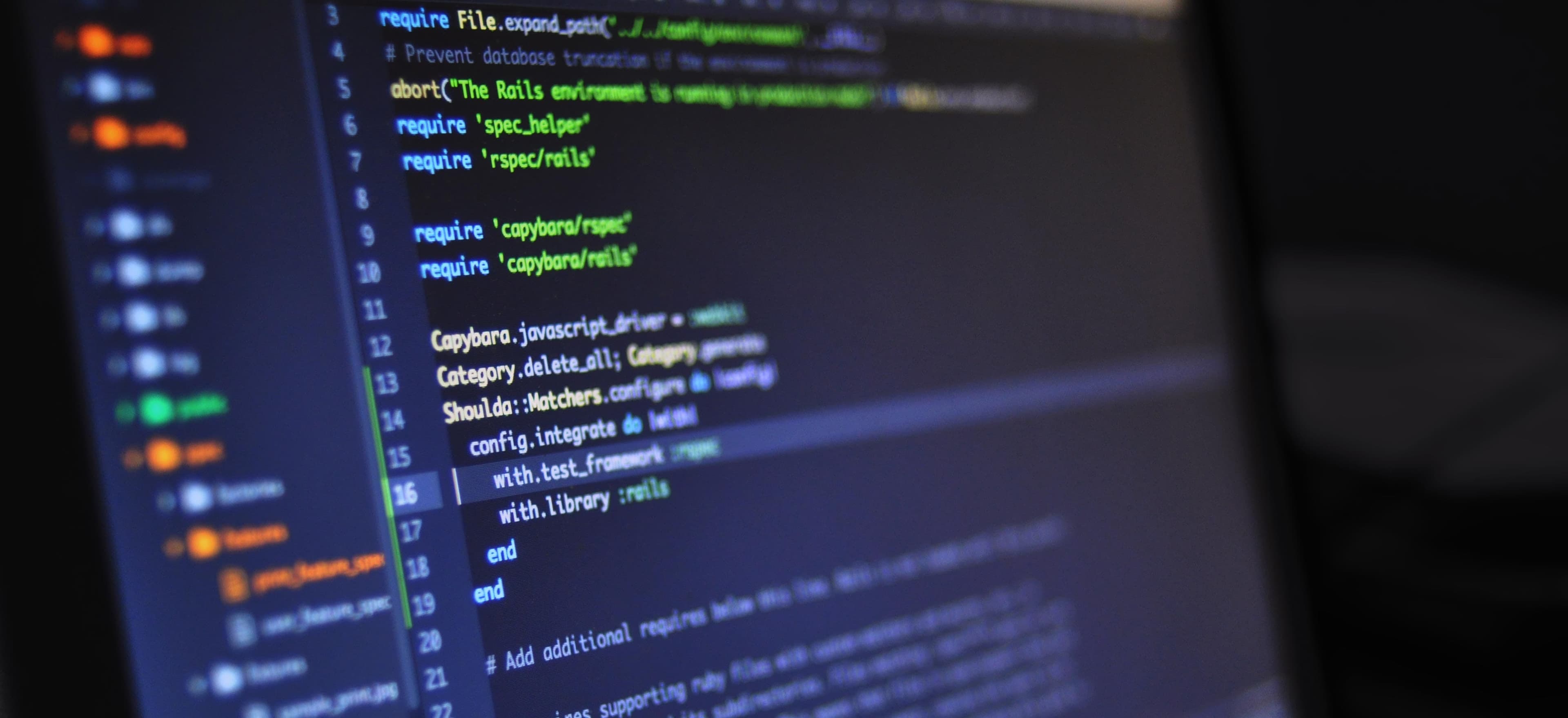
- Published on
Mastering Spring Boot: Testing Conditionals Effectively
When developing Spring Boot applications, it is common to have different configurations or behaviors based on certain conditions. These conditions may depend on the environment (e.g., development, production), the presence of certain dependencies or beans, or any other criteria.
In this blog post, we will explore how to effectively test conditionals in Spring Boot applications. We will discuss various techniques and strategies that will help you ensure the correctness and reliability of your application's logic.
Why Test Conditionals?
Testing conditionals is crucial because they define the behavior of your application under different circumstances. These conditionals may determine whether certain features are enabled or disabled, specific configurations are applied, or certain components are instantiated or skipped.
By testing conditionals, you can verify that your application is correctly behaving in different scenarios, ensuring that the expected logic is executed and unwanted behavior is avoided. This is especially important if you have multiple branches or conditions in your codebase, as it can lead to hidden bugs or unexpected behavior if not adequately tested.
Unit Testing Conditionals
When testing conditionals at the unit level, we focus on testing individual components or classes in isolation. This allows us to verify the behavior of specific parts of the application without dependencies on other components or the Spring Boot environment.
Using Mockito for Mocking Dependencies
To effectively test conditionals, it is often necessary to mock dependencies that are used in the conditional logic. Mocking frameworks like Mockito provide a convenient way to create mock objects that behave as expected during tests.
Consider the following example, where we have a UserService
that performs some logic based on the presence of an EmailService
:
public class UserService {
private EmailService emailService;
public UserService(EmailService emailService) {
this.emailService = emailService;
}
public void sendWelcomeEmail(User user) {
if (emailService != null) {
emailService.sendEmail(user.getEmail(), "Welcome to our platform!");
}
}
}
To test the conditional behavior of sendWelcomeEmail
, we can mock the EmailService
and verify if the sendEmail
method is called when the emailService
is not null:
public class UserServiceTest {
@Mock
private EmailService emailService;
@InjectMocks
private UserService userService;
@BeforeEach
public void setup() {
MockitoAnnotations.openMocks(this);
}
@Test
public void testSendWelcomeEmail_withEmailService() {
User user = new User("john@example.com");
userService.sendWelcomeEmail(user);
verify(emailService, times(1)).sendEmail(user.getEmail(), "Welcome to our platform!");
}
@Test
public void testSendWelcomeEmail_withoutEmailService() {
User user = new User("john@example.com");
userService.setEmailService(null);
userService.sendWelcomeEmail(user);
verify(emailService, never()).sendEmail(anyString(), anyString());
}
}
In this example, @Mock
is used to create a mock EmailService
, and @InjectMocks
is used to inject the mock into the UserService
. The verify
method is then used to check if the sendEmail
method is called under the expected conditions.
Using ConditionalOnProperty
Spring Boot provides an annotation called @ConditionalOnProperty
, which allows you to conditionally enable or disable certain components or configurations based on the value of a property.
To test conditionals based on @ConditionalOnProperty
, you can use the org.springframework.boot.test.context.SpringBootTest
annotation to load the relevant configurations. By providing different property values, you can test the behavior under different conditions.
Consider the following example, where we have a PaymentService
that is enabled or disabled based on a property called payment.enabled
:
@Service
@ConditionalOnProperty(value = "payment.enabled", havingValue = "true")
public class PaymentService {
// payment service logic...
}
To test the conditional behavior of PaymentService
, you can create a test class and provide different property values using the @SpringBootTest
annotation:
@SpringBootTest(properties = {
"payment.enabled=true"
})
public class PaymentServiceTest {
@Autowired
private PaymentService paymentService;
@Test
public void testPaymentServiceEnabled() {
// test logic when payment.enabled=true
}
}
In this example, the @SpringBootTest
annotation is used to enable the loading of application context, and the properties
attribute is used to set the payment.enabled
property value accordingly.
By providing different property values, you can test the behavior of the conditional logic under various scenarios.
Integration Testing Conditionals
In addition to unit testing, it is also important to test conditionals at the integration level. Integration tests allow you to verify the behavior of your application as a whole, including the interaction between components and the Spring Boot environment.
Using @ConditionalOnBean
The @ConditionalOnBean
annotation is another powerful tool provided by Spring Boot. It allows you to conditionally enable or disable certain components or configurations based on the presence or absence of a bean in the application context.
To test conditionals based on @ConditionalOnBean
, you can create an integration test class and manually configure the application context to include or exclude certain beans.
Consider the following example, where we have a NotificationService
that depends on the presence of EmailService
and SmsService
beans:
@Service
@ConditionalOnBean({EmailService.class, SmsService.class})
public class NotificationService {
// notification service logic...
}
To test the conditional behavior of NotificationService
, you can create an integration test class and configure the application context accordingly:
@SpringBootTest
public class NotificationServiceTest {
@Autowired(required = false)
private NotificationService notificationService;
@Test
public void testNotificationServiceEnabled() {
// test logic when EmailService and SmsService beans are present
assertNotNull(notificationService);
}
}
In this example, the @SpringBootTest
annotation is used to enable the loading of application context. The required = false
attribute is used to indicate that the NotificationService
bean is optional. Therefore, if the required beans are present, the NotificationService
bean will be instantiated and available for testing.
By manually configuring the application context, you can test the behavior of the conditional logic when certain beans are present or absent.
A Final Look
Testing conditionals in Spring Boot applications is essential to ensure the correctness and reliability of your application's behavior under different conditions. By using techniques such as mocking dependencies, using @ConditionalOnProperty
, and @ConditionalOnBean
, you can effectively test the behavior of your conditionals at the unit and integration levels.
Remember to consider all possible branches and scenarios when writing your test cases, and ensure that you cover both positive and negative cases to achieve thorough test coverage.
Mastering the art of testing conditionals in Spring Boot applications will greatly contribute to the stability and robustness of your codebase, enabling you to confidently release high-quality software.
Further reading:
- Introduction to Unit Testing in Java
- Mockito Documentation
- Spring Boot Testing Reference Guide
- Testing Conditional Beans in Spring Boot
Happy coding and testing!