Fixing Spring Data Binding Issues in Thymeleaf
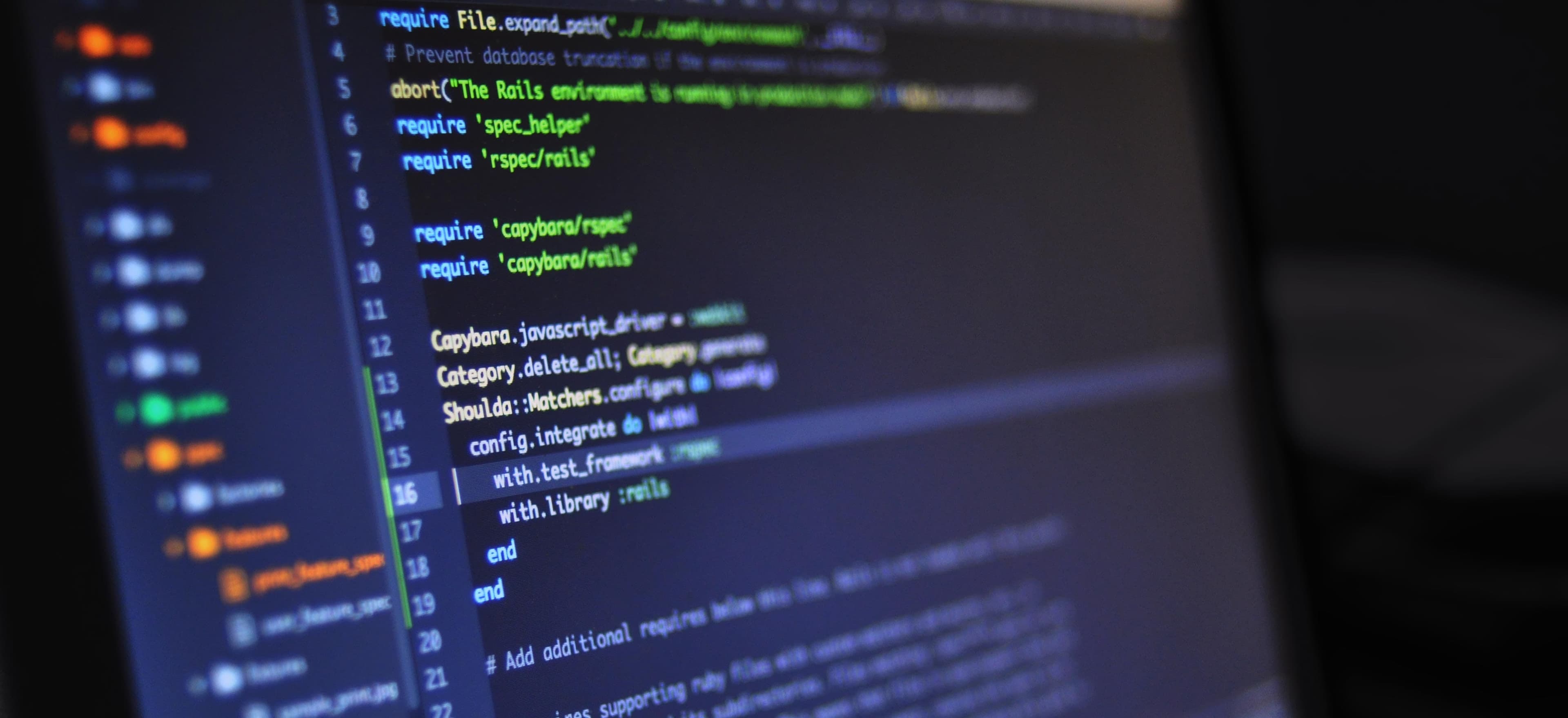
- Published on
Fixing Spring Data Binding Issues in Thymeleaf
!Thymeleaf and Spring
The Opening Bytes
Purpose: The purpose of this article is to explain the common data binding issues faced in Spring with Thymeleaf and provide solutions to resolve them. By the end of this article, readers will have a better understanding of best practices and specific fixes for data binding issues when working with Spring and Thymeleaf.
Background: Spring is a popular Java framework that is used to build enterprise-scale applications. It provides a comprehensive and flexible platform for developing web applications, including features like dependency injection and MVC architecture. Thymeleaf, on the other hand, is a modern server-side Java templating engine that integrates seamlessly with Spring and provides powerful data binding capabilities.
Problem Statement: When using Spring and Thymeleaf together, there can be some common data binding issues that developers may encounter. These issues can range from handling form submissions to complex object bindings and date/number formatting. This article aims to address these issues and provide solutions to overcome them.
Preview Solution: In this article, we will start by explaining the concept of data binding and how it works in the context of Spring and Thymeleaf. We will then proceed to discuss the common data binding issues and their solutions, providing step-by-step instructions and code samples. Additionally, we will explore advanced data binding techniques, such as custom converters and formatters, and show how to handle dynamic forms with collections. Finally, we will cover debugging techniques to help diagnose and fix data binding issues effectively.
Prerequisites
To make the most out of this article, readers should have the following prerequisites:
- Spring Framework Knowledge: It is recommended to have a basic understanding of the Spring Framework and the MVC pattern it follows.
- Thymeleaf Experience: Familiarity with Thymeleaf templating engine will be helpful in understanding the examples and solutions provided.
- Java Development Environment: Ensure that you have a working Java development environment, including tools like Java JDK, Maven or Gradle, and an IDE of your choice.
Understanding Data Binding in Spring and Thymeleaf
What Is Data Binding?
Data binding is the process of connecting the data from the user interface (UI) to the backend of an application. In the context of web applications, data binding is essential for handling form submissions and capturing user inputs. When a user submits a form, the data entered in the form fields needs to be bound to the corresponding variables or objects on the server side.
In Spring, data binding is facilitated by the use of model attributes and controllers. Model attributes are objects that hold the data to be displayed or processed in a view. These attributes are populated by the controller and can be accessed by the Thymeleaf template for rendering or binding purposes.
Thymeleaf's Integration with Spring
Thymeleaf is a server-side Java templating engine that offers seamless integration with the Spring Framework. It allows developers to write dynamic and expressive templates that can be easily bound to Spring model attributes.
Thymeleaf leverages the power of Spring's data binding capabilities by enabling developers to bind form inputs directly to model attributes. This allows for efficient data handling and eliminates the need for manual mapping between form inputs and data objects.
To illustrate this integration, consider the following example of a basic Thymeleaf form binding to a Spring model:
<form action="#" th:action="@{/save}" th:object="${person}" method="post">
<input type="text" th:field="*{firstName}" />
<input type="text" th:field="*{lastName}" />
<button type="submit">Save</button>
</form>
In the above example, the th:field
attribute is used to bind the form inputs to the corresponding properties of the person
model attribute. When the form is submitted, Spring will automatically bind the values entered in the form fields to the person
object.
Common Data Binding Issues and Their Solutions
In this section, we will explore the most common data binding issues that developers may encounter when working with Spring and Thymeleaf. For each issue, we will provide a detailed explanation, list the common symptoms, and offer step-by-step solutions along with code snippets.
Issue 1: Field Errors After Submission
Description: One common issue when working with form submissions is the appearance of field errors after submitting the form. This typically happens when there are validation errors or failed data conversions in the submitted form data.
Common Symptoms:
- Form fields showing error messages or highlighting in red.
- Error messages being displayed next to the respective form fields.
Replicable Code Snippet:
To replicate this issue, consider the following code snippet:
Spring Controller:
@Controller
public class UserController {
@PostMapping("/save")
public String saveUser(@ModelAttribute("user") User user, BindingResult bindingResult) {
// Perform validation and data processing
// ...
if (bindingResult.hasErrors()) {
return "user-form";
} else {
return "success";
}
}
}
Thymeleaf Template (user-form.html
):
<form action="#" th:action="@{/save}" th:object="${user}" method="post">
<input type="text" th:field="*{username}" />
<span th:if="${#fields.hasErrors('username')}" th:errors="*{username}"></span>
<input type="password" th:field="*{password}" />
<span th:if="${#fields.hasErrors('password')}" th:errors="*{password}"></span>
<button type="submit">Save</button>
</form>
Code Commentary:
In the above code snippet, we have a Spring controller that handles the form submission. The @PostMapping
annotation maps the request to the /save
endpoint, where the submitted form data is bound to the User
object using the @ModelAttribute
annotation. The BindingResult
argument is used to capture any errors during data binding or validation.
The Thymeleaf template user-form.html
renders the form fields, and the th:field
attribute binds the form inputs to the corresponding properties of the User
object. The th:if
and th:errors
attributes are used to display field-specific error messages.
Solution and Best Practices
Step-by-Step Solution: To fix the issue of field errors appearing after form submission, follow these steps:
- Add validation annotations to the relevant properties in the
User
class. For example, to validate theusername
property, you can use the@NotBlank
annotation:
public class User {
@NotBlank(message = "Username is required")
private String username;
// Other properties and getters/setters
}
- In the Spring controller, add the
@Valid
annotation before the@ModelAttribute
annotation to enable validation:
@PostMapping("/save")
public String saveUser(@Valid @ModelAttribute("user") User user, BindingResult bindingResult) {
// Perform validation and data processing
// ...
if (bindingResult.hasErrors()) {
return "user-form";
} else {
return "success";
}
}
- Modify the Thymeleaf template to display the field errors:
<form action="#" th:action="@{/save}" th:object="${user}" method="post">
<input type="text" th:field="*{username}" />
<span th:if="${#fields.hasErrors('username')}" th:errors="*{username}"></span>
<!-- Other form fields -->
<button type="submit">Save</button>
</form>
Why It Works: The above solution works by adding validation annotations to the User
class. These annotations ensure that the form data meets the specified criteria (e.g., not blank, within a certain range).
When the form is submitted, Spring performs data binding and validation. If there are any errors, they are captured in the BindingResult
object. Thymeleaf's th:if
and th:errors
attributes are then used to display the error messages next to the respective form fields.
Best Practices: To avoid field errors after form submission, consider the following best practices:
- Use validation annotations such as
@NotBlank
,@NotEmpty
, or@Size
to enforce constraints on form inputs. - Include informative and user-friendly error messages with the validation annotations.
- Ensure that the properties in the bound object have appropriate nullability and default values to avoid unexpected behavior.
- Test form submissions with both valid and invalid data to catch any potential issues.
Advanced Data Binding Techniques
In addition to the common data binding issues, there are some advanced techniques that can be helpful when working with Spring and Thymeleaf. In this section, we will explore two such techniques: custom converters and formatters, and handling dynamic forms with collections.
Subsection 1: Custom Converters and Formatters
Introduce the concept of custom converters and formatters in Spring.
Show how to write a custom converter/formatter and use it in a Thymeleaf template.
Subsection 2: Dynamic Forms with Collections
Explain how to handle dynamic forms, such as forms with variable numbers of fields.
Demonstrate creating and binding a form to a list of items.
Debugging Data Binding Issues
- Explain Tools and Methods
- Tips and Tricks
Closing Remarks
In this article, we have explored the common data binding issues faced when using Spring with Thymeleaf and provided solutions to overcome them. We discussed the concept of data binding and how Spring and Thymeleaf facilitate this process. We then addressed common issues such as field errors after submission, binding complex objects, date and number formatting, handling null values in forms, and binding nested objects.
We also explored advanced data binding techniques, including custom converters and formatters, and dynamic forms with collections. Additionally, we provided tips and tricks for debugging data binding issues effectively.
We hope this article has been helpful in resolving data binding issues and improving your experience in developing Java web applications with Spring and Thymeleaf. If you have any comments, questions, or would like to share your experiences, please leave a comment below.
Additional Resources
- Spring Framework Documentation
- Thymeleaf Documentation
- Baeldung - Spring Data Binding
- Baeldung - Thymeleaf Form Handling
- Official Spring Boot website
Checkout our other articles