Ensuring Kids' Safety in Android Group Activities
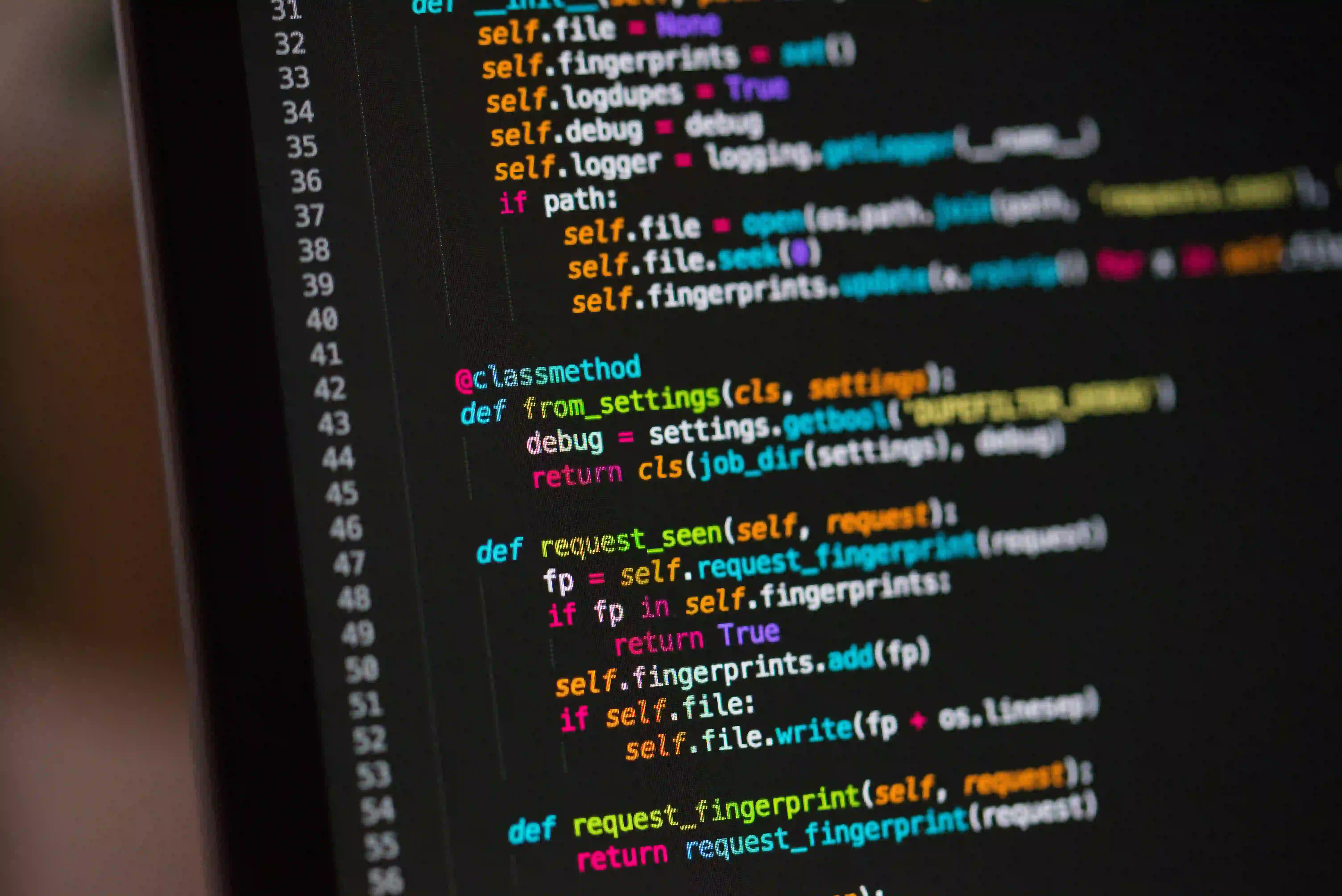
Ensuring Kids' Safety in Android Group Activities: Implementing Parental Controls
In today's connected world, mobile devices have become a staple in the lives of children and adolescents, making the responsibility of protecting them from unsuitable content and ensuring their safety during group activities an ever-growing challenge. Particularly within Android applications, which often foster communal interactions, developers must prioritize features that enable parents to maintain oversight and control.
Understanding the Need for Parental Controls in Android Apps
Young users are often involved in group activities through apps, whether they're collaborative educational games, messaging platforms, or social media. While these interactions can be enriching, they also expose children to potential risks like inappropriate content, cyberbullying, or interaction with strangers.
Implementing parental controls in Android apps helps mitigate these risks by allowing parents to monitor and manage their children's app usage. But what does it entail from a programming standpoint?
Starting with Android's Built-in Solutions
Android provides built-in APIs and services that can aid in developing robust parental controls. For instance, Google's Family Link lets parents set digital ground rules and monitor app activities. As a developer, using these existing frameworks not only saves time but also ensures compliance with Google's recommended practices.
For more advanced features, delve into the Android application programming interface (API) that offers comprehensive support for user accounts, permissions, and content restrictions.
Let's explore how to tap into these features to create a safer environment for kids.
Sample Code: Setting Up User Profiles
import android.content.Context;
import android.os.UserManager;
public class ProfileManager {
private Context context;
public ProfileManager(Context context) {
this.context = context;
}
public void createUserProfile(String name) {
UserManager userManager = (UserManager) context.getSystemService(Context.USER_SERVICE);
if (userManager != null && userManager.isSystemUser()) {
userManager.createUser(name, UserManager.USER_TYPE_FULL_RESTRICTED);
}
}
}
This code snippet demonstrates how to programmatically create a new restricted user profile, which parents can customize with specific limitations on app usage and content access.
Going a Step Further: Custom Implementations
While built-in solutions are a good starting point, custom implementations give you the freedom to tailor parental controls to the specifics of your app. Let's dive into a few key areas and how to implement them.
Monitoring Activity With Custom Logs
Logs serve as a record of user activity and can be invaluable to parents keeping tabs on their children's app interactions.
Sample Code: Implementing Activity Logs
import java.util.Date;
public class ActivityLogger {
// Method to record user activity
public static void logActivity(String userId, String activityType, String details) {
// Assume there's a method to store these logs appropriately
storeLog(userId, new Date(), activityType, details);
}
private static void storeLog(String userId, Date timestamp, String activityType, String details) {
// Logic to store log entries, possibly in a cloud database, local storage, etc.
// This could include writing to a log file, sending to a server, etc.
}
}
With the logActivity
method, you can track various actions within your app, such as message exchanges or group events. It's crucial to ensure that this information is stored securely and is only accessible by authorized users, respecting both privacy and security best practices.
Setting Permissions and Restrictions
Creating permission structures within your app can help you control what content is accessible to different user accounts and under what conditions it can be accessed.
Sample Code: Setting Permissions
import java.util.HashMap;
import java.util.Map;
public class PermissionManager {
private Map<String, Boolean> permissions;
public PermissionManager() {
permissions = new HashMap<>();
}
public void setPermission(String userId, String permission, boolean isAllowed) {
permissions.put(userId + ":" + permission, isAllowed);
}
public boolean isAllowed(String userId, String permission) {
return permissions.getOrDefault(userId + ":" + permission, false);
}
}
In this example, your app might have a functionality that calls isAllowed
before allowing users to participate in certain group activities, ensuring that only children with the appropriate permissions from their parents can access them.
Communication Filtering
For apps that feature messaging or interaction, a filtering mechanism can prevent exposure to harmful content or language.
Sample Code: Message Filtering
public class MessageFilter {
private static List<String> bannedWords = Arrays.asList("badword1", "badword2"); // This should be more comprehensive.
public static String filterMessageContent(String originalContent) {
String filteredContent = originalContent;
for (String word : bannedWords) {
if (filteredContent.toLowerCase().contains(word)) {
filteredContent = filteredContent.replaceAll("(?i)" + word, "***");
}
}
return filteredContent;
}
}
In the filterMessageContent
method, we look for banned words and replace them with asterisks, ensuring that children aren't exposed to inappropriate language. This list should be thorough and continuously updated to keep up with the evolving landscape of online communication.
Collaborating with Parents and Caregivers
Developing intricate parental controls is futile unless parents are aware and able to use these features effectively. Including tutorials, help guides, or even dedicated support can be pivotal in bridging the gap between the app's capabilities and parental involvement.
Balancing Safety with Privacy and User Experience
Incorporating safety mechanisms should not come at the cost of violating user privacy or hindering user experience. It is imperative to strike a balance by implementing controls that respect users' rights to privacy and provide a seamless user experience.
Complying with Legal Standards
Developers must be vigilant in complying with legal standards such as the Children's Online Privacy Protection Act (COPPA) in the United States and the General Data Protection Regulation (GDPR) in the European Union, which set strict guidelines for collecting and handling children's personal information.
Conclusion
Implementing sophisticated parental controls in Android group activities requires careful consideration and a multi-faceted approach. By leveraging Android's built-in APIs and crafting bespoke features designed for your specific application, developers can create a safer digital space for children without infringing on their privacy or enjoyment.
Nurturing the delicate balance between supervision and independence is key; the goal is to empower parents to protect their children while allowing young users to explore and benefit from the positive aspects of communal digital experiences.
It's not just about writing code; it's about creating a responsible digital environment where our younger generation can thrive safely. After all, ensuring the safety of children in an increasingly digital world is not just the task for parents and guardians alone – it’s a collective responsibility that starts with the development of mindful, secure, and engaging applications.