Fixing Cold Start Delays in AWS Lambda Functions
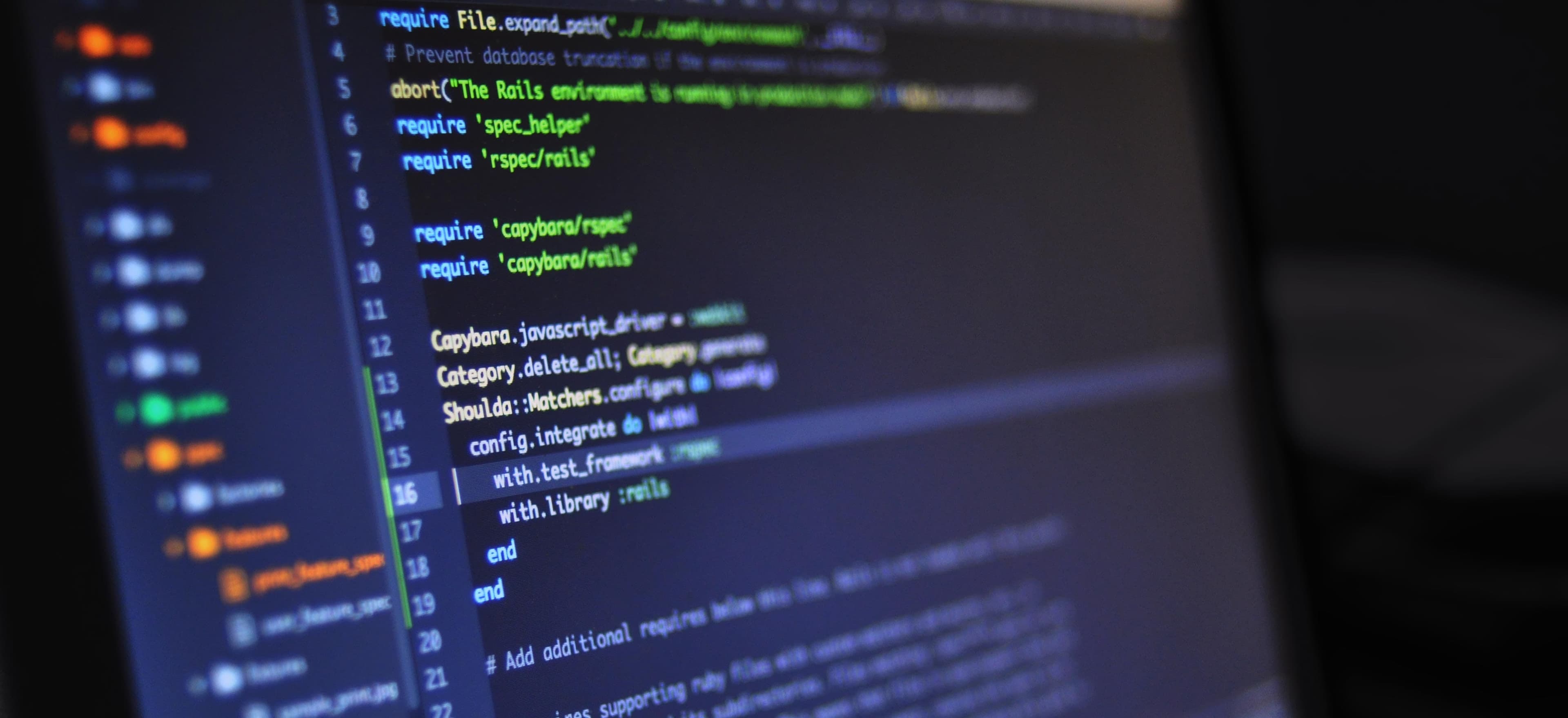
- Published on
Fixing Cold Start Delays in AWS Lambda Functions for Java Developers
As a Java developer diving into the world of serverless architecture on AWS Lambda, you may have experienced the notorious 'cold start' issue. This latency during function initialization not only impacts user experience but also plays a critical role in the overall performance of your serverless applications. Fortunately, with a handful of optimization strategies and best practices, you can significantly reduce cold start times, ensuring a smoother operation for your Lambda-based services.
What is a Cold Start?
In the realm of AWS Lambda, a cold start occurs when a function is invoked after not being used for a prolonged period, resulting in longer execution times as the service has to instantiate a new execution context.
Why Cold Starts Occur:
- Lack of a pre-warmed container
- Extended initialization time for runtime and code
- Time taken to establish database connections
Quickening the Warm-up: Techniques for Java Developers
Java, being a statically typed, compiled language, often faces longer cold starts compared to dynamically typed, interpreted languages. However, Java developers are not condemned to suffer these delays silently. Let's explore actionable solutions to combat cold starts in AWS Lambda.
1. Keep the Lambda Functions Warm
"Prewarming" your Lambda functions can mitigate cold starts. This is achieved by periodically invoking your Lambda function to ensure it stays active. Here's a simple example of how to keep a Lambda function warm using CloudWatch Events:
public class KeepWarmHandler implements RequestHandler<Map<String,Object>, String> {
public String handleRequest(Map<String,Object> data, Context context) {
// Detect the "warm-up" trigger
if (data.containsKey("keepWarm")) {
return "Warming up the function";
}
// Handle the actual business logic here
// ...
return "Business logic executed";
}
}
By scheduling a CloudWatch Event to trigger this function every 5-10 minutes with a keepWarm
flag, you can ensure that your Java Lambda function stays warm.
2. Reduce Your Deployment Package Size
The size of your deployment package significantly affects your Lambda's cold start time. Declutter your deployment package:
- Remove unused dependencies.
- Use tools like ProGuard to minimize the JAR file.
- Leverage the AWS Lambda Layer feature to share common dependencies across functions.
3. Optimize JVM Start-up Time
Optimizing the start-up time of the Java Virtual Machine (JVM) can lead to shorter cold starts. Utilize the following JVM options:
-XX:+UseContainerSupport -XX:MaxRAMFraction=2
These options enable the JVM to better operate in a containerized environment and optimize memory allocation.
4. Use Native Compiled Languages with GraalVM
Compiling Java code ahead-of-time (AOT) to a native binary using tools like GraalVM can significantly reduce the start-up time since it eliminates the JVM start-up overhead. Here's how you would run a basic Java application using GraalVM:
native-image -cp myapp.jar com.example.MyApp
Your compiled application can be packaged into an AWS Lambda deployment package.
5. Adjust the Function's Memory Size
Contrary to what you might think, adjusting your function's memory size influences not just memory but CPU and network throughput as well. Experimenting with the memory allocation to find a balance is key.
6. Embrace Async Initialization
In Java, static block initialization can delay function readiness. Asynchronous initialization can spread out the loading effort:
public class AsyncInitHandler implements RequestHandler<APIGatewayProxyRequestEvent, APIGatewayProxyResponseEvent> {
private static CompletableFuture<HeavyResource> heavyResourceFuture = CompletableFuture.supplyAsync(() -> {
// Initialize a resource that's expensive to create
return new HeavyResource();
});
public APIGatewayProxyResponseEvent handleRequest(APIGatewayProxyRequestEvent request, Context context) {
heavyResourceFuture.join(); // Forces the handler to wait only if the resource is not yet ready
// ...
}
}
While your handler method is still synchronous, by leveraging Java's CompletableFuture
, we're ensuring heavy resources are initialized only once, saving time on subsequent invocations.
7. Application Frameworks
Modern application frameworks like Quarkus or Micronaut are designed to minimize cold start times and memory usage for Java applications. These frameworks support compiling Java code to native executables with GraalVM or having fast booting JVM-based artifacts.
// A simple REST endpoint in Quarkus
@Path("/hello")
public class HelloResource {
@GET
@Produces(MediaType.TEXT_PLAIN)
public String hello() {
return "hello";
}
}
This code represents a lightweight REST endpoint that would have a very short cold start time when run on AWS Lambda.
8. Reassess Your Architecture
Sometimes, the solution isn't in the code. Considering an event-driven architecture or breaking down your application into smaller, single-purpose Lambda functions can minimize the impact of cold starts.
9. Take Advantage of Provisioned Concurrency
AWS Lambda allows you to configure Provisioned Concurrency. With this setting, you can maintain a specific number of pre-initialized function instances, completely eliminating cold starts for a predictable workload.
10. Monitor and Log
Keep a close watch on performance metrics using AWS CloudWatch. Establishing a robust logging and monitoring strategy will help you identify cold start patterns and devise solutions.
LambdaLogger logger = context.getLogger();
logger.log("Cold start detected!"); // Log a message if a cold start occurs
Conclusion
Despite its challenges, AWS Lambda's serverless paradigm still offers compelling benefits, such as scalability and cost-efficiency. By optimizing for Java's specific needs, you can significantly mitigate cold start issues. Remember, the key to a performant serverless Java application lies in continuous profiling, optimization, and integration of best practices. Happy coding!
Further Reading
- AWS Lambda Documentation
- ProGuard
- GraalVM
- AWS Blog - Optimizing Lambda Performance
- Quarkus
- Micronaut
Next time you encounter a cold start delay, tackle it head-on with these strategies. Remember, a smooth user experience starts with an efficiently running back-end – optimize your Lambda functions and keep your serverless Java applications performing at their best.
Checkout our other articles