Securing Privacy: Mastering Data Anonymization Techniques
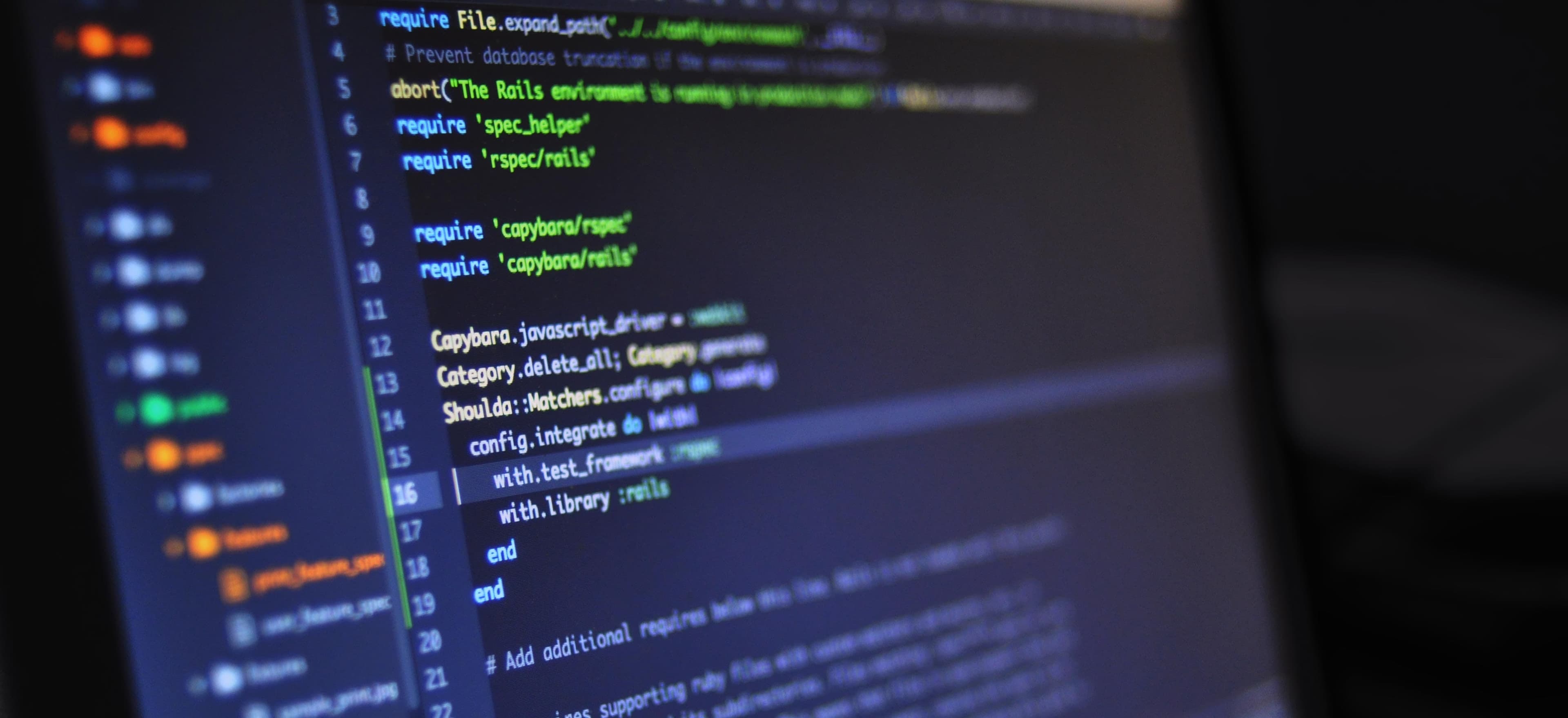
- Published on
Securing Privacy: Mastering Data Anonymization Techniques in Java
In today's data-driven world, where big data analytics and machine learning walk hand-in-hand, protecting individual privacy has risen prominently in the public conscience. Data anonymization stands at the forefront of this privacy battleground, providing techniques to mask personal identifiers from data sets. Let's explore how Java, one of the most utilized programming languages for enterprise-scale applications, serves as a tool for effectively implementing data anonymization.
Understanding Data Anonymization
Data anonymization is the process of protecting private or sensitive information by erasing or encrypting identifiers that connect an individual to stored data. This technique is not just a protective measure; it’s a requirement under laws like GDPR and HIPAA. By anonymizing data, organizations can utilize datasets while upholding privacy standards.
Java and Anonymization: A Perfect Pair?
Java's strict type system, robust standard libraries, and vast ecosystem of third-party libraries make it an excellent choice for data anonymization tasks. Java's platform-independent nature means anonymization code written in Java can be deployed across a multitude of environments, which is invaluable for businesses operating on a global scale.
Implementing Anonymization in Java
We'll walk through various anonymization techniques using Java, delving into the 'why' behind each coding strategy to ensure you can apply these methods effectively in your data processing workflows.
Masking Personal Identifiers
One of the most straightforward anonymization techniques is to mask data. This involves replacing characters in a string (like a name or email) with a placeholder.
public static String maskEmail(String email) {
int atIndex = email.indexOf("@");
if (atIndex == -1) return email; // Not a valid email, return unchanged
String domain = email.substring(atIndex);
return "****" + domain; // Masks the username part
}
Why this code? The maskEmail
function hides the user portion of an email address, leaving the domain visible for analytical purposes. This balance maintains partial data integrity while fulfilling anonymization requirements.
Generalization of Data
Generalization reduces the precision of data, thereby increasing privacy. For example, replacing an exact birth date with just a year.
public static String generalizeDateOfBirth(String dob) {
String[] parts = dob.split("-");
if(parts.length < 3) return dob; // Not a valid date, return unchanged
return parts[0]; // Returns only the year part
}
Why this code? The generalizeDateOfBirth
function takes a date string and returns just the year, providing a rough approximation of age without revealing the exact birthday.
Data Shuffling
Data shuffling involves permuting values within a dataset to dissociate the original context while maintaining the overall statistical distribution.
public static void shuffleList(List<String> data) {
Collections.shuffle(data);
}
Why this code? The shuffleList
method provided by Java's Collections framework can be used to shuffle data values, thus disassociating sensitive data from particular records.
Pseudonymization
Pseudonymization replaces private identifiers with fake identifiers or pseudonyms. This allows the dataset to be matched with its original records if the pseudonym mapping is retained.
public static String pseudonymizeUser(String userName, Map<String, String> pseudonymMap) {
return pseudonymMap.getOrDefault(userName, userName);
}
Why this code? This pseudonymizeUser
method swaps the actual usernames with pseudonyms using a provided mapping. This is useful when data must be reversible yet non-identifiable to unauthorized parties.
Encryption
While not strictly anonymization, encrypting data with a key can provide reversible anonymity.
import javax.crypto.Cipher;
import javax.crypto.SecretKey;
import javax.crypto.spec.SecretKeySpec;
public static byte[] encryptData(byte[] data, byte[] keyBytes) throws Exception {
SecretKey key = new SecretKeySpec(keyBytes, "AES");
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, key);
return cipher.doFinal(data);
}
Why this code? Encryption is a robust method for protecting sensitive information. The encryptData
function uses the AES algorithm, which is a standard for secure information exchange.
Differential Privacy
Differential privacy adds mathematical noise to aggregate data, ensuring individual entries do not affect the output significantly.
public static double addDifferentialPrivacy(double value, double sensitivity, double epsilon) {
double noise = Math.random() * (sensitivity / epsilon);
return value + noise;
}
Why this code? The addDifferentialPrivacy
method is an example of injecting controlled noise into the data. The epsilon
parameter controls the trade-off between privacy and data accuracy.
Limitations and Considerations
Anonymizing data in Java is powerful, but it's not without its challenges. Developers must be vigilant about potential data leaks, be it through inference attacks or improper implementation of anonymization methods. Additionally, the choice of technique must align with the desired level of data utility and legal requirements.
Further Resources
To deepen your knowledge of data anonymization and privacy in Java, explore the following resources:
- Oracle Java SE Documentation
- Open Web Application Security Project (OWASP)
- Java Cryptography Extension (JCE)
Conclusion
Data anonymization in Java is a critical skill for software developers who process sensitive information in the age of privacy. With a clear understanding of the techniques and best practices highlighted in this article, you can confidently tackle the task of protecting user privacy while still unlocking the potential of data analytics.
By responsibly implementing the data anonymization methods presented, you can achieve the delicate balance between data utility and privacy, ensuring your Java applications are both powerful and privacy-compliant. Remember, while anonymized data can greatly reduce privacy risks, it's crucial to stay informed of the latest security advancements and legal guidelines to continually safeguard against data vulnerabilities.