Unlocking Android Assets: Easier File Reading Secrets!
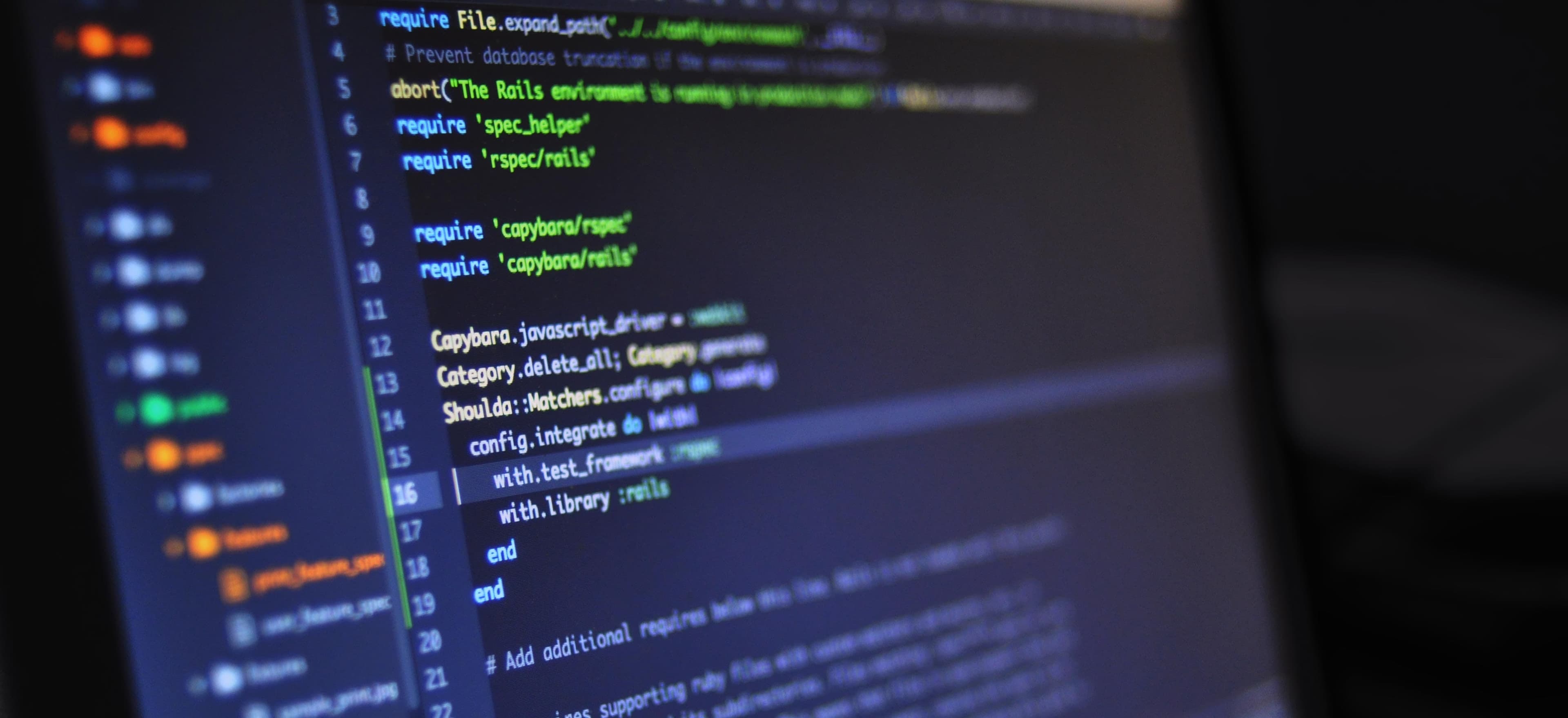
- Published on
Unlocking Android Assets: Easier File Reading Secrets!
Android development can be as thrilling as it is complex, especially when you delve into the intricate task of asset management. The AssetManager in Android is a powerful ally, granting developers the ability to read files seamlessly from the 'assets' folder. But, as many seasoned Android developers know, working with files can be a tricky endeavor. That's where this guide comes in – to illuminate the path to easier file reading in Android, and contribute to your app's smooth and efficient performance.
Why Focus on the 'assets' Folder?
The 'assets' folder in an Android project is a special directory where you can store files that you want to include in your Android application package (APK). Unlike resources, assets can be read directly, allowing for the flexibility to include files such as custom fonts, text files, or configuration data.
Benefits of Using 'assets':
- Flexibility: Store any filetype.
- Maintainability: Keep complex data or configurations out of your code.
- Performance: Stream large files without needing to preload them into memory.
Accessing and Reading Files from 'assets'
Here's where the magic happens! Accessing files from the 'assets' folder involves the use of AssetManager
. Let's dive into the specifics.
Step 1: Getting an Instance of AssetManager
Firstly, you'll need an AssetManager
instance. This instance is typically obtained from the application's context.
AssetManager assetManager = context.getAssets();
Simple and straight to the point: the getAssets()
method retrieves the necessary object that allows us to dive into the assets directory.
Step 2: Reading a File from 'assets'
Now onto the reading. Suppose you've got a configuration file config.txt
that needs to be read. Here's an exemplary method to accomplish that.
public String loadConfigFile(String filename, AssetManager assetManager) throws IOException {
StringBuilder configContent = new StringBuilder();
try (InputStream is = assetManager.open(filename);
BufferedReader reader = new BufferedReader(new InputStreamReader(is))) {
String line;
while ((line = reader.readLine()) != null) {
configContent.append(line).append('\n');
}
}
return configContent.toString().trim();
}
Here's the breakdown:
assetManager.open(filename)
opens anInputStream
to the designated file.- We wrap this stream with a
BufferedReader
for efficiency during reading. - Looping through each line, we build up a
StringBuilder
with the file's contents. - Finally, we close resources automatically using try-with-resources, limiting memory leaks.
Why try-with-resources
? It ensures that all resources opened will be closed automatically when the block is exited, which is crucial for preserving your application's performance and preventing resource leaks.
Example Usage
try {
String configData = loadConfigFile("config.txt", assetManager);
// Do something with your config data
} catch (IOException e) {
e.printStackTrace();
// Handle exception
}
This snippet demonstrates reading our config.txt
utilising the method we created. The try-catch block ensures robustness, capturing any anomalies related to file reading operations.
Best Practices for Efficient Asset Management
While reading files from assets might seem straightforward, following best practices can prevent headaches down the line.
- Keep it Organized: Structuring your assets folder with subdirectories can help maintain a tidy workspace.
- Avoid Hardcoding: Use constants or configuration settings for file paths and names, making changes less error-prone.
- Stream Large Files: For large assets, consider streaming the file content instead of loading it entirely into memory to avoid OutOfMemory errors.
Additional Tips and Tricks
Using Asset File Descriptors
Sometimes, you may need to work with APIs that require FileDescriptor
instead of streams. Android's AssetManager
has got your back!
AssetFileDescriptor descriptor = assetManager.openFd("sound.mp3");
FileInputStream fileStream = descriptor.createInputStream();
// Use the fileStream as needed and close when done.
This opens a file descriptor for the asset, allowing you to utilize it with APIs, such as media players, which require raw file descriptors.
Handling Asset Updates
What if your assets need updating without shipping a whole app update? Consider downloading assets to the app's internal storage or cache directory on the fly.
File internalFile = new File(context.getFilesDir(), "downloaded_config.txt");
// Use appropriate methods to download and save the file to internalFile.
This strategy provides flexibility for dynamic content and can be a lifesaver for applications that require regular updates.
Conclusion
Mastering file reading from Android's 'assets' folder can empower your apps with rich media and complex configuration without cluttering your codebase. It's a technique that enhances both the functionality and professionalism of your app. Remember to handle files responsibly and your assets will turn into valuable treasures that enrich the user experience.
By following the insights and practices we've shared, you're now equipped with the essential knowledge to utilize the Android AssetManager
to its fullest. Happy coding!
Need more clarity or further information? Dive into the Android documentation on AssetManager to explore additional capabilities and nuanced details of file handling on Android.
Feel free to comment below for any questions or share your own experiences with Android's AssetManager
. And if you found this blog post useful, share it with fellow Android developers!