Navigating Challenges of Bounded Context in DDD
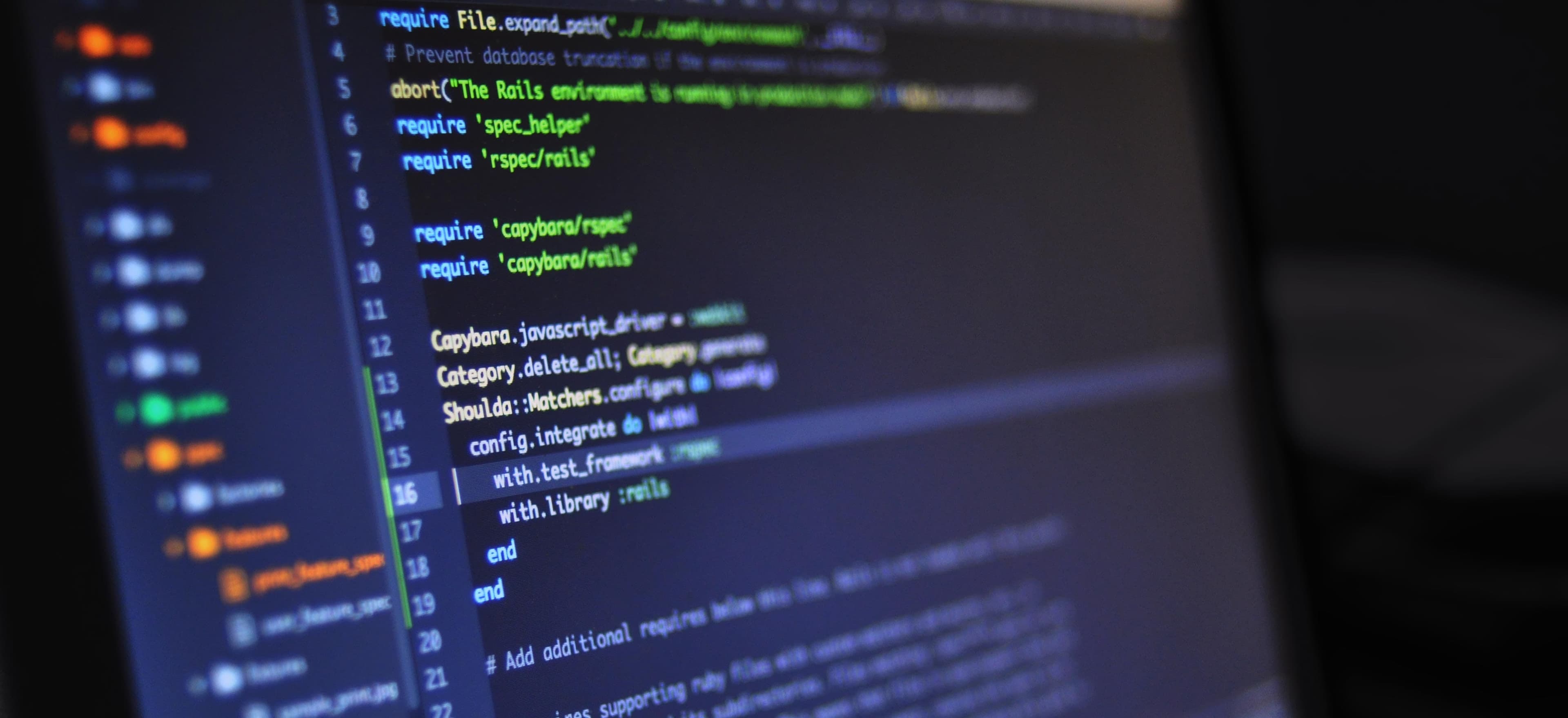
- Published on
Understanding and Overcoming Bounded Context Challenges in Domain-Driven Design (DDD)
When diving into complex software systems, Domain-Driven Design (DDD) emerges as a beacon, guiding the structuring and design of software to reflect the complexities and intricacies of the real-world domain it aims to model. But, as all sailors navigating treacherous waters must understand the complexities of their charts, so too must software developers comprehend one of the most critical yet challenging components of DDD: the Bounded Context. Mastering Bounded Context can be the difference between a well-architected system and a tangled web of dependencies.
Grasping the Bounded Context Concept
Bounded Context is a central pattern in Domain-Driven Design that defines a conceptual boundary within which a particular domain model is defined and applicable. It serves as a linguistic and conceptual framework for discussions between team members and stakeholders, ensuring clarity and consistency.
Why Bounded Context Matters
- Reduces Complexity: By dividing the system into manageable chunks, developers can focus on one context at a time.
- Increases Cohesion: Ensures that all elements within the boundary relate closely to one another.
- Fosters Clear Communication: Establishes a common language suited for each specific context.
- Improves Flexibility: Independent contexts allow for separate scalability and technology choices.
If neglected, this DDD tenet could result in muddy communication, tangled codebases, and a software model that fails at representing real-life scenarios.
Overcoming Bounded Context Challenges
Addressing the hurdles associated with implementing Bounded Context requires a diligent approach to software design. Here are several strategies to conquer the common challenges.
Define Context Boundaries Explicitly
A clear definition of where one Bounded Context ends, and another begins avoid confusion. This can be done through:
- Context Mapping: Creating a visual representation of how different contexts interact with each other.
- Ubiquitous Language: Developing a domain-specific language that's exclusive to each Bounded Context.
- Documentation: Keeping a relevant and updated documentation that explains the boundaries and rules of each context.
Code Snippet - Context Mapping
For example, consider a simple e-commerce application with distinct contexts for Ordering
and Inventory
.
// In the Ordering context
public class Order {
// Order-related properties and methods
}
// In the Inventory context
public class Product {
// Inventory-related properties and methods
}
// ContextMap can illustrate how these two separate contexts interact
public class ContextMap {
private final Order order;
private final Product product;
public ContextMap(Order order, Product product) {
this.order = order;
this.product = product;
}
// Methods to translate or transfer data between the two contexts
}
In this snippet, Order
and Product
classes are distinct to their respective contexts. The ContextMap
provides a bridge between these two bounded contexts.
Handle Context Integration Carefully
When bounded contexts need to interact, which they often do, the integration must be handled to preserve each context's integrity. Techniques to facilitate this include:
- Anti-Corruption Layer (ACL): A layer that prevents unwanted noise and ensures external systems can communicate without corrupting the context's ubiquitous language.
- Shared Kernel: A common subset of the domain model shared between multiple bounded contexts.
- Open Host Service: An API that translates the internal model into a more generalized one understood by other contexts.
Code Snippet - Anti-Corruption Layer
Below is a simplified example of an Anti-Corruption Layer.
// In the Ordering context, Assuming another system will communicate with our order system.
public class OrderServiceACL {
private final ExternalOrderService externalOrderService;
public OrderServiceACL(ExternalOrderService externalService) {
this.externalOrderService = externalService;
}
public Order translateToOrder(ExternalOrder externalOrder) {
// Conversion logic here
return new Order(/* parameters based on the conversion */);
}
}
The OrderServiceACL
ensures that any external communication is translated into terms understood by the Ordering
context without jeopardizing the model's integrity.
Embrace Continuous Refinement
Bounded Contexts are not set in stone; they must evolve. Regularly reviewing and refining the contexts ensures they continue to fit the domain's realities. This practice includes:
- Feedback Loops: Establishing ongoing communication with domain experts to keep domain models accurate.
- Refactoring: Continuously improving the code within a bounded context to align with changing domain insights.
Navigate Technology Transitions
If one bounded context uses a different stack or database than another, it can lead to integration challenges. Using the right integration patterns and understanding both the limitations and capabilities of different technologies is essential.
- Polyglot Persistence: Using different data storage technologies that are best suited to each context's needs.
- API Gateway Pattern: Implementing an API Gateway to provide a single entry point for different bounded contexts, especially in a microservices architecture.
Keep Teams Aligned
In large development environments, different teams may handle different Bounded Contexts. Ensuring alignment between teams is crucial for maintaining a cohesive domain model across contexts.
- Cross-Functional Teams: Encouraging collaboration between domain experts, developers, and QA to foster understanding of the bounded context.
- Inter-Team Communication: Establishing protocols such as Scrum of Scrums can help sync progress and challenges across teams working on separate contexts.
Conclusion
Bounded Context is a cornerstone of effective Domain-Driven Design and can be the pillar upon which the success of a complex software project rests. By explicitly defining context boundaries, handling context integration with precision, embracing continuous refinement, carefully navigating technology transitions, and keeping teams aligned, developers can harness the full power of DDD.
In closing, consider Bounded Context as not just a technical mandate but as an exercise in empathy. It's a tool for understanding the domain, preserving its integrity in software form, and facilitating better communication among teams. With the insights and code examples provided, you are better equipped to navigate the nuanced seas of Bounded Context, ensuring your voyage through the world of software development remains steadfast and true. To further explore the depth of Domain-Driven Design, Eric Evans' seminal book, Domain-Driven Design: Tackling Complexity in the Heart of Software, is an invaluable resource.
Remember, the art of programming lies not just in coding but in creating models that live and breathe the essence of the domain they represent. Bounded Context is your compass; use it wisely.
Checkout our other articles