Mastering Software Localization: One Vital Lesson Learned
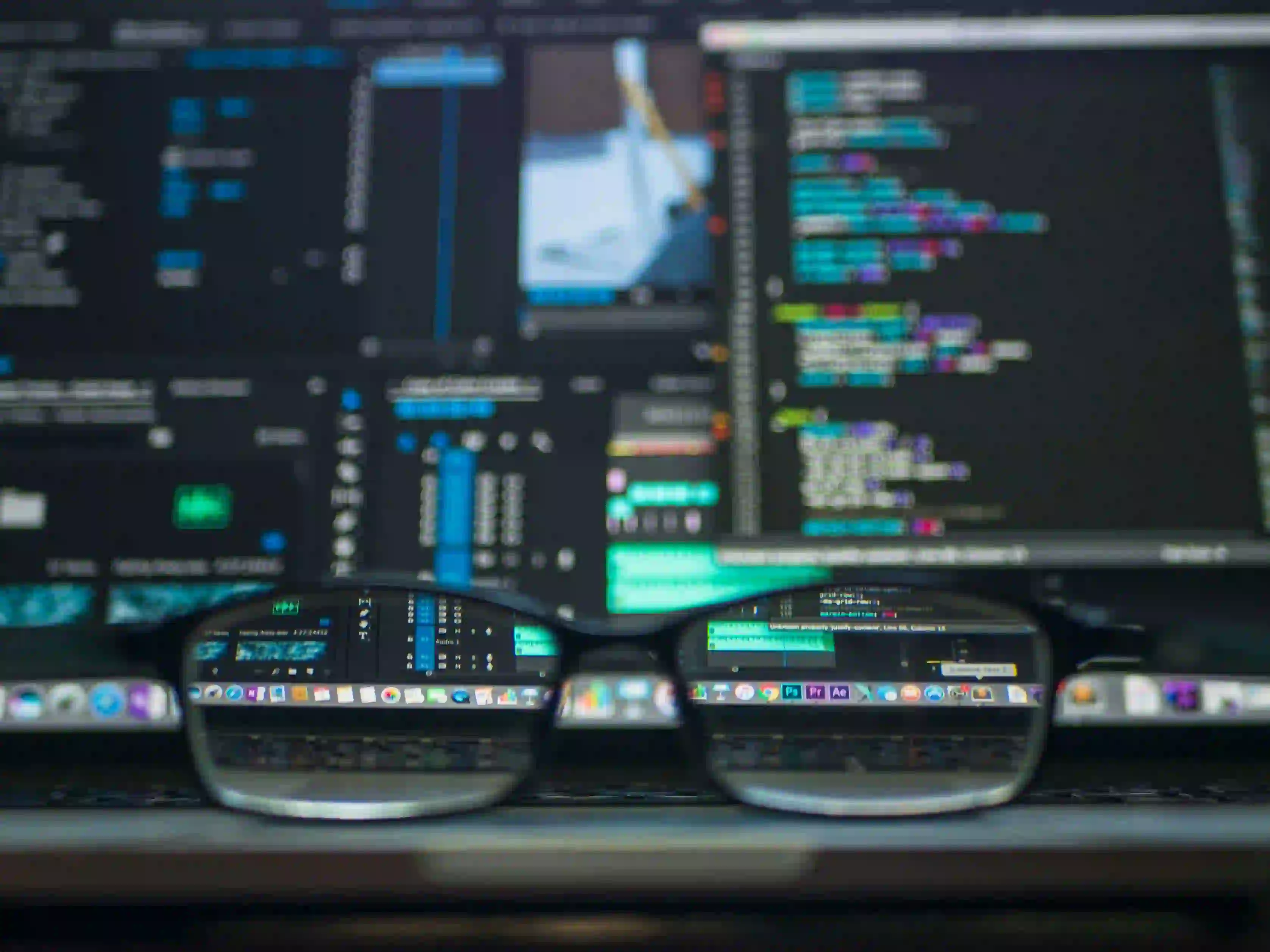
Mastering Software Localization: One Vital Lesson Learned
Software localization is essential to scaling your Java application globally. Without careful consideration to this aspect, your otherwise brilliant application might fail to resonate with users across different geographies due to language and cultural barriers. In our exploration of Java localization practices, we highlight a paramount lesson that is often overshadowed by technical specifics: empathy.
Sink into the User’s Shoes: Empathy in Code
It’s not just about translating text or switching date formats. Localization involves immersing into the user's culture and understanding the nuances—something machines can't fully capture. In practice, this means not just replacing strings but adapting the user experience entirely.
Here are a few strategies that reflect this overarching lesson:
- Integrate Internationalization (i18n) from the Start
Design your application with global users in mind right from the start. This doesn't just save development time down the line; it fosters an inclusive design mindset.
// An example of defining resource bundles for different locales
ResourceBundle messages = ResourceBundle.getBundle("MessagesBundle", currentLocale);
Why? This code snippet highlights the use of Java’s ResourceBundle
class, which abstracts out locale-specific data (like strings) from your codebase, making it easy to adapt your application for different languages later on.
- Test Locales Thoroughly
Real-world testing is more than crucial; it's a window into user’s contexts. Don’t just automate; get real feedback from local users.
Locale testLocale = new Locale("es", "ES");
// assume we have a method that takes the locale and performs tests
performLocalizationTest(testLocale);
Why? Here we create a Locale
object for testing. The method performLocalizationTest
signifies the process of ensuring your localization works for the specific locale. This ensures your testing isn't just theoretical.
- Be Mindful of External Content
Your application might pull data from external APIs or databases. Never assume their content is localization-ready.
// Fetching external content and applying locale-based formatting
String externalData = fetchData();
String localizedData = applyLocaleFormatting(externalData, userLocale);
Why? This exemplifies the need to not only localize your static content but also any dynamic content fetched. The function applyLocaleFormatting
would tackle locale-specific formatting concerns, ensuring consistency across all data presented to the user.
- Use Unicode Encoding
Unicode is the lingua franca of text encoding, supporting practically all scripts.
// Writing to a file with UTF-8 encoding
try (Writer out = new BufferedWriter(new OutputStreamWriter(new FileOutputStream("out.txt"), StandardCharsets.UTF_8))) {
out.write(localizedContent);
}
Why? This snippet uses UTF-8 encoding, a type of Unicode, which is vital for supporting an extensive array of characters used in different languages. This avoids encoding issues that can arise when using character sets that don’t support certain languages.
- Don’t Concatenate Translated Strings
Sentence structures vary by language. Concatenation can ruin the meaning.
// Bad practice - concatenation can lead to grammatical errors in different languages
String greeting = "Hello " + userName + ", welcome back!";
// Good practice - use MessageFormat or similar methods that consider locale grammar
String pattern = getMessagePattern("welcomeMessage", userLocale);
String greeting = MessageFormat.format(pattern, userName);
Why? MessageFormat.format
ensures that tokens for replacements (like {0}
) are manipulated according to grammatical rules of the target language, whereas simple concatenation does not.
The Technical Backbone of Localization in Java
With the empathy aspect covered, let’s dig into the technical gears of software localization in Java.
Utilizing Java’s Locale Class
The Locale
class is your primary tool for managing language-specific objects. It encapsulates specific geographical, cultural, and political preferences of a user.
Locale spanishLocale = new Locale("es", "ES");
Resource Bundles: Your Dictionary for Internationalization
Resource bundles store locale-specific objects, like text and images. Java looks up the appropriate bundle based on the given Locale
.
ResourceBundle bundle = ResourceBundle.getBundle("Messages", new Locale("en", "US"));
String greeting = bundle.getString("greeting");
Learn more about Java's ResourceBundle
Formatting Data Types Based on Locale
NumberFormat
and DateFormat
are classes in Java that take Locale
into account for formatting numbers and dates, respectively.
NumberFormat currencyFormatter = NumberFormat.getCurrencyInstance(locale);
DateFormat dateFormatter = DateFormat.getDateInstance(DateFormat.LONG, locale);
String formattedCurrency = currencyFormatter.format(1000000);
String formattedDate = dateFormatter.format(new Date());
Character Encoding: Unicode and UTF-8
Java uses Unicode natively, which simplifies string management across different languages. It is always advisable to use UTF-8 when dealing with I/O operations to maintain consistency and prevent data corruption.
Right to Left (RTL) Support
Languages such as Arabic and Hebrew are written from right to left. Java’s java.awt.Component
class provides the applyComponentOrientation
method to adapt the layout accordingly.
Component c = ...;
c.applyComponentOrientation(ComponentOrientation.RIGHT_TO_LEFT);
Keys to Success
Here are key takeaways for developing a localized Java application:
- Start Early: Bake localization into the architecture from day one.
- User Testing: Regularly test with real users from diverse locales.
- Continuous Learning: Stay updated with Java's latest localization features.
- Tools and Services: Utilize Java-based localization tools and services like Transifex or Crowdin.
In conclusion, while technicalities form the spine of localization, empathy muscles them to life. Practice coding with empathy, and your Java application will not just function across borders; it will belong there.
Remember, software localization isn't a checkmark on a to-do list; it's a fundamental element that allows your application to interact meaningfully with a diverse user base. By implementing these strategies and tips, you can avoid common pitfalls and build a Java application that excels globally.