Boosting Performance with Java Virtual Threads!
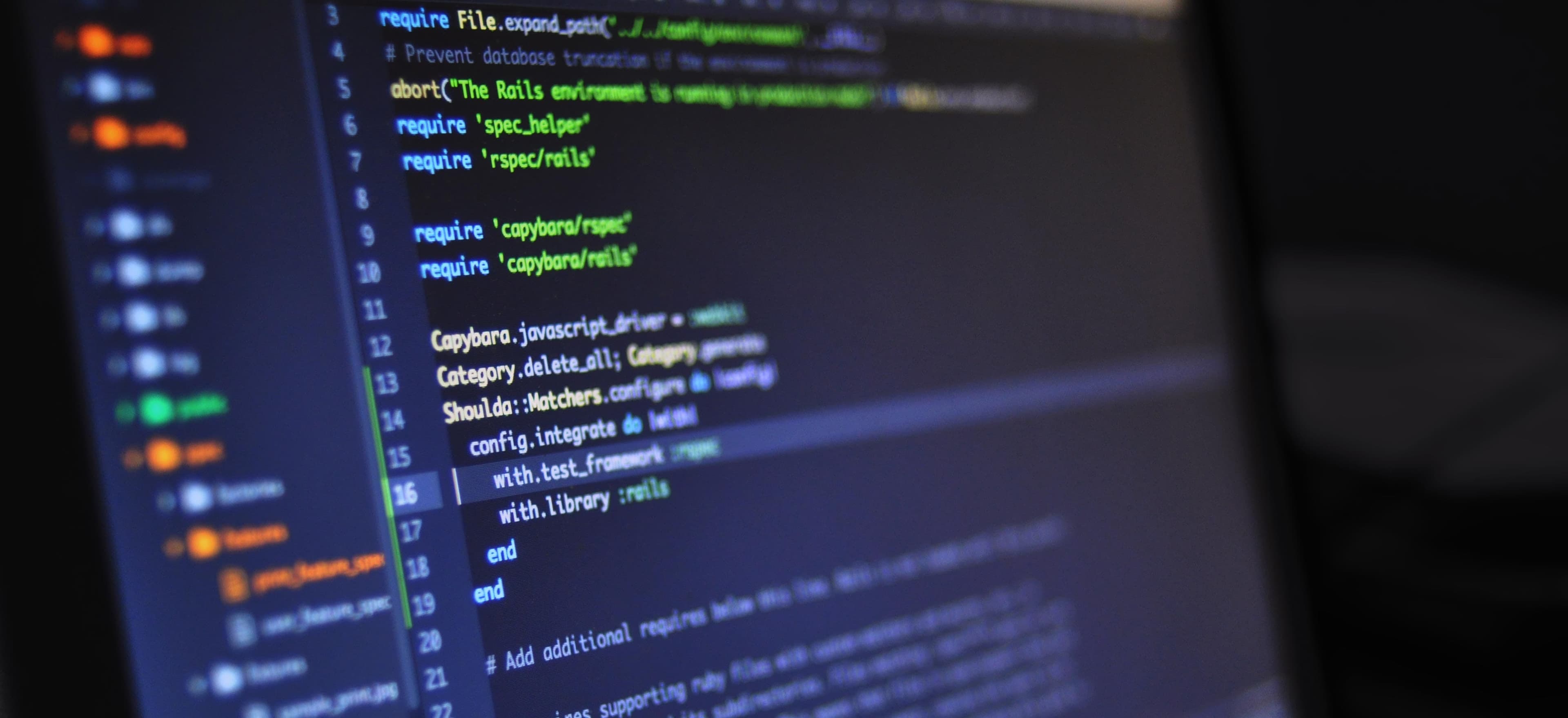
- Published on
Boosting Performance with Java Virtual Threads!
As the landscape of programming evolves, so too does the landscape of Java, a language that has solidified its place at the core of enterprise and cloud applications. With the Project Loom initiative, Java aims to revolutionize how developers handle concurrency by introducing a powerful feature: virtual threads. Virtual threads aim to solve long-standing issues with traditional concurrency models, boosting performance and greatly simplifying Java code at the same time.
In this post, we'll dive deep into the potential of virtual threads, explore how to use them, and learn why they could be transformative for your Java applications.
Traditional Threads vs Virtual Threads
Before we unwrap the potential of virtual threads, let's understand the shortcomings of traditional threads. Traditional threads, also known as platform threads, are mapped directly to operating system threads. This match-up comes with overhead in terms of memory and context-switching, making it less efficient to spawn a large number of concurrent threads. The result? Developers have had to use complex patterns like thread pools, which can lead to underutilization of system resources or complex synchronization nightmares.
Enter virtual threads, a key feature of Project Loom that's set to become part of the standard Java platform in future releases. Virtual threads are lightweight, managed by the Java Virtual Machine (JVM) rather than the operating system. This allows for the creation of a massive number of concurrent tasks without the overhead traditionally associated with threading.
Why Use Java Virtual Threads?
The primary advantage of virtual threads is their ability to scale with much less overhead. They make asynchronous programming easier and more efficient, particularly for I/O-intensive tasks that can benefit from massive concurrency. By reducing complexity and sidestepping the limitations of the number of available OS threads, virtual threads allow you to write straightforward, linear code that performs at the level of highly complex, asynchronous code.
How to Use Java Virtual Threads
Now that we've whetted your appetite with the why, let's dig into the how. Here are the steps to use virtual threads in Java.
Step 1: Ensure You Have the Correct Java Version
As of my knowledge cutoff in 2023, virtual threads are part of the early-access builds of Java. You'll need to download the latest compatible version to use virtual threads. They might not be available in standard Java distributions yet, so it's important to check the Project Loom Early-Access Builds.
Step 2: Create a Virtual Thread
Virtual threads are created through the Thread.ofVirtual()
factory method. Here’s an example of how to create and start a simple virtual thread:
import java.util.concurrent.Executors;
public class VirtualThreadExample {
public static void main(String[] args) {
Runnable task = () -> {
System.out.println("Hello, Virtual Thread!");
};
Thread.startVirtualThread(task);
}
}
This code snippet demonstrates the creation of a virtual thread that, once started, executes a simple task. Notice how the code is not fundamentally different from starting a traditional thread; the distinction lies under the hood, in how the JVM handles this thread.
Step 3: Understand How Virtual Threads Work
Virtual threads are managed by the JVM, which means that they are scheduled and switched by the Java runtime and not the underlying operating system. This is key to their lightweight nature. When a virtual thread is not actively using the CPU—say, it's waiting for an I/O operation—it can be parked by the JVM with minimal cost, allowing other virtual threads to use CPU resources.
Step 4: Integrate Virtual Threads into Existing Concurrency Models
Virtual threads can be used with existing concurrency constructs in Java. For example, you can use a ThreadFactory
to create virtual threads in a ThreadPoolExecutor
:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ThreadPoolWithVirtualThreads {
public static void main(String[] args) {
ExecutorService executor = Executors.newThreadPerTaskExecutor(Thread.ofVirtual().factory());
executor.submit(() -> {
System.out.println("Running in a virtual thread from a pool!");
});
executor.shutdown();
}
}
In this example, newThreadPerTaskExecutor
is a convenient method that creates an executor service compatible with virtual threads.
Best Practices with Java Virtual Threads
While virtual threads simplify concurrent programming in Java, there are best practices to follow to unlock their full potential:
Prefer Virtual Threads for I/O-Bound Tasks
Use virtual threads for tasks that are I/O-bound or spend a lot of time waiting for things to happen, such as database calls or reading files. Compute-intensive tasks that use the CPU consistently may not see as much benefit.
Monitor Thread Usage
With the capability to spawn millions of threads, there's a risk of creating too many, which can still overwhelm system resources albeit not as quickly as with platform threads. Monitoring and managing the creation of virtual threads is still important.
Take Advantage of Executors
Use the Executors
framework to manage virtual threads rather than creating and managing them manually. This provides a layer of abstraction and control over thread life cycles.
Real-World Applications and Performance Considerations
Adoption of virtual threads can lead to significant performance improvements in real-world applications, especially those relying on microservices, asynchronous APIs, or server-side request handling. Applications can handle far more concurrent tasks without the bottled neck of limited OS threads.
When it comes to performance, benchmark your application with and without virtual threads to understand their impact. Measure not only throughput but also latency, as virtual threads can show improved responsiveness under load due to more efficient task scheduling.
Conclusion
Virtual threads represent a significant advancement in Java's concurrency model, with the potential to simplify code and enhance performance. Although still in early access as of early 2023, they showcase Java's commitment to evolving in a way that meets the needs of modern application development.
To stay up to date on virtual threads and Project Loom, follow the OpenJDK Project Loom site and look out for future JDK releases that incorporate this exciting feature. As virtual threads transition from an early-access feature to a standard part of the Java platform, they are set to change the way we think about writing concurrent applications in Java.
Maximize concurrency, simplify your code, and let the JVM handle the complexity—virtual threads are the future of Java threading.