Lock Down Tomcat: SSL & Spring Security in 5 Steps
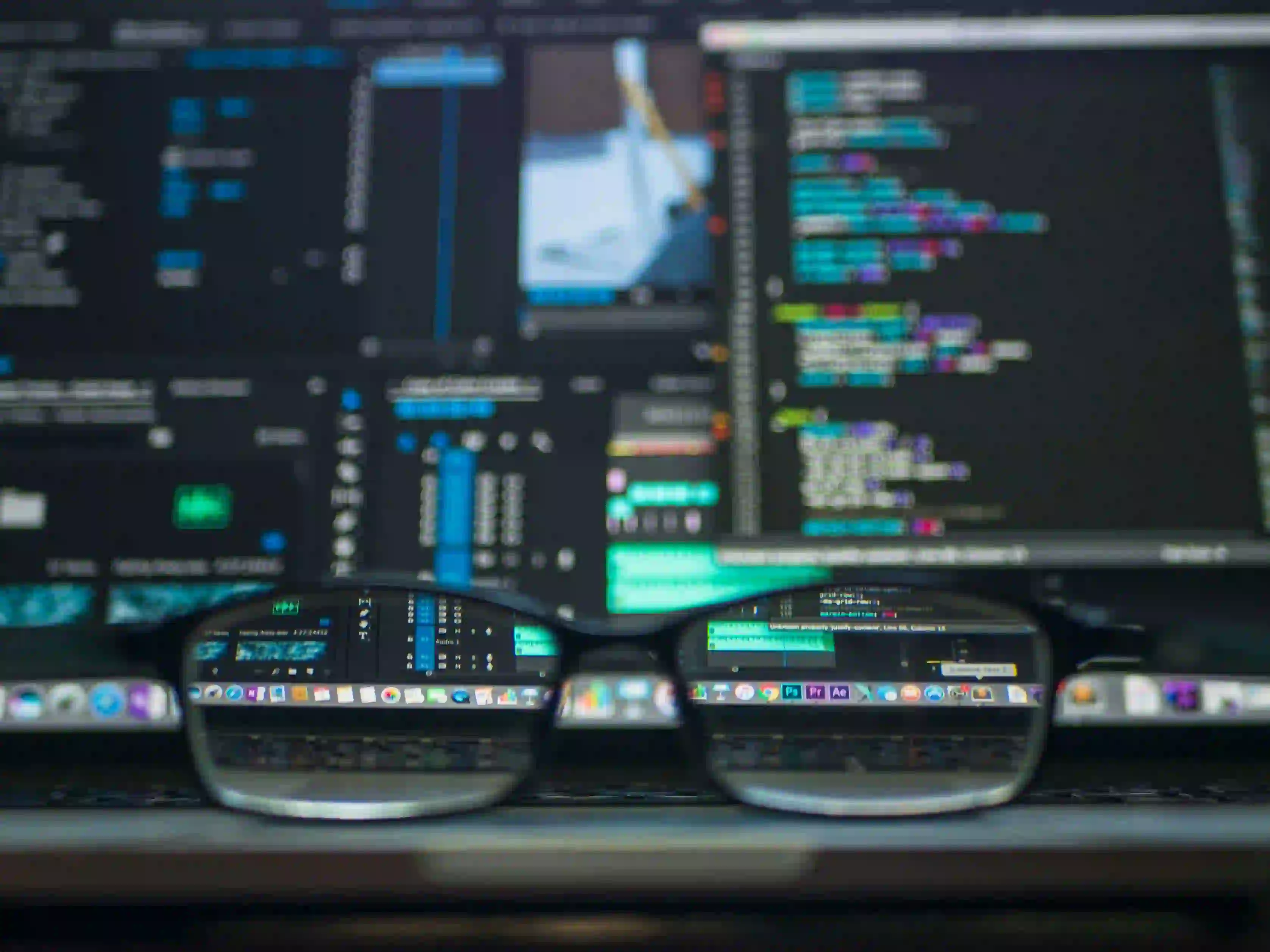
Lock Down Tomcat: SSL & Spring Security in 5 Steps
Securing web applications is paramount to ensuring the safety of your data and the trust of your users. When developing Java applications with Spring and deploying on Apache Tomcat, a widely-used web server and servlet container, implementing SSL (Secure Socket Layer) and integrating Spring Security are crucial steps toward a hardened security posture.
Let's embark on a journey to lock down your Tomcat server with SSL and reinforce it with Spring Security, all achieved in just five manageable steps.
Step 1: Acquire an SSL Certificate
Before delving into the configurations, you need to secure an SSL certificate. SSL certificates come in various forms, but they all serve the same purpose: to encrypt data transmitted between the client and the server.
You can generate a self-signed certificate for development purposes or acquire one from a trusted Certificate Authority (CA) for a production environment. Here’s how to generate a self-signed certificate:
keytool -genkeypair -alias tomcat -keyalg RSA -keysize 2048 -keystore keystore.jks -validity 365
This command creates a keystore file named keystore.jks
with a newly generated key pair. It's essential because Tomcat will utilize this keystore to facilitate SSL encryption.
Step 2: Configure SSL in Tomcat
Locate your Tomcat's server.xml
configuration file, usually found in the conf
directory of your Tomcat installation. Add an SSL connector configuration like so:
<Connector port="8443" protocol="org.apache.coyote.http11.Http11NioProtocol"
maxThreads="150" scheme="https" secure="true" SSLEnabled="true"
keystoreFile="conf/keystore.jks" keystorePass="password"
clientAuth="false" sslProtocol="TLS"/>
Ensure to replace keystoreFile
and keystorePass
values with the actual path to your keystore file and its password respectively.
After configuring Tomcat with SSL, the next logical step is to set up Spring Security to secure the endpoints within your application.
Step 3: Set Up Spring Security
Spring Security is an authentication and access control framework that is highly customizable. It provides robust security features that are essential for modern web applications.
To get started, add Spring Security to your project's Maven pom.xml
:
<dependencies>
<!-- Other dependencies -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
</dependencies>
With Spring Security added to your classpath, you can now define a security configuration class. Create a new Java class within your project that extends WebSecurityConfigurerAdapter
. Here is a basic example:
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@EnableWebSecurity
public class WebSecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/public/**").permitAll()
.anyRequest().authenticated()
.and()
.formLogin()
.and()
.httpBasic();
}
}
This configuration secures your application in a simple way, allowing public access to anything in the /public/
path and requiring authentication for everything else.
Step 4: Configure HTTPS in Spring Security
With both Tomcat and Spring Security in place, the next step is to configure your Spring application to enforce the use of HTTPS. In the same WebSecurityConfig
class, add a configuration to redirect all HTTP traffic to HTTPS:
@Override
protected void configure(HttpSecurity http) throws Exception {
http.requiresChannel()
.anyRequest()
.requiresSecure()
.and()
// ... other configurations
}
This configuration snippet ensures any request to the application is secured using HTTPS.
Step 5: Refine Your Security Policies
Now it's time to tweak and refine your security policies. Depending on your application's needs, Spring Security provides several mechanisms to further safeguard your application.
For example, if you want to configure session management and handle concurrent sessions, you could extend your configuration with:
@Override
protected void configure(HttpSecurity http) throws Exception {
http
// ... other configurations
.sessionManagement()
.sessionCreationPolicy(SessionCreationPolicy.IF_REQUIRED)
.maximumSessions(1)
.maxSessionsPreventsLogin(true);
}
With this snippet, the application allows only one session per user at a time and prevents new logins if a session already exists.
Remember that security is context-dependent; different applications may require different security configurations. Therefore, it’s important to understand the specific needs of your application when locking it down.
Testing Your Secured Application
After implementing the configurations, it’s crucial to test your application to ensure everything is working as expected. Here are some points to check:
- Your application should be accessible via
https://
and not viahttp://
. - Public endpoints should be accessible without authentication.
- Private endpoints should prompt for credentials and reject unauthorized access.
Conclusion
In this blog post, we covered how to secure a Tomcat server with SSL and integrate Spring Security into a Java application. By following these five steps, you're not only enhancing your application's security but also nurturing trust with your user base by protecting their data with industry-standard practices.
Remember that security is an ongoing process. Keeping your dependencies up to date, regularly reviewing your security configurations, and staying informed about the latest security vulnerabilities and patches are key to maintaining a strong security posture.
For more advanced configurations and understanding Spring Security in-depth, visit the official Spring Security documentation.
Your journey doesn't end here. Keep learning, keep coding, and stay secure!