Unmasking Facades: Solving Design Pattern Dilemmas
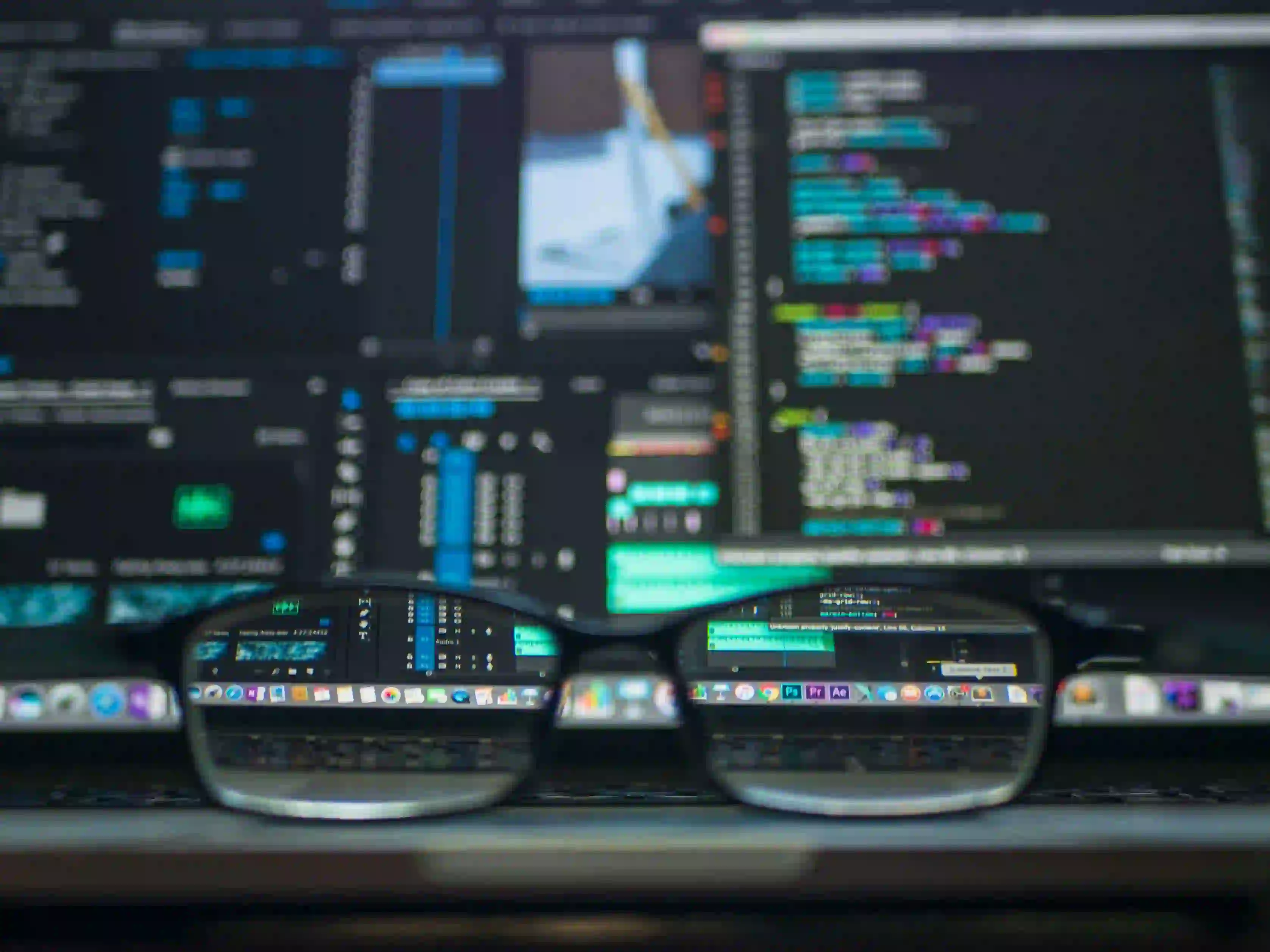
Unmasking Facades: Solving Design Pattern Dilemmas in Java
When delving into the world of software development, design patterns stand as a testament to years of accumulated wisdom. Among those, the Facade pattern is a star player, known for its adeptness at unscrambling complex systems. Today, we’ll uncover the essence of the Facade pattern in Java, why it deserves a place in your toolbox, and how it can mitigate design pattern dilemmas.
Simplifying Complexity: The Facade Pattern Explained
Imagine this: you've stumbled upon a codebase with a kaleidoscope of operations, a maze of classes and methods. Now, you're assigned the task of interacting with this labyrinth. Enter the Facade pattern. This design pattern provides a simplified interface to a complex subsystem, serving as a single point of entry which abstracts away the intricacies beneath.
Why Use a Facade?
- Simplicity: It offers a simple interface to a complex system.
- Reduced Dependency: Clients depend on a single facade instead of numerous classes.
- Readable Code: It promotes legible and maintainable code.
- Segregation: It decouples the subsystem from its clients and other subsystems.
Now that we’ve laid the groundwork, let’s cement our understanding with a real-world analogy. Consider an office building. You, as an employee, need various services like electricity, internet, and heating. Instead of understanding the inner workings of these utilities, you rely on the switches and controls within your reach—your facade to a world of complexity.
In Java, the Facade pattern matures into an invaluable tool, smoothing out creases in development and maintenance. Let’s dive deeper, shall we?
Crafting a Facade in Java: A Walkthrough
The Scenario
We’re tasked with creating an e-commerce application. It involves complexities like inventory management, payment processing, and shipping. Instead of interacting with these subsystems directly, we're better off simplifying it with a facade.
Step-by-step Implementation
Here’s a snippet to set the stage for our Java implementation:
// Subsystems that are complex and possibly intricate
class InventoryManager {
public void checkInventory(String itemId) {
// Logic to check inventory
}
}
class PaymentGateway {
public void processPayment(String cardDetails, double amount) {
// Logic to process payment
}
}
class ShippingService {
public void arrangeShipping(String itemId) {
// Logic to arrange shipping
}
}
These classes are an abstraction of services, which, in reality, are far more elaborate. Now, we'll encapsulate them with our facade.
public class OrderFacade {
private InventoryManager inventoryManager;
private PaymentGateway paymentGateway;
private ShippingService shippingService;
public OrderFacade() {
inventoryManager = new InventoryManager();
paymentGateway = new PaymentGateway();
shippingService = new ShippingService();
}
public void placeOrder(String itemId, String cardDetails, double amount) {
inventoryManager.checkInventory(itemId);
paymentGateway.processPayment(cardDetails, amount);
shippingService.arrangeShipping(itemId);
}
}
Notice how OrderFacade
provides placeOrder()
. It masks the trio of complexity - inventory checks, payment, and shipping - behind a singular method call. This simplicity is the soul of the facade.
Exemplifying the Facade's Utility
Here’s where it gets even more interesting. A client—say, our web controller—can now place an order with minimal fuss:
public class OrderController {
private OrderFacade orderFacade;
public OrderController() {
orderFacade = new OrderFacade();
}
public void submitOrder(String itemId, String cardDetails, double amount) {
orderFacade.placeOrder(itemId, cardDetails, amount);
System.out.println("Order has been placed successfully");
}
}
The elegance lies in the submitOrder
: it does not bother with what's behind the curtain. The facade has taken center stage, allowing for a performance free of the entanglements of backstage complexities.
Comprehending the Why Behind the Code
Why the abstraction? The facade’s role is to shield clients from the storm of complexity. It ensures that if there's a change in the subsystems, the facade absorbs the hit, leaving the client’s interaction undisturbed.
Why maintain separate subsystems? This promotes the Single Responsibility Principle. Each subsystem focuses on a specific task, making it more manageable and reducing the risk of a domino effect when changes occur.
Why instantiate subsystems inside the facade? It’s a design choice. You could opt for dependency injection to introduce greater flexibility and facilitate testing.
Flexing with Dependency Injection
For illustration purposes, let’s flex our code with dependency injection:
public class OrderFacade {
private InventoryManager inventoryManager;
private PaymentGateway paymentGateway;
private ShippingService shippingService;
public OrderFacade(InventoryManager inventory, PaymentGateway payment, ShippingService shipping) {
this.inventoryManager = inventory;
this.paymentGateway = payment;
this.shippingService = shipping;
}
// placeOrder() method remains the same
}
// Meanwhile in the OrderController...
public class OrderController {
private OrderFacade orderFacade;
public OrderController() {
orderFacade = new OrderFacade(
new InventoryManager(),
new PaymentGateway(),
new ShippingService()
);
}
// submitOrder() method remains the same
}
With dependency injection, you can slide in mocked versions of subsystems for testing or replace parts without tinkering with your facade.
Wrapping Up: The Takeaway of the Facade Pattern
To sum up, the Facade pattern is about:
- Easing the Pain of Complexity: It streamlines interactions with complicated systems.
- Preserving Flexibility: It’s amenable to change with lesser ripples throughout your codebase.
- Robust Design: It fortifies your architecture by clearly defining the points of interaction.
Questions Linger
Is Facade always the right tool? Like any design pattern, it has its place. When overused, it can turn into an anti-pattern—a monolithic block harboring too many dependencies. Therefore, wield it judiciously. For more intricate insights, explore sources like the GoF's "Design Patterns: Elements of Reusable Object-Oriented Software" or proliferative Java-focused blogs that delve into design pattern wisdom.
As a Java developer, the Facade pattern offers you more than a mere curtain to draw across complexity—it affords you clarity. May your code be expressive and your design patterns aptly applied.
Remember, every line of code is a decision. Make yours count.