Boost Performance with Java 8 Lazy Instantiation!
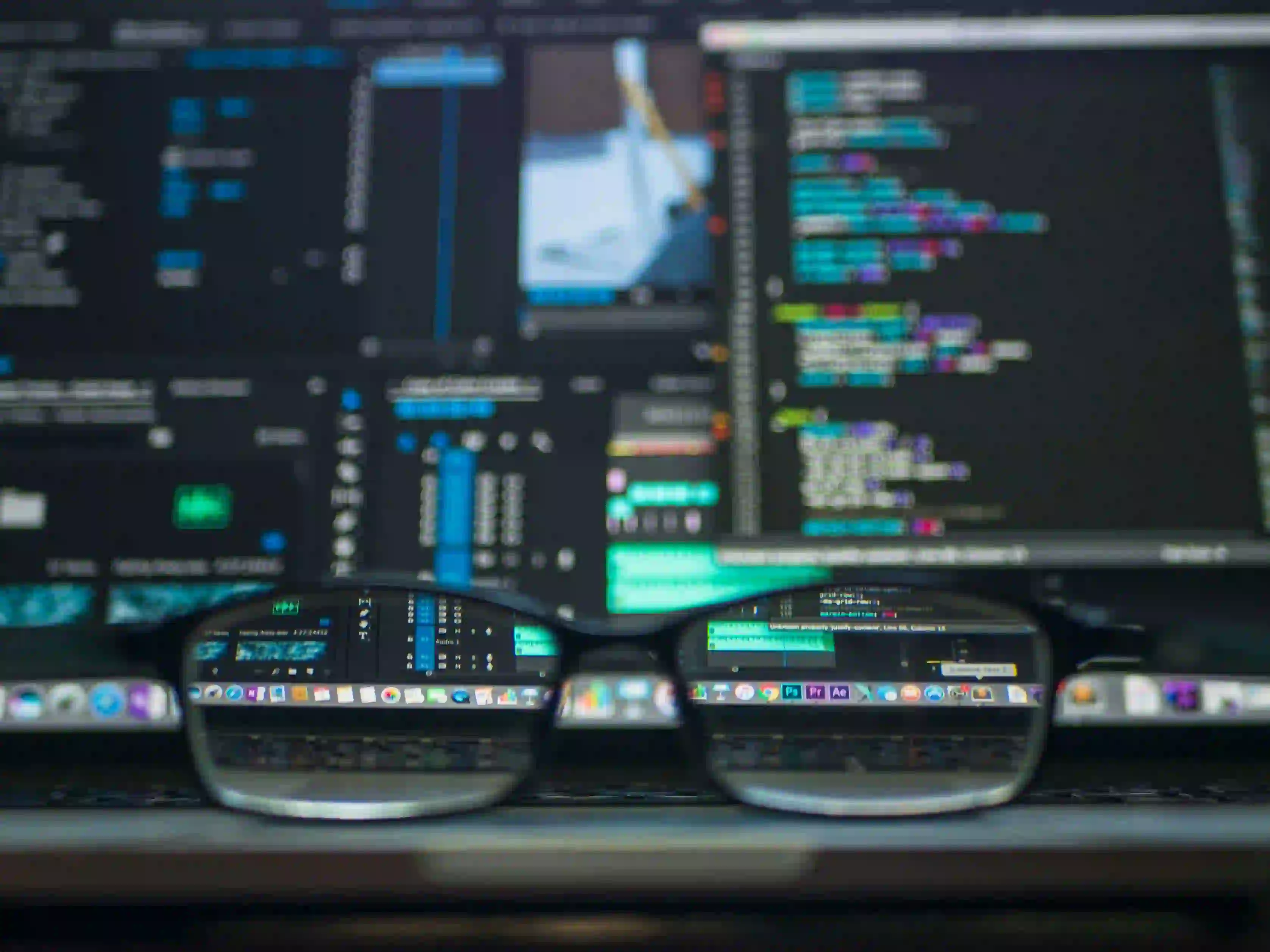
Boost Performance with Java 8 Lazy Instantiation!
Are you looking to supercharge your Java application's performance? One secret weapon you should definitely be utilizing is lazy instantiation — a powerful technique that can significantly enhance your program's efficiency, especially when initialized objects are heavy and not always necessary. In this Java-focused post, we'll delve into the nitty-gritty of lazy instantiation using Java 8, provide concrete examples, and discuss the "why" behind its impact. Prepare to give your Java skills a turbo boost!
Understanding Lazy Instantiation in Java
Lazy instantiation, also known as lazy initialization, is a design pattern that defers the creation of an object until the point at which it is needed. Why is this beneficial? It's simple — it reduces unnecessary computation, cuts down memory usage, and can speed up application startup time.
Imagine a scenario where your application has to deal with substantial configuration objects which are rarely used. Initializing these objects eagerly (immediately) at startup can be a waste of resources. Lazy instantiation serves as an effective solution to this issue.
Implementing Lazy Instantiation with Java 8
In Java 8, the addition of lambda expressions and the Supplier<T>
interface simplifies the implementation of lazy instantiation. Let's look at an example.
Example Without Lazy Instantiation
Before we dive into lazy instantiation, let's set our baseline with the standard (or eager) instantiation approach.
class HeavyResource {
public HeavyResource() {
// Imagine a time-consuming operation here
try {
Thread.sleep(5000); // Simulate a heavy resource initialization
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
public void doWork() {
System.out.println("Heavy resource doing work.");
}
}
class ResourceManager {
private HeavyResource heavyResource = new HeavyResource();
public void useResource() {
heavyResource.doWork();
}
}
public class Main {
public static void main(String[] args) {
ResourceManager resourceManager = new ResourceManager();
// heavyResource is already created, consuming resources
resourceManager.useResource();
}
}
In the above code snippet, the HeavyResource
object is created whenever ResourceManager
is instantiated, regardless of whether or not it's actually used. This may lead to a waste of resources and slower application startup.
Example With Lazy Instantiation
Now, see how laziness can make things more efficient:
import java.util.function.Supplier;
class HeavyResource {
// Resource implementation stays the same
}
class LazyResourceManager {
// The supplier takes a lambda expression to create the HeavyResource on demand
private Supplier<HeavyResource> heavyResourceSupplier = this::createHeavyResource;
private HeavyResource heavyResource;
private synchronized HeavyResource createHeavyResource() {
// Lazy instantiation using double-checked locking
if (heavyResource == null) {
heavyResource = heavyResourceSupplier.get();
}
return heavyResource;
}
public void useResource() {
getHeavyResource().doWork();
}
public HeavyResource getHeavyResource() {
// Call our lazy instantiation method
return createHeavyResource();
}
}
public class Main {
public static void main(String[] args) {
LazyResourceManager resourceManager = new LazyResourceManager();
// heavyResource is not created until it's actually needed
resourceManager.useResource();
}
}
In the revised LazyResourceManager
, the HeavyResource
is not created in the constructor. Instead, we have a Supplier<HeavyResource>
, which contains a lambda expression to create the resource. The createHeavyResource
method ensures that the HeavyResource
is created only once, when useResource
is invoked for the first time, and is then cached for subsequent uses.
Why Go Lazy?
Using lazy instantiation has several advantages:
- Reduced startup time: Objects are not created at application startup, leading to a faster launch.
- Decreased memory footprint: Memory is allocated only when needed, which is especially beneficial for applications with limited resources.
- Improved performance: By avoiding the creation of heavyweight objects that may never be used, overall application performance can improve.
When to Use Lazy Instantiation
While lazy instantiation sounds great, it's not a silver bullet for all performance issues. Here are some scenarios where it is particularly useful:
- When dealing with objects that are expensive to create or consume significant memory/resources.
- If there's a good chance that the object may not be used during the application's lifetime.
- For objects that are only used under certain conditions which may not always be true.
Caveats to Consider:
- Thread safety: If your lazy instantiation is to be used in a multi-threaded environment, ensure you handle it properly, like using double-checked locking as demonstrated.
- Complexity: Lazy instantiation adds complexity. Assess if the potential gains are worth the added complexity in your specific case.
- Over optimization: Not every object needs lazy instantiation. Avoid premature or unnecessary optimization.
Conclusion
Lazy instantiation is a powerful tool in any Java developer's kit for boosting performance and resource management. By thoughtfully implementing lazy instantiation in your Java 8 applications, you can optimize startup times, reduce memory footprint, and potentially improve application responsiveness.
Remember, the key is to evaluate your application's specific needs and apply lazy instantiation where it makes sense — and where it provides tangible benefits. Now that you're equipped with a deeper understanding and a practical example, you're ready to level up your Java applications with smart, performance-oriented design patterns.
And there you have it — a quick and effective lesson on lazy instantiation with Java 8. Always stay tuned for more insights and remember, happy coding!
For more advanced resources on Java optimization techniques and design patterns, check out the Oracle Java Documentation or dive into books such as "Effective Java" by Joshua Bloch, which provides an in-depth exploration of good practices in Java programming.