Boost Security: The Risk of Self-Signed Certificates Explained
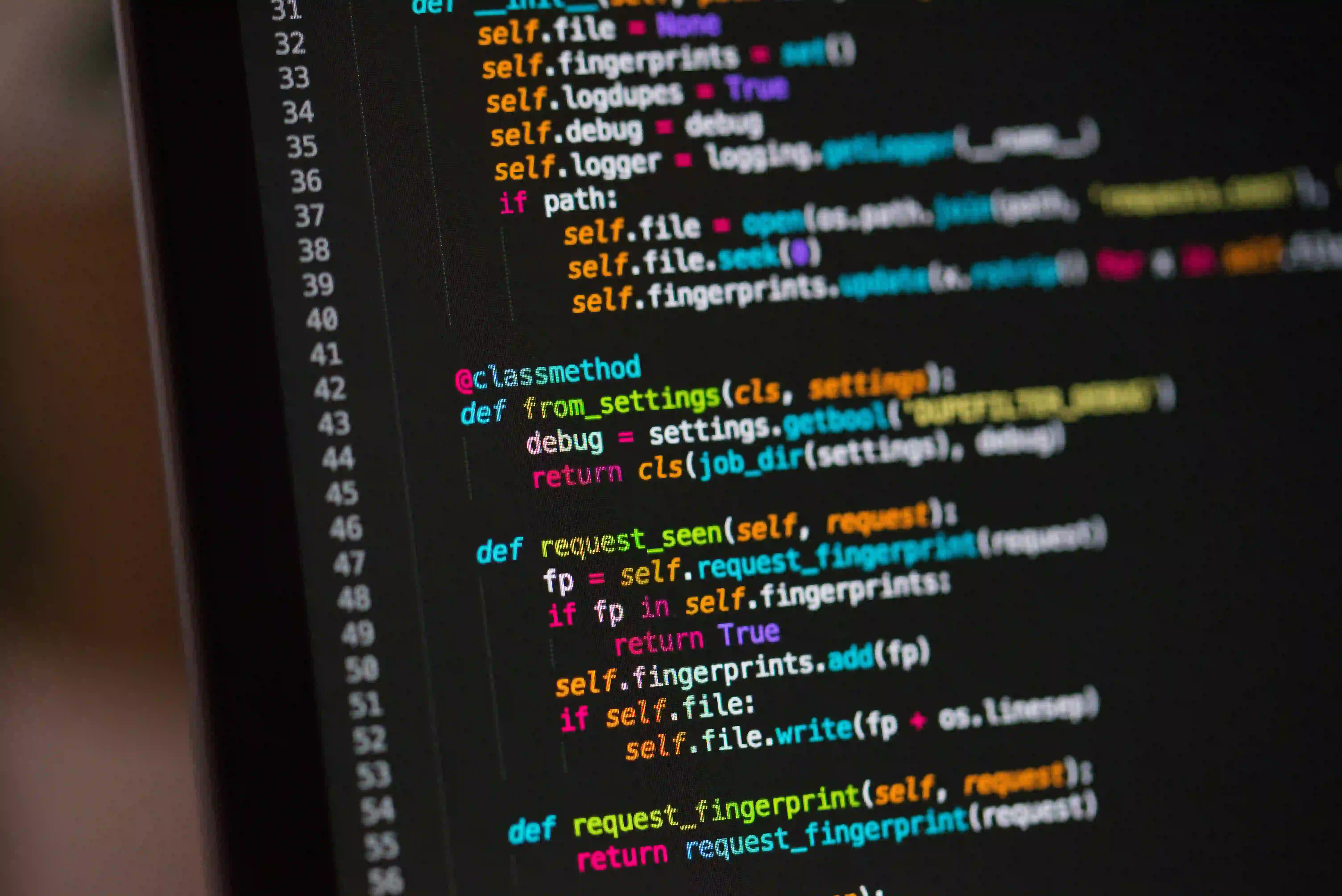
Boost Security: The Risk of Self-Signed Certificates Explained
In the world of web security, certificates play a pivotal role. They act as digital passports that provide authentication for the communication between a client and a server and ensure a trusted connection. While self-signed certificates can be tempting due to their cost-effectiveness and the ease with which they can be generated, they can pose significant risks that every Java developer should be aware of. In this post, we'll delve deep into the pitfalls of relying on self-signed certificates, and why opting for Certificates Authority (CA) issued certificates is the smarter choice.
Understanding Certificates in Java
Before we define the risks, let's lay the groundwork. What exactly are certificates? In Java, and web applications in general, certificates are utilized in SSL/TLS connections to encrypt and secure data exchange. Java provides a robust API for handling security, including classes like SSLContext
, KeyStore
, and TrustManager
, which assist developers in implementing SSL connections.
Certificates come into play in Java's security ecosystem through the KeyStore
. Let's take a look at a basic example of loading a certificate into a Java KeyStore:
KeyStore keyStore = KeyStore.getInstance(KeyStore.getDefaultType());
char[] password = "changeit".toCharArray();
try (InputStream is = new FileInputStream("mykeystore.jks")) {
keyStore.load(is, password);
}
Here, we're loading a KeyStore from a file named mykeystore.jks
using the default KeyStore type and a predetermined password. It's a straightforward process, but the key here is the source of your certificates.
Self-Signed Certificates: A Tempting Risk
Now, what's a self-signed certificate? It's a certificate that has been signed by the entity it represents, rather than a trusted Certificate Authority. This is akin to creating your own ID card and declaring it valid without any form of official vetting.
In Java, generating a self-signed certificate is relatively easy, as Java's keytool
can be employed to do so:
keytool -genkeypair -alias example -keyalg RSA -validity 365 -keystore mykeystore.jks
With just that command, you've created a self-signed certificate valid for 365 days. Quick and simple, right? But simplicity doesn't equate to security.
The Dangers of Self-Signed Certificates
While self-signed certificates offer a no-cost avenue for encrypting traffic, they carry undeniable risks:
-
Trust Issues: Because they aren't issued by a recognized CA, self-signed certificates inherently don't evoke trust from client systems or browsers. It leads to warning messages that can erode users' trust in your application.
-
Vulnerability to Attacks: They are more susceptible to man-in-the-middle attacks. Attackers can potentially intercept the communication by presenting another self-signed certificate, and without CA validation, it's harder to detect the breach.
-
Maintenance Overhead: Managing your certificates can become burdensome, especially as the number of self-signed certificates grows. You'll have to manually trust each certificate on every client that connects, which is logistically impractical on a larger scale.
-
Lack of Revocation: With self-signed certificates, there's no straightforward way to revoke them if they are compromised. CAs offer Certificate Revocation Lists (CRLs) and use the Online Certificate Status Protocol (OCSP), but self-signed certificates do not.
-
Non-compliance: If you're in an industry governed by specific compliance requirements, using self-signed certificates might simply not be an option. Regulations might mandate the use of CA-issued certificates for data protection.
Choosing Certificate Authority Issued Certificates
Given these risks, it becomes a no-brainer to invest in certificates from reliable CAs. Here's why CA-issued certificates are the way to go:
- Trust: They are inherently trusted by browsers and operating systems. The CA acts as a neutral third party verifying the identity of the certificate holder.
- Security: CAs have strict issuance requirements that mitigate the risk of impersonation and fraud.
- Maintenance: CA-issued certificates can be easier to manage thanks to the availability of tools and services offered by many CAs.
- Revocation: They can be revoked if compromised, and the revocation status can be easily communicated to clients.
Implementing CA Certificates in Java
Acquiring a certificate from a CA is a more complex process than generating a self-signed one. You'll need to:
-
Generate a Key Pair and Certificate Signing Request (CSR): This is performed using the
keytool
much like generating a self-signed certificate, but you'll export the CSR for the CA to sign it. -
Submit the CSR: Send the CSR to your chosen CA. Once they validate the information, they'll issue a certificate.
-
Import the CA Certificate: Once you have the CA's root certificate and your signed certificate, you'll need to import them into your Java KeyStore.
Here is how you would import a CA certificate using Java's keytool
:
keytool -import -alias rootCA -keystore mykeystore.jks -trustcacerts -file CARoot.crt
keytool -import -alias mydomain -keystore mykeystore.jks -file mydomain.crt
In the above, CARoot.crt
is the CA's root certificate, and mydomain.crt
is your signed certificate. The aliases rootCA
and mydomain
will be used to identify the certificates within the keystore.
Final Thoughts
Security is paramount when it comes to web applications and the handling of sensitive data. While self-signed certificates might seem like an attractive quick fix due to their easy setup and zero financial cost, the associated risks can far outweigh the benefits. Most web environments demand the trust that only CA-issued certificates can provide.
When developing Java applications, understanding the importance of a secure SSL/TLS implementation cannot be overstated. The peace of mind that comes with using CA-issued certificates, from both a security and a business standpoint, is invaluable. It's security you cannot afford to compromise.
Resources are widely available for Java developers looking to implement robust security in their applications, including official Java documentation on SSL and the use of keystores, as well as resources provided by CAs themselves on how to obtain and implement their certificates.
In conclusion, while self-signed certificates may work for quick tests or development environments, they should not be the standard for any production system. Elevating the security of your Java applications by using certificates from trusted CAs is not just a best practice—it's a necessity. Your users and clients deserve the highest level of security and trust that only CA-issued certificates can provide.