Mastering Bitwise Tricks: Boost Your Coding Efficiency!
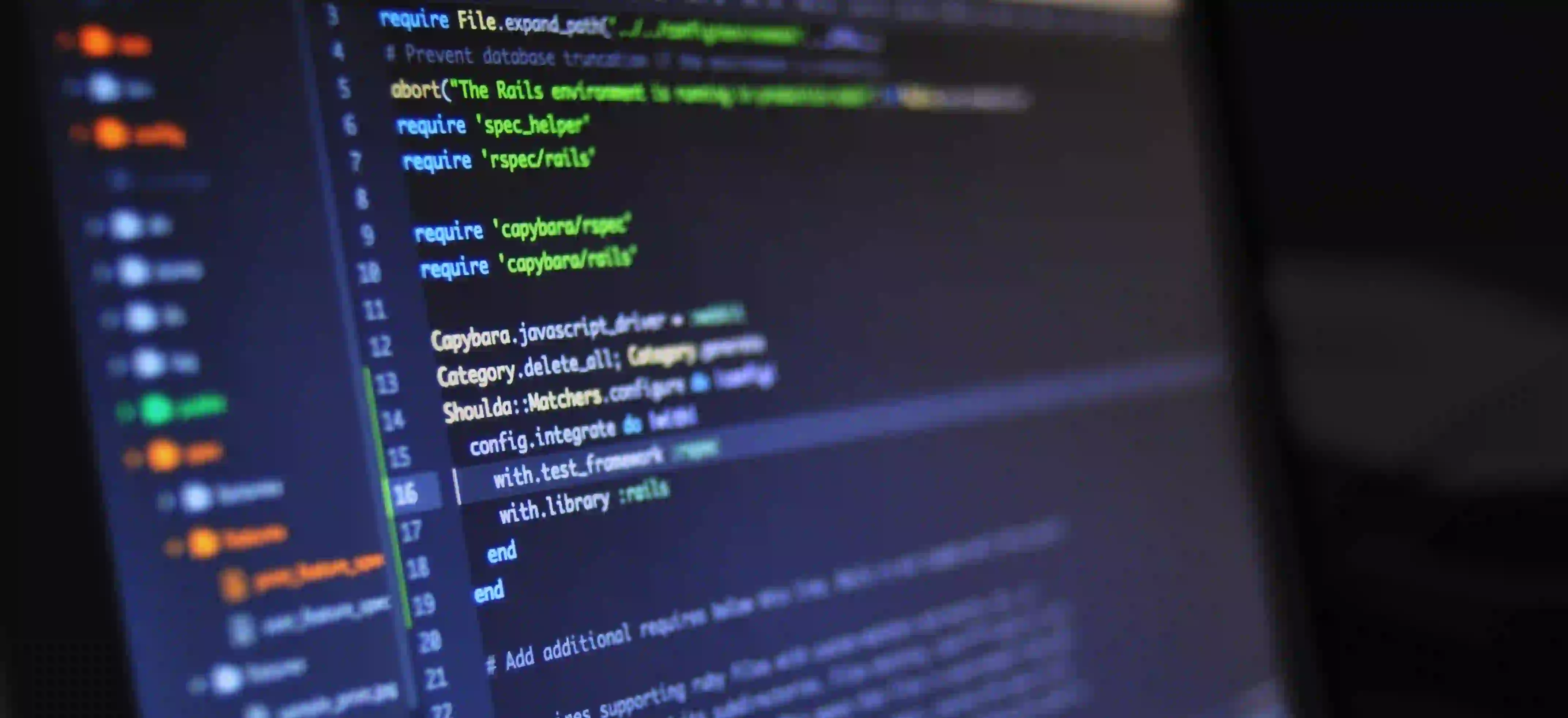
Mastering Bitwise Tricks: Boost Your Coding Efficiency!
Bitwise operations are a powerful tool in a programmer's toolbelt, offering a fast and efficient way to handle low-level data manipulation. If you develop in Java or are learning the language, understanding bitwise operators can give you a significant performance edge, especially in domains like cryptography, image processing, or when working on embedded systems. In this post, we'll explore some of the most useful bitwise tricks that can enhance your coding efficiency.
Unlock the Power of Bitwise Operations
At first glance, bitwise operators might seem esoteric, but they manipulate individual bits and perform boolean algebra on numbers, which are represented as bits in memory. Java provides several bitwise operators:
&
(AND)|
(OR)^
(XOR)~
(NOT)<<
(left shift)>>
(right shift)>>>
(unsigned right shift)
These operators work with int
, long
, short
, char
, and byte
data types.
The Why: Performance Benefits
Why should you bother with bitwise manipulation? The primary reason is performance. Bitwise operations are incredibly fast as they directly manipulate the binary representation of data, usually in a single CPU cycle. Also, they can reduce memory usage by packing multiple boolean flags into a single byte or integer.
Let's look at some practical examples to understand how to apply these operations in a real-world scenario.
Example 1: Setting and Clearing Bits
Imagine you're developing a game, and you need to track the state of various flags, such as whether a player has received a power-up or completed a challenge. You could use a separate boolean
for each flag or use a single int
with each bit representing a different flag.
Code Snippet
public class GameState {
private static final int POWER_UP_RECEIVED = 1; // 0001
private static final int CHALLENGE_COMPLETED = 2; // 0010
// Other game states can be added here
private int state = 0;
public void receivePowerUp() {
state |= POWER_UP_RECEIVED;
}
public boolean hasPowerUp() {
return (state & POWER_UP_RECEIVED) != 0;
}
public void completeChallenge() {
state |= CHALLENGE_COMPLETED;
}
public boolean isChallengeCompleted() {
return (state & CHALLENGE_COMPLETED) != 0;
}
// Add methods for other states as needed
}
In this example, we're using the |
operator to set a bit (turn it to 1
) and the &
operator to check whether a bit is set.
Why This Code?
Using bitwise operations for state management allows us to store multiple boolean states within a single int
. It's much more efficient than having an array of boolean
values for each flag.
Example 2: Bitwise Complement and Negative Numbers
The bitwise NOT operator ~
flips every bit in a number. This can be used to generate the complement of a number in binary format. In Java, integers are stored using two's complement, which can sometimes lead to unexpected results when using ~
.
Code Snippet
public class BitwiseComplement {
public static void main(String[] args) {
int number = 5; // Binary: 0000 0101
int complement = ~number; // Binary: 1111 1010
System.out.println("Number: " + number); // Output: 5
System.out.println("Complement: " + complement); // Output: -6
}
}
Why This Code?
This example shows how the complement of 5
(0000 0101
) becomes -6
(1111 1010
) after applying ~
. Understanding the representation of negative numbers in binary is crucial when working with bitwise NOT, particularly when performing operations related to two's complement arithmetic.
Example 3: Efficiently Checking if a Number is a Power of Two
Determining if a number is a power of two is a common task, and there's a clever bitwise trick to do that in an efficient manner.
Code Snippet
public class PowerOfTwo {
public static boolean isPowerOfTwo(int number) {
return (number > 0) && ((number & (number - 1)) == 0);
}
public static void main(String[] args) {
System.out.println(isPowerOfTwo(16)); // true
System.out.println(isPowerOfTwo(18)); // false
}
}
Why This Code?
This trick works because a power of two in binary is a 1
followed by all 0
s (e.g., 16
is 10000
in binary), and its predecessor is all 1
s in those same positions (e.g., 15
is 01111
). The AND operation in this case will always result in 0
for powers of two, which is what we're checking.
Example 4: Swapping Two Numbers Without a Temporary Variable
Another neat trick with bitwise operations is the ability to swap two numbers without using a temporary variable.
Code Snippet
public class NumberSwapper {
public static void swap(int a, int b) {
a ^= b;
b ^= a;
a ^= b;
System.out.printf("a: %d, b: %d%n", a, b);
}
public static void main(String[] args) {
swap(10, 20); // Outputs "a: 20, b: 10"
}
}
Why This Code?
The XOR operator ^
here is the star of the show. The property that a ^ a
equals 0
and a ^ 0
equals a
is what makes this trick work. Swapping numbers without a temporary variable reduces memory usage, which can be a micro-optimization in scenarios where memory is limited.
Example 5: Counting the Number of Set Bits
Counting the number of 1
s in the binary representation of a number is a common operation, sometimes referred to as computing the Hamming weight.
Code Snippet
public class BitCounter {
public static int countSetBits(int number) {
int count = 0;
while (number > 0) {
count += number & 1;
number >>>= 1;
}
return count;
}
public static void main(String[] args) {
int number = 29; // Binary: 11101
System.out.println("Number of set bits: " + countSetBits(number)); // 4
}
}
Why This Code?
Iterating over each bit of the number and checking whether it's set (1
) by ANDing with 1
gives us the count of set bits. The unsigned right shift operator >>>
is used to make sure that the sign bit is filled with 0
when shifting, which is important for negative numbers.
Conclusion
While bitwise operations can seem like a trick of the old guard, they're still relevant and incredibly useful in modern programming, especially when every bit of performance counts. Java's syntax makes it easy to apply these operations, turning complex tasks into simple lines of code with no need for loops or conditionals.
Understanding bitwise operations is not just about writing efficient code; it's also about reasoning more effectively about data and algorithms. As you continue on your journey to becoming a Java expert, mastering these tricks will help you stand out, particularly when dealing with systems-level or performance-critical applications.
Remember, as with all programming skills, practice makes perfect. Incorporate these techniques into your daily coding, and you'll soon be thinking in bits and bytes with ease. For further reading and in-depth explanations of the bitwise and other operators, the official Java documentation is an invaluable resource. Happy coding!