Crafting Immutable Value Objects: A How-To Guide
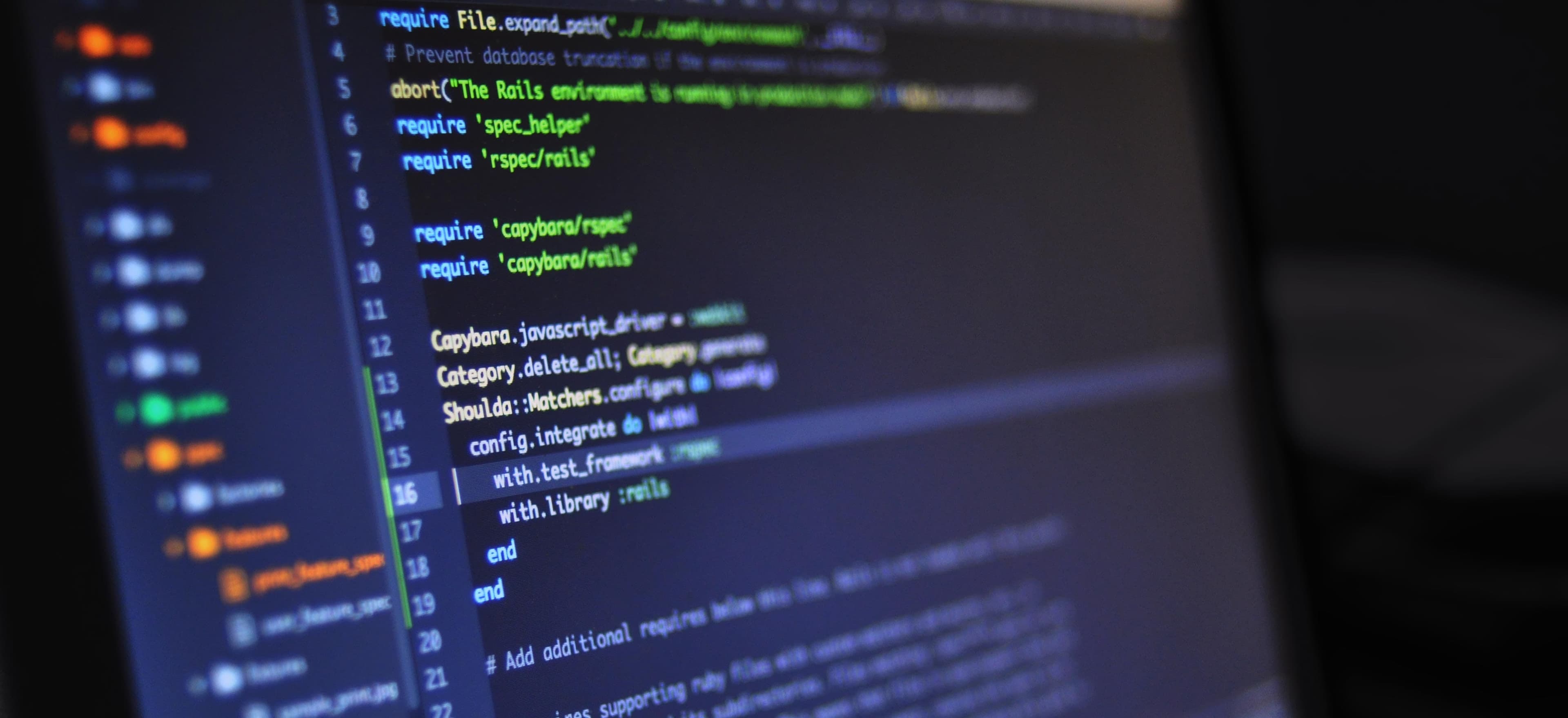
- Published on
Crafting Immutable Value Objects: A How-To Guide in Java
Java's object-oriented nature makes it an ideal candidate for building robust applications, but it's easy to overlook the subtleties of class design, particularly when it comes to immutability. Immutable objects are those whose state cannot be changed after they are created. They offer several advantages: they are simple to understand and debug, inherently thread-safe, and less prone to errors. In this guide, we'll explore how to effectively create immutable value objects in Java, guaranteeing reliability and performance in your applications.
Understanding Immutable Objects
Before we delve into the code, it's crucial to understand why immutability is so prized. Immutable objects:
- Simplify concurrency: Since their state doesn't change, there's no need for synchronization.
- Increase security: They cannot be tampered with once created, thus preserving their integrity.
- Are cache-friendly: Their consistent state makes them safe for reuse.
- Promote functional programming: They naturally support operations without side effects.
Creating Immutable Objects in Java
The process of creating immutable objects involves a few key steps. Let's go through them one by one.
Step 1: Declaring Final Fields
All fields within an immutable object must be final. This ensures that their values are set once upon object construction and never again.
public class Point {
private final int x;
private final int y;
// Constructor
public Point(int x, int y) {
this.x = x;
this.y = y;
}
}
Step 2: No Setters
In an immutable class, you should omit setter methods. Without setters, the state of the object can't be changed after its creation.
public class Point {
// Fields and constructor as above...
// Notice the lack of setter methods.
}
Step 3: Providing Access through Getters
While we can't allow object state to change, we still need to access its data. Therefore, provide getters for fields that need to be visible outside the class.
public class Point {
// Fields and constructor as above...
public int getX() {
return x;
}
public int getY() {
return y;
}
}
Step 4: Ensuring Class Cannot Be Extended
To truly enforce immutability, make sure the class can't be extended, as a subclass could potentially add mutability. To prevent this, declare the class as final.
public final class Point {
// Fields, constructor, and getters as above...
}
Step 5: Making Copies of Mutable Fields
If your class contains fields that are mutable objects, make sure to return copies of these objects from the getters or create immutable wrapper objects.
import java.util.Date;
public final class ImmutableDate {
private final Date date;
public ImmutableDate(Date date) {
this.date = new Date(date.getTime()); // Safely create a copy
}
public Date getDate() {
return new Date(date.getTime()); // Return a copy to preserve immutability
}
}
Step 6: Preventing Leaks Through Method References
Ensure that references to internal mutable objects aren't leaked through methods or constructors.
public final class ImmutableCollection {
private final List<String> items;
public ImmutableCollection(List<String> items) {
this.items = new ArrayList<>(items); // Create a copy to prevent external mutations
}
public List<String> getItems() {
return Collections.unmodifiableList(items); // Return an unmodifiable view
}
}
Step 7: Avoiding Mutable Static Variables
Lastly, ensure that any static variables in your class are also immutable, as these can also be a source of hidden mutable state.
public final class Constants {
public static final String APPLICATION_NAME = "MyImmutableApp";
// No mutable static fields!
}
A Full Example
Let's put everything together with a comprehensive example—a UserInfo class that holds user data.
import java.util.HashMap;
import java.util.Map;
public final class UserInfo {
private final String name;
private final int age;
private final Map<String, String> preferences;
public UserInfo(String name, int age, Map<String, String> preferences) {
this.name = name;
this.age = age;
this.preferences = new HashMap<>(preferences); // Copy for immutability
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public Map<String, String> getPreferences() {
return Collections.unmodifiableMap(preferences); // Unmodifiable view
}
}
In this example, the UserInfo
class's state is designed to be immutable. Note how we create deep copies of the Map
instance both in the constructor and in the getter. This practice ensures that changes to the Map
outside of the UserInfo
class do not affect internal state.
Concluding Thoughts
Designing immutable objects in Java hinges on discipline and a handful of programming patterns. By following the principles outlined here, you can create solid value objects that provide safety, predictability, and efficiency.
Remember that immutability isn't always the answer. There are cases where mutable state is required or performance concerns dictate a different approach. However, when immutability fits the bill, it can simplify your design and make your code cleaner and more maintainable.
Begin embracing the practice of creating immutable objects in your next Java project, and watch as your code stability improves alongside multi-threaded performance. For a deeper dive into immutability and its implications in Java, check out Oracle's documentation on the subject and Effective Java by Joshua Bloch, which provides extensive best practices for Java developers.
Happy coding, and may your objects always remain in a state of unchangeable bliss!