Spring Security: Solving Common Login Issues
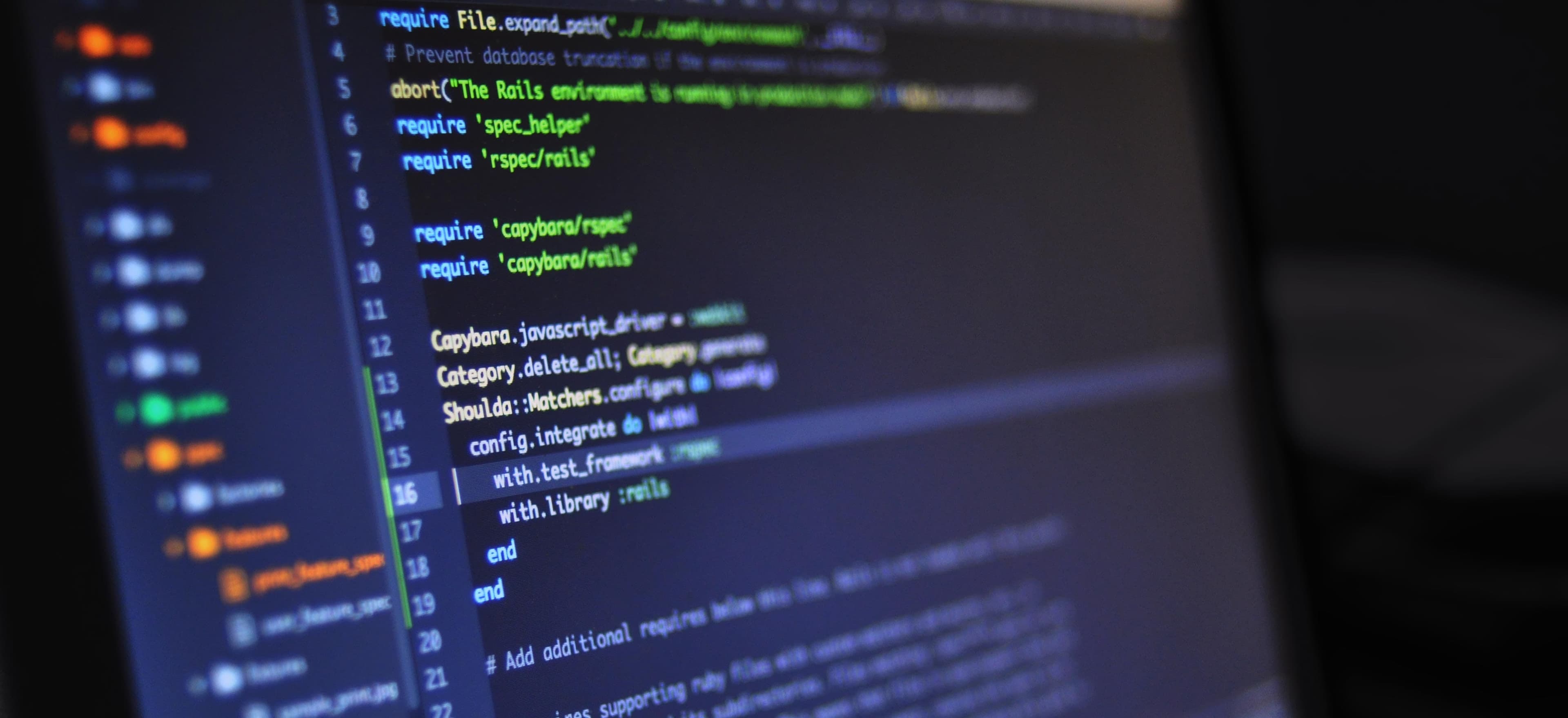
- Published on
Spring Security: Solving Common Login Issues
When it comes to building secure applications in Java, Spring Security is one of the go-to frameworks. Offering both robust authentication and authorization, it's a preferred choice for many developers. However, as with any complex framework, integrating Spring Security can sometimes introduce frustrating login issues that are not immediately apparent. In this blog post, we'll tackle common login challenges encountered in Spring Security and provide clear, actionable solutions.
Before we dive into specifics, it's important to ensure you have a basic understanding of how Spring Security works. You can find an official introduction to Spring Security that provides an excellent starting point.
Issue #1: Incorrect Credentials Handling
One of the most common issues is incorrect handling of user credentials. This can lead to users being unable to log in even with the correct username and password.
Cause:
This often stems from misconfigured UserDetailsService
or incorrect setup in the UserDetails
implementation.
Solution:
Ensure your UserDetailsService
correctly fetches user information, and the UserDetails
implementation accurately represents the security properties of a user (password, username, authorities, account status flags).
Example Code:
@Service
public class CustomUserDetailsService implements UserDetailsService {
@Autowired
private UserRepository userRepository;
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
User user = userRepository.findByUsername(username)
.orElseThrow(() -> new UsernameNotFoundException("User not found with username: " + username));
return new org.springframework.security.core.userdetails.User(user.getUsername(),
user.getPassword(), getAuthorities(user));
}
private Collection<? extends GrantedAuthority> getAuthorities(User user) {
return user.getRoles().stream()
.map(role -> new SimpleGrantedAuthority(role.getName()))
.collect(Collectors.toList());
}
}
Why this code works:
This CustomUserDetailsService
ensures that for each username provided, we fetch the corresponding User
from our UserRepository
and convert it into a UserDetails
object that Spring Security can use for authentication.
Issue #2: Forgotten PasswordEncoder
Many login issues arise from not using a password encoder or not matching the encoder used during the registration process with login.
Cause:
Spring Security requires you to use a password encoder due to security reasons. If you store plain-text passwords or your PasswordEncoder
doesn't match the one used during registration, authentication will fail.
Solution:
Use a PasswordEncoder
bean and ensure it's the same for both registration and login phases.
Example Code:
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
Why this code works:
Using BCryptPasswordEncoder
is a common practice, as it's a strong, adaptive, and widely supported hashing algorithm. By applying this encoder uniformly throughout your security configuration, you guarantee that passwords are being properly encoded and validated.
Issue #3: Session Misconfiguration
Improperly managed sessions can cause users to experience immediate logouts after login or session mix-ups.
Cause:
This may occur due to incorrect session management settings, such as creating a new session when it's unnecessary or not properly invalidating sessions on logout.
Solution:
Configure your session settings in Spring Security correctly and ensure that old session information is cleared out on logout to prevent stale sessions from causing issues.
Example Code:
@Override
protected void configure(HttpSecurity http) throws Exception {
http
// ...
.sessionManagement()
.sessionCreationPolicy(SessionCreationPolicy.IF_REQUIRED)
.invalidSessionUrl("/login?invalid-session=true")
.and()
.logout()
.invalidateHttpSession(true)
.deleteCookies("JSESSIONID") // if using cookies
.logoutSuccessUrl("/login?logout=true");
}
Why this code works:
This security configuration sets up session management to create sessions as needed. It invalidates sessions on logout, preventing unwanted access via old session IDs. The .invalidSessionUrl
specifies where to redirect users with invalid sessions (commonly due to timeout), thus enhancing the user experience by providing clear feedback.
Issue #4: Inadequate Exception Handling
Many login issues may go unresolved due to inadequate exception handling. Users may encounter a generic error without understanding the cause.
Cause:
Spring Security may throw various exceptions during the authentication process. Not handling these exceptions properly may leave users in the dark.
Solution:
Implement an AuthenticationFailureHandler
to provide specific error messages or redirections based on the exception raised.
Example Code:
@Component
public class CustomAuthenticationFailureHandler implements AuthenticationFailureHandler {
@Override
public void onAuthenticationFailure(HttpServletRequest request, HttpServletResponse response,
AuthenticationException exception) throws IOException, ServletException {
if (exception instanceof BadCredentialsException) {
response.sendRedirect("/login?error=bad-credentials");
} else if (exception instanceof DisabledException) {
response.sendRedirect("/login?error=disabled-account");
} else {
response.sendRedirect("/login?error=generic");
}
}
}
Why this code works:
By implementing a custom AuthenticationFailureHandler
, we can catch specific exceptions (BadCredentialsException
, DisabledException
, etc.) and redirect the user to an appropriate error page or flag, enhancing transparency.
Issue #5: Misconfigured Security Filters
Sometimes the order or existence of security filters in the filter chain can cause unexpected behavior.
Cause:
Spring Security is highly dependent on the order in which filters are applied. If the filter chain is misconfigured, users may face redirect loops or incorrect authentication.
Solution:
Dive into the Spring Security documentation and ensure the filter chain is configured according to your application's needs.
Example Code:
@Override
protected void configure(HttpSecurity http) throws Exception {
http
// ...
.addFilterBefore(new CustomFilter(), UsernamePasswordAuthenticationFilter.class);
// ...
}
Why this code works:
By using .addFilterBefore
, we insert our CustomFilter
before the UsernamePasswordAuthenticationFilter
. This placement is critical because it allows our custom filter to process requests before Spring Security performs its standard username and password authentication, giving us control to handle specific cases or pre-filter requests.
Conclusion
Integrating Spring Security is a quintessential task for creating secure Java applications, but it's not without its challenges. Understanding common issues like incorrect credentials handling, forgetting the PasswordEncoder
, session mismanagement, inadequate exception handling, and misconfigured security filters will arm you with the knowledge to troubleshoot effectively.
If you encounter other issues, remember to refer to the Spring Security documentation or community resources such as Stack Overflow. With vigilant configuration and careful coding, your Spring Security integration can be seamless and robust.
Remember, security is an ongoing process. Keep your dependencies up-to-date, sunset legacy algorithms in favor of modern ones, and always heed the advice of security best practices. Your users' security depends on it.