Flawless JEE Web Projects: Mastering Structure Setup
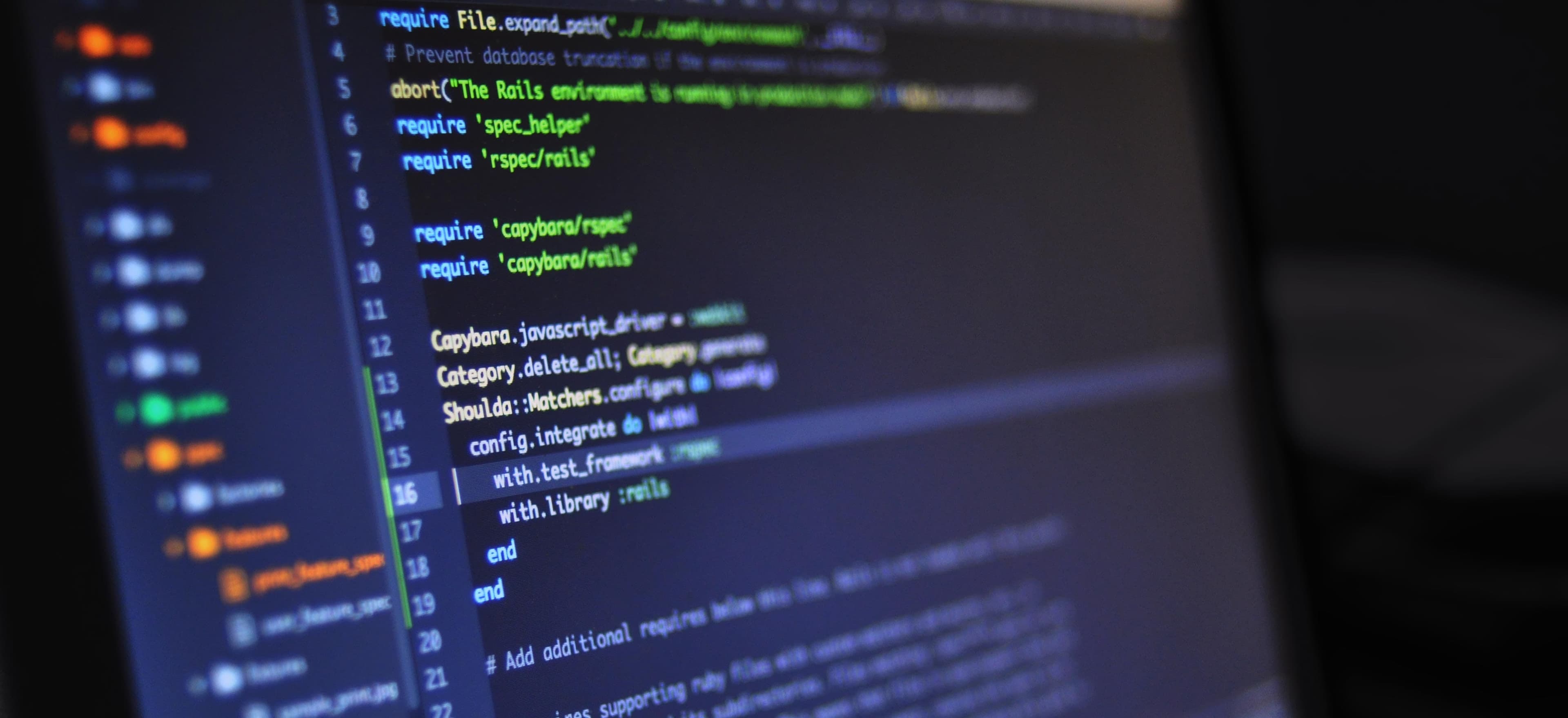
- Published on
Flawless JEE Web Projects: Mastering Structure Setup
Creating an engaging and well-structured Java Enterprise Edition (JEE) web project can be a formidable task for even the most seasoned developers. However, a strong foundation is essential to ensure scaling, maintainability, and performance. This blog post guides you through setting up a JEE web project that is primed for search engine optimization (SEO) and provides an excellent starting point for your development process.
Understanding Java Enterprise Edition (JEE)
Before diving into project setup, it's important to understand what JEE entails. JEE is a standard used for enterprise-grade applications and large-scale projects. It extends Java SE (Standard Edition) with specifications for enterprise features such as distributed computing and web services.
Initial Project Setup
The initial structure of your JEE project is critical. Here's how you should start:
-
Choose a Project Structure: Commonly, Maven or Gradle-based project structures are used. These tools help automate the build process and manage dependencies.
-
Decide on a Web Framework: Options include JSF, Spring MVC, or Struts. Your choice depends on your project requirements and personal preference.
-
Prepare the Development Environment: Ensure that your IDE is set up with all necessary plugins and configurations for JEE development. Eclipse and IntelliJ IDEA are popular choices offering extensive support for JEE.
-
Set Up Version Control: Tools like Git are essential for team collaboration and version tracking. Hosting platforms like GitHub or Bitbucket provide remote repositories and additional tools for pull requests and code reviews.
Maven Project Structure
Maven is widely used and favored for its convention-over-configuration approach.
my-app/
|-- pom.xml
|-- src/
|-- main/
| |-- java/
| | `-- mypackage/
| |-- resources/
| `-- webapp/
| |-- WEB-INF/
| `-- views/
`-- test/
|-- java/
| `-- mypackage/
`-- resources/
The pom.xml
is your project's lifeline. It defines dependencies, plugins, and build profiles:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-app</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>war</packaging>
<!-- Define your dependencies here -->
<dependencies>
<!-- JEE APIs -->
<!-- ... -->
</dependencies>
<!-- Build and plugin configurations -->
<build>
<finalName>MyApp</finalName>
<plugins>
<!-- Compiler plugin, resources plugin, etc. -->
<!-- ... -->
</plugins>
</build>
</project>
Why Maven?
Maven's structure and lifecycle are designed for consistency. It's easier to understand a Maven project if you're familiar with the standard Maven layout.
JEE Framework Choices
Spring MVC
Spring MVC is a part of the larger Spring Framework and is known for its simplicity and flexibility in designing web applications.
@Controller
@RequestMapping("/hello")
public class HelloWorldController {
@GetMapping
public String welcome(Model model) {
model.addAttribute("message", "Hello, World!");
return "hello";
}
}
In this example, HelloWorldController
handles HTTP GET requests to /hello
path and returns a view named hello
.
Why Spring MVC?
Spring MVC provides a robust framework with powerful features like Dependency Injection and aspect-oriented programming. This makes it ideal for projects with complex business requirements.
Optimize Your JEE Project for SEO
While SEO is mostly about content, the technology stack and server responses play a significant role as well.
- Friendly URLs: Use URL rewriting techniques to create human-readable URLs.
- Load Time: Optimize load time with efficient code, database queries, and using CDN for static resources.
- HTTP Status Codes: Properly handle error pages and resources not found with correct HTTP status codes such as 404 or 301 redirects.
Deploying Your Application
The culmination of your JEE project setup is deploying it. If you're using Maven, the maven-war-plugin
can be configured to output a .war
file, which can be deployed to a JEE container like Apache Tomcat or GlassFish.
<plugin>
<artifactId>maven-war-plugin</artifactId>
<version>3.2.3</version>
<configuration>
<!-- Additional configuration here -->
</configuration>
</plugin>
Why Use the Maven WAR Plugin?
The maven-war-plugin
simplifies the WAR file creation and ensures that your project follows the Java EE web application directory structure. It makes deployment consistent and repeatable.
Conclusion
Correctly setting up your JEE web project will pave the way for a smoother development experience and can have a significant impact on your application's success. By following best practices with project setup, structure, and choosing the right frameworks and tools, you’ll lay a solid ground for both project sustainability and SEO.
The intricacies of JEE programming demand attention to detail. It's these details, like project structure and best coding practices, that set you on the path to creating a flawless web application. Remember, SEO starts at the code level, and an optimized server response and a well-configured environment are the backbone of a great user and search engine experience.
Embrace these guidelines, and your journey through Java Enterprise development will be a smooth sail toward creating performant, scalable, and SEO-ready web applications.