Smooth Streaming: Fixing Android HTTP Live Camera Lags
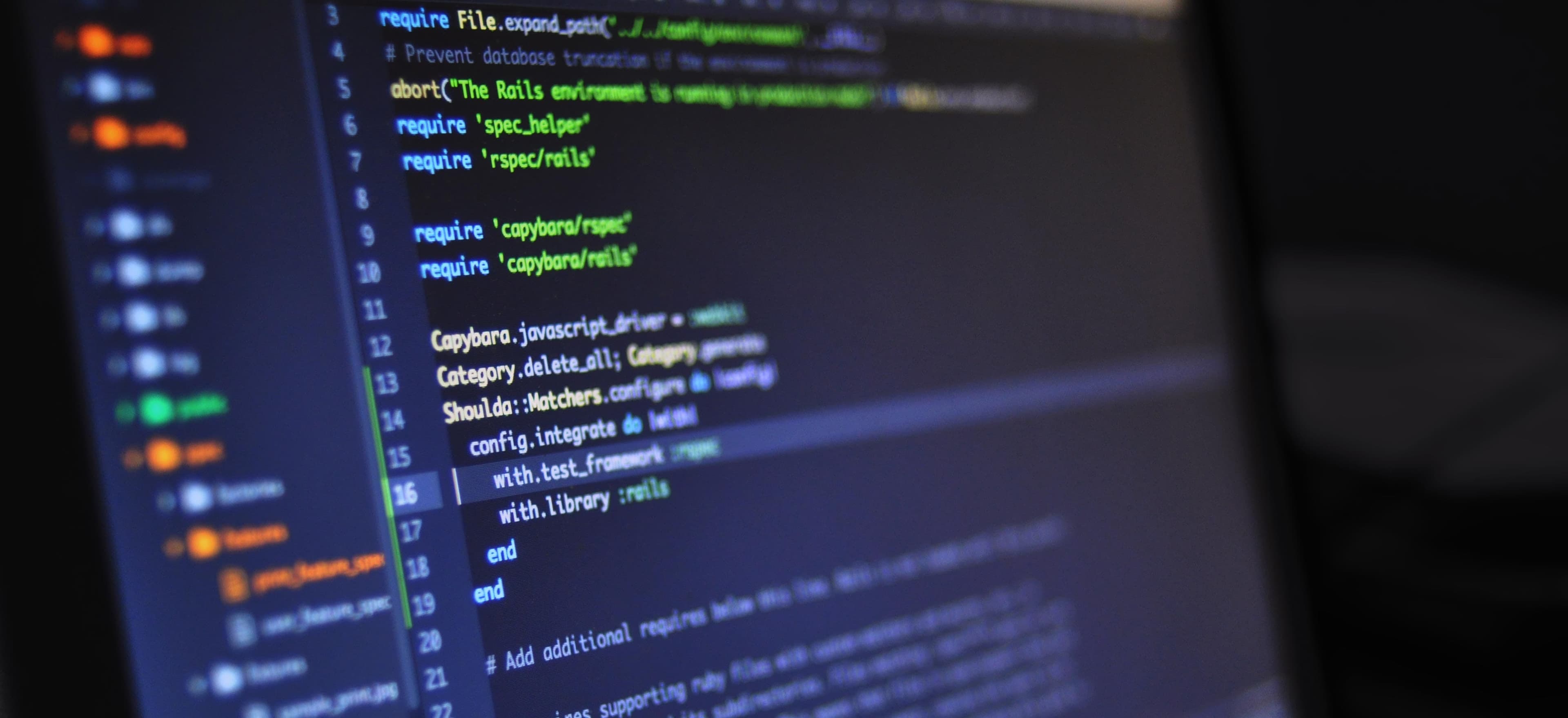
- Published on
Smooth Streaming: Fixing Android HTTP Live Camera Lags
In the relentless world of mobile app development, delivering a seamless streaming experience is crucial to keep users engaged. Particularly, when it comes to live video streaming using Android's camera over HTTP, every millisecond of lag accumulates into a mountain of frustration. As developers, it is our duty to surmount this challenge and ensure that the user experience is as smooth as silk.
In this comprehensive guide, we'll delve into the intricacies of minimizing lag in HTTP live streaming on Android, ensuring your applications provide real-time experiences that will keep users connected and satisfied.
Understanding HTTP Live Streaming (HLS)
HTTP Live Streaming, often abbreviated as HLS, is a protocol devised by Apple for sending live and on-demand video content over the internet. It works by breaking down the stream into a sequence of small HTTP-based file downloads, each containing one short chunk of the overall stream. As the stream plays, the client downloads the successive chunks and thus continues to receive the video content.
Why HLS on Android?
HLS is widely supported on Android devices, making it a go-to choice for developers looking to implement live streaming without worrying about device compatibility. Moreover, Android provides native support for HLS via the ExoPlayer
library, which we'll be using in our example.
ExoPlayer is a powerful and flexible media player for Android, developed by Google. It offers an alternative to Android's MediaPlayer API with improved performance, ease of use, and additional features like HLS support, making it ideal for our use case.
Addressing the Lags
When users complain about lag, they're often referring to two different issues: latency and buffering delays. These are the villains in our streaming storyline, but fear not. We have the strategies to combat both.
Reducing Latency
Latency is the delay between the camera capturing an event and the event being displayed on the viewer's screen. To tackle this, we aim to minimize the time spent in capturing, processing, and transmitting the video data.
Here's how you can reduce the latency effectively:
- Optimize the encoder settings: Use lower resolutions, fewer frames per second, or different encoder settings to reduce the time it takes to encode and transmit the video data.
- Lower the chunk size: Smaller chunks mean quicker processing and transfer times, although too small a size can cause its own issues. It's a delicate balance.
- Use a low-latency HLS protocol: Newer versions of HLS support low-latency streaming, and ensuring your server and client support this can cut down on lag significantly.
Minimizing Buffering Delays
Buffering delays occur when the video player pauses playback to download more data, causing an interruption in the stream. To combat buffering, you can:
- Optimize the network conditions: Use a reliable and fast network connection to ensure the data is transmitted as quickly as possible.
- Increase the buffer size: More buffered data generally means fewer interruptions due to network fluctuations.
- Optimize the player settings: Configure the player to handle network changes and adapt the quality of the stream to maintain smooth playback.
Implementing HLS in Android with ExoPlayer
Now, let's get hands-on and implement a low-latency HLS stream using ExoPlayer
for our Android application. Note that you’ll need a working HTTP server serving a live camera feed over HLS.
Step 1: Adding Dependencies
First, include the ExoPlayer dependency in your build.gradle
file:
dependencies {
implementation 'com.google.android.exoplayer:exoplayer:2.X.X' // Replace with the latest version
}
Step 2: Preparing ExoPlayer
Set up the ExoPlayer
instance in your activity or fragment:
import com.google.android.exoplayer2.ExoPlayer;
import com.google.android.exoplayer2.MediaItem;
import com.google.android.exoplayer2.SimpleExoPlayer;
SimpleExoPlayer player;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Initialize ExoPlayer
player = new SimpleExoPlayer.Builder(this).build();
// Bind the player to the view
PlayerView playerView = findViewById(R.id.player_view);
playerView.setPlayer(player);
}
Step 3: Configuring the HLS Media Source
Create and configure the HLS media source, particularly focusing on minimizing lag:
import com.google.android.exoplayer2.source.hls.HlsMediaSource;
import com.google.android.exoplayer2.upstream.DefaultHttpDataSource;
// URL of the live HLS stream
String hlsUrl = "https://example.com/live.m3u8";
// Increase the buffer for reducing buffering delays
player.setBufferSize(10 * 1024 * 1024); // 10MB
DefaultHttpDataSource.Factory dataSourceFactory = new DefaultHttpDataSource.Factory()
.setAllowCrossProtocolRedirects(true);
HlsMediaSource hlsMediaSource = new HlsMediaSource.Factory(dataSourceFactory)
.setAllowChunklessPreparation(true) // Reduces latency introduced by chunk boundaries
.createMediaSource(MediaItem.fromUri(hlsUrl));
// Prepare the player with the HLS media source
player.setMediaSource(hlsMediaSource);
player.prepare();
player.playWhenReady = true;
Step 4: Handling Lifecycle Events
Don't forget to release the ExoPlayer
resources when not in use to prevent memory leaks:
@Override
public void onStart() {
super.onStart();
if (Util.SDK_INT > 23) {
player.play();
}
}
@Override
public void onResume() {
super.onResume();
if (Util.SDK_INT <= 23 || player == null) {
player.play();
}
}
@Override
public void onPause() {
super.onPause();
if (Util.SDK_INT <= 23) {
player.pause();
}
}
@Override
public void onStop() {
super.onStop();
if (Util.SDK_INT > 23) {
player.pause();
}
}
@Override
public void onDestroy() {
super.onDestroy();
player.release(); // Don’t forget to release player’s resources!
}
Why These Steps?
Breaking down the above code, we see a conscious effort to thread the needle between performance and quality. The buffer size is a pivotal parameter, ensuring that the player has enough data to continue playback during network fluctuations without lagging behind the live edge too much. The setAllowChunklessPreparation(true)
configuration helps to reduce the latency by allowing playback to start without having to wait for a new chunk to be prepared.
Combating Real-world Challenges
Remember, the theory is pristine but the real world is messy; network conditions vary, devices differ in capabilities, and users have high expectations. Be prepared to dynamically adjust your settings based on real-world usage data. Use analytics and user feedback to tweak encoder settings, buffer sizes, and other configurations.
Conclusion
Ensuring smooth live streaming on Android can seem daunting, buy with the right tools and a detailed approach, it's an achievable feat. By optimizing encoder settings, carefully choosing your chunk sizes, utilizing low-latency protocols, and fine-tuning your player's buffer, you can dramatically improve the streaming experience in your Android applications.
Happy streaming and may the live be with you!