Jumping from Go to Java: Mastering Control Structures
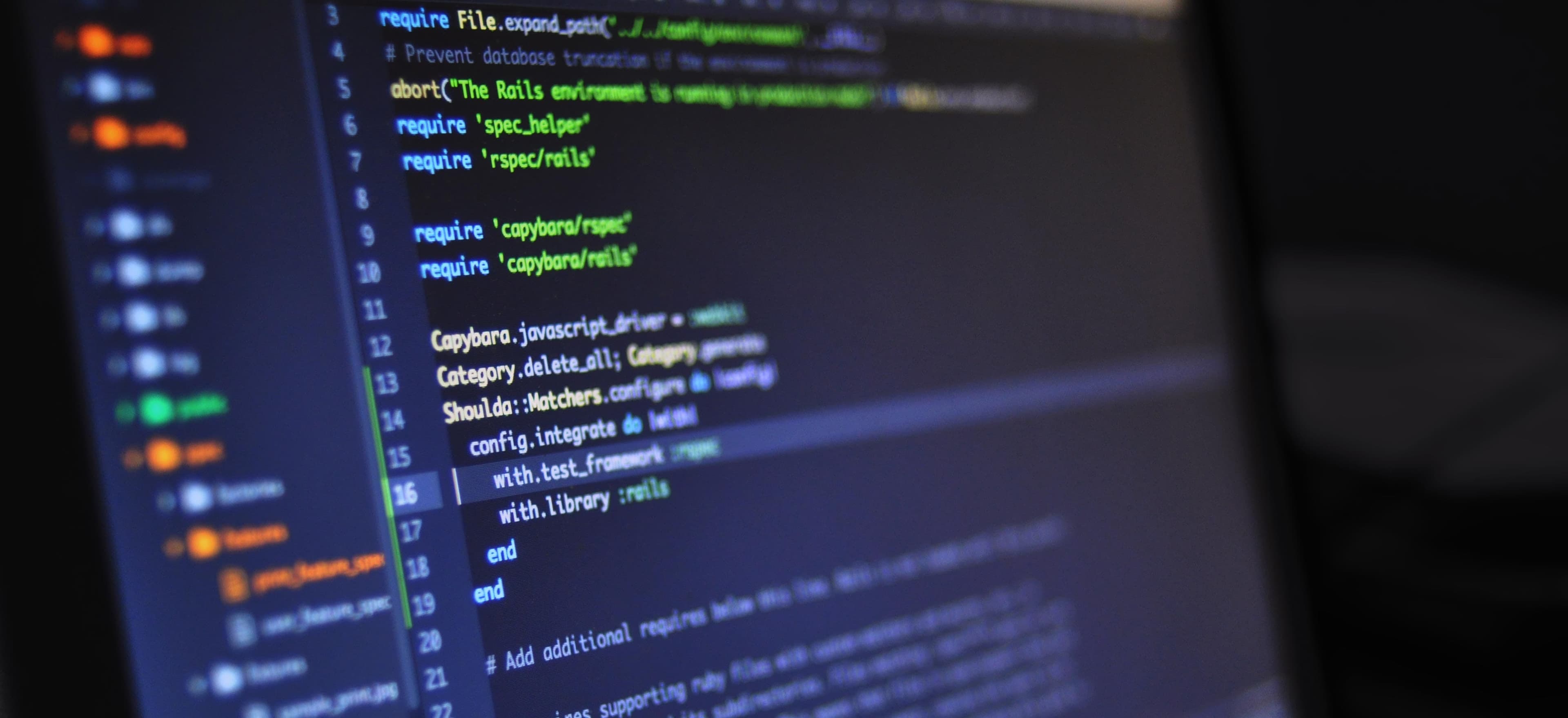
- Published on
Jumping from Go to Java: Mastering Control Structures
Are you familiar with Go and now dipping your toes into the Java ecosystem? Transitioning between programming languages can be a daunting task, but understanding each language's control structures is a pivotal step toward gaining proficiency. Control structures form the backbone of your program's flow, and thankfully, many concepts from Go translate well into Java.
In this post, we'll navigate through the different control structures in Java, especially focusing on those who are already familiar with their counterparts in Go, offering comparisons and highlighting nuances. By the end, you'll not only be comfortable with Java's control structures but also understand the whys behind the code snippets we discuss.
Conditional Statements
Conditional statements offer a direct comparison between Go and Java. Let's start by exploring the if-else
statement — a fundamental control structure present in both languages.
If-Else in Java vs. Go
Go Example:
if condition {
// Code to execute if the condition is true
} else {
// Code to execute if the condition is false
}
Java Equivalent:
if (condition) {
// Code to execute if the condition is true
} else {
// Code to execute if the condition is false
}
The syntax for if-else
is nearly identical in both languages, with the key difference being the use of parentheses in Java. Why does Java use parentheses? Mainly because it clearly defines the boundary of the condition expression, adhering to Java's stricter syntax rules.
Switch-Case
Switch-case statements offer a way to execute different parts of code based on the value of an expression. Java's switch
statement is similar to Go's, but with some syntax differences.
Go Example:
switch value {
case 1:
// Code for case 1
case 2:
// Code for case 2
default:
// Code for all other cases
}
Java Equivalent:
switch (value) {
case 1:
// Code for case 1
break;
case 2:
// Code for case 2
break;
default:
// Code for all other cases
}
Why the break
? In Java, without a break
, execution falls through to subsequent cases, which can lead to unexpected behavior if not intentionally designed. Newer versions of Java (Java 12 onwards) introduced the switch
expressions, which handle cases differently and don't require a break
. Read more about switch expressions here: Java Switch Expressions.
Loops
Loops are a way to repeat a set of operations multiple times. Java supports several types of loops that are also available in Go, with some syntax tweaks.
For Loop
For loops in Java are slightly more verbose than in Go, primarily due to Java's strong typing and the declaration of the looping variable inside the loop statement.
Go Example:
for i := 0; i < 10; i++ {
// Loop body
}
Java Equivalent:
for (int i = 0; i < 10; i++) {
// Loop body
}
Why the type declaration? Java is statically-typed and requires you to specify the type of the variable, which provides compile-time checking and optimization that can prevent unexpected runtime errors.
While Loop
While loops in Java function exactly as you expect coming from Go.
Go Example:
i := 0
for i < 10 {
// Loop body
i++
}
Java Equivalent:
int i = 0;
while (i < 10) {
// Loop body
i++;
}
In both snippets, we explicitly manage the loop variable i
. However, Java demands declaration of the variable's type.
Do-While Loop
Java, unlike Go, offers a do-while
loop, which guarantees that the loop body will execute at least once.
Java Example:
int i = 0;
do {
// Loop body
i++;
} while (i < 10);
Go does not have a direct do-while
equivalent. Why have this control structure? Sometimes, you want the code within the loop to execute before the condition is checked, such as when prompting for user input and validating it.
Enhanced For Loop (For-Each)
Java's enhanced for
loop (also known as the for-each
loop) is an elegant way to iterate over arrays or collections. There's no direct feature like this in Go; you use the range
keyword instead.
Go Example with range
:
for index, value := range array {
// Use index and/or value
}
Java Equivalent:
for (int value : array) {
// Use value
}
Why use the enhanced for loop in Java? It simplifies the iteration process and eliminates the need to manage the index variable manually. This construct minimizes the chance of errors like off-by-one mistakes.
Break and Continue
Both Java and Go use break
and continue
statements within loops to alter the flow of control.
Go Example:
for i := 0; i < 10; i++ {
if i == 5 {
continue // Skip the rest of this iteration if i is 5
}
if i == 8 {
break // Exit the loop if i is 8
}
// Loop body
}
Java Equivalent:
for (int i = 0; i < 10; i++) {
if (i == 5) {
continue; // Skip the rest of this iteration if i is 5
}
if (i == 8) {
break; // Exit the loop if i is 8
}
// Loop body
}
The logic remains consistent across both languages — continue
skips the current iteration, and break
exits the loop. Both are essential for fine-grained control over loop execution.
Exception Handling
Exception handling in Java is robust, allowing you to manage errors predictably with try-catch
blocks. In Go, error handling is done by returning an error
type from functions.
Go Example:
val, err := mightFail()
if err != nil {
// Handle the error
}
Java Equivalent:
try {
// Code that might throw an exception
} catch (ExceptionType e) {
// Handle the exception
}
Why the discrepancy? Java's exception handling mechanism provides a structured way to catch and handle different types of errors separately, while also allowing the propagation of errors up the call stack until they are properly handled.
Conclusion
Transitioning from Go to Java can seem intimidating, but comparing similar control structures can ease the learning curve. As we have seen, the constructs that govern the flow of a program remain consistent in concept, even though syntax and usage details may differ. Taking full advantage of Java's control structures can make your code more readable, robust, and maintainable.
While we've covered the main control structures, Java has many other nuances and features to explore. Don't hesitate to delve into the Java documentation to expand your knowledge. Keep experimenting with code snippets, understand the underlying principles, and soon, you'll be writing Java code with the same fluency as you wrote Go.
Remember, whether it's conditional logic, loops, or error handling, mastering control structures is a vital step on the path to becoming a proficient Java developer, and taking the time to explore the details will pay off in your programming journey.