Maximizing Impact: Thriving as an Individual Contributor
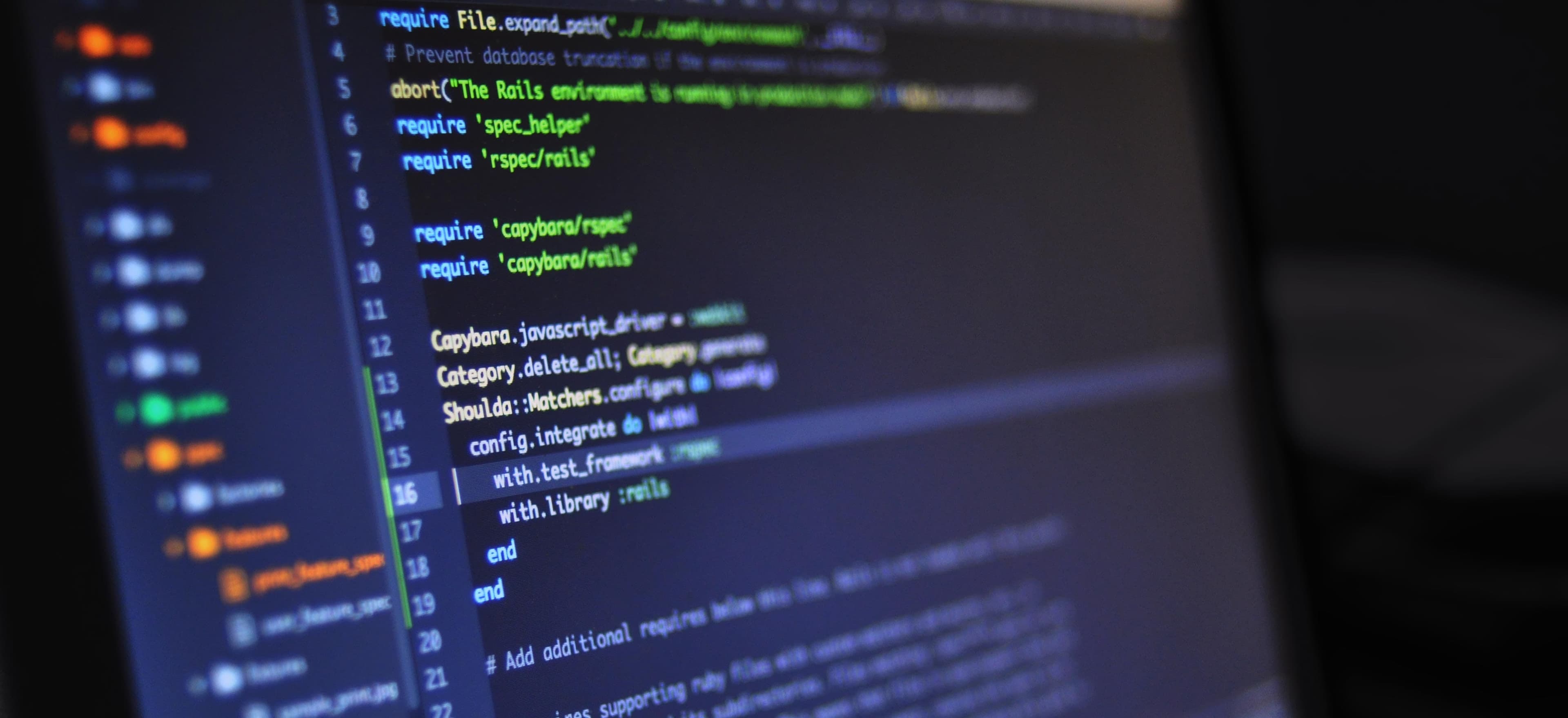
- Published on
Maximizing Impact: Thriving as an Individual Contributor in the Java Ecosystem
Java remains one of the most popular and widely used programming languages in the world—a testament to its versatility, efficiency, and community support. As an individual contributor in the Java ecosystem, you have the power to make remarkable impact through code quality, innovation, and collaboration. But what strategies can you employ to ensure your contributions not only stand out but also drive projects forward? Let’s explore.
Understanding Java’s Place in the Digital Landscape
Java, developed by Sun Microsystems in the mid-1990s and now maintained by Oracle, is used by millions of developers. Its platform independence, robust libraries, and time-tested frameworks underpin countless applications, from Android apps to enterprise software.
To fully appreciate the impact you can have as an individual contributor, it's crucial to recognize Java's penetration across industries. Learn more by exploring Oracle's official Java resources.
Write Clean, Maintainable Code
Writing clean code isn't just about making it look nice—it's about ensuring that your code is readable, maintainable, and scalable. This is especially important in large Java projects where various components are interconnected.
Best Practices
Here are some Java best practices that can significantly enhance code quality:
- Follow Naming Conventions: Use clear, meaningful variable, method, and class names.
- Adhere to SOLID Principles: These design principles promote code reusability and maintainability.
- Document Code Well: Javadocs should accurately describe methods and classes for fellow developers.
- Refactor Ruthlessly: Regularly revisit and refine code to improve its structure and clarity.
Example: Refactoring for Clarity
// Before refactoring
public void pM(List<String> l) {
for (String s : l) {
if (s.length() > 10) {
System.out.println(s);
}
}
}
// After refactoring
public void printLongStrings(List<String> strings) {
strings.stream()
.filter(string -> string.length() > 10)
.forEach(System.out::println);
}
Why this code? The refactored version uses descriptive names making it immediately clear what the method does. Plus, employing streams aligns with modern Java practices.
Leverage Frameworks and Libraries
Java's rich ecosystem of frameworks and libraries can significantly speed up development and provide solutions to common problems.
Popular Frameworks
- Spring Boot: Ideal for building stand-alone, production-grade applications.
- Hibernate: Targets the problem of ORM (Object-Relational Mapping) with ease.
- Apache Kafka: A distributed streaming platform that can handle high-throughput data pipelines.
Example: Simplifying REST API Creation with Spring Boot
@RestController
public class GreetingController {
@GetMapping("/greeting")
public ResponseEntity<String> greet(@RequestParam(value = "name", defaultValue = "World") String name) {
return new ResponseEntity<>("Hello, " + name + "!", HttpStatus.OK);
}
}
Why this code? Spring Boot makes REST API development trivial with annotations and embedded servers. This snippet demonstrates a simple API without boilerplate.
Embrace Testing
Testing is often overlooked but is fundamental to ensuring code reliability. In the Java ecosystem, there are numerous tools at your disposal.
Testing Tools
- JUnit: The de-facto standard for unit testing in Java.
- Mockito: A mocking framework for isolating unit tests.
- Selenium: Used for automating web browser interaction, perfect for end-to-end testing.
Example: Unit Testing with JUnit
class MathUtilsTest {
@Test
void testAdd() {
assertEquals(5, MathUtils.add(2, 3), "Addition should add two numbers");
}
}
Why this code? JUnit tests help catch regressions and ensure code behaves as expected. The simplicity of writing tests in JUnit promotes test-driven development (TDD).
Keep Learning and Stay Updated
The Java world is dynamic. Staying informed about the latest trends, releases, and best practices is crucial.
Continuous Learning Resources
- Oracle's Java Magazine: Offers deep insights into Java development.
- Java conferences: Events like Oracle Code One and Jfokus are great for networking and learning.
- Online courses: Platforms like Coursera and Udemy have myriad Java courses.
Contribute to Open Source
Contributing to open source projects can enhance your skills, expand your network, and open up new career opportunities.
How to Get Started
- Find a Java project: Explore GitHub for projects that interest you.
- Start small: Look for
good first issue
tags or documentation fixes to get your feet wet. - Be responsive: Engage with the community and respond to feedback on your contributions.
Example: Contributing to OpenJDK
# Getting the OpenJDK source code
hg clone http://hg.openjdk.java.net/jdk8/jdk8 openjdk8
cd openjdk8
# Building OpenJDK
bash get_source.sh
make all
Why this code? OpenJDK is the reference implementation of Java, and contributing to it requires knowing how to get and build the source code—key steps for any aspiring contributor.
Network and Collaborate
Collaborating with others can lead to better solutions and more robust software. Leverage platforms like LinkedIn, Twitter, and Reddit to connect with other Java developers.
Networking Tips
- Join Java User Groups (JUGs): Connect with local community members.
- Participate in Code Reviews: Offer constructive feedback and learn from peers.
- Speak at Meetups or Conferences: Sharing knowledge can establish you as a thought leader.
Conclusion
Thriving as an individual contributor in the Java ecosystem is about more than writing code—it's about writing excellent code that is part of a larger community and industry effort. Clean coding, learning, testing, and connecting are just the starting points. Engage with the fabric of the Java community through open source and networking, and maintain an insatiable curiosity towards the new and the challenging.
Embark on the journey to maximize your impact in Java. Code with intent, collaborate with heart, and continuously strive for excellence. Your journey as a significant contributor begins now.