Mastering SOAP: Fixing Enveloped XML Signature Issues
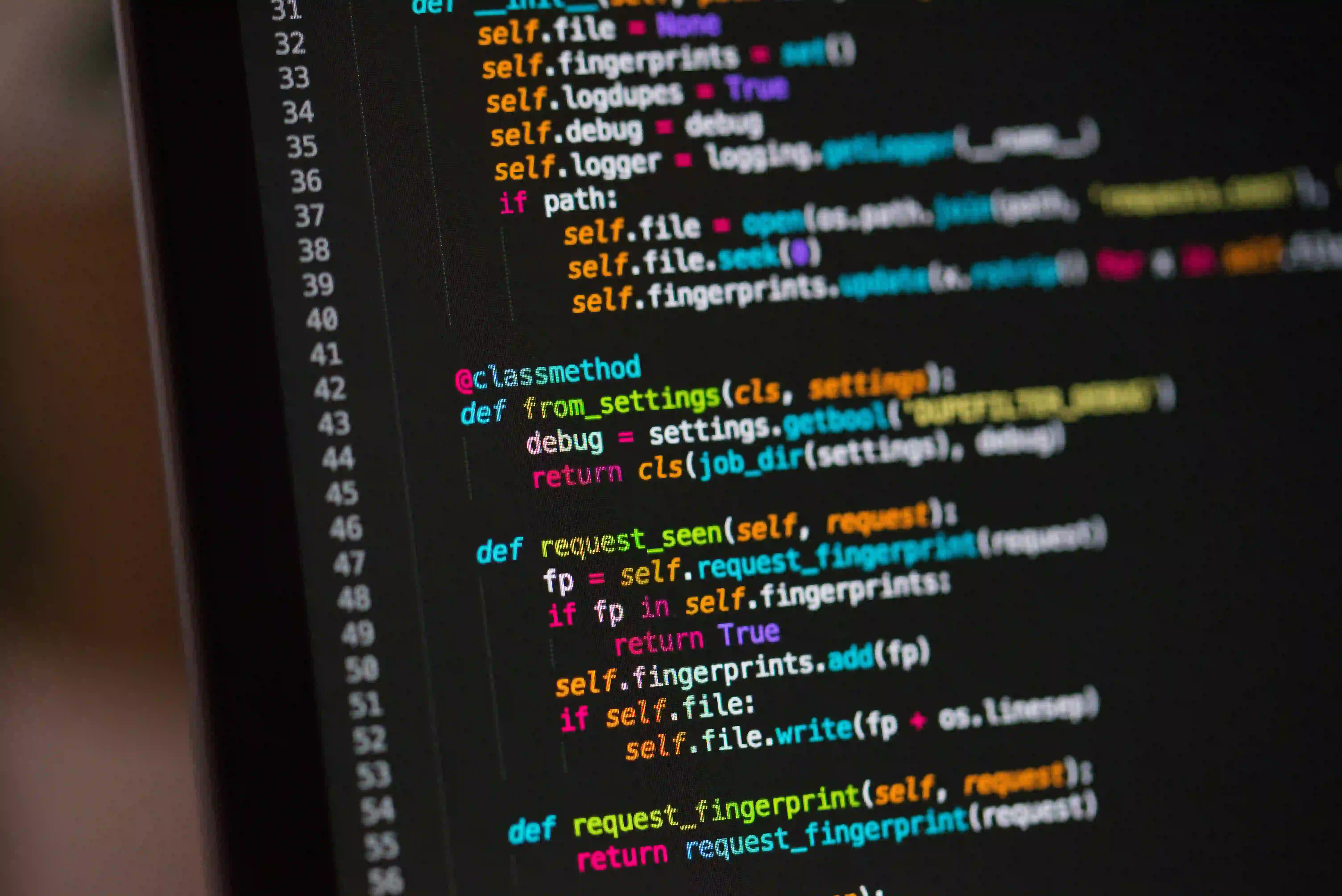
Mastering SOAP: Fixing Enveloped XML Signature Issues in Java
SOAP (Simple Object Access Protocol) is a protocol in web services offering a means for applications to communicate with each other over the Internet. It is characterized by its use of XML (Extensible Markup Language) to format the data, which gives rise to a set of challenges unique to SOAP. One of the common intricacies developers face when working with SOAP services is managing XML signatures, particularly when fixing enveloped XML signature issues. Understanding the intricacies of SOAP and XML signatures is essential for any developer looking to excel in web services development, and Java provides a robust toolkit for that purpose.
Understanding Enveloped XML Signatures
Enveloped XML signatures are a type of XML digital signature where the signature is located within the SOAP message itself, specifically, within the XML that it is signing. This structure ensures the authentication and integrity of the sent message but can also lead to complications. Common issues include incorrect formatting, validation errors, or issues with the signature's reference URI.
The Importance of XML Signatures in Java
XML signatures are critical for secure communication in distributed systems. They allow both message integrity and authentication, which are essential for web services operating in an environment where trustworthiness is paramount. Java, with its extensive libraries like Apache Santuario and Java Cryptography Architecture (JCA), offers developers powerful tools for managing these signatures.
Fixing Common Enveloped XML Signature Issues
In order to resolve issues related to enveloped XML signatures in Java, it's important to grasp both the theoretical and practical aspects of it. Let's delve into some coding examples to illustrate how you would manage these issues.
1. Generating a Correct Enveloped XML Signature
To generate a proper enveloped XML signature in Java, you often use the Apache Santuario library – a comprehensive toolkit for XML security tasks. Here is a sample code snippet for creating an enveloped signature:
import org.apache.xml.security.c14n.Canonicalizer;
import org.apache.xml.security.signature.XMLSignature;
import org.apache.xml.security.transforms.Transforms;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
public void createEnvelopedSignature(Document doc) {
// Initialize the XML security library
org.apache.xml.security.Init.init();
// Create a new XMLSignature object
XMLSignature sig = new XMLSignature(doc, null, XMLSignature.ALGO_ID_SIGNATURE_RSA);
// Get the root element of the document and append the signature node
Element root = doc.getDocumentElement();
root.appendChild(sig.getElement());
// Prepare to generate the signature by specifying the transforms and canonicalization method
Transforms transforms = new Transforms(doc);
transforms.addTransform(Transforms.TRANSFORM_ENVELOPED_SIGNATURE);
transforms.addTransform(Canonicalizer.ALGO_ID_C14N_WITH_COMMENTS);
// Add the transforms object to the signature
sig.addDocument("", transforms, Constants.ALGO_ID_DIGEST_SHA1);
// Perform additional steps to generate the signature using private key, etc.
// ...
}
In this code, org.apache.xml.security.Init.init()
initializes the security library, Transforms
are set up to describe how the XML content should be treated before signing, and XMLSignature
creates the signature element you need to append to the XML document. The code implies but does not explicitly show the need for you to provide a private key and likely a signing certificate.
For more intricate details, Apache Santuario documentation offers a deeper dive.
2. Validating an Enveloped XML Signature
Validating an XML signature ensures its authenticity and integrity. Here's how to approach it in Java:
import org.apache.xml.security.signature.XMLSignature;
import org.apache.xml.security.utils.Constants;
import org.apache.xml.security.keys.KeyInfo;
import org.w3c.dom.Document;
import org.w3c.dom.Node;
public boolean validateSignature(Document doc) {
// Initialize the library
org.apache.xml.security.Init.init();
// Find the Signature element
Node signatureNode = doc.getElementsByTagNameNS(Constants.SignatureSpecNS, "Signature").item(0);
// Create an XMLSignature from the signature node
XMLSignature signature = new XMLSignature((Element) signatureNode, null);
// Retrieve the KeyInfo data and the certificate for validation
KeyInfo keyInfo = signature.getKeyInfo();
// If we can retrieve the public key from the KeyInfo object, proceed with validation
if (keyInfo != null) {
PublicKey publicKey = keyInfo.getPublicKey();
// Check signature validity
boolean isValid = signature.checkSignatureValue(publicKey);
return isValid;
}
return false;
}
This simple validation routine involves finding the signature XML element within the SOAP message, constructing an XMLSignature
object from it, extracting public key information via KeyInfo
, and then checking if the signature is valid.
Common Error Handling
When errors occur, detailed error messages are crucial in finding a resolution. Remember to catch exceptions, such as XMLSignatureException
or XMLSecurityException
, and log or handle them accordingly to diagnose issues effectively.
Advanced Considerations
- Performance: Enveloped XML signatures can add computational overhead. Always profile and optimise your code for performance-critical applications.
- Security: Keep your libraries up to date to mitigate known security vulnerabilities, and use strong algorithms that are considered secure as of current standards.
- Compatibility: Ensure that the SOAP services and the signatures are compatible with client and server-side applications.
Conclusion
Enveloped XML signatures are a fundamental aspect of working with SOAP using Java, providing a means for secure and reliable message exchange. By mastering the creation and validation of these signatures, you can avoid common pitfalls and build robust and trustable web services. Using libraries like Apache Santuario, adhering to security best practices, and applying thorough error handling strategies are keys to success in managing enveloped XML signatures. With the knowledge from this post, you're one step closer to mastering Java's web services landscape.
Don't stop here! Explore the libraries mentioned, do some hands-on coding, and keep up with the latest in Java security to excel in this space. Happy coding!