Boost Performance: Optimize Spring Data with Read Replicas
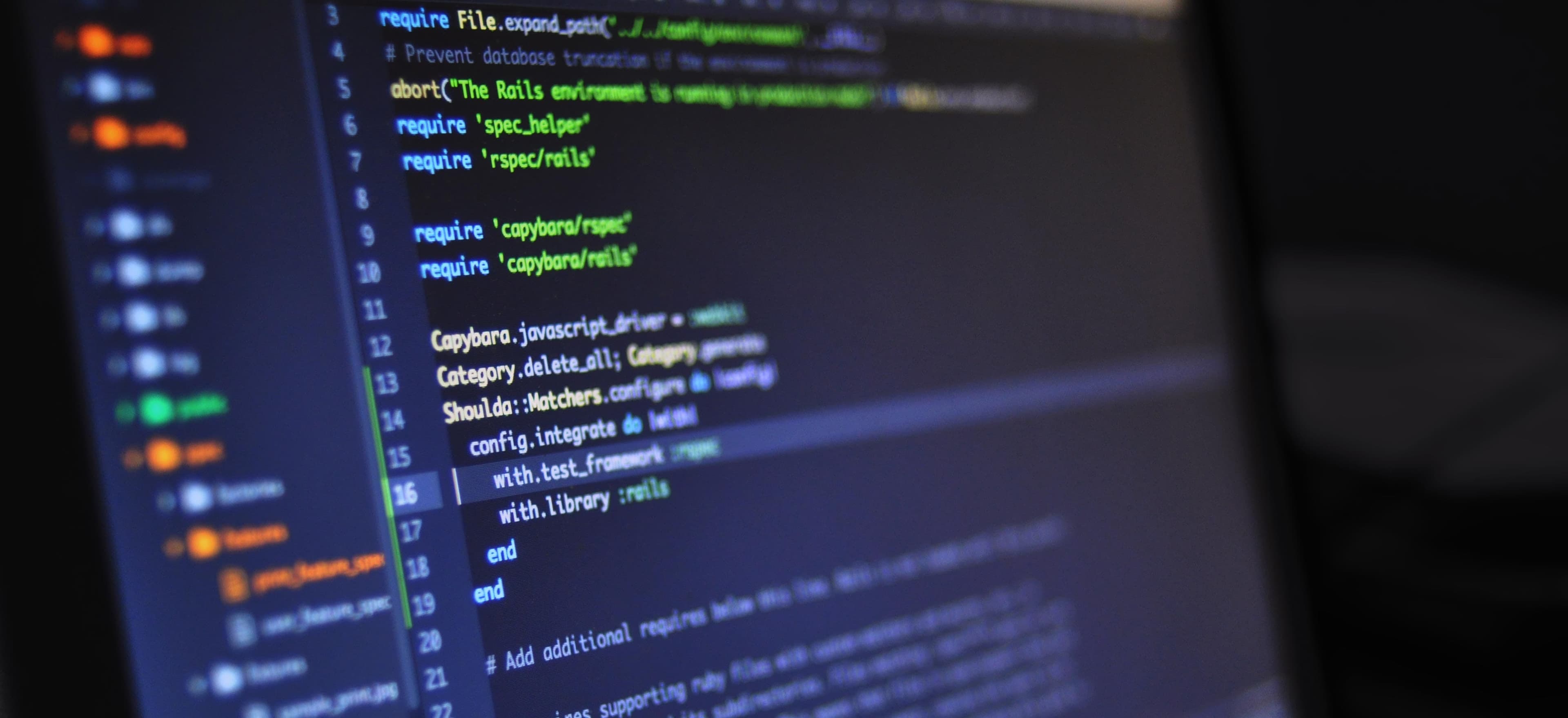
- Published on
Boost Performance: Optimize Spring Data with Read Replicas
For many developers, Spring Data represents the golden standard for persisting data in Java applications. Its simple, powerful repository abstractions make accessing a database almost trivial. However, as your application scales, performance can take a hit, especially when it comes to read-heavy workloads. One solution is to introduce read replicas, an optimization technique that can significantly improve your application's read performance.
In this deep dive, we'll explore how to turbocharge your Spring Data JPA application by configuring read replicas—ensuring you extract maximum efficiency with minimal fuss. Let's get started!
Understanding Read Replicas
Typically, a database setup will have a primary (or master) node that handles both read and write operations. As the number of transactions increase, the primary node can become a bottleneck. Enter read replicas: extra database instances that mirror your primary database. These replicas handle read queries, which can dramatically reduce the load on your primary database and speed up response times for read operations.
!Read Replicas Concept
Why use Read Replicas?
- Scalability: Distributes the query load across multiple databases.
- Performance: Reduces the read latency by handling multiple requests in parallel.
- High Availability: Provides redundancy, as other replicas can be promoted to primary if needed.
Configuring Read Replicas in Spring Data
Let's jump into the practical side. Assume you already have a Spring Boot application with Spring Data JPA configured. Now, you want to add read replicas into the mix. Here's a step-by-step guide on how to do just that.
Prerequisites
- Spring Boot application with Spring Data JPA
- Primary database and read replicas set up
- Basic knowledge of Java and Spring Framework
Step 1: Define the Datasource Properties
Start by defining the primary and read replica datasource properties in your application.properties
or application.yml
.
spring.datasource.primary.url=jdbc:mysql://primary-database:3306/your-db
spring.datasource.primary.username=primary-user
spring.datasource.primary.password=primary-pass
spring.datasource.replica.url=jdbc:mysql://replica-database:3306/your-db
spring.datasource.replica.username=replica-user
spring.datasource.replica.password=replica-pass
Step 2: Configure the DataSources
Create a configuration class to set up your primary and replica DataSources. You'll need to make sure that transactions are still routed correctly, with writes going to the primary database.
@Configuration
public class DataSourceConfig {
@Primary
@Bean(name = "primaryDataSource")
@ConfigurationProperties(prefix = "spring.datasource.primary")
public DataSource primaryDataSource() {
return DataSourceBuilder.create().build();
}
@Bean(name = "replicaDataSource")
@ConfigurationProperties(prefix = "spring.datasource.replica")
public DataSource replicaDataSource() {
return DataSourceBuilder.create().build();
}
}
Step 3: Configure the EntityManager
The EntityManager
is the key to interact with the persistence context. Configure separate EntityManagerFactory
beans for the primary and replica DataSources.
@Bean(name = "transactionManager")
public PlatformTransactionManager transactionManager(
@Qualifier("entityManagerFactory") EntityManagerFactory entityManagerFactory) {
return new JpaTransactionManager(entityManagerFactory);
}
@Primary
@Bean(name = "entityManagerFactory")
public LocalContainerEntityManagerFactoryBean primaryEntityManagerFactory(
EntityManagerFactoryBuilder builder,
@Qualifier("primaryDataSource") DataSource dataSource) {
return builder
.dataSource(dataSource)
.packages("com.yourpackage.model")
.persistenceUnit("primary")
.build();
}
To route read-only operations to the read replica, you have to define a replica EntityManagerFactory
with a different transaction manager.
Step 4: Routing to the Correct DataSource
With multiple DataSources configured, you now need a routing mechanism. For this, you can implement Spring's AbstractRoutingDataSource
to route database operations to the correct DataSource based on the transaction's read-only status.
public class ReplicaRoutingDataSource extends AbstractRoutingDataSource {
@Override
protected Object determineCurrentLookupKey() {
return TransactionSynchronizationManager.isCurrentTransactionReadOnly()
? "replicaDataSource"
: "primaryDataSource";
}
}
When a transaction is marked as read-only, the ReplicaRoutingDataSource
will route to the replica; otherwise, it'll use the primary DataSource.
Step 5: Marking Transactions as Read-Only
Properly annotating your service methods with @Transactional(readOnly = true)
is essential to ensure queries go to your replica.
@Service
public class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
@Transactional(readOnly = true)
public User getUserById(Long id) {
return userRepository.findById(id)
.orElseThrow(() -> new ResourceNotFoundException("User not found"));
}
@Transactional
public User saveUser(User user) {
return userRepository.save(user);
}
}
It's all about context: getUserById
reads data and should be routed to the replica, while saveUser
modifies data and must go to the primary database.
Real-World Considerations
Configuration is only part of the picture. Using read replicas in production comes with additional considerations:
- Consistency: There could be a lag between the primary and the replicas. Ensure your application can handle eventual consistency.
- Monitoring: Keeping an eye on replication lag and query performance is critical.
- Failure Handling: Implementing failover strategies is crucial if a replica goes down.
Conclusion
Optimizing Spring Data with read replicas is a powerful strategy to enhance the scalability and performance of your Java applications. By distributing the load across multiple nodes, you minimize bottlenecks and maintain a snappy user experience, even under heavy read demands.
While there's a bit of upfront work to correctly configure and manage multiple data sources, the dividends it pays in improved performance and reliability are well worth it. Dive into the world of read replicas and unleash the full potential of your Spring Boot applications!
Want to learn more about read replicas and Spring? Check out Spring's official documentation.
Happy coding, and may your reads always be swift!