Unzip with Ease: Groovy & 7-Zip-JBinding Integration
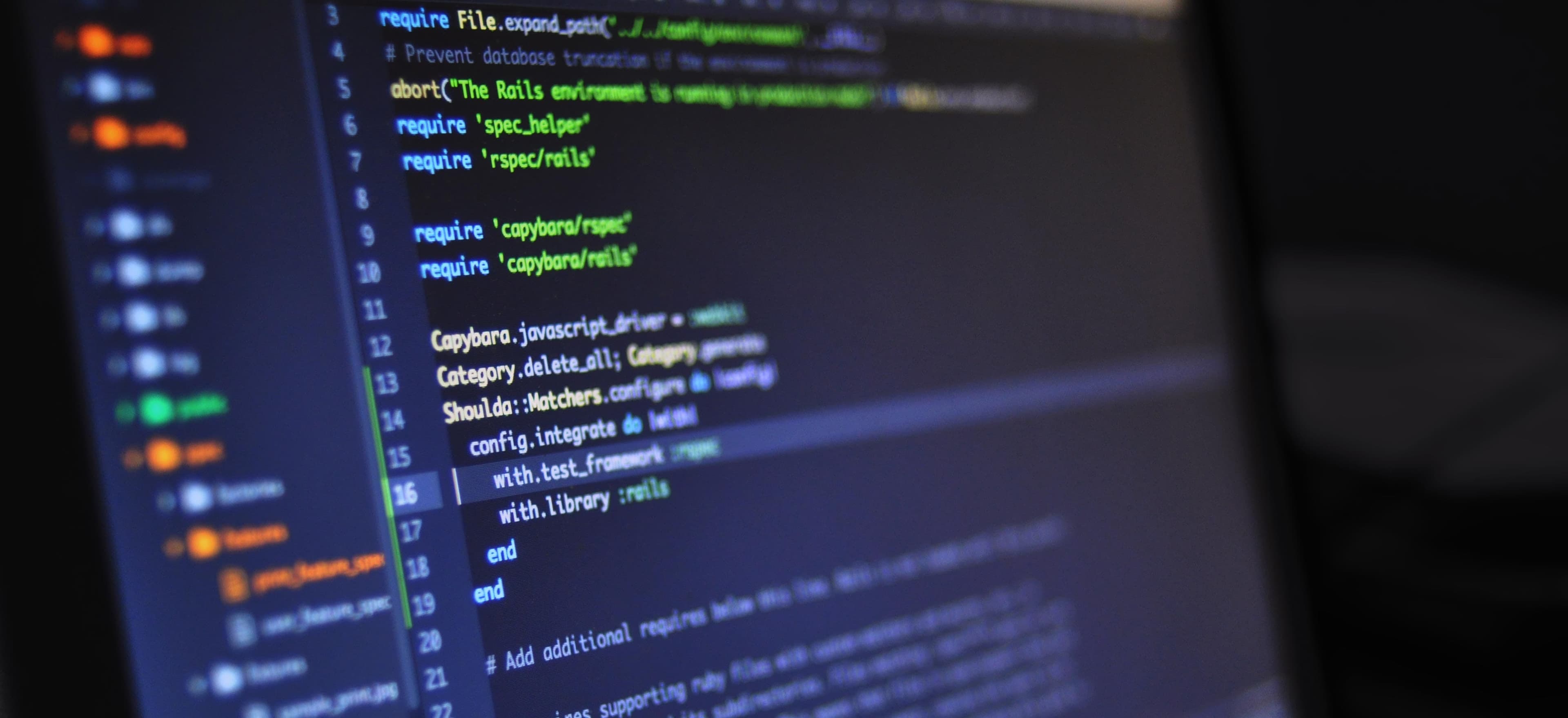
- Published on
Unzip with Ease: Groovy & 7-Zip-JBinding Integration
When it comes to handling archive files like ZIP, RAR, or 7z, Java developers have a range of options at their disposal. However, for a powerful and seamless experience, integrating 7-Zip-JBinding with Groovy offers a unique solution that's both efficient and developer-friendly. In this post, we'll explore how the Java library 7-Zip-JBinding can tap into the Native 7-Zip archive handling capabilities, and we'll leverage Groovy's scripting prowess to make the code more expressive and concise.
Harnessing The Power of 7-Zip-JBinding in Java
7-Zip-JBinding is a java library which allows you to leverage the powerful 7-Zip compression algorithm. It's an unparalleled tool for accessing a diverse array of archive formats in Java, thanks to its native integration with the 7-Zip engine.
Why 7-Zip-JBinding?
- Broad Format Support: 7-Zip-JBinding supports a multitude of archive formats out of the box.
- Native Performance: By using the native 7-Zip library, you gain performance that's hard to match with pure Java solutions.
- Flexibility: Extract single files, stream content, or manage archives - all with equal ease.
However, working with JNI (Java Native Interface) libraries can sometimes feel daunting due to the complexity involved in handling native resources. This is where Groovy comes into the picture, simplifying the coding process with its syntactic sugar and dynamic capabilities.
Groovy: Java's Concise Companion
Groovy is a dynamic scripting language that runs on the Java Virtual Machine (JVM) and is fully interoperable with Java. This means you can use all Java libraries, including 7-Zip-JBinding, within Groovy just as you would in a Java application but with less boilerplate code and a more expressive syntax.
Advantages of Groovy:
- Simplified Syntax: Write code that is easier to read and maintain.
- Scripting Capabilities: Execute scripts without the need for compilation steps, aiding in rapid development.
- Dynamic Language Features: Take advantage of metaprogramming, closures, and builders to accomplish more with less code.
Now let's dive into the practical implementation.
Unzipping Files with Groovy and 7-Zip-JBinding
To begin, we need to set up our Groovy environment and include the 7-Zip-JBinding library in our project. You can manage dependencies through Gradle or Maven, ensuring you have the correct native binaries for your platform.
Gradle Dependency
dependencies {
implementation 'net.sf.sevenzipjbinding:sevenzipjbinding:16.02-2.01'
implementation 'net.sf.sevenzipjbinding:sevenzipjbinding-all-platforms:16.02-2.01'
}
Maven Dependency
<dependency>
<groupId>net.sf.sevenzipjbinding</groupId>
<artifactId>sevenzipjbinding</artifactId>
<version>16.02-2.01</version>
</dependency>
<dependency>
<groupId>net.sf.sevenzipjbinding</groupId>
<artifactId>sevenzipjbinding-all-platforms</artifactId>
<version>16.02-2.01</version>
</dependency>
Ensure you have the correct version of the library that matches your system's architecture.
Writing the Unzip Logic:
The following Groovy script demonstrates extracting files from a ZIP archive:
import net.sf.sevenzipjbinding.*
import net.sf.sevenzipjbinding.impl.RandomAccessFileInStream
import java.io.RandomAccessFile
import java.nio.file.Files
import java.nio.file.Paths
class ArchiveExtractor {
static void extract(String archivePath, String outputPath) {
RandomAccessFile randomAccessFile = new RandomAccessFile(archivePath, "r")
IInArchive inArchive = null
try {
inArchive = SevenZip.openInArchive(null, new RandomAccessFileInStream(randomAccessFile))
inArchive.extract(null, false, new IArchiveExtractCallback() {
override void setTotal(long total) {}
override void setCompleted(long complete) {}
override void getStream(int index, IOutStream[] outStream, int askExtractMode) {
if (askExtractMode != IInArchive.EXTRACT) {
return
}
String filePath = inArchive.getArchiveProperty(IInArchive.PROP_PATH) +
inArchive.getProperty(index, PropID.PATH)
File file = new File(outputPath, filePath)
file.parentFile?.mkdirs()
outStream[0] = new RandomAccessFileOutStream(new RandomAccessFile(file, "rw"))
}
override void prepareOperation(int askExtractMode) {}
override void setOperationResult(int resultEOperationResult) {}
})
} finally {
inArchive?.close()
randomAccessFile?.close()
}
}
static void main(String[] args) {
if (args.length != 2) {
println "Usage: groovy ArchiveExtractor.groovy <archivePath> <outputPath>"
System.exit(1)
}
extract(args[0], args[1])
println "Extraction complete"
}
private static class RandomAccessFileOutStream extends IOutStreamImplementation {
RandomAccessFile file
RandomAccessFileOutStream(RandomAccessFile file) {
this.file = file
}
@Override
int write(byte[] data) throws SevenZipException {
file.write(data)
return data.length
}
@Override
long seek(long offset, int seekOrigin) throws SevenZipException {
if (seekOrigin == IOutStream.SEEK_SET) {
file.seek(offset)
} else if (seekOrigin == IOutStream.SEEK_CUR) {
file.seek(file.getFilePointer() + offset)
} else {
file.seek(file.length() + offset)
}
return file.getFilePointer()
}
@Override
long size() throws SevenZipException {
return file.length()
}
@Override
void close() throws IOException {
file.close()
}
}
}
Why This Approach?
- Random Access File Management:
RandomAccessFileInStream
andRandomAccessFileOutStream
classes allow us to read and write to the disk efficiently, crucial for working with large files. - Closure Simplification: Groovy's closure syntax means that our callback implementation requires less code and is easier to read.
- Intuitive Error Handling: Exception handling is streamlined, thanks to Groovy's safe navigation operator
?.
, which gracefully managesnull
references without boilerplate checks.
Key Takeaways:
- Integration Simplicity: Groovy and Java libraries like 7-Zip-JBinding come together to tackle complex tasks with relative ease.
- Elegant Code: Groovy allows for more elegant code compared to traditional Java, enhancing readability and maintainability.
In conclusion, integrating Groovy with 7-Zip-JBinding in your Java applications is a great way to manage archives efficiently and with less code. This combination gives you the robustness of the 7-Zip compression algorithm with the agility and expressiveness of Groovy. Feel free to explore more Groovy features and get the most out of your Java applications by visiting the official Groovy documentation.
Happy Coding!
(Please note that the code examples provided are for educational purposes and should be tested and adapted as needed before using in a production environment.)