Unveil Queries: Print Hibernate SQL with Params in Spring Boot
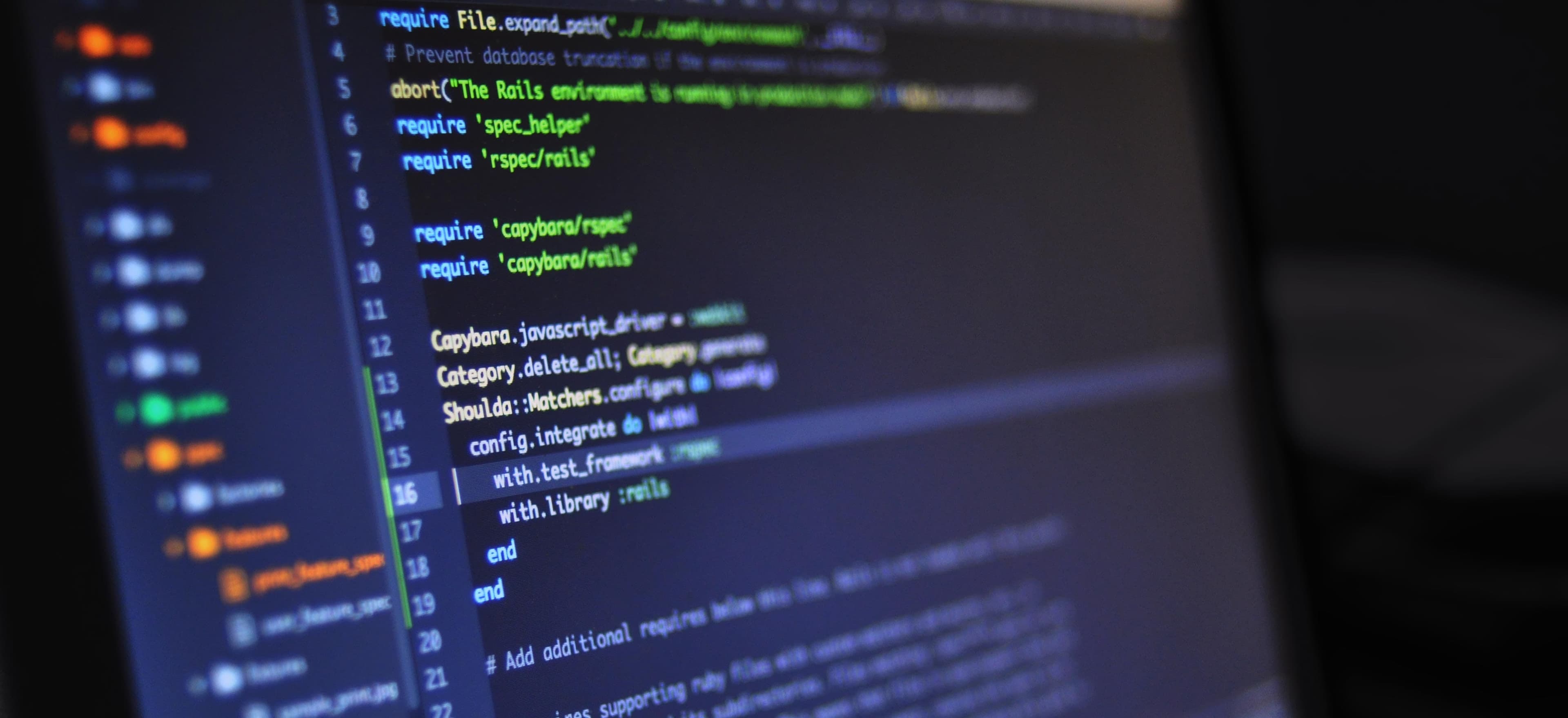
- Published on
Unveil Queries: Print Hibernate SQL with Params in Spring Boot
In the world of Java development, Hibernate is an essential tool in the arsenal of many developers, offering a seamless ORM solution for database operations. Spring Boot, with its convention-over-configuration philosophy, takes the simplicity a step further, allowing you to integrate Hibernate effortlessly. But, have you ever found yourself in a situation where deciphering what Hibernate is doing under the hood becomes a necessity? It's a common scenario when things don't quite go according to plan. Printing the SQL that Hibernate generates, complete with the parameter values, is an invaluable tool in debugging. This article will guide you through the process of achieving just that in a Spring Boot application.
The Why and How of Logging Hibernate SQL
Understanding the SQL that Hibernate generates can shed light on performance issues, unexpected behavior, and even bugs related to the ORM's interpretation of your code. So how do you get Hibernate to give up its secrets? Let's explore.
Step 1: Basic Configuration
Spring Boot has a application.properties
or application.yml
file where you can simply add a few lines to start seeing the generated SQL in your stdout (Standard Output):
# application.properties
spring.jpa.show-sql=true
spring.jpa.properties.hibernate.format_sql=true
Alternatively, if you prefer YAML:
# application.yml
spring:
jpa:
show-sql: true
properties:
hibernate:
format_sql: true
This basic configuration shows the SQL output in the logs. However, the parameters displayed are placeholders (?
), which is not helpful if you need the actual executed SQL.
Step 2: Detailed Logging
For the real deal, you'll need to configure Hibernate to output the exact statements with their parameter values. You can accomplish this by setting log levels for Hibernate's SQL logger and its parameter logger.
# application.properties
logging.level.org.hibernate.SQL=DEBUG
logging.level.org.hibernate.type.descriptor.sql.BasicBinder=TRACE
The DEBUG
level will print the SQL statements, and the TRACE
level reveals the bind parameters.
Step 3: Streamlined Output
You may notice that the log contains various timestamps, log level, and other verbosity. To streamline the output, you can configure the logging pattern in application.properties
:
# application.properties
logging.pattern.console=%d{HH:mm:ss.SSS} %-5level - %msg%n
This pattern displays the time, log level (trimmed to 5 characters), and the message, omitting class names and thread info for brevity.
Understanding the Output
Let's look at an example. Suppose you have a User
entity, and you execute a query to retrieve all users with a certain name:
// In a @Service or @Repository component
List<User> users = userRepository.findByName("John Doe");
The output in your log might look something like this:
-- Console output with TRACE logging enabled
12:34:56.789 DEBUG - select user0_.id as id1_0_, user0_.name as name2_0_ from users user0_ where user0_.name=?
12:34:56.790 TRACE - binding parameter [1] as [VARCHAR] - [John Doe]
You can now see the exact SQL and the bind parameter John Doe
in place of the ?
.
Code Snippets: Logging Spring Data JPA Repository Methods
Now, let's ground the discussion with a practical example. Here is a simple UserRepository
in a Spring Boot application that extends JpaRepository
:
public interface UserRepository extends JpaRepository<User, Long> {
List<User> findByName(String name);
}
The findByName
method translates into a SQL query behind the scenes. By enabling detailed logging as described above, you can see that query along with the parameter value when the method is called.
Why?
So why is it important to understand the exact queries and parameters Hibernate uses? It comes down to clarity and performance. By seeing the raw SQL, you can:
- Catch Errors: Spot issues like an unintentionally complex query or a missing index.
- Optimize Performance: Observe and optimize queries for better performance, like avoiding N+1 select issues.
- Understand Behavior: Gain insights into Hibernate's inner workings, understanding how JPQL/HQL is translated to SQL.
- Debug Efficiently: Quickly diagnose issues when the code's behavior doesn't match your expectations.
Caveats and Considerations
While printing Hibernate SQL in the logs is incredibly useful during development and debugging, consider the following before using it in production:
- Sensitive Data: SQL logs can contain sensitive information. Ensure they are not exposed.
- Performance Impact: Logging can affect performance; extra caution is required when enabling it in production.
- Log Management: Ensure proper log management practices are in place to handle the increased log volume.
Conclusion
Mastering Hibernate and JPA in a Spring Boot context is a key skill for Java developers. Being able to see the SQL Hibernate is executing can demystify what's happening in your application and is an indispensable tool for debugging and optimization. Experiment with the settings described above and gain the clarity you need to confidently manage your data layer.
Remember, having the ability to unveil the veil of ORM and peek into the actual SQL is a power that comes with responsibility. Use it wisely, use it well, and happy coding!
For further guidance on Hibernate, Spring Boot, and other JPA-related topics, the official Spring Data JPA documentation and the Hibernate User Guide are excellent resources.
Would you like to delve deeper into other aspects of Java, Hibernate, or Spring Boot? Stay tuned for more insightful posts that could help unravel more complexities and enhance your applications.