Quasar vs Akka: Battle of the JVM Concurrency Giants
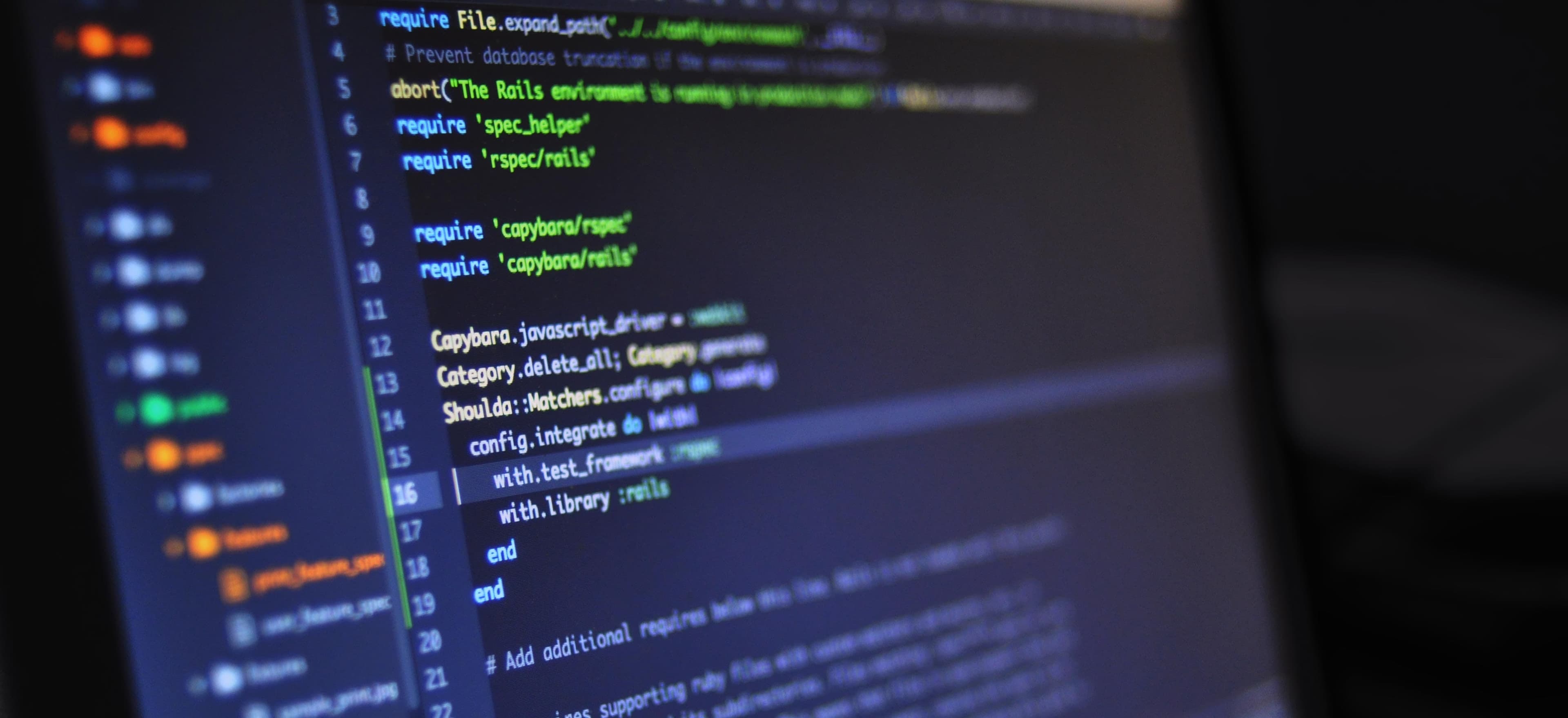
- Published on
Quasar vs Akka: Battle of the JVM Concurrency Giants
In the realm of concurrent programming on the Java Virtual Machine (JVM), two heavyweights stand out for their ability to handle massive concurrency with grace and efficiency: Quasar and Akka. Both are designed to make it easier to write concurrent, scalable applications, but they take different approaches to tackle the challenge. In this blog post, we'll compare Quasar and Akka to see how they stack up against each other, and we'll provide you with some insights that can help you choose the right tool for your next project.
Understanding Concurrency on the JVM
Before we dive into Quasar and Akka, let's briefly touch on concurrency in the context of the JVM. Concurrency allows for executing multiple tasks in an overlapping manner, which is essential for applications that need to handle numerous tasks simultaneously without waiting for each one to complete sequentially. The traditional approach to concurrency on the JVM involves using threads, but thread management can be complex and resource-intensive, leading to the popularity of frameworks that abstract these complexities.
Quasar: Lightweight Threads and Fibers
Quasar provides true lightweight threads, called fibers, which can be spawned in the millions without the heavy resource footprint typically associated with native threads. Fibers in Quasar are implemented at the library level and scheduled by the Quasar runtime. This allows for writing highly concurrent code that looks synchronous while running efficiently in an asynchronous fashion.
Why Choose Quasar?
- Lightweight Concurrency: Quasar's fibers are extremely lightweight, meaning you can have a much larger number of concurrent tasks compared to traditional Java threads.
- Simple Programming Model: Quasar allows you to write code that appears blocking but is actually non-blocking, thanks to its fiber-blocking mechanisms.
- Integration with Existing Libraries: Quasar can work with existing Java libraries that use standard blocking I/O operations without alterations.
Here's an example of how to create and run a fiber in Quasar:
import co.paralleluniverse.fibers.Fiber;
import co.paralleluniverse.fibers.SuspendExecution;
public class QuasarExample {
public static void main(String[] args) {
new Fiber<Void>("SampleFiber", () -> {
try {
// Simulate some work with sleep
Fiber.sleep(1000);
System.out.println("Quasar Fiber Running");
} catch (SuspendExecution | InterruptedException e) {
e.printStackTrace();
}
}).start();
}
}
In this example, we define a fiber that simulates some work using Fiber.sleep
. The start
method triggers the execution of the fiber.
Akka: Actor Model and Message Passing
On the other hand, Akka is a toolkit and runtime for building highly concurrent, distributed, and resilient message-driven applications on the JVM. Akka implements the Actor Model, wherein each actor is an encapsulated unit of computation that communicates with other actors through message passing. Actors in Akka can be distributed across a cluster, making it a great choice for distributed systems.
Why Choose Akka?
- Decentralized Concurrency: The Actor Model encourages designing systems with no central bottleneck. Each actor processes messages independently.
- Fault Tolerance: Akka's "let it crash" philosophy means that actors can be automatically restarted in case of failure, leading to more resilient systems.
- Distributed by Design: Akka has built-in support for distributed computing, making it easier to scale out your application.
Below is a simple example of creating an actor and sending messages in Akka:
import akka.actor.{Actor, ActorSystem, Props}
class GreetingActor extends Actor {
def receive: Receive = {
case "hello" => println("Hello from Akka Actor!")
}
}
object AkkaExample {
def main(args: Array[String]): Unit = {
val system = ActorSystem("GreetingSystem")
val greeter = system.actorOf(Props[GreetingActor], name = "greeter")
greeter ! "hello"
system.terminate()
}
}
In this Scala snippet (since Akka is more idiomatic to Scala), we define GreetingActor
, which reacts to receiving a "hello" message by printing a greeting. The actor system is started, the actor is created, and a message is sent to it.
Comparison: Quasar vs Akka
When comparing Quasar and Akka, there are several factors to consider, namely, the programming model, performance, scalability, and ease of use.
Programming Model
- Quasar offers a straightforward transition for developers familiar with synchronous and blocking code. It allows them to write asynchronous, scalable code without changing much of their programming style.
- Akka's Actor Model requires a paradigm shift as it revolves around message passing, making it inherently suitable for distributed systems and microservices.
Performance
- Quasar shines in scenarios where you have a massive number of small, fast tasks that would be cumbersome to handle with traditional Java threads.
- Akka's performance is more about efficient message processing between actors and less about minimizing the overhead of individual tasks.
Scalability
- For Quasar, scalability is achieved within a single JVM instance by allowing many fibers to run on a limited number of threads.
- Akka not only scales within a single JVM but also across multiple nodes in a cluster, making it a powerful choice for large, distributed applications.
Ease of Use
- Quasar might be easier to grasp for developers with a strong background in synchronous, imperative programming.
- Akka may have a steeper learning curve due to the Actor Model, but it comes with extensive documentation and a strong community, especially within the Scala ecosystem.
Making Your Choice
When to pick Quasar:
- You need massive concurrency but not necessarily a distributed system.
- Your team is more comfortable with Java's traditional thread-based concurrency model.
- You want to leverage existing Java blocking I/O libraries.
Opt for Akka when:
- You are building a distributed system or microservices where actors can reside on different nodes.
- You want to embrace the Actor Model for better fault tolerance and message-driven architecture.
- Your team is open to or experienced with the Scala programming language.
Both Quasar and Akka are robust concurrency frameworks that offer unique advantages. Your choice will largely depend on the specific needs of your project and the expertise of your development team. Whether you choose the lightweight threads of Quasar or the message-driven Actors of Akka, both will enable you to write concurrent, scalable applications on the JVM with greater ease than ever before.
When evaluating these tools, consider experimenting with small prototypes to get a feel for their programming models and concurrency handling. Remember, each has its strengths, and the best choice is the one that aligns with your application's requirements and your team’s skills. Happy coding!
Checkout our other articles