Master Newest Spring Shell: Overcome Upgrade Issues
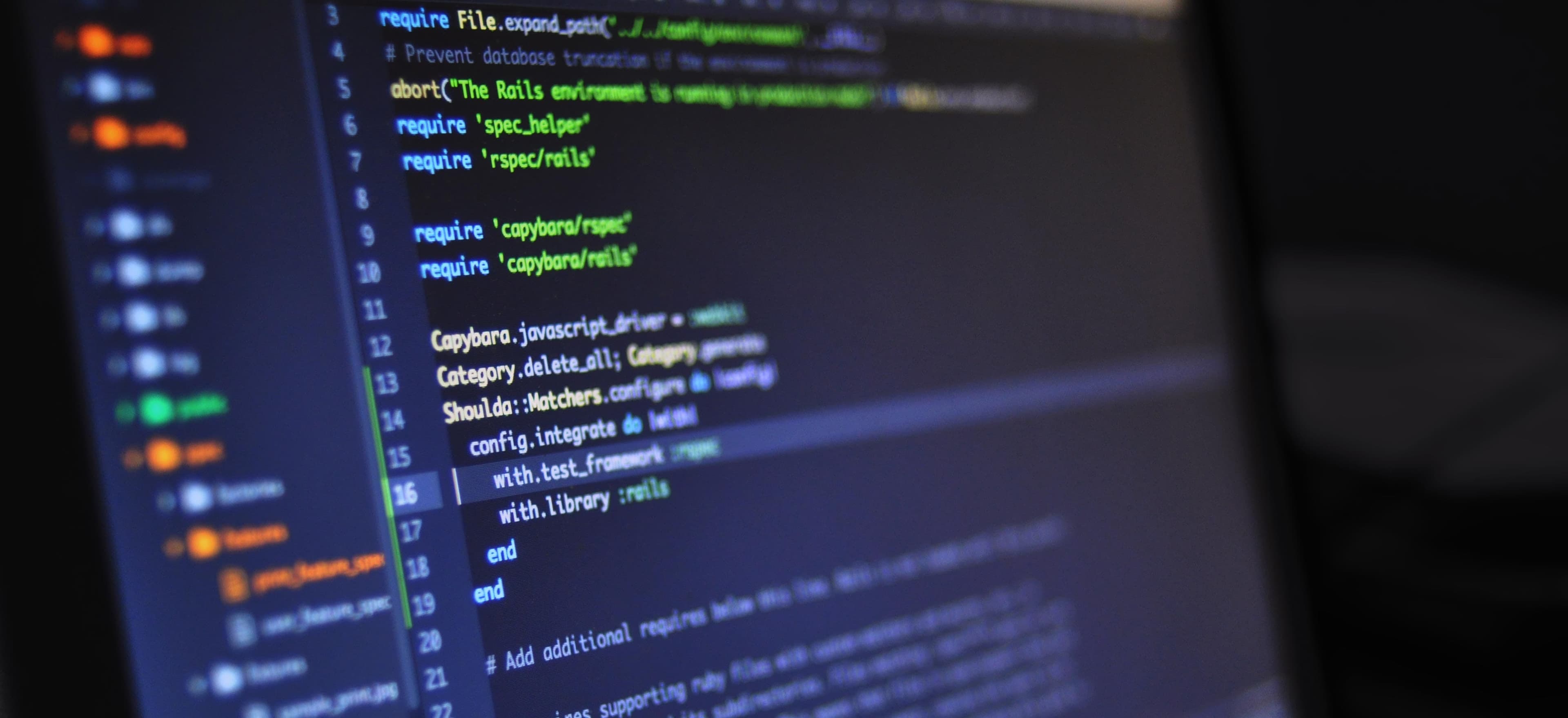
- Published on
Master Newest Spring Shell: Overcome Upgrade Issues
Upgrading to the latest version of a framework can be a thrilling journey. It's like stepping foot onto a new, unexplored territory equipped with better tools and mechanisms to improve your software craftsmanship. The Spring Shell project is no exception. With every update, the team provides new features and functionalities that make creating interactive shell applications in Spring easier and more efficient.
But with new territory comes new challenges. Upgrading often brings along a set of issues that developers need to overcome to reap the benefits of the latest enhancements fully. This post will walk you through the must-know tips and troubleshooting techniques to master the newest Spring Shell and overcome those upgrade obstacles. Whether you're facing compatibility problems or configuration headaches, we have you covered.
Understanding Spring Shell
Spring Shell is an extension of the Spring framework that facilitates the creation of interactive command-line applications. It is designed with simplicity in mind, providing a foundation for building a comprehensive CLI (Command Line Interface) application that integrates seamlessly with your Spring Boot projects.
Before you dive into the upgrade process, familiarize yourself with the current version of Spring Shell. You can find the latest documentation here.
Preparing for Upgrade
Start by reviewing the release notes of the new version. This will provide you with details about new features, bug fixes, and any breaking changes that could impact your existing application.
Ensure your codebase has a good suite of automated tests. These tests will be crucial in identifying issues directly caused by the upgrade.
Common Upgrade Issues and Solutions
Dependency Management
When upgrading Spring Shell, pay close attention to the dependencies in your application. Incompatible version pairings between Spring Boot and Spring Shell can lead to unexpected behavior.
Here is how you can declare dependency to the newest Spring Shell:
<dependency>
<groupId>org.springframework.shell</groupId>
<artifactId>spring-shell-starter</artifactId>
<version>LATEST_VERSION_HERE</version>
</dependency>
Always replace LATEST_VERSION_HERE
with the latest version you want to use.
Deprecated Features
With major upgrades come deprecations. It’s essential to address these to avoid future compatibility issues. Search for @Deprecated
annotations in your code and consult the upgrade guide on how to replace these features.
Configuration Adaptation
You may need to modify the configurations defined in your application.properties
or application.yml
files. Look for any properties that have been renamed or removed and adjust accordingly.
API Changes
Review notable API changes that may affect your application's existing commands. Update your methods and classes to align with the new API definitions.
For example, if there's a change in the way commands are registered, ensure you modify your command beans appropriately.
Code Example of Command Registration
Here's a simple command registration before and after the upgrade:
// Before upgrade
@ShellComponent
public class MyCommands {
@ShellMethod("Say hello")
public String sayHello() {
return "Hello, World!";
}
}
// After upgrade, assuming registration has changed
@ShellComponent
public class MyCommands {
// Assuming the newer version requires new annotations or mechanisms
@NewShellMethod("Say hello")
public String sayHello() {
return "Hello, World!";
}
}
This example assumes a hypothetical change where @ShellMethod
is updated to @NewShellMethod
in the newer version. This is illustrative only—check the actual documentation for the correct changes.
Error Handling
New versions can introduce different error handling mechanisms. Be prepared to handle exceptions differently if needed. Update your exception handlers to conform to the new project philosophy, which might allow for more detailed error reports to your users.
Testing with the New Version
One of the most effective ways to resolve issues is by thorough testing. Write unit tests, integration tests, and employ End-to-End testing to ensure that all components of your application behave as expected.
For a smooth testing phase, consider the following stub:
@SpringBootTest(properties = {"spring.shell.interactive.enabled=false"})
public class MyCommandsTest {
@Autowired
private Shell shell;
@Test
public void testCommand() {
Object result = shell.evaluate(() -> "say-hello");
assertEquals("Hello, World!", result);
}
}
This test disables interactive mode and simulates the input of the "say-hello" command, expecting "Hello, World!" to be returned.
Logging and Troubleshooting
Enhance your logging to capture more details during the upgrade process. With more information at hand, you can track down the source of any issues that surface.
Remember to use the appropriate logging level to avoid log bloat:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MyCommands {
private static final Logger logger = LoggerFactory.getLogger(MyCommands.class);
public String problematicCommand() {
try {
// ... command logic ...
} catch (Exception e) {
logger.error("An error occurred during command execution", e);
}
return "Command finished with an error";
}
}
Community Support
Leverage the power of the community. If you encounter a problem, it’s likely that someone else has faced it too. Engage with communities on Stack Overflow, GitHub, or the official Spring forums.
Summary
Upgrading to the newest Spring Shell version does not need to be daunting. By carefully preparing, addressing known issues, testing comprehensively, and utilizing community wisdom, you can overcome the upgrade challenges and leverage the power of the latest advancements in the framework.
Keep your project's dependencies up to date, embrace the improvements, and continuously learn. Your command-line applications will be more powerful, user-friendly, and maintainable than ever before, as you align with the robust architecture Spring Shell has to offer.
Remember, staying current is critical in the rapidly evolving landscape of software development. Happy coding!
Checkout our other articles