Speed Up Code Reviews: Tree-Sitter for Light Linting
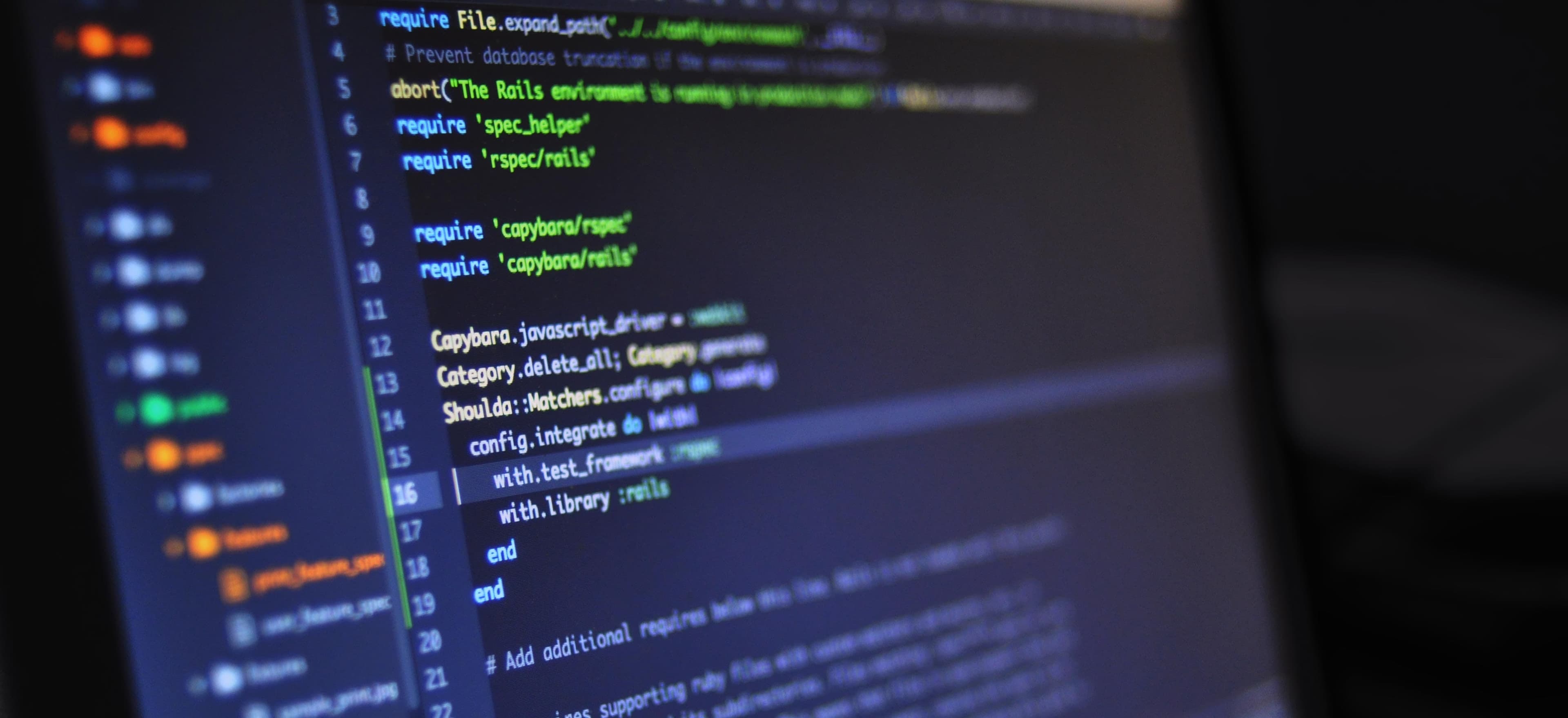
- Published on
Speed Up Code Reviews: Tree-Sitter for Light Linting
Code reviews are a critical aspect of the software development lifecycle, enhancing code quality and fostering team collaboration. However, they can often become burdensome and time-consuming when reviewers have to manually identify syntax errors or styling issues. This is where automation tools, such as linters, can drastically improve the efficiency of code reviews. One such tool that has been gaining popularity is Tree-Sitter, which offers a way to perform light linting on codebases. Here, we will explore how Tree-Sitter can be used to streamline code reviews in Java projects.
Understanding Tree-Sitter
Tree-Sitter is a parsing library that generates syntax trees for source code in various languages and can be used to build powerful text editing and analysis features. It is language-agnostic and runs in realtime, allowing it to be used for syntax highlighting, code navigation, and of course, light linting purposes.
Why Choose Tree-Sitter for Linting?
Linting tools traditionally analyze code to flag programming errors, bugs, stylistic errors, and suspicious constructs. However, most linters come with a fixed set of rules and can sometimes be cumbersome to configure. Tree-Sitter, on the other hand, is highly flexible in its approach. It allows developers to craft rules that pertain specifically to their codebase's requirements and can work in tandem with existing linters to provide an additional layer of quick syntax checks.
Integrating Tree-Sitter in Your Java Project
To get started with using Tree-Sitter for light linting in your Java project, you need to first integrate it. Here is a simple guide:
1. Install Tree-Sitter
Install Tree-Sitter using your favorite package manager or build it from the source. If you are using npm:
npm install tree-sitter
npm install tree-sitter-java
2. Initialize and Parse the Code
With Tree-Sitter installed, you can now write a script to parse your Java code. The following example shows how you can initialize Tree-Sitter and run it against your Java files.
import org.tree_sitter.TreeSitter;
public class TreeSitterExample {
public static void main(String[] args) {
// Initialize Tree-Sitter and the Java language parser
TreeSitter treeSitter = new TreeSitter();
Language javaLanguage = Language.load("path/to/tree-sitter-java.wasm");
treeSitter.setLanguage(javaLanguage);
// Parse a Java file
Node rootNode = treeSitter.parseString(null, "public class Main { ... }");
System.out.println(rootNode.toString());
// Traverse the syntax tree or perform linting as needed
// ...
}
}
In the code snippet above, we use Tree-Sitter to parse a Java code sample. The method parseString
is used to parse the contents of a Java file and create a syntax tree from it.
3. Define Custom Light Linting Rules
Once your project’s files are being parsed by Tree-Sitter, you can create custom linting rules. You can walk through the syntax tree and analyze different nodes to enforce coding standards or detect anti-patterns.
public void lintMethodLength(Node rootNode) {
// Walk through the syntax tree nodes to find method declarations
// ...
for (Node methodNode : methodNodes) {
// Check the length of the method
if (methodNode.endByte - methodNode.startByte > SOME_MAX_LENGTH) {
System.out.println("Method is too long: " + methodNode);
// Provide suggestions or fixes
// ...
}
}
}
The above example demonstrates a simple rule that lints methods to check if they exceed a certain length, which is often a common code style check.
Speeding Up Code Reviews
With Tree-Sitter in place doing light linting, reviewers can expect to find many issues pre-flagged, allowing them to focus more on the logic and design of the code rather than the syntax and style. Tree-Sitter does this efficiently in real-time, meaning that the feedback loop for developers is much faster compared to traditional linting processes.
Best Practices with Tree-Sitter Linting
- Integrate with Continuous Integration (CI): Including Tree-Sitter as a step in your CI pipeline ensures that light linting occurs automatically with every commit, keeping your codebase clean.
- Custom Rules for Project Specifics: Use Tree-Sitter's ability to create custom rules that enforce conventions specific to your project's needs.
- Combine with Other Linters: Tree-Sitter doesn't have to replace existing linters; it can complement them by handling cases that are outside the scope or tricky to implement in conventional linters.
Conclusion
Tree-Sitter provides an innovative way of ensuring code quality and consistency through its real-time parsing and easy-to-define linting rules. Integrating Tree-Sitter into your Java project can enhance the code review process by automating the detection of syntax and stylistic issues. By combining it with the power of customized linting rules and other automation tools, developers and reviewers can make the most out of their code reviews, focusing their attention on what truly matters for delivering high-quality software.
Tree-Sitter bridges the gap between complex static code analysis and real-time linting to provide immediate feedback to developers, ultimately speeding up the code review process. By investing some time into setting up and defining rules specific to your needs, you not only maintain a healthier codebase but also support a more efficient and productive development workflow.
Remember that a tool like Tree-Sitter is meant to aid human reviewers, not replace them. While it can catch many issues early in the development cycle, the value of human insight and experience cannot be overlooked during code reviews. Keep a balance between automated checks and manual oversight to achieve the best code quality.
Checkout our other articles