Harnessing the Power of Java Servlets and JSP Tags for Rapid Java Web App Development
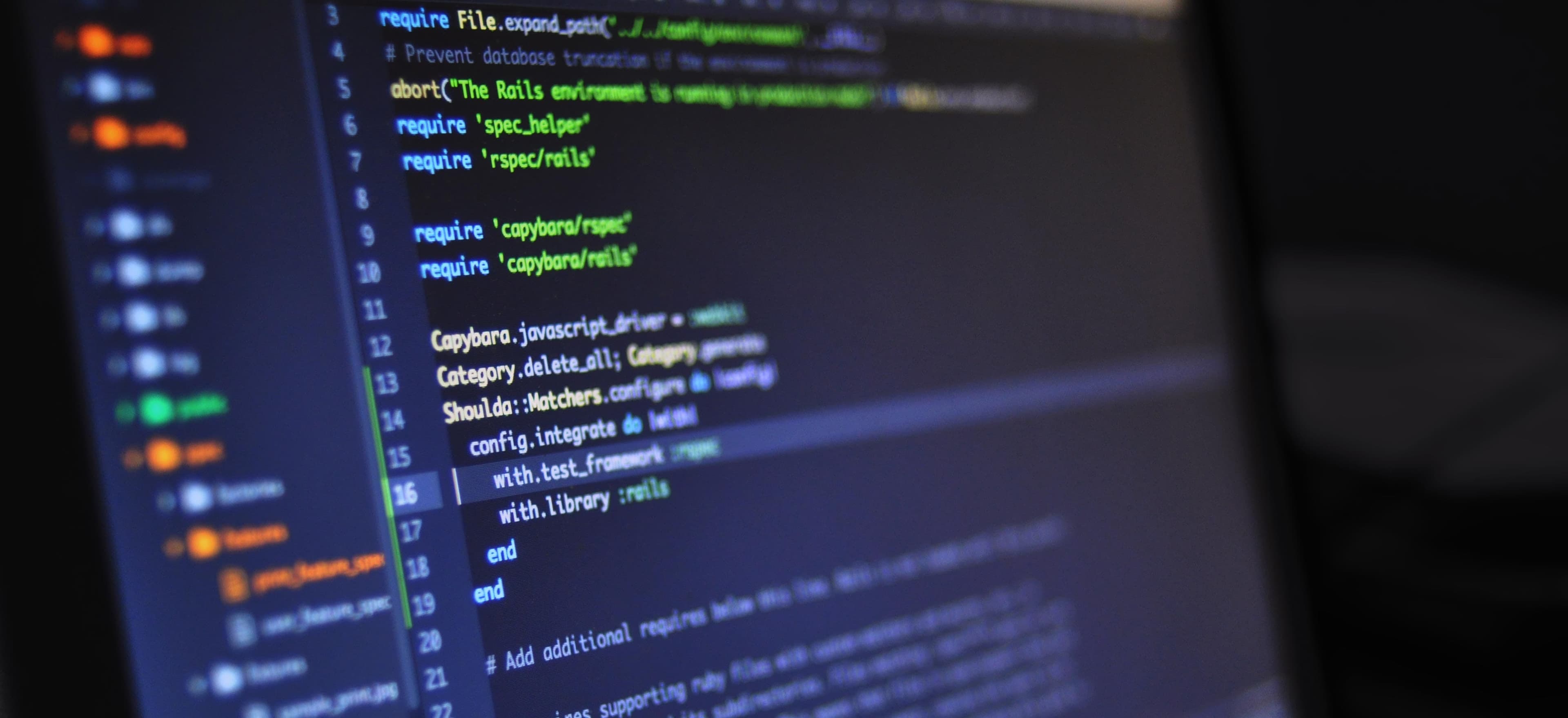
- Published on
In the world of web application development, speed and efficiency are key. Developers are constantly looking for tools and technologies that can help them build robust and scalable applications in less time. One such technology is Java Servlets and JSP (JavaServer Pages) tags, which provide a powerful framework for creating dynamic web pages and handling client requests.
Java Servlets are the foundation of Java web application development. They are small, platform-independent Java classes that run on a web server, processing client requests and generating dynamic responses. Servlets provide a way to handle HTTP requests and perform tasks such as database access, form handling, and session management.
JSP, on the other hand, is a technology that allows Java code to be embedded in HTML pages. It provides a way to separate the presentation logic from the business logic, making web application development more maintainable and scalable. JSP tags, also known as JSTL (JavaServer Pages Standard Tag Library), are a set of pre-defined tags that can be used to perform common tasks in web applications, such as looping through collections, formatting dates, and manipulating strings.
The combination of Java Servlets and JSP tags offers a powerful and efficient way to develop Java web applications. Here are some of the benefits:
-
Reusability: Servlets and JSP tags can be reused across multiple pages and applications, reducing duplication of code and improving maintainability. For example, if you have a form validation logic that needs to be performed on multiple pages, you can encapsulate it in a servlet or JSP tag and reuse it wherever required.
-
Separation of concerns: With Servlets and JSP tags, you can separate the presentation logic from the business logic. The HTML code can be written in JSP pages, while the Java code can be written in Servlets and JSP tags. This separation makes the code more modular and easier to maintain.
-
Rapid development: Java Servlets and JSP tags provide a high level of abstraction, allowing developers to focus on the business logic rather than low-level details. This enables rapid development of web applications without sacrificing flexibility or performance.
-
Robustness: Servlets and JSP tags are part of the Java EE (Enterprise Edition) platform, which provides a robust and scalable environment for building enterprise applications. Java EE offers features such as transaction management, security, and clustering, which can be easily integrated into web applications developed using Servlets and JSP tags.
To illustrate the power of Servlets and JSP tags, let's consider an example of a simple registration form for a web application. The form collects user information such as name, email, and password, and validates the input before storing it in a database.
First, we need to create a servlet to handle the form submission and perform the validation. Here is a sample code snippet:
@WebServlet("/register")
public class RegistrationServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// Retrieve form data from request
String name = request.getParameter("name");
String email = request.getParameter("email");
String password = request.getParameter("password");
// Validate form data
boolean isValid = true;
if (name.isEmpty()) {
isValid = false;
request.setAttribute("nameError", "Name is required");
}
if (email.isEmpty()) {
isValid = false;
request.setAttribute("emailError", "Email is required");
}
if (password.isEmpty()) {
isValid = false;
request.setAttribute("passwordError", "Password is required");
}
// If form data is valid, save it to the database
if (isValid) {
// Save data to the database
// ...
// Redirect to a success page
response.sendRedirect("success.jsp");
} else {
// Forward to the registration page with error messages
request.getRequestDispatcher("register.jsp").forward(request, response);
}
}
}
In this servlet, we retrieve the form data from the request using the getParameter()
method. We then perform the validation and set appropriate error messages using the setAttribute()
method. If the form data is valid, we save it to the database and redirect the user to a success page. Otherwise, we forward the user back to the registration page with the error messages.
Next, let's create a JSP page to display the registration form and handle the error messages. Here is a sample code snippet:
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<!DOCTYPE html>
<html>
<head>
<title>Registration Form</title>
</head>
<body>
<h1>Registration Form</h1>
<form action="register" method="post">
<label for="name">Name:</label>
<input type="text" name="name" id="name">
<span style="color: red;"><c:out value="${nameError}"/></span><br>
<label for="email">Email:</label>
<input type="text" name="email" id="email">
<span style="color: red;"><c:out value="${emailError}"/></span><br>
<label for="password">Password:</label>
<input type="password" name="password" id="password">
<span style="color: red;"><c:out value="${passwordError}"/></span><br>
<input type="submit" value="Register">
</form>
</body>
</html>
In this JSP page, we use JSP tags to display the error messages returned by the servlet. The <c:out>
tag is used to output the value of the error message attribute. For example, the expression ${nameError}
outputs the value of the nameError
attribute set in the servlet.
By combining Servlets and JSP tags, we have created a simple yet powerful web application that handles form submission and validation. This example demonstrates the speed and efficiency of using Java Servlets and JSP tags for rapid web application development.
In conclusion, Java Servlets and JSP tags provide a robust and efficient framework for developing Java web applications. They offer reusability, separation of concerns, rapid development, and robustness. By harnessing the power of these technologies, developers can build scalable and maintainable web applications in less time. So, if you're looking to accelerate your Java web app development, give Java Servlets and JSP tags a try!
References:
- Java Servlet Specification
- JavaServer Pages Technology Overview