Creating SVG barcodes using ZXing and jfreeSVG in Java
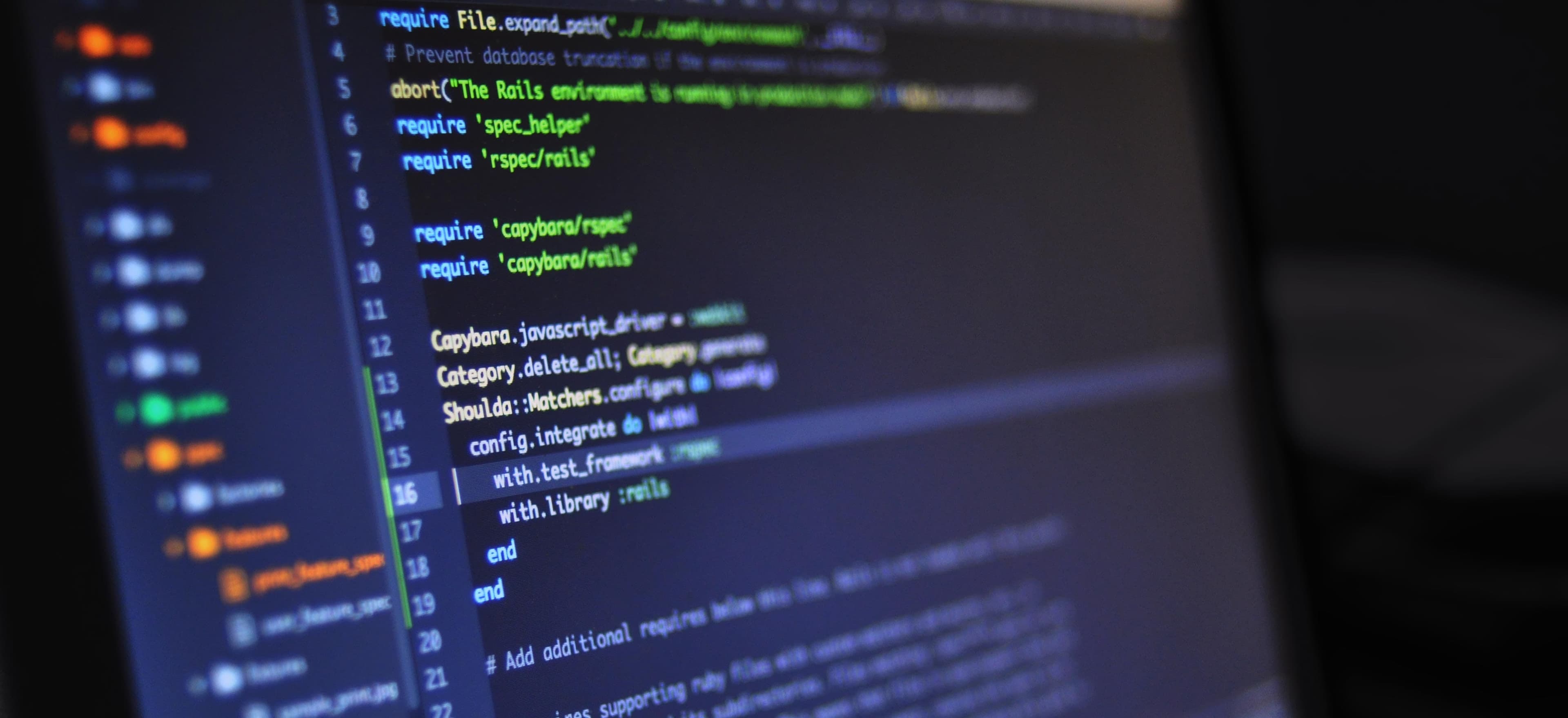
- Published on
Barcodes are widely used in various applications, from retail to logistics, to track and identify products. In Java, there are several libraries available to generate barcodes, and in this article, we will look at how to create SVG barcodes using ZXing and jfreeSVG.
ZXing is a popular open-source barcode scanning and generation library in Java. It supports a wide range of barcode formats, including QR codes, UPC codes, and more. On the other hand, jfreeSVG is a lightweight Java library that allows you to generate SVG (Scalable Vector Graphics) images.
By combining these two libraries, we can easily create SVG barcodes that can be scaled without any loss of quality, making them ideal for various applications. Let's dive into the details of how to do this.
First, we need to set up our project by adding the necessary dependencies. You can use Maven or Gradle to manage your project dependencies. Add the following dependencies to your pom.xml
file if you are using Maven:
<dependencies>
<dependency>
<groupId>com.google.zxing</groupId>
<artifactId>core</artifactId>
<version>3.4.1</version>
</dependency>
<dependency>
<groupId>org.jfree</groupId>
<artifactId>jfreesvg</artifactId>
<version>3.5</version>
</dependency>
</dependencies>
Once you have set up the project, let's look at an example of generating an SVG barcode using ZXing and jfreeSVG.
import com.google.zxing.BarcodeFormat;
import com.google.zxing.EncodeHintType;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.oned.Code128Writer;
import com.google.zxing.qrcode.decoder.ErrorCorrectionLevel;
import org.jfree.graphics2d.svg.SVGGraphics2D;
import org.jfree.graphics2d.svg.SVGUtils;
import java.awt.*;
import java.io.File;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
public class SVGBarcodeGenerator {
public static void main(String[] args) {
String barcodeData = "1234567890"; // The data to be encoded in the barcode
// Set the barcode format and encoding hints
BarcodeFormat barcodeFormat = BarcodeFormat.CODE_128;
Map<EncodeHintType, Object> hints = new HashMap<>();
hints.put(EncodeHintType.ERROR_CORRECTION, ErrorCorrectionLevel.L);
// Create the barcode writer and encode the data
Code128Writer writer = new Code128Writer();
BitMatrix bitMatrix = writer.encode(barcodeData, barcodeFormat, 200, 80, hints);
// Create an SVGGraphics2D object to draw the barcode
SVGGraphics2D svgGraphics = new SVGGraphics2D(200, 80);
svgGraphics.setBackground(Color.WHITE);
svgGraphics.clearRect(0, 0, 200, 80);
// Set the rendering hints
RenderingHints hints = new RenderingHints(
RenderingHints.KEY_ANTIALIASING,
RenderingHints.VALUE_ANTIALIAS_ON);
svgGraphics.setRenderingHints(hints);
// Set the barcode color and draw the barcode on the SVGGraphics2D object
svgGraphics.setColor(Color.BLACK);
for (int x = 0; x < bitMatrix.getWidth(); x++) {
for (int y = 0; y < bitMatrix.getHeight(); y++) {
if (bitMatrix.get(x, y)) {
svgGraphics.fillRect(x, y, 1, 1);
}
}
}
// Save the barcode as an SVG file
try {
File file = new File("barcode.svg");
SVGUtils.writeToSVG(file, svgGraphics.getSVGElement());
System.out.println("Barcode saved to: " + file.getAbsolutePath());
} catch (IOException e) {
e.printStackTrace();
}
}
}
Let's go through the code step by step to understand how it works.
- Firstly, we define the data to be encoded in the barcode, in this case, the string "1234567890".
- Next, we set the barcode format to
CODE_128
and the error correction level toL
. These are encoding hints that we pass to the barcode writer. - We create an instance of the
Code128Writer
class from ZXing to encode the data into aBitMatrix
, specifying the barcode width and height (200x80 in this example). - Then, we create an instance of
SVGGraphics2D
from jfreeSVG, with the same dimensions as the barcode. We set the background color to white and clear the graphics area. - We enable antialiasing for smooth rendering by setting the rendering hints.
- Next, we set the barcode color to black and iterate over each pixel in the
BitMatrix
. If the pixel is set (true), we draw a filled rectangle at that position on the SVGGraphics2D object. - Finally, we save the barcode as an SVG file using the
SVGUtils.writeToSVG()
method provided by jfreeSVG.
When you run the code, you will get a barcode.svg file in the project directory. Open it in a browser or any SVG viewer to see the generated barcode.
This is just a basic example of generating a barcode using ZXing and jfreeSVG. You can customize the barcode appearance by changing the color, font, and other properties. Additionally, ZXing supports various barcode formats, such as QR codes, UPC codes, and more. You can explore the ZXing documentation for more information on barcode formats and customization options.
To learn more about ZXing and jfreeSVG, you can refer to the following resources:
- ZXing Documentation
- jfreeSVG Documentation
In conclusion, generating SVG barcodes in Java is a straightforward task with the help of libraries like ZXing and jfreeSVG. You can integrate barcode generation into your Java applications to create scalable and high-quality barcodes suitable for a variety of use cases.
Checkout our other articles